Laravel - Task Scheduling
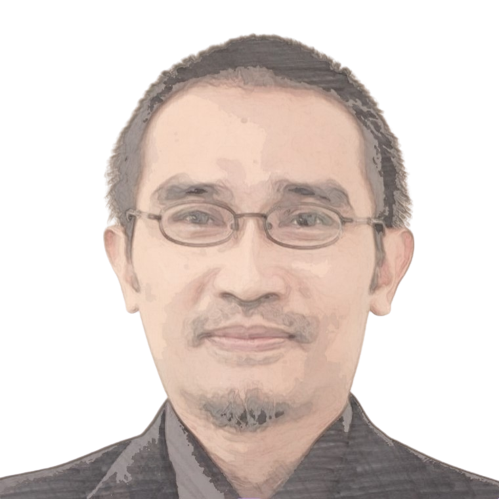
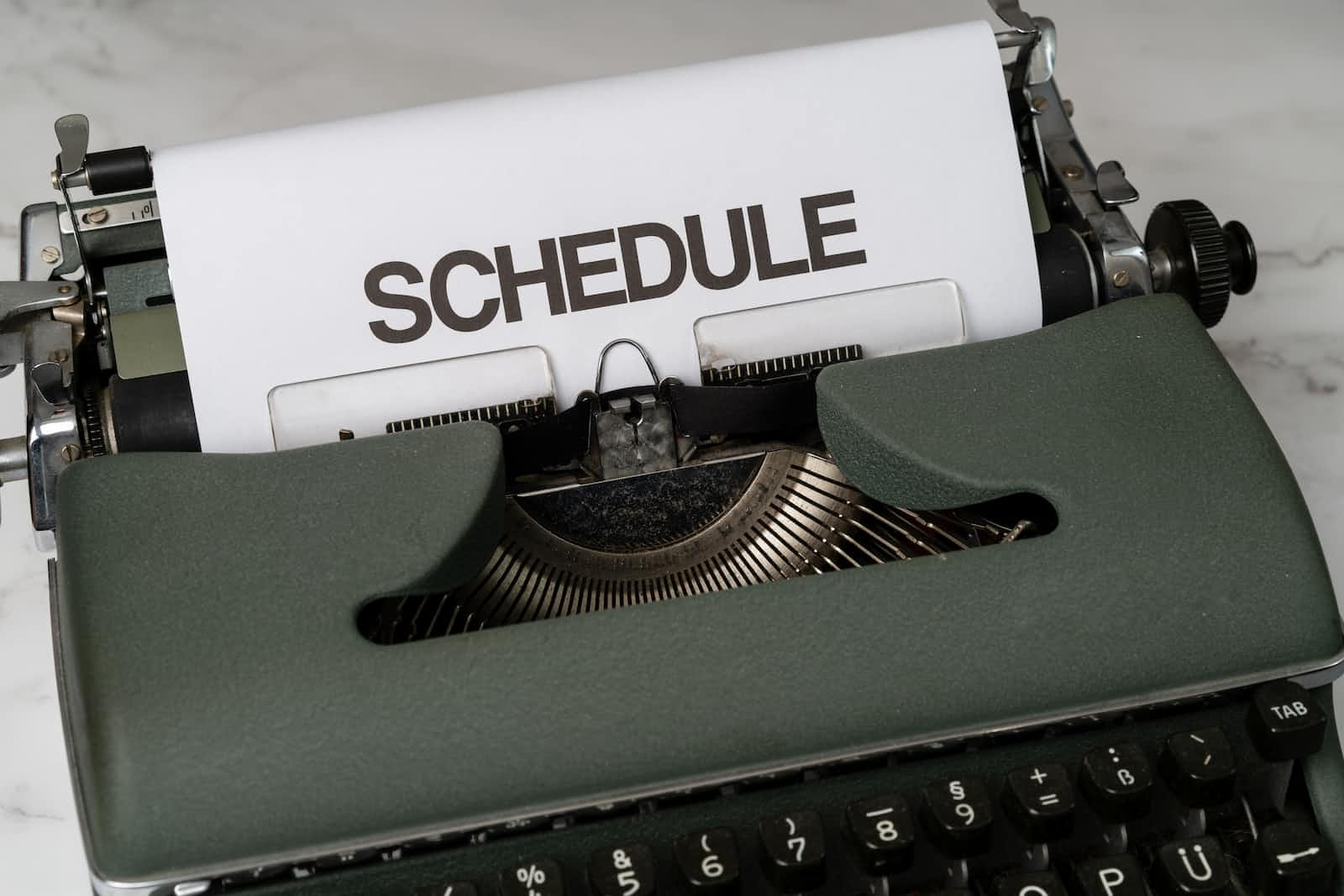
[0] Create demo project
composer create-project --prefer-dist laravel/laravel laraschedule
or using phpsandbox.io:
Template=Laravel 8
Title=laraschedule
[1] Create schedule command example
php artisan make:command MyTask --command=my:task
Outcome:
[2] Add task to do
Edit handle method:
public function handle()
{
$dt = new DateTime();
Log::info("Schedule runs at:".$dt->format('Y-m-d H:i:s'));
return 0;
}
Import class:
use Illuminate\Support\Facades\Log;
use DateTime;
Full codes:
(app\console\commands\MyTask.php)
<?php
namespace App\Console\Commands;
use Illuminate\Console\Command;
use Illuminate\Support\Facades\Log;
use DateTime;
class MyTask extends Command
{
/**
* The name and signature of the console command.
