Using Lock Among Multiple Applications via Mutex, Redis and Zookeeper
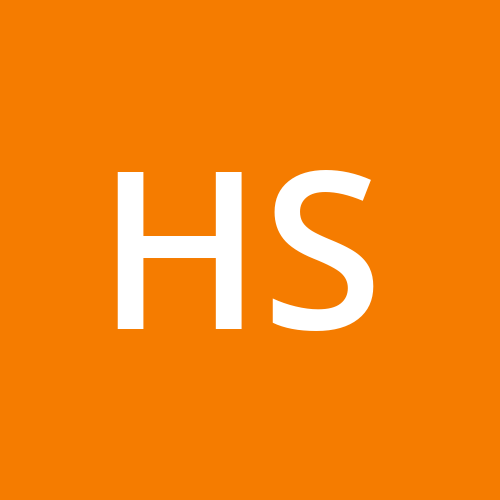
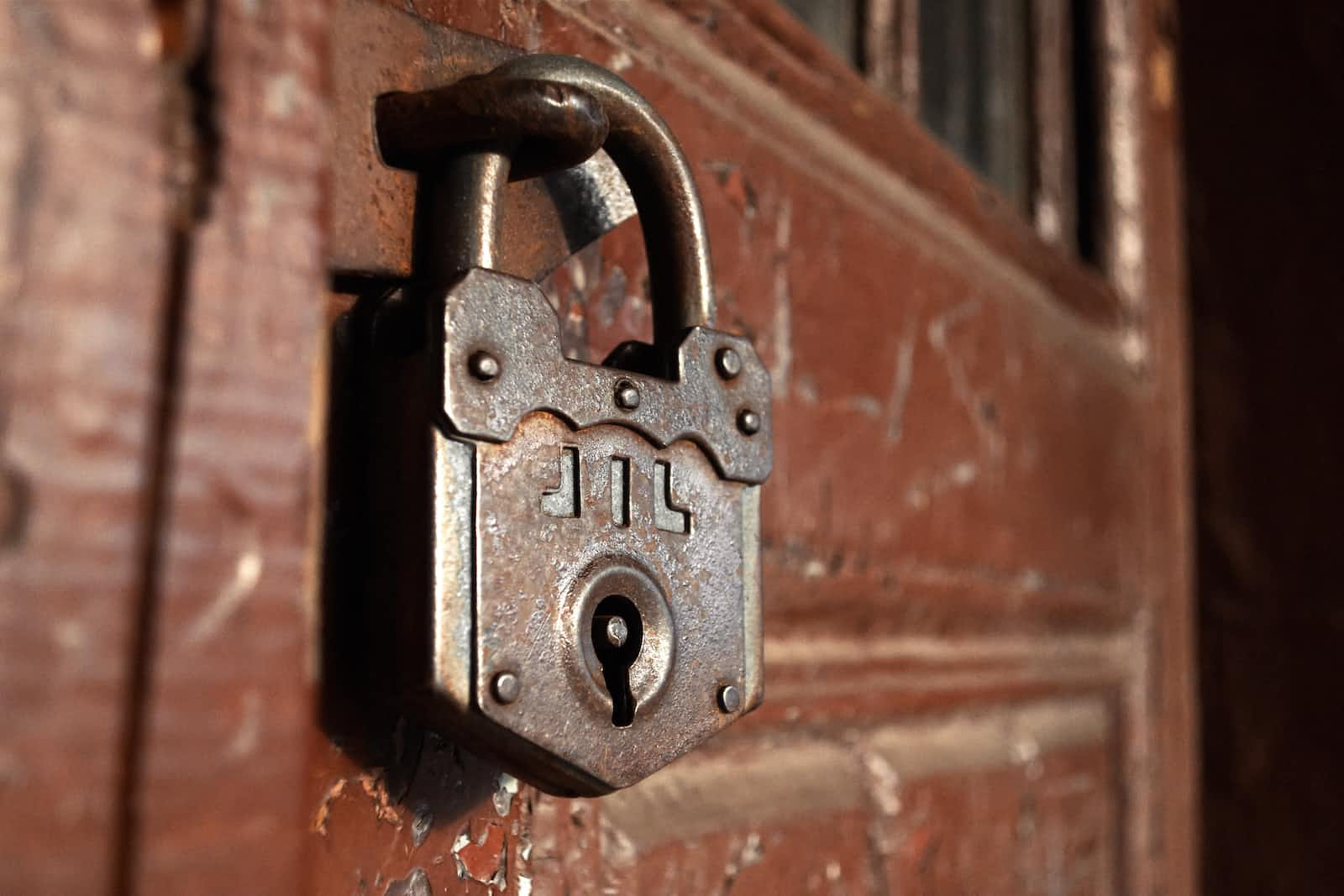
Implementing locking among multiple applications requires inter-process communication and synchronization mechanisms. Below we will see various approaches to do this.
