Exploring Delegates in C#
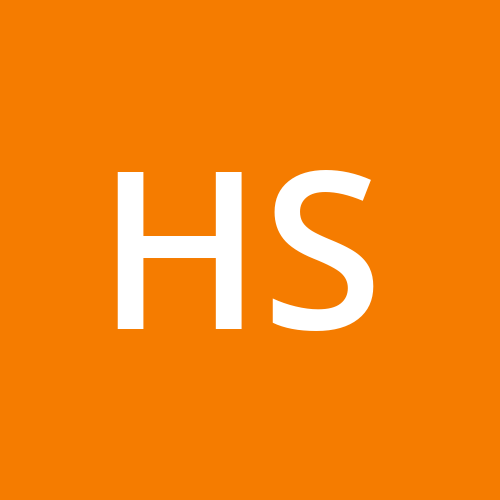
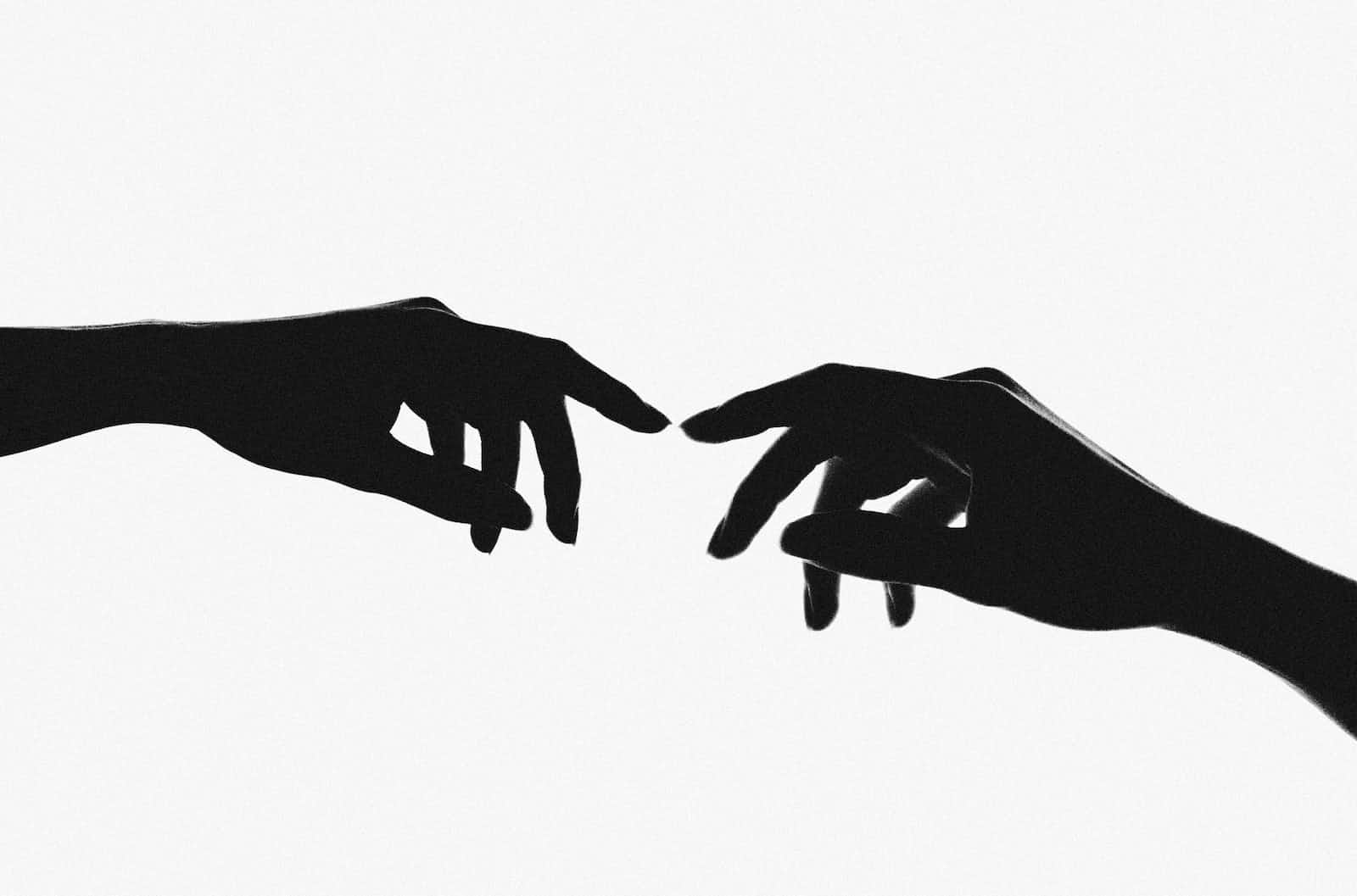
Delegates are a powerful and fundamental concept in the C# programming language, enabling developers to achieve greater flexibility and code reusability by representing method references. They serve as a mechanism for defining, passing, and invoking methods at runtime, making them a crucial tool for implementing callback mechanisms, event handling, and more. This article dives into the world of delegates, providing a comprehensive understanding of their usage, implementation, and showcasing numerous examples to solidify the concepts.
Understanding Delegates
At its core, a delegate is a type that represents references to methods with a specific signature. It essentially acts as a pointer to a function. This allows developers to treat methods as first-class entities, passing them as parameters to other methods or storing them in variables.
In C#, delegates are declared using the delegate
keyword followed by the method signature the delegate will reference. Delegates can point to both static and instance methods. The basic syntax for declaring a delegate looks like this:
delegate returnType DelegateName(parameterList);
Here, returnType
is the return type of the method the delegate will point to, DelegateName
is the name of the delegate type, and parameterList
is the list of parameters that the method accepts.
Delegate Declaration and Usage
Let's start by considering a simple example. Suppose we have two methods that perform addition and subtraction:
class MathOperations
{
public int Add(int a, int b) => a + b;
public int Subtract(int a, int b) => a - b;
}
Now, we can declare a delegate type that matches the signature of these methods:
