Different Executor Services in Java for Efficient Multithreading
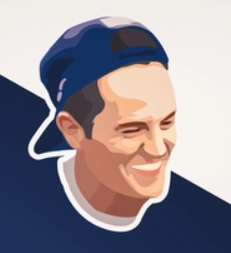
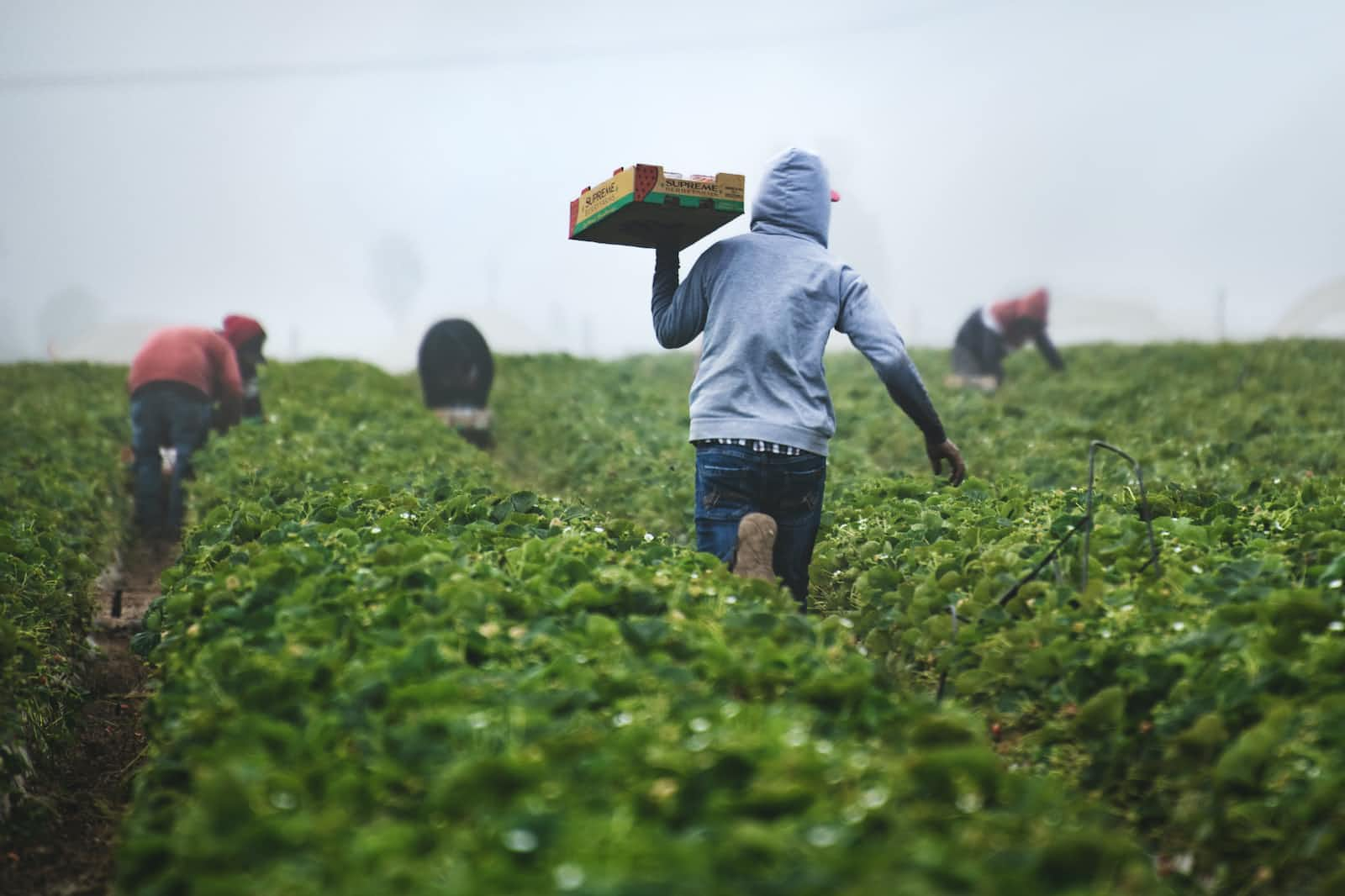
In our earlier blog post, we explained the concept of a thread pool, its significance, and how the ExecutorService framework assists in its implementation. This blog serves as a continuation of our previous discussion. If you haven't read the preceding article, you can access it by clicking here: "A Comprehensive Guide to Thread Pools in Java". This link will direct you to the previous blog for your reference.
Understanding ExecutorService
ExecutorService
is part of the java.util.concurrent
package and offers a high-level API to manage and control the execution of tasks concurrently. It abstracts the complexities of thread management, allowing developers to focus on task logic rather than thread creation and management. Executor services provide a pool of worker threads that can be reused for executing tasks, thus improving performance by eliminating the overhead of frequently creating and destroying threads.
Let's explore different implementations of ExecutorService
, each tailored to different use cases:
1. ThreadPoolExecutor
ThreadPoolExecutor
is a versatile and highly customizable implementation of ExecutorService
. It allows you to define the core pool size, maximum pool size, and other parameters to fine-tune the behavior of the thread pool. This type of executor is suitable when you require precise control over the number of threads and their behavior.
Example:
import java.util.concurrent.*;
public class ThreadPoolExecutorExample {
public static void main(String[] args) {
ExecutorService executorService = new ThreadPoolExecutor(
5, // Core pool size
10, // Maximum pool size
1, TimeUnit.MINUTES, // Keep-alive time
new LinkedBlockingQueue<>() // Task queue
);
// Submit tasks for execution
// ...
executorService.shutdown();
}
}
2. FixedThreadPool
FixedThreadPool
is a simpler variant of ThreadPoolExecutor
where you specify a fixed number of threads in the pool. It's ideal for scenarios where you want to limit the number of concurrent tasks.
