useReducer() Hook
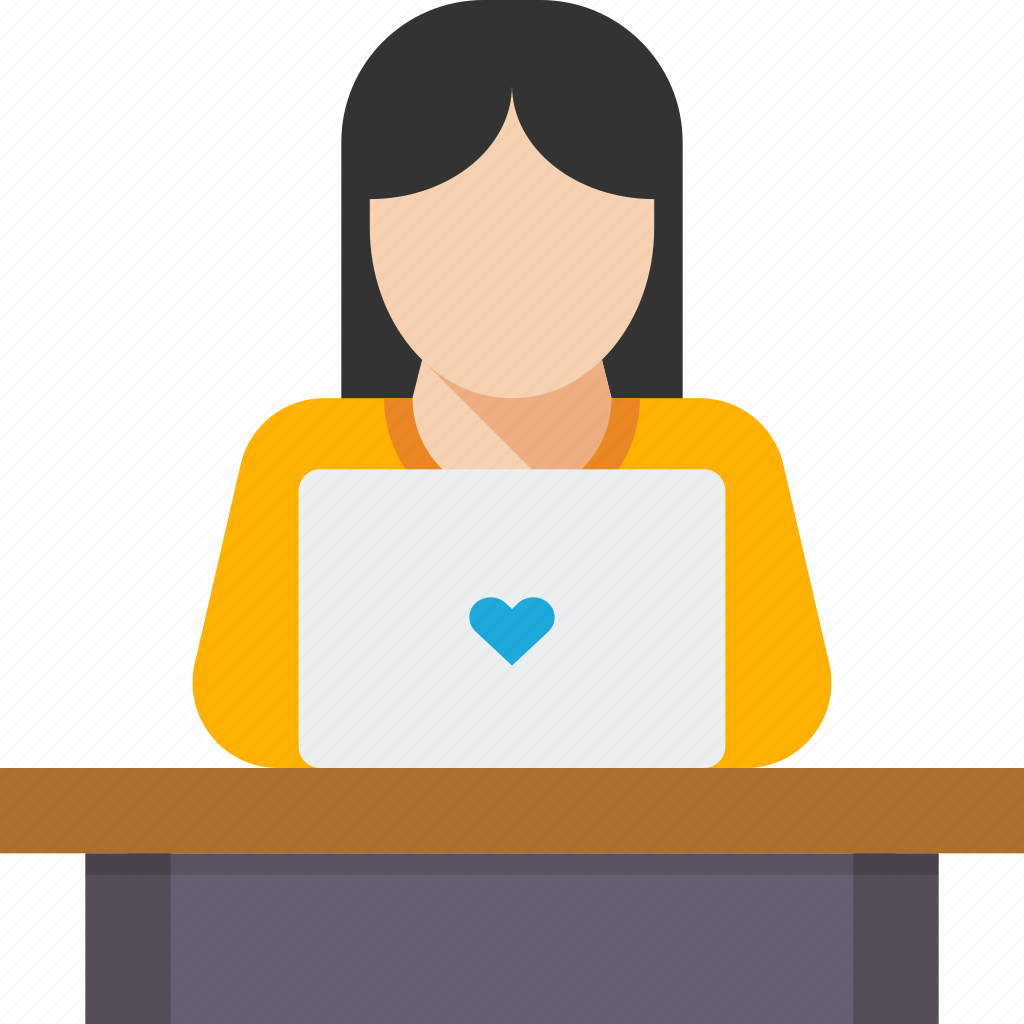
What is a useReducer hook?
It is just like useState hook but with more capabilities and for managing complex states, for managing states that are related instead of writing them separately, write together in one state, which is not possible with useState.
Syntax
const [inputState,dispatch]=useReducer(reducerFn,initialState);
useReducer returns an array with two things we use array destructuring to pull out these values; First one is the current state and the second one is the function which changes the state just like useState but the difference is that useState changes the state directly while useReducer, you need to pass an action to dispatch a function .
reducer function
const reducerFn=(prevState,action)=>{}
reducerFn returns a newly updated state. The reducerFn can be created outside the component.
