useState Hook in React : All you need to know
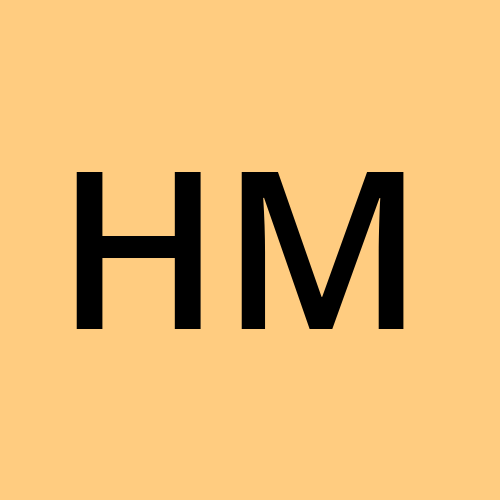
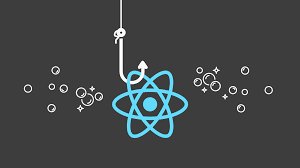
Introduction:-
One of the most frequently used hooks in React is the useState hook. useState is used to track the current value/state of a variable that is used inside your react component. For example if there is a component which increments or decrements the value of a variable on click of a button then to change the variables state on click event we would need the useState hook. We change the value of the variable using a method that is provided by useState hook.
Explanation using example described in the first point:-
import { useState } from "react";
export default function BasicExample() {
const [count, setCount] = useState(0);
function increment(){
setCount(prevCount => prevCount + 1);
}
function decrement(){
setCount(prevCount => prevCount - 1);
}
return (
<>
<button onClick={decrement}>-</button>
<span>{count}</span>
<button onClick={increment}>+</button>
</>
)
}
Here we use useState hook to set initial value of count variable to 0, and then use setCount method provided by useState hook to change the state of count variable when required i.e on click of the buttons. Now the prevCount variable used in setCount method in the increment and decrement functions gives us the most recent state of count variable to which we can perform our operation else if we directly use count to increment/derement it may result in wrong computation in some cases.
Lesser known facts about useState hook:-
useState is called whenever the component gets rendered. So suppose in case if we set initial value of variable after some long computation which takes much time, the complex computation will be performed every time component renders which is not optimized way. So if want to perform computation only once then we can use a function to set the initial value.
How to compute starting values for variables only once inside useState hook ?
Explanation using exapmle
import { useState } from "react";
export default function BasicExample() {
const [count, setCount] = useState(() => {
//complex computation
//return answer;
});
function increment(){
setCount(prevCount => prevCount + 1);
}
function decrement(){
setCount(prevCount => prevCount - 1);
}
return (
<>
<button onClick={decrement}>-</button>
<span>{count}</span>
<button onClick={increment}>+</button>
</>
)
}
- The above example illustrates how one should use complex logics to set initial values in useState hooks.
Subscribe to my newsletter
Read articles from Husain Modiwala directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
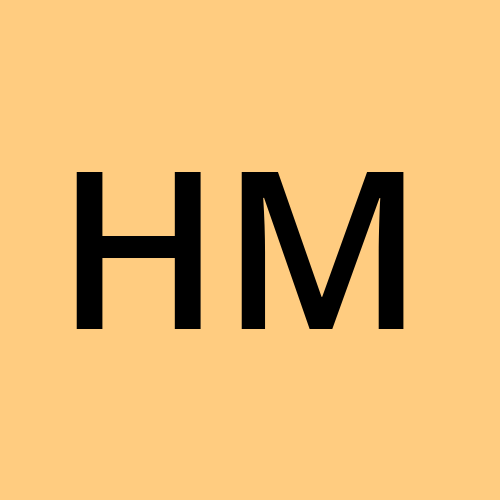