delegate int MathDelegate(int a, int b);
With the delegate type defined, we can create delegate instances that point to the Add
and Subtract
methods:
MathOperations math = new MathOperations();
MathDelegate addDelegate = math.Add;
MathDelegate subtractDelegate = math.Subtract;
We can then invoke these delegate instances just like regular methods:
int result1 = addDelegate(5, 3); // Result: 8
int result2 = subtractDelegate(10, 4); // Result: 6
Delegates for Callbacks
We can pass a function itself as an input parameter to another function, with the help of a delegate.
using System;
// Declare a delegate named CalculationComplete
delegate void CalculationComplete(int result);
class Calculator
{
// Define a method that performs addition and invokes the callback
public void Add(int a, int b, CalculationComplete callback)
{
int result = a + b;
callback(result); // Invoke the callback method with the result
}
}
class Program
{
static void Main()
{
// Create an instance of the Calculator class
Calculator calculator = new Calculator();
// Declare a callback method (an instance of CalculationComplete delegate)
CalculationComplete callback = DisplayResult;
// Call the Add method of the calculator and pass the callback
calculator.Add(5, 3, callback);
}
// Callback method to display the calculation result
static void DisplayResult(int result)
{
Console.WriteLine($"Calculation result: {result}");
}
}
In the above example, the function DisplayResult()
is assigned to the delegate CalculationComplete
, and then passed to Add()
function as an input parameter (callback). The Add()
function then invokes the delegate by calling callback(result)
, indirectly invoking DisplayResult()
.