Example
import { useReducer } from 'react'
import './App.css'
const initialState={count:0}
const reducerFn=(state,action)=>{
if(action.type === 'INCREMENT'){
return {count:state.count+1}
}
if(action.type === 'DECREMENT'){
return {count:state.count-1}
}
return state;
}
function App() {
const [state,dispatch]=useReducer(reducerFn,initialState)
const incrementHandler=()=>{
dispatch({type:'INCREMENT'})
}
const decrementHandler=()=>{
dispatch({type:'DECREMENT'})
}
return(
<>
<h1>{state.count}</h1>
<button onClick={incrementHandler}>Increment</button>
<button onClick={decrementHandler}>Decremnt</button>
</>
)
}
export default App;
In this article, we explored Reactβs useReducer
Hook, reviewing how it works, and when to use it.
I hope you enjoyed this article, and be sure to leave a comment if you have any questions. Happy coding! πππ
Subscribe to my newsletter
Read articles from Rumaisa directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
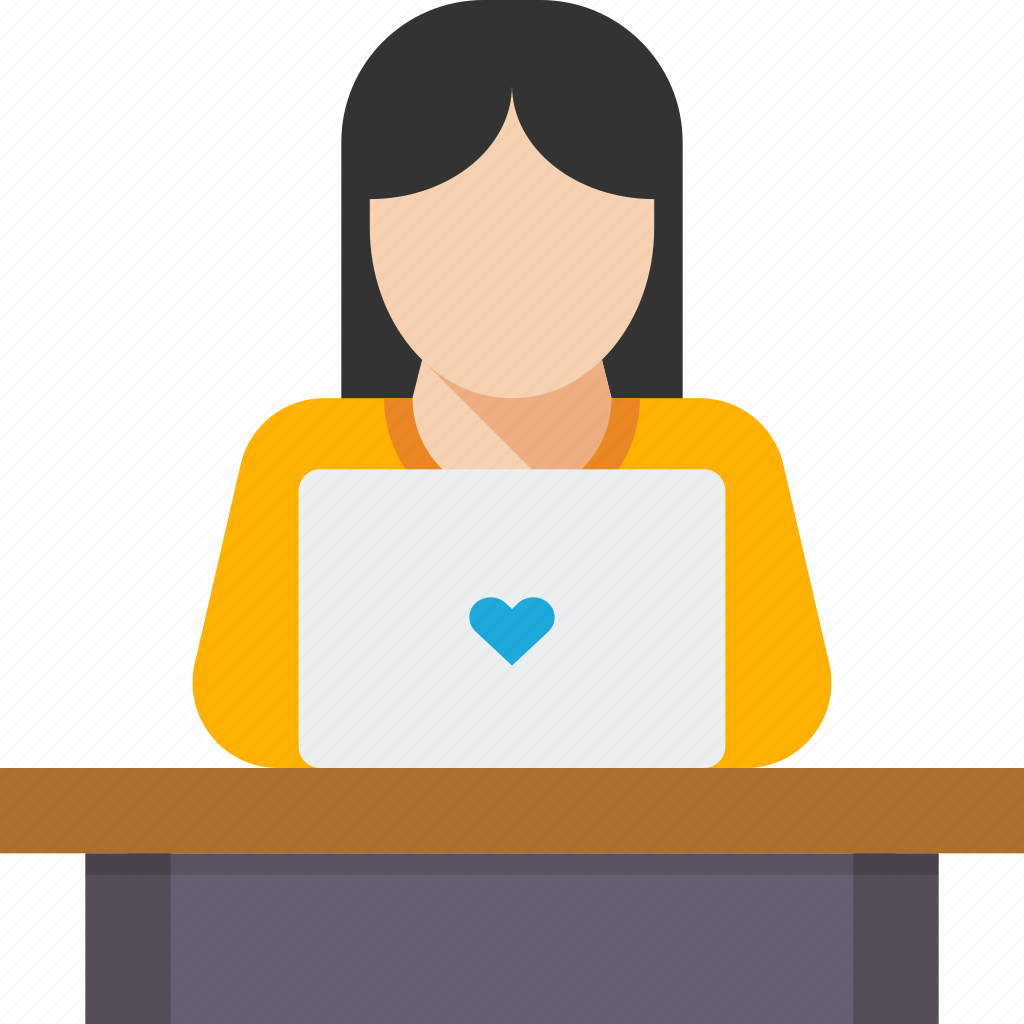