*
* @var string
*/
protected $signature = 'my:task';
/**
* The console command description.
*
* @var string
*/
protected $description = 'Command description';
/**
* Create a new command instance.
*
* @return void
*/
public function __construct()
{
parent::__construct();
}
/**
* Execute the console command.
*
* @return int
*/
public function handle()
{
$dt = new DateTime();
Log::info("Schedule runs at:".$dt->format('Y-m-d H:i:s'));
return 0;
}
}
[3] Register Task Scheduler
Register command path:
protected $commands = [
Commands\MyTask::class,
];
Register command call:
protected function schedule(Schedule $schedule)
{
$schedule->command('My:Task')->everyMinute();
}
Full codes:
(app\console\kernel.php)
<?php
namespace App\Console;
use Illuminate\Console\Scheduling\Schedule;
use Illuminate\Foundation\Console\Kernel as ConsoleKernel;
class Kernel extends ConsoleKernel
{
protected $commands = [
Commands\MyTask::class,
];
/**
* Define the application's command schedule.
*
* @param \Illuminate\Console\Scheduling\Schedule $schedule
* @return void
*/
protected function schedule(Schedule $schedule)
{
// $schedule->command('inspire')->hourly();
$schedule->command('My:Task')->everyMinute();
}
/**
* Register the commands for the application.
*
* @return void
*/
protected function commands()
{
$this->load(__DIR__.'/Commands');
require base_path('routes/console.php');
}
}
[4] Test run schedule
php artisan schedule:run
Console Outcome:
Log outcome:
(storage/logs/laravel.log)
Reference:
https://laravel.com/docs/10.x/scheduling
Example codes:
https://phpsandbox.io/n/laraschedule-nsix3#app/Console/Commands/MyTask.php
https://phpsandbox.io/n/laraschedule-nsix3#app/Console/Kernel.php
https://phpsandbox.io/n/laraschedule-nsix3#storage/logs/laravel.log
Subscribe to my newsletter
Read articles from Mohamad Mahmood directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
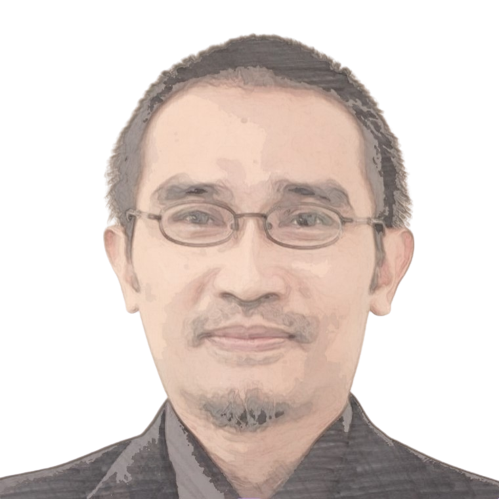
Mohamad Mahmood
Mohamad Mahmood
Mohamad's interest is in Programming (Mobile, Web, Database and Machine Learning). He studies at the Center For Artificial Intelligence Technology (CAIT), Universiti Kebangsaan Malaysia (UKM).