Interfaces in Go
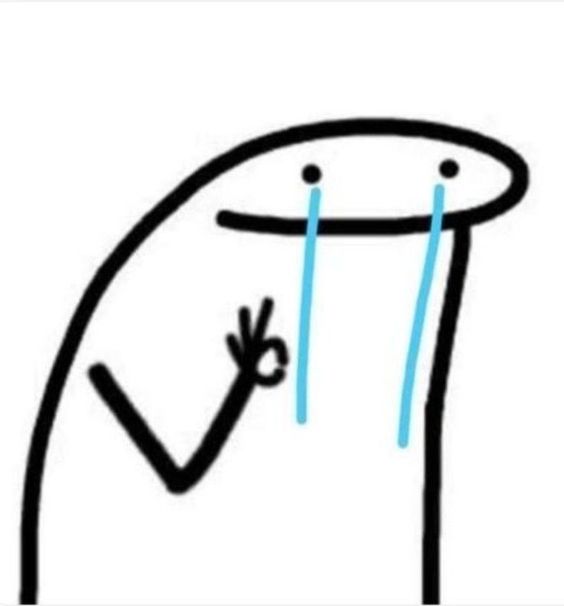
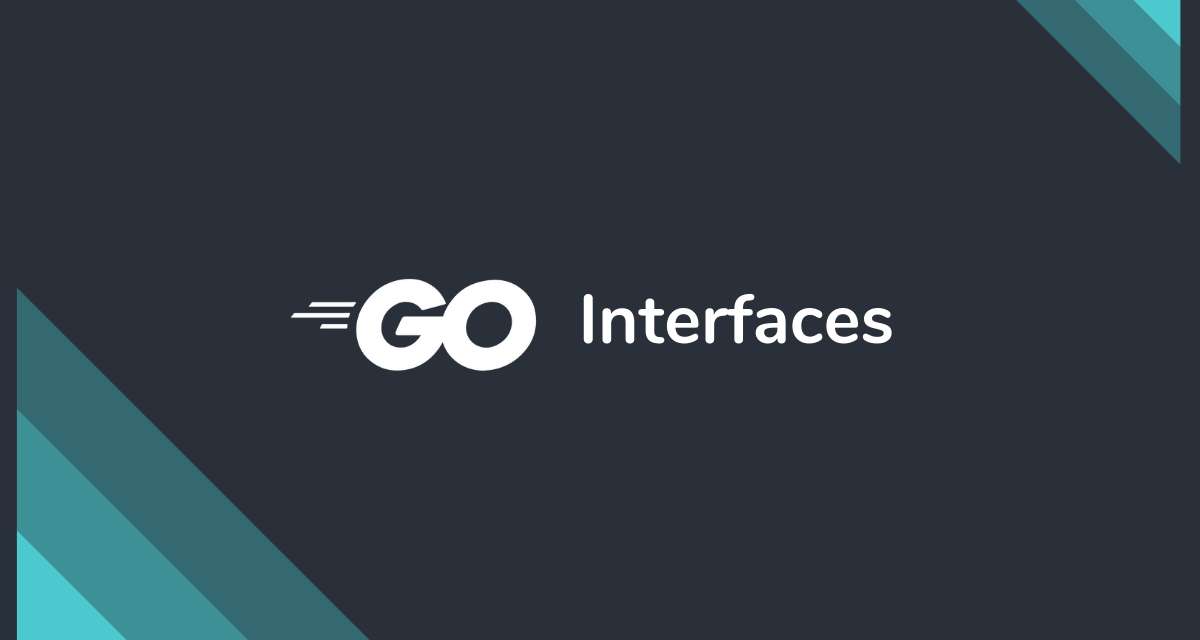
According to https://go.dev/tour/methods/9, an interface is a collection of method signatures.
type Shape interface{
area() float64
}
As you can see in the above code snippet, I define Shape which is an interface and simply put a method(you can add multiple methods) in it. Now according to the definition, it is correct i.e. a collection of method signatures.
Now, any type that implements the area method and matches the signature of the method will implement the Shape interface. Let's understand with an example below
type Circle struct{
Radius float64
} //defining a struct Circle
func (c Circle) area() float64{
return c.Radius*c.Radius
} // area() method defined on Circle struct
In the above code, I define a struct and its method. As you can see the area method of Circle has the same signature as of the method in the interface. Interfaces are implemented implicitly means when we have a type eg. in the above code snippet the circle same method area() that is defined in the interface then it automatically implemented the shape interface, we don't define anywhere that it implements the Shape.
type Shape interface{
area() float64
power(x int) float64() //takes in parameter of type int
}
Now, in this case, our Circle struct doesn't implement the Shape interface because it doesn't satisfy the conditions i.e. the Circle doesn't have a method called power.
Here is the complete code where I use an interface.
package main
import "log"
type Shape interface{
area() float64
}
type Circle struct{
Radius float64
}
func(c Circle) area() float64{
return c.Radius*c.Radius
}
func main() {
c := Circle{3.2}
var s Shape = c
log.Println(s.area())
}
Upto main function everything we have done before, now in main what I am doing is first I declared a 'c' variable of type Circle with a value 3.2 which is the radius. After that, we are initializing an interface of type Shape and assign the value of 'c'(instance of the circle) to 's'. This is possible because 'Circle' implements the 'area()' method required by the 'Shape' interface. After this, we call the 'area()' method which will be executed on the underlying 'Circle' instance due to the polymorphism provided by interfaces. This allows you to work with different types that implement the same interface uniformly, even if they have different implementations for the methods.
Happy Coding
Subscribe to my newsletter
Read articles from averageDev directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
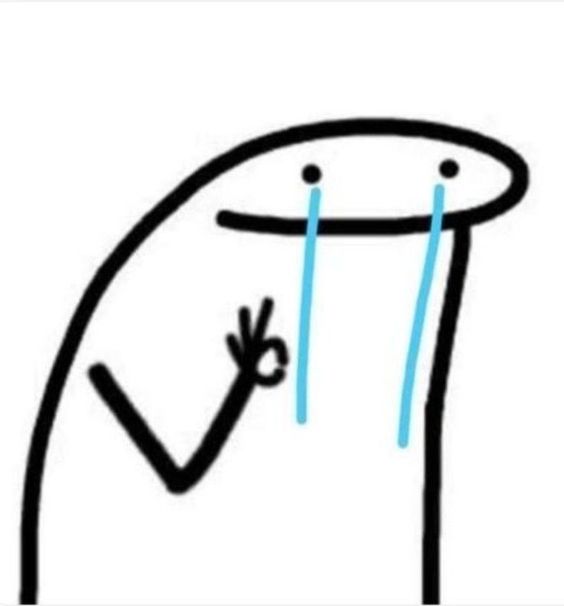