Set Operations and Methods in Python Programming
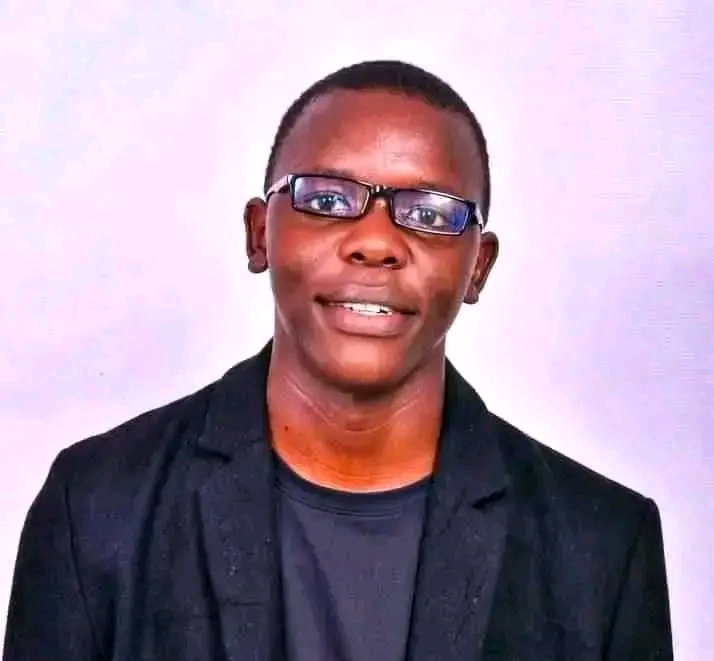
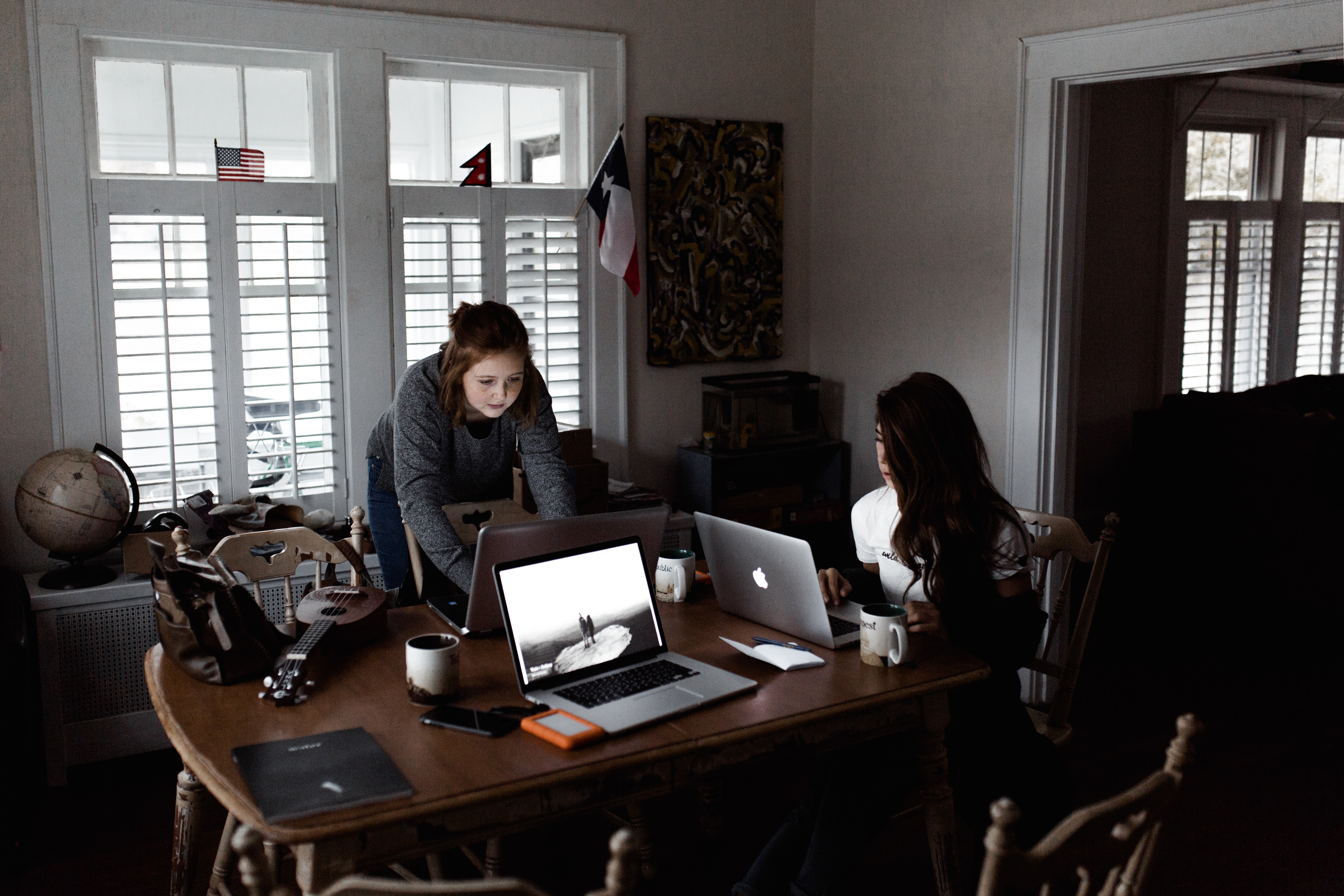
Unleashing the Power of Unordered Collections
Set operations and methods in Python programming provide developers with powerful tools for working with unordered collections of unique elements. Sets are invaluable when you need to perform tasks like eliminating duplicates, testing membership, and conducting various mathematical operations. In this comprehensive article, we'll delve into the intricacies of set operations and methods, exploring their applications, use cases and best practices.