Delegates and Anonymous Methods
C# also supports anonymous methods, which are methods without a defined name. These are particularly useful when working with delegates. Continuing with the button click example, let's see how anonymous methods can be used:
using System;
// Declare a delegate named PrintDelegate
delegate void PrintDelegate(string message);
class Program
{
static void Main()
{
// Declare a delegate variable named print and assign an anonymous method to it
PrintDelegate print = delegate (string msg)
{
Console.WriteLine($"Anonymous: {msg}");
};
// Call the delegate, which invokes the anonymous method
print("Hello from anonymous method!");
}
}
In this example, a delegate variable named print
is declared with the type PrintDelegate
. It is assigned an anonymous method using the delegate
keyword followed by its parameter list and body. In this case, the anonymous method takes a string
parameter named msg
and writes a formatted message to the console.
Delegates and Lambda Expressions
Lambda expressions provide a concise way to create delegate instances. The previous example can be rewritten using lambda expressions:
using System;
// Declare a delegate named MathOperation
delegate int MathOperation(int a, int b);
class Program
{
static void Main()
{
// Define delegate variables using lambda expressions
MathOperation add = (a, b) => a + b;
MathOperation subtract = (a, b) => a - b;
// Call the delegates with lambda expressions
Console.WriteLine(add(5, 3)); // Output: 8
Console.WriteLine(subtract(5, 3)); // Output: 2
}
}
Lambda expressions make the code more readable and reduce the verbosity of delegate instantiation. In the above example, two delegate variables are declared: add
and subtract
, both with the type MathOperation
. These variables are initialized using lambda expressions. Lambda expressions are enclosed in parentheses and use the =>
operator to separate the input parameters from the expression body.
Multicast Delegates
Delegates can also be combined to form multicast delegates, allowing multiple methods to be invoked in a sequence. This is particularly useful for scenarios like event handling:
using System;
// Declare a delegate named ActionDelegate
delegate void ActionDelegate();
class Program
{
static void Main()
{
// Declare delegate variables and attach methods using the += operator
ActionDelegate actions = Method1;
actions += Method2;
actions += Method3;
// Call the multicast delegate, invoking all attached methods
actions();
}
static void Method1() { Console.WriteLine("Method 1"); }
static void Method2() { Console.WriteLine("Method 2"); }
static void Method3() { Console.WriteLine("Method 3"); }
}
In the above example, the actions
delegate is assigned the Method1
, Method2
, and Method3
methods using the +=
operator. This establishes a multicast delegate, where actions
holds references to multiple methods. Later, the actions
delegate is called using the ()
operator. Since it is a multicast delegate, calling it invokes all the attached methods (Method1
, Method2
, and Method3
) in the order they were attached.
Utilizing Delegates for Event Handling
Delegates truly shine when used in scenarios where dynamic invocation is required. A classic use case is event handling. Consider a scenario where a door opened event needs to be handled. Delegates provide a clean way to achieve this. You can read more on event handling here - Mastering Events in C#
using System;
class Door
{
// Declare the delegate for the event
public delegate void DoorHandler();
// Declare the event using the delegate
public event DoorHandler Opened;
public void Open()
{
Console.WriteLine("Door is opened.");
// Raise the event when the door is opened
Opened?.Invoke();
}
}
class Program
{
static void Main()
{
Door door = new Door();
// Subscribe to the event using a method
door.Opened += HandleDoorOpened;
door.Open();
}
static void HandleDoorOpened()
{
Console.WriteLine("Event handler: Door is now open.");
}
}
In this example:
We have a
Door
class that defines an event namedOpened
.The
Opened
event is declared using theDoorHandler
delegate type.The
Open
method of theDoor
class simulates opening a door and raises theOpened
event.In the
Main
method, we create an instance of theDoor
class and subscribe to theOpened
event using theHandleDoorOpened
method.When the
Open
method of theDoor
instance is called, the event handler is invoked, and a message is displayed.
Built-in Delegate Types
C# provides several built-in delegate types in the System
namespace for common scenarios. Some of these include:
Action
: Represents a delegate that doesn't return a value.Func
: Represents a delegate that returns a value.Predicate
: Represents a delegate that performs a test and returns a boolean value.