Using Mutex Lock (for single machine)
One common approach is to use named mutexes to coordinate access to shared resources across different applications. However, this approach only works when the apps are deployed on a single machine. Here's an example using C# to demonstrate how you can achieve this:
Suppose you have two separate applications, AppA and AppB, both deployed on the same machine, and you want to implement locking between them.
AppA Code:
using System;
using System.Threading;
class AppA
{
static Mutex mutex = new Mutex(false, "MyMutex");
static void Main(string[] args)
{
Console.WriteLine("AppA started.");
// Attempt to acquire the mutex
bool acquiredMutex = mutex.WaitOne();
if (acquiredMutex)
{
try
{
Console.WriteLine("AppA has acquired the lock.");
// Perform critical section operations
// Simulate some work
Thread.Sleep(5000);
}
finally
{
// Release the mutex when done
mutex.ReleaseMutex();
Console.WriteLine("AppA released the lock.");
}
}
else
{
Console.WriteLine("AppA failed to acquire the lock.");
}
Console.WriteLine("AppA finished.");
}
}
AppB Code:
using System;
using System.Threading;
class AppB
{
static Mutex mutex = new Mutex(false, "MyMutex");
static void Main(string[] args)
{
Console.WriteLine("AppB started.");
// Attempt to acquire the mutex
bool acquiredMutex = mutex.WaitOne();
if (acquiredMutex)
{
try
{
Console.WriteLine("AppB has acquired the lock.");
// Perform critical section operations
// Simulate some work
Thread.Sleep(3000);
}
finally
{
// Release the mutex when done
mutex.ReleaseMutex();
Console.WriteLine("AppB released the lock.");
}
}
else
{
Console.WriteLine("AppB failed to acquire the lock.");
}
Console.WriteLine("AppB finished.");
}
}
In this example, both AppA
and AppB
use a named mutex named "MyMutex" to synchronize access to a shared resource. When AppA
acquires the mutex, it enters the critical section, performs its work, and then releases the mutex. Similarly, when AppB
acquires the mutex, it enters its critical section, performs its work, and then releases the mutex.
The named mutex ensures that only one of the applications can acquire the mutex at a time. If one application holds the mutex, the other application will wait until the mutex is released.
Please note that for locking between different applications, you need to ensure that they have the necessary permissions to create and access named mutexes in the operating system. Also, be careful to avoid deadlocks and ensure that you release the mutex after completing the critical section.
Using distributed lock via Redis
Implementing distributed locking among multiple applications, especially across different systems, often requires the use of external tools or services due to the complexities involved in coordinating processes over a network. One common approach is to use a distributed coordination service like Apache ZooKeeper or Redis, which provides the infrastructure needed for distributed locking. Let's illustrate the concept using an example with Redis and C#.
In this example, we will use the StackExchange.Redis library to interact with Redis for distributed locking.
Note: Before running the example, make sure you have a Redis server up and running.
