A Comprehensive Guide to Thread Pools in Java
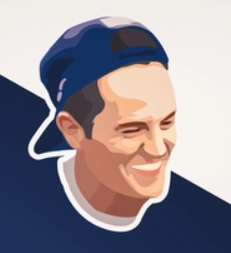
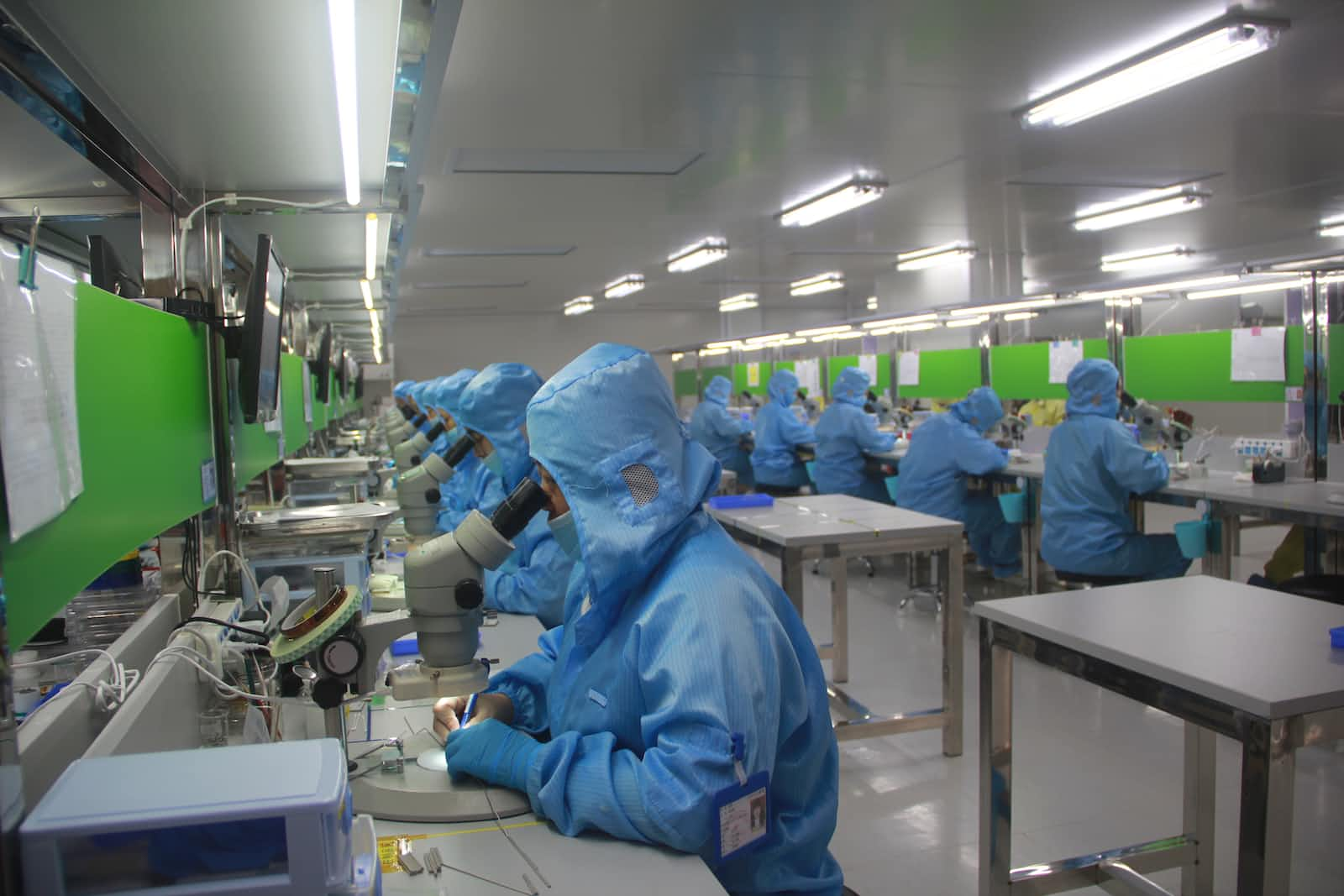
In the world of concurrent programming, managing threads efficiently is a challenge that developers often face. Threads are essential for executing tasks concurrently, but creating and managing threads can be resource-intensive and complex. This is where thread pools, provided by the ExecutorService framework in Java, come to the rescue. In this blog, we will explore what thread pools are, and why they are needed, provide a basic example, and delve into different types of thread pools offered by ExecutorService.
What is a Thread Pool?