Action Delegate
The Action
delegate is used when you want to define a method that takes one or more parameters but doesn't return a value (void). It's commonly used for scenarios where you need to perform an action or operation without expecting a result.
The Action
delegate comes in various forms based on the number of input parameters it takes. The following are a few examples:
Action
: Represents a method that takes no parameters and doesn't return a value.Action<T>
: Represents a method that takes one parameter of typeT
and doesn't return a value.Action<T1, T2>
: Represents a method that takes two parameters of typesT1
andT2
and doesn't return a value.And so on...
Example using Action
:
Action<string> printMessage = message => Console.WriteLine(message);
printMessage("Hello, Action delegate!"); // Output: Hello, Action delegate!
Func Delegate
The Func
delegate is used to define a method that takes one or more parameters and returns a value. It's often used for scenarios where you need to perform a computation and return a result.
Like the Action
delegate, the Func
delegate comes in various forms based on the number of input parameters and the return type. The last type parameter always represents the return type.
Example using Func
:
//Example 1: Using func with 2 int params and return param is also int (3rd)
Func<int, int, int> add = (a, b) => a + b;
int result = add(5, 3); // Result: 8
// Example 2: Using Func with no parameters and an int return type
Func<int> getNumber = () => 42;
int result = getNumber();
Console.WriteLine($"Result: {result}");
// Example 3: Using Func with two parameters (int, int) and a bool return type
Func<int, int, bool> isGreaterThan = (x, y) => x > y;
bool greater = isGreaterThan(5, 3);
Console.WriteLine($"5 is greater than 3: {greater}");
// Example 4: Using Func with three parameters (string, int, int) and a string return type
Func<string, int, int, string> formatString = (text, num1, num2) => $"{text}: {num1 + num2}";
string formatted = formatString("Sum", 10, 20);
Console.WriteLine(formatted);
Predicate Delegate
The Predicate
delegate is specifically used to define a method that performs a test on an object and returns a boolean value. It's commonly used for filtering and testing conditions.
Example using Predicate
:
Predicate<int> isEven = num => num % 2 == 0;
bool even = isEven(6); // Result: true
bool odd = isEven(7); // Result: false
These built-in delegate types provide a standardized and convenient way to work with method signatures, making it easier to create more modular and flexible code. By using these delegates, you can enhance code readability, improve maintainability, and reduce the need for defining custom delegate types in many scenarios.
Conclusion
Delegates are a powerful feature of C# that allow for dynamic method invocation and enable scenarios such as event handling, callbacks, and more. By understanding how to declare, instantiate, and use delegates, developers can write more flexible and maintainable code. With the ability to create anonymous methods and utilize lambda expressions, delegates provide a modern and elegant approach to handling methods as first-class citizens in the language. By mastering delegates, developers can harness their potential for crafting robust and versatile applications in C#.
In this article, we've covered the fundamentals of delegates, their usage, and provided numerous examples to illustrate their versatility. From simple method references to advanced event handling scenarios, delegates play a pivotal role in enhancing the expressive power of C# programs. As you continue to explore C# and its features, make sure to leverage the potential of delegates to build more modular, efficient, and extensible codebases.
Subscribe to my newsletter
Read articles from Hemant Singh directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
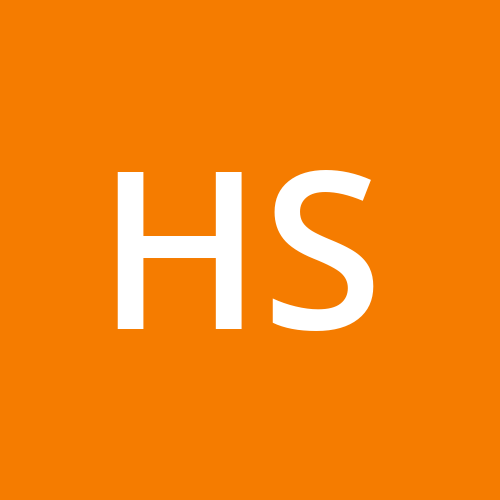