Interface Segragation Principle (ISP)
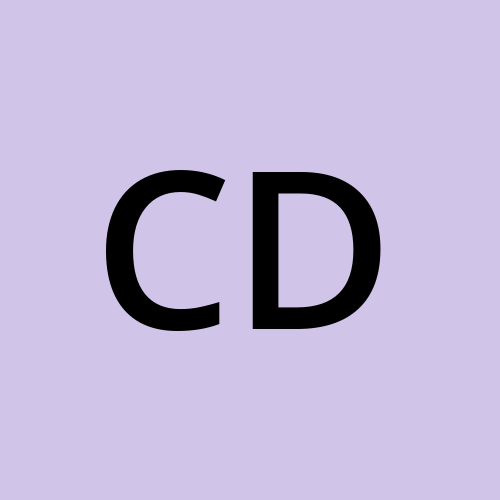
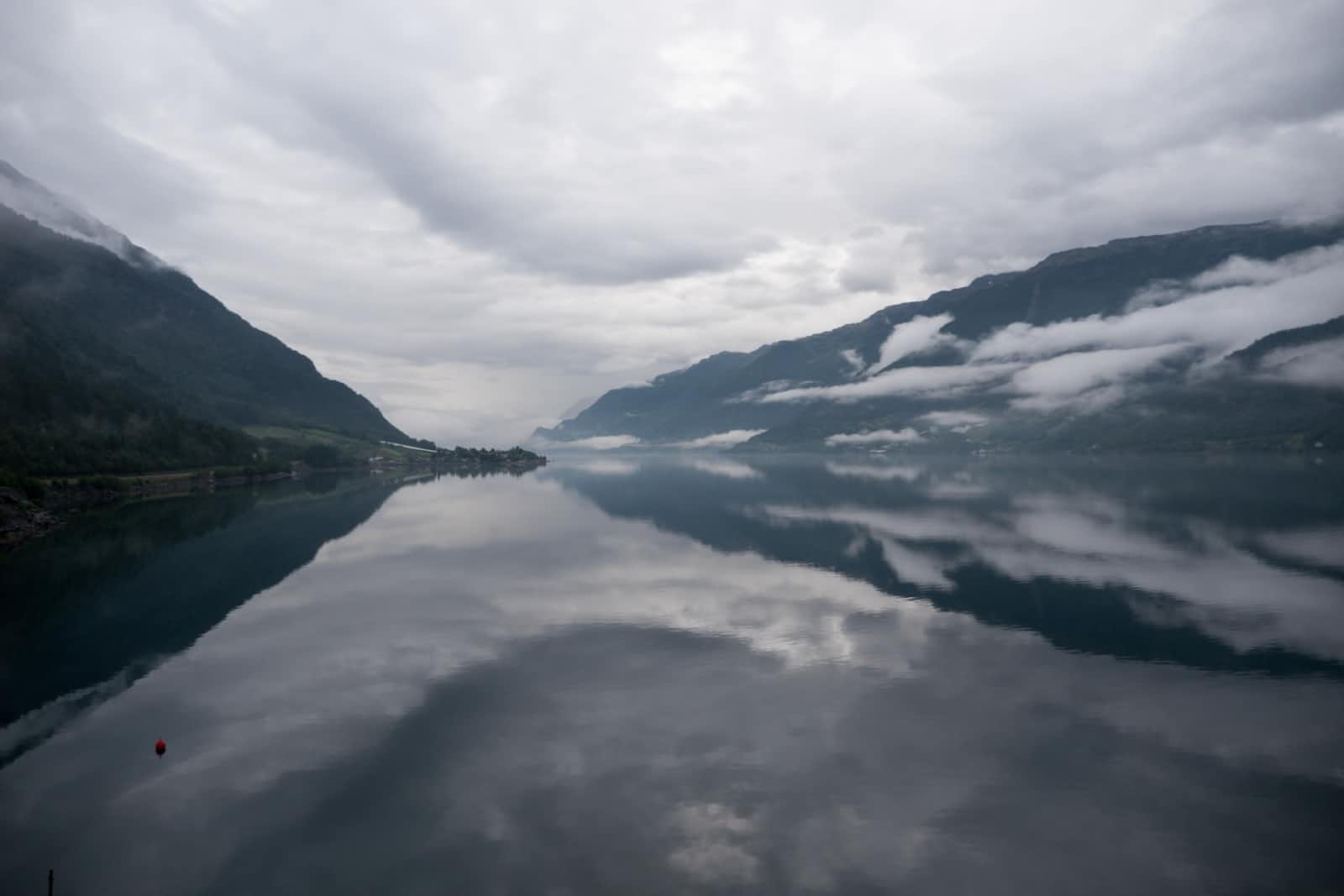
Interfaces should be such that clients should not implement unnecessary functions they do not need
The Interface Segregation Principle (ISP) is one of the SOLID principles of object-oriented design that emphasizes the importance of creating focused and cohesive interfaces for classes. ISP states that interfaces should be designed in such a way that the classes that implement those interfaces do not have many unused functions.