A thread pool is a managed collection of threads that are maintained by the system to execute tasks concurrently. It's like having a team of workers ready to perform various tasks without the overhead of creating and destroying threads for each task. Threads in a thread pool can be reused, which saves time and resources compared to creating a new thread for each task.
In simpler terms, suppose we need to add 100 records to a database at the same time. One approach could involve using a loop and within this loop, creating threads 100 times, each responsible for inserting data. However, this method results in generating 100 threads for this task, which is resource-intensive due to the significant cost associated with creating individual threads for the CPU.
A more efficient and viable solution involves establishing a thread pool comprising a fixed number of threads, let's say 10. These 10 threads collaboratively manage the concurrent insertion of records into the database. By adopting this strategy, we eliminate the need to spawn 100 threads for data insertion, accomplishing the task effectively with just 10 threads. And this concept is called Thread Pool.
Why Do We Need Thread Pools?
Creating and managing threads manually can be resource-intensive due to the overhead of thread creation, context switching, and memory allocation. Thread pools address these issues and provide several advantages:
Resource Management: Thread pools limit the number of active threads, preventing excessive resource consumption and potential system overload.
Improved Performance: Reusing threads minimizes the overhead of thread creation and destruction, leading to faster task execution.
Scalability: Thread pools allow you to control the number of threads, ensuring optimal performance regardless of the system's capabilities.
Controlled Concurrency: By specifying the maximum number of threads, you can control the level of concurrency, avoiding situations where too many threads compete for resources.
Load Balancing: Thread pools can distribute tasks evenly among available threads, preventing situations where some threads are overloaded while others remain idle.
A Basic Example of Thread Pool
Let's explore a simple example of using the ExecutorService framework to create a thread pool and submit tasks for execution:
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
public class ThreadPoolExample {
public static void main(String[] args) {
// Create a thread pool with 3 threads
ExecutorService executor = Executors.newFixedThreadPool(3);
// Submit tasks for execution
for (int i = 0; i < 10; i++) {
int taskId = i;
executor.execute(new TaskRunnable(taskId));
}
// Shutdown the thread pool
executor.shutdown();
}
}
class TaskRunnable implements Runnable {
private int taskId;
public TaskRunnable(int taskId) {
this.taskId = taskId;
}
@Override
public void run() {
System.out.println("Task " + taskId + " executed by " + Thread.currentThread().getName());
}
}
In this example, we create a fixed-size thread pool with three threads using Executors.newFixedThreadPool(3)
. We then submit ten tasks for execution using the execute method of the ExecutorService. The thread pool automatically assigns available threads to execute these tasks concurrently.
Types of Thread Pools (ExecutorService)
Java's ExecutorService framework offers several types of thread pools to cater to different use cases:
Fixed Thread Pool: As shown in the example, a fixed thread pool maintains a constant number of threads and executes tasks concurrently. It's suitable when the number of tasks is known and needs to be executed efficiently.
Cached Thread Pool: This type of thread pool adjusts the number of threads dynamically based on the task load. Threads are added or removed as needed. It's ideal for scenarios where tasks have varying execution times.
Single Thread Executor: This pool consists of a single thread and executes tasks sequentially. It's useful for scenarios where tasks must be executed one after another in a specific order.
Scheduled Thread Pool: This pool is designed for scheduling tasks to run at specific intervals or after a delay. It's commonly used for periodic tasks like cleaning up resources or sending regular reports.
Work Stealing Pool: Introduced in Java 8, this pool type supports parallel processing using multiple worker threads that can "steal" tasks from each other's queues, leading to better task distribution and load balancing.
Conclusion
Thread pools, provided by the ExecutorService framework in Java, offer an efficient way to manage and execute tasks concurrently. They alleviate the overhead of manual thread management, provide resource management, and enhance performance and scalability. By understanding the different types of thread pools and their use cases, developers can make informed decisions on selecting the appropriate pool for their applications. Incorporating thread pools into your codebase can lead to more efficient and responsive applications in the world of concurrent programming.
Important blogs:
Subscribe to my newsletter
Read articles from Hemant Besra directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
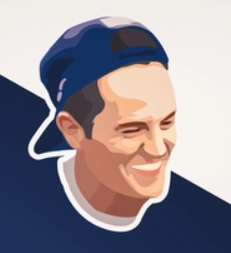
Hemant Besra
Hemant Besra
Experienced Full Stack Java developer. Have Strong Experience in JSP/Servlet, JSF, Jasper Report, Spring Framework, hibernate, Angular 5+, Microservices. Experienced in Front-end technologies such as HTML, CSS, JavaScript, angular 6+, AJAX, JSON, and XML. Strong Hands-on experience on working with Reactive Forms to build form-based application in Angular 6+.