Install the StackExchange.Redis library using NuGet:
Install-Package StackExchange.Redis
DistributedLockManager.cs:
using System;
using System.Threading.Tasks;
using StackExchange.Redis;
public class DistributedLockManager
{
private readonly ConnectionMultiplexer _connection;
private const string LockKey = "MyDistributedLock";
public DistributedLockManager(string connectionString)
{
_connection = ConnectionMultiplexer.Connect(connectionString);
}
public async Task<IDisposable> AcquireLockAsync()
{
var database = _connection.GetDatabase();
while (true)
{
if (await database.LockTakeAsync(LockKey, "my-unique-id", TimeSpan.FromSeconds(10)))
{
return new LockReleaser(database);
}
await Task.Delay(50);
}
}
private class LockReleaser : IDisposable
{
private readonly IDatabase _database;
public LockReleaser(IDatabase database)
{
_database = database;
}
public void Dispose()
{
_database.LockRelease(LockKey, "my-unique-id");
}
}
}
AppA Code:
using System;
using System.Threading.Tasks;
class AppA
{
static async Task Main(string[] args)
{
var lockManager = new DistributedLockManager("your-redis-connection-string");
using (var distributedLock = await lockManager.AcquireLockAsync())
{
Console.WriteLine("AppA acquired the distributed lock.");
// Perform critical section operations
// Simulate some work
await Task.Delay(5000);
Console.WriteLine("AppA released the distributed lock.");
}
Console.WriteLine("AppA finished.");
}
}
AppB Code:
using System;
using System.Threading.Tasks;
class AppB
{
static async Task Main(string[] args)
{
var lockManager = new DistributedLockManager("your-redis-connection-string");
using (var distributedLock = await lockManager.AcquireLockAsync())
{
Console.WriteLine("AppB acquired the distributed lock.");
// Perform critical section operations
// Simulate some work
await Task.Delay(3000);
Console.WriteLine("AppB released the distributed lock.");
}
Console.WriteLine("AppB finished.");
}
}
In this example, the DistributedLockManager
class uses StackExchange.Redis to implement distributed locking using Redis as the backend. The class provides a method AcquireLockAsync()
that attempts to acquire the distributed lock and returns a LockReleaser
instance when the lock is acquired. The lock is released automatically when the LockReleaser
is disposed.
Both AppA
and AppB
use the DistributedLockManager
to acquire the distributed lock. When one of the applications successfully acquires the lock, the other application waits until the lock is released.
Remember to replace "your-redis-connection-string"
with the actual connection string to your Redis server.
This example demonstrates the concept of distributed locking using Redis. Similar concepts can be applied using other distributed coordination tools like Apache ZooKeeper, Consul, or cloud-based services depending on your requirements.
Using distributed lock via Apache Zookeeper
Apache ZooKeeper is a popular distributed coordination service that can be used for implementing distributed locking. It provides a hierarchical file system-like structure called ZNodes, which can be used to create locks. Here's an example of implementing distributed locking using ZooKeeper and C#.
Note: Before running the example, make sure you have a ZooKeeper server up and running.