Understanding Sets
A set is an unordered collection of distinct elements enclosed in curly braces {}
. Sets offer two primary advantages: they eliminate duplicate values automatically and provide efficient membership testing.
1. Creating Sets:
Creating sets is straightforward:
fruits = {"apple", "banana", "cherry"}
numbers = {1, 2, 3, 4, 5}
mixed_set = {1, "apple", True, 3.14}
2. Set Operations and Methods:
Python supports a variety of operations and methods for sets.
Set Operations:
Union (
|
): Combines two sets, resulting in a new set containing all unique elements from both sets.Intersection (
&
): Produces a new set containing elements common to both sets.Difference (
-
): Generates a new set with elements present in the first set but not in the second.Symmetric Difference (
^
): Creates a new set containing elements that are present in either of the sets, but not in both.
Set Methods:
Python provides numerous built-in methods for sets, including:
add()
: Adds an element to the set.remove()
: Removes a specific element. Raises an error if the element is not found.discard()
: Removes an element, but doesn't raise an error if the element is not found.pop()
: Removes and returns an arbitrary element from the set.clear()
: Removes all elements from the set.copy()
: Creates a shallow copy of the set.
Set Membership and Subsets:
in
operator: Tests whether an element is present in the set.not in
operator: Tests whether an element is not present in the set.issubset()
: Checks if a set is a subset of another set.issuperset()
: Checks if a set is a superset of another set.
Set Comprehensions:
Similar to list comprehensions, set comprehensions allow you to create sets using concise expressions:
squares = {x**2 for x in range(5)}
Best Practices for Using Set Operations and Methods
To effectively work with set operations and methods, consider these best practices:
1. Choose Sets for Uniqueness:
Use sets when you need to work with collections of unique elements. Sets automatically remove duplicates, ensuring efficient storage and retrieval.
2. Keep Sets Immutable:
Maintain set immutability by not directly modifying the contents. Instead, use set methods to manipulate the data.
3. Set Operations for Data Manipulation:
Set operations like union, intersection, and difference can be particularly useful for data manipulation tasks, such as merging and comparing datasets.
4. Efficient Membership Testing:
Sets excel at membership testing due to their efficient hash-based lookup. Use sets to check if an element exists within a collection quickly.
5. Avoid Indexing:
Sets are unordered, so they do not support indexing. If you require ordered collections with indexing, consider using lists.
6. Consistent Element Types:
While sets can hold various data types, it's generally best to maintain consistent element types within a set for clarity and predictability.
7. Set Comprehensions for Conciseness:
Set comprehensions provide a concise way to generate sets based on expressions. They are particularly useful for creating unique collections from sequences.
Set operations and methods offer an efficient and powerful way to work with collections of unique elements in Python. Sets are essential when you need to eliminate duplicates, perform membership testing, and conduct various mathematical operations. By mastering the concepts and methods associated with sets, developers can create more efficient, readable, and maintainable code. Whether you're working on data manipulation, algorithm design, or any programming task involving unique elements, the ability to leverage set operations and methods will be a valuable skill in your programming journey.
Subscribe to my newsletter
Read articles from Brandon Opere directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
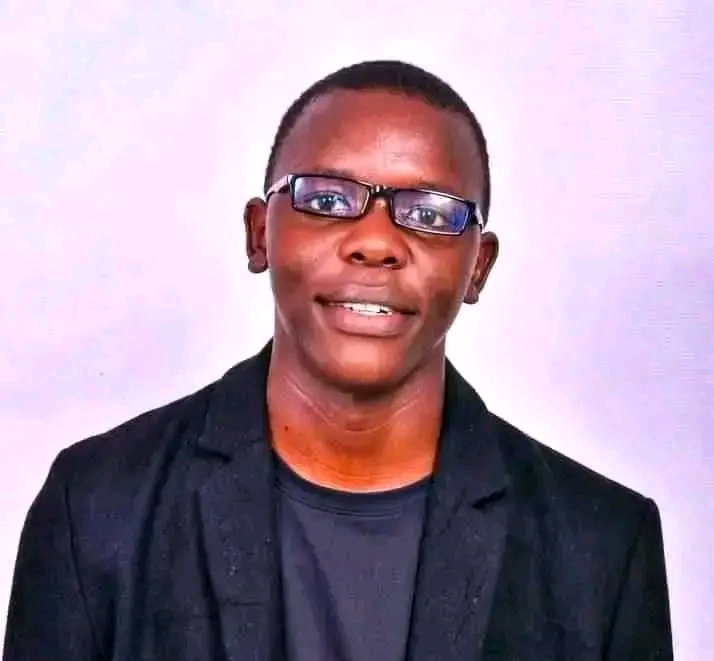
Brandon Opere
Brandon Opere
Building Highly Resilient and Scalable System Softwares.