Always remember to do the following for ISP
Design multiple smaller interfaces
One interface should not be handling a lot of responsibilities. Instead, multiple interfaces should be handling different responsibilities
Analogy: There are two different kinds of ATM machines – one for cash withdrawal and another for cash deposit. A single ATM can also offer both functionalities – withdrawal and deposit. However, there is an advantage to having two separate machines. People who want to deposit cash will not have to wait in the queue, where most are waiting for cash withdrawal, and vice versa if it is a combined machine. Therefore, having two separate machines ensures that the traffic is directed to the appropriate machine.
The analogy of separate cash withdrawal and deposit ATMs mirrors the Interface Segregation Principle in software design. Just as distinct ATMs cater to specific needs, segregated interfaces ensure classes implement only necessary functions. This segregation prevents unnecessary coupling, enhancing code clarity. Like separate ATMs, focused interfaces promote efficient utilization and minimize unwanted dependencies.
Example:
Imagine you're designing a notification system for a messaging application. You have various types of notifications: email notifications, SMS notifications, and push notifications. Initially, you might consider creating a single, large notification interface like this:
public interface Notification {
void sendEmail();
void sendSMS();
void sendPushNotification();
}
However, this violates the Interface Segregation Principle because different types of notifications require different functionalities, and not all classes that implement notifications will need to support all three methods. For instance, an SMS notification class wouldn't need to implement the sendEmail()
or sendPushNotification()
methods.
To adhere to the Interface Segregation Principle, you should break down the fat interface into smaller, more focused interfaces, each catering to a specific type of notification:
public interface EmailNotification {
void sendEmail();
}
public interface SMSNotification {
void sendSMS();
}
public interface PushNotification {
void sendPushNotification();
}
Now, the classes that implement these interfaces can selectively implement only the methods that are relevant to their functionality. For example, the SMSService
class would implement the SMSNotification
interface:
public class SMSService implements SMSNotification {
@Override
public void sendSMS() {
// Send SMS notification
}
}
Similarities with Single Responsibility Principle (SRP):
ISP and SRP share a common essence. While ISP revolves around interfaces, SRP delves into the realm of classes.
Conclusion:
Always strive to maintain simple and compact interfaces. If classes implementing these interfaces contain methods with empty definitions, it serves as a signal to consider restructuring the interface into smaller, more focused ones.
Subscribe to my newsletter
Read articles from Chetan Datta directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
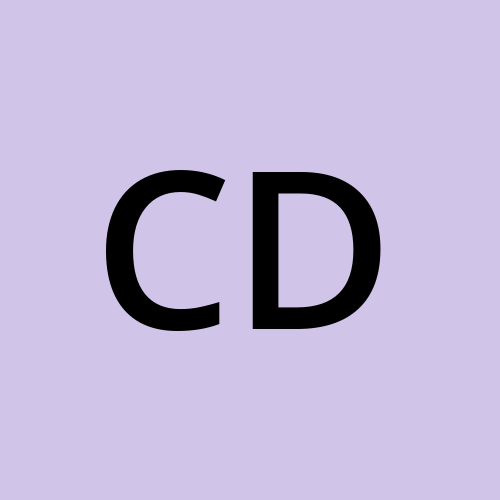
Chetan Datta
Chetan Datta
I'm someone deeply engrossed in the world of software developement, and I find joy in sharing my thoughts and insights on various topics. You can explore my exclusive content here, where I meticulously document all things tech-related that spark my curiosity. Stay connected for my latest discoveries and observations.