Install the ZooKeeperNetEx library using NuGet:
Install-Package ZooKeeperNetEx
DistributedLockManager.cs:
using System;
using System.Threading.Tasks;
using org.apache.zookeeper;
public class DistributedLockManager : IDisposable
{
private readonly ZooKeeper _zooKeeper;
private readonly string _lockPath;
private string _lockNodePath;
private bool _isAcquired = false;
public DistributedLockManager(string connectionString, string lockPath)
{
_zooKeeper = new ZooKeeper(connectionString, TimeSpan.FromSeconds(10), null);
_lockPath = lockPath;
}
public async Task AcquireLockAsync()
{
_lockNodePath = await _zooKeeper.createAsync(_lockPath + "/lock-", new byte[0], ZooDefs.Ids.OPEN_ACL_UNSAFE, CreateMode.EPHEMERAL_SEQUENTIAL);
var children = await _zooKeeper.getChildrenAsync(_lockPath, false);
foreach (var child in children)
{
if (_lockNodePath.EndsWith(child))
{
_isAcquired = true;
break;
}
var lockAcquired = new TaskCompletionSource<bool>();
_zooKeeper.exists(_lockPath + "/" + child, new LockWatcher(_zooKeeper, lockAcquired));
await lockAcquired.Task;
}
}
public void Dispose()
{
if (_isAcquired)
{
_zooKeeper.deleteAsync(_lockNodePath, -1);
}
_zooKeeper.closeAsync();
}
private class LockWatcher : Watcher
{
private readonly ZooKeeper _zooKeeper;
private readonly TaskCompletionSource<bool> _lockAcquired;
public LockWatcher(ZooKeeper zooKeeper, TaskCompletionSource<bool> lockAcquired)
{
_zooKeeper = zooKeeper;
_lockAcquired = lockAcquired;
}
public override async Task process(WatchedEvent @event)
{
if (@event.get_Type() == Event.EventType.NodeDeleted)
{
var children = await _zooKeeper.getChildrenAsync(@event.getPath(), false);
foreach (var child in children)
{
var lockAcquired = new TaskCompletionSource<bool>();
_zooKeeper.exists(@event.getPath() + "/" + child, new LockWatcher(_zooKeeper, lockAcquired));
await lockAcquired.Task;
}
_lockAcquired.TrySetResult(true);
}
}
}
}
AppA Code:
using System;
class AppA
{
static void Main(string[] args)
{
using (var lockManager = new DistributedLockManager("your-zookeeper-connection-string", "/mylocks"))
{
Console.WriteLine("AppA trying to acquire the distributed lock...");
lockManager.AcquireLockAsync().Wait();
Console.WriteLine("AppA acquired the distributed lock.");
// Perform critical section operations
// Simulate some work
System.Threading.Thread.Sleep(5000);
Console.WriteLine("AppA released the distributed lock.");
}
Console.WriteLine("AppA finished.");
}
}
AppB Code:
using System;
class AppB
{
static void Main(string[] args)
{
using (var lockManager = new DistributedLockManager("your-zookeeper-connection-string", "/mylocks"))
{
Console.WriteLine("AppB trying to acquire the distributed lock...");
lockManager.AcquireLockAsync().Wait();
Console.WriteLine("AppB acquired the distributed lock.");
// Perform critical section operations
// Simulate some work
System.Threading.Thread.Sleep(3000);
Console.WriteLine("AppB released the distributed lock.");
}
Console.WriteLine("AppB finished.");
}
}
In this example, the DistributedLockManager
class uses the ZooKeeperNetEx library to implement distributed locking using ZooKeeper. The class creates sequential ephemeral nodes under a given lock path, and the client that creates the lowest numbered node is granted the lock. Other clients watch the previous node in sequence and wait for its deletion before attempting to acquire the lock.
Both AppA
and AppB
use the DistributedLockManager
to acquire the distributed lock. When one of the applications successfully acquires the lock, the other application waits until the lock is released.
Replace "your-zookeeper-connection-string"
with the actual ZooKeeper server connection string.
Please keep in mind that distributed locking can introduce complexities, and a thorough understanding of ZooKeeper and distributed systems is recommended before implementing such solutions in production environments.
Implementing your own Distributed Lock from scratch
Implementing your own distributed locking mechanism from scratch is a complex task that requires a deep understanding of distributed systems, network programming, and synchronization concepts. It involves dealing with potential issues such as network failures, node crashes, and race conditions, which can be challenging to handle correctly.
However, I can provide you with a high-level approach for implementing a basic distributed locking mechanism using sockets in C#. Please note that this example is simplified for demonstration purposes and lacks many features needed for a production-ready distributed locking system.
Here's a basic outline of how you could approach this:
Lock Server:
Create a lock server application that listens for incoming connections from clients.
Maintain a list of locks and their current status (locked/unlocked).
When a client requests to acquire a lock, check if the lock is available. If available, mark it as locked and notify the client.
When a client releases a lock, mark it as unlocked and notify any waiting clients.
Lock Client:
Create a lock client application that connects to the lock server.
To acquire a lock, send a request to the server. If the lock is available, the server responds with a success message; otherwise, the client waits until the lock becomes available.
To release a lock, send a release request to the server.