Example:
import java.util.concurrent.*;
public class FixedThreadPoolExample {
public static void main(String[] args) {
ExecutorService executorService = Executors.newFixedThreadPool(5);
// Submit tasks for execution
// ...
executorService.shutdown();
}
}
3. CachedThreadPool
CachedThreadPool
is a dynamic implementation that creates new threads as needed and reuses idle threads if available. It's suitable for scenarios where the number of tasks can vary widely.
Example:
import java.util.concurrent.*;
public class CachedThreadPoolExample {
public static void main(String[] args) {
ExecutorService executorService = Executors.newCachedThreadPool();
// Submit tasks for execution
// ...
executorService.shutdown();
}
}
4. SingleThreadExecutor
SingleThreadExecutor
maintains a single thread for executing tasks sequentially. This is useful when you need tasks to be processed in a specific order without concurrency.
Example:
import java.util.concurrent.*;
public class SingleThreadExecutorExample {
public static void main(String[] args) {
ExecutorService executorService = Executors.newSingleThreadExecutor();
// Submit tasks for execution
// ...
executorService.shutdown();
}
}
5. ScheduledExecutorService
The ScheduledExecutorService
interface in Java's java.util.concurrent
package, is designed to execute tasks at specific intervals or after a delay. It's particularly useful for scheduling tasks like periodic data updates, sending emails at regular intervals, or performing cleanup operations.
The Scheduled Thread Pool can create threads that execute tasks periodically, and it also supports scheduling tasks for one-time execution with a specified delay.
Here's a brief overview of how it works:
schedule()
: This method allows you to schedule a task for one-time execution after a specified delay.scheduleAtFixedRate()
: This method schedules a task for periodic execution at a fixed rate. It starts executing the task after the initial delay and then continues at the specified interval.scheduleWithFixedDelay()
: Similar toscheduleAtFixedRate()
, this method schedules a task for periodic execution, but it introduces a delay between the end of the previous execution and the start of the next.
Example:
import java.util.concurrent.Executors;
import java.util.concurrent.ScheduledExecutorService;
import java.util.concurrent.TimeUnit;
public class ScheduledThreadPoolExample {
public static void main(String[] args) {
// Create a ScheduledExecutorService with a pool size of 1
ScheduledExecutorService scheduler = Executors.newScheduledThreadPool(1);
// Schedule a task to run every 2 seconds
Runnable task = () -> {
System.out.println("Task is running at: " + System.currentTimeMillis());
};
int initialDelay = 0;
int period = 2; // seconds
// Schedule the task to run at a fixed rate
scheduler.scheduleAtFixedRate(task, initialDelay, period, TimeUnit.SECONDS);
// Allow the scheduler to execute tasks
try {
Thread.sleep(10000); // Let the scheduler run for 10 seconds
} catch (InterruptedException e) {
e.printStackTrace();
}
// Shutdown the scheduler
scheduler.shutdown();
}
}
/*
Task is running at: 1659984560970
Task is running at: 1659984563972
Task is running at: 1659984566972
Task is running at: 1659984569972
Task is running at: 1659984572973
*/
In this example, we create a ScheduledExecutorService
using the newScheduledThreadPool()
method with a pool size of 1. We then define a task that prints the current time. The scheduleAtFixedRate()
method is used to schedule this task to run every 2 seconds. We let the scheduler run for 10 seconds before shutting it down.
f. ForkJoinPool
(Work Stealing Pool)
ForkJoinPool
is an implementation of ExecutorService
that's particularly suited for tasks that can be divided into smaller subtasks, making it ideal for parallel processing and recursive algorithms. It's often referred to as a "work-stealing pool" because idle threads steal tasks from the queues of other busy threads, ensuring efficient utilization of resources.
import java.util.concurrent.*;
public class ForkJoinPoolExample {
public static void main(String[] args) {
ForkJoinPool forkJoinPool = new ForkJoinPool();
// Submit tasks for execution
// ...
forkJoinPool.shutdown();
}
}
In this example, the ForkJoinPool
is created without specifying the number of threads. It dynamically adapts to the available processors, creating threads as needed.
The "Work Stealing Pool", represented by ForkJoinPool
, is another important implementation of ExecutorService
. It's designed for scenarios where tasks can be divided into subtasks, allowing for efficient parallel processing. By adding this type of executor to our discussion, we ensure a comprehensive overview of the various ExecutorService
implementations available in Java for efficient multithreading.
Conclusion
Java's ExecutorService
framework offers a robust and efficient solution for managing multithreaded tasks. By employing different implementations such as ThreadPoolExecutor
, FixedThreadPool
, CachedThreadPool
, ScheduledExecutorService
, ForkJoinPool,
and SingleThreadExecutor
, you can harness the power of multithreading without the complexities of manual thread management. Choosing the right executor service for your application's requirements can lead to smoother and more efficient multithreading in your Java programs.
Subscribe to my newsletter
Read articles from Hemant Besra directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
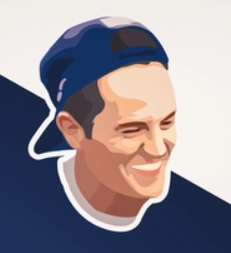
Hemant Besra
Hemant Besra
Experienced Full Stack Java developer. Have Strong Experience in JSP/Servlet, JSF, Jasper Report, Spring Framework, hibernate, Angular 5+, Microservices. Experienced in Front-end technologies such as HTML, CSS, JavaScript, angular 6+, AJAX, JSON, and XML. Strong Hands-on experience on working with Reactive Forms to build form-based application in Angular 6+.