Here's a simplified example of how the lock server and client could be implemented using C# and sockets:
Lock Server:
using System;
using System.Collections.Generic;
using System.Net;
using System.Net.Sockets;
using System.Text;
using System.Threading;
class LockServer
{
private static Dictionary<string, bool> locks = new Dictionary<string, bool>();
private static object locksLock = new object();
static void Main(string[] args)
{
int port = 12345;
TcpListener listener = new TcpListener(IPAddress.Any, port);
listener.Start();
Console.WriteLine("Lock server started on port " + port);
while (true)
{
using (TcpClient client = listener.AcceptTcpClient())
using (NetworkStream stream = client.GetStream())
{
byte[] data = new byte[1024];
int bytesRead = stream.Read(data, 0, data.Length);
string request = Encoding.ASCII.GetString(data, 0, bytesRead).Trim();
string response = ProcessRequest(request);
byte[] responseBytes = Encoding.ASCII.GetBytes(response);
stream.Write(responseBytes, 0, responseBytes.Length);
}
}
}
private static string ProcessRequest(string request)
{
string[] parts = request.Split(' ');
if (parts.Length != 2)
return "Invalid request";
string operation = parts[0];
string lockName = parts[1];
lock (locksLock)
{
if (operation == "AcquireLock")
{
if (!locks.ContainsKey(lockName))
{
locks.Add(lockName, true);
return "Lock acquired";
}
else
{
return "Lock already acquired";
}
}
else if (operation == "ReleaseLock")
{
if (locks.ContainsKey(lockName))
{
locks.Remove(lockName);
return "Lock released";
}
else
{
return "Lock not found";
}
}
else
{
return "Invalid operation";
}
}
}
}
In this example, the locks
dictionary is used to keep track of locks and their statuses. The locksLock
object is used to synchronize access to the locks
dictionary to ensure thread safety. The ProcessRequest
method processes the client requests for acquiring and releasing locks.
Lock Client:
using System;
using System.Net.Sockets;
using System.Text;
class LockClient
{
static void Main(string[] args)
{
string serverIp = "127.0.0.1";
int serverPort = 12345;
using (TcpClient client = new TcpClient(serverIp, serverPort))
using (NetworkStream stream = client.GetStream())
{
// Send request to acquire lock
byte[] data = Encoding.ASCII.GetBytes("AcquireLock");
stream.Write(data, 0, data.Length);
// Receive response from the server
data = new byte[1024];
int bytesRead = stream.Read(data, 0, data.Length);
string response = Encoding.ASCII.GetString(data, 0, bytesRead);
Console.WriteLine("Server response: " + response);
}
}
}
Remember that this example is highly simplified and lacks error handling, proper synchronization, and other crucial features needed for a robust distributed locking system. In a real-world scenario, you would need to handle edge cases, implement timeouts, deal with network failures, ensure proper synchronization, and handle scalability concerns.
Creating a production-quality distributed locking mechanism involves deep architectural considerations and thorough testing to ensure its correctness, reliability, and performance. In practice, it's often better to use established distributed coordination tools like ZooKeeper, Redis, or specialized libraries designed for this purpose.
Subscribe to my newsletter
Read articles from Hemant Singh directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
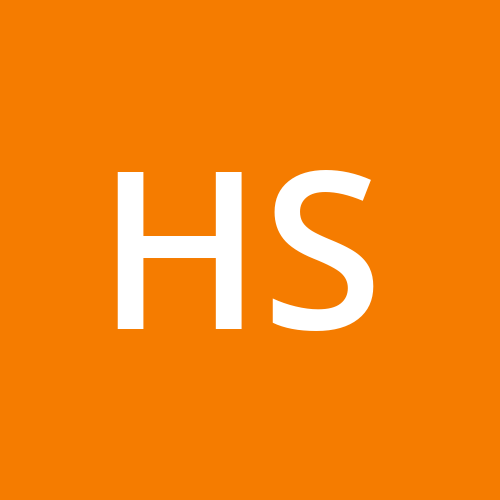