Create a blog website using NextJs and Keystatic - Setup (Part 1)
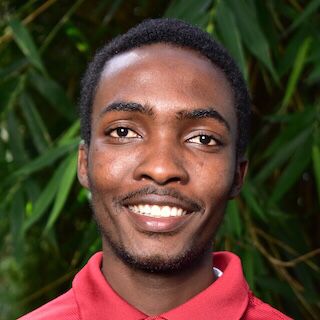
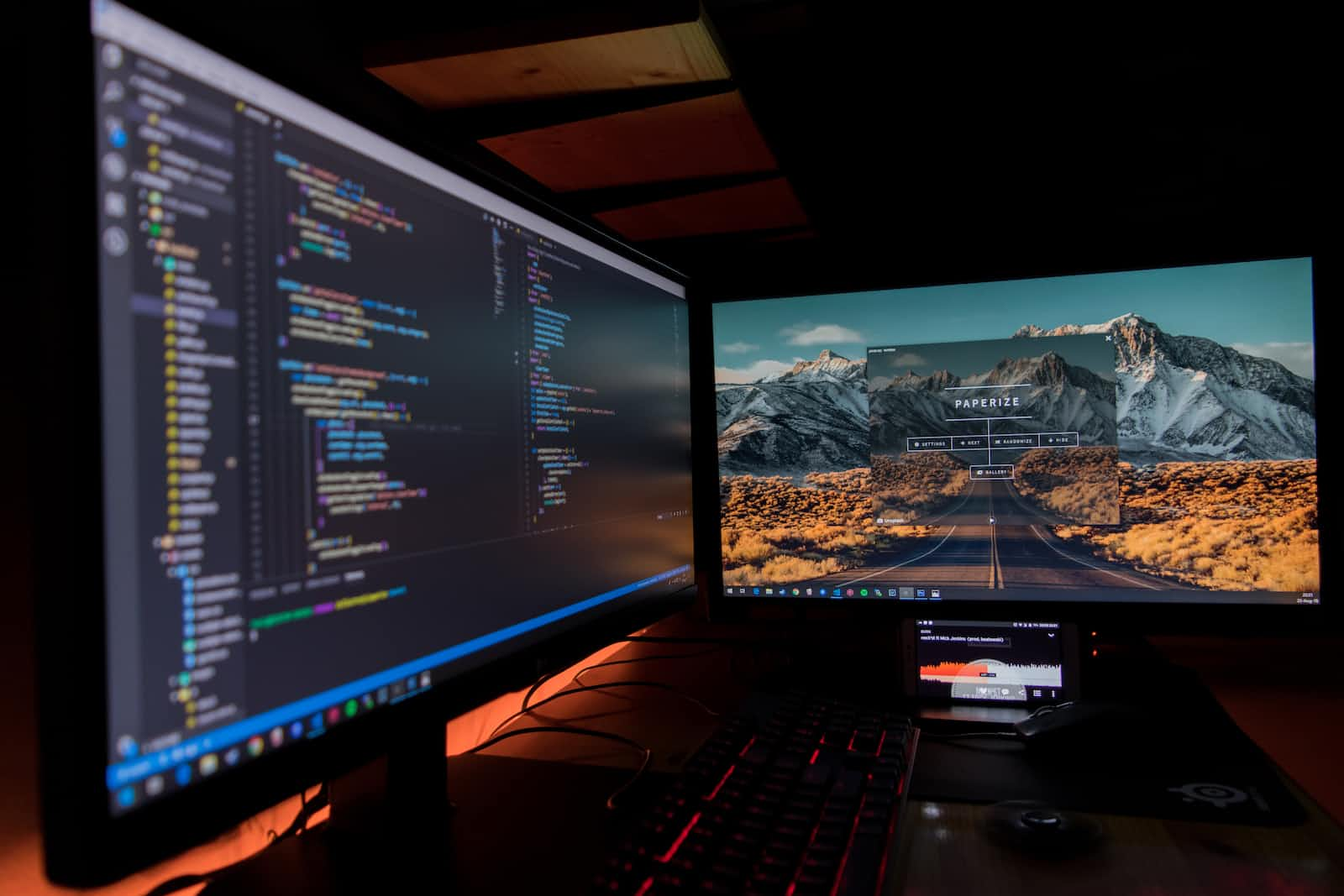
In the world of creating websites, it's like starting with a blank page and a burst of ideas. I took a little break recently from writing blogs, sort of on purpose, to figure out the best way to do things. Now, I've decided to take on a fun project: making a blog website. But don't worry, I'll guide you through it step by step, making sure we're all on the same page.
In this series of blog posts, I want to show you how to make your own blog site using Next.js and Keystatic. They're like the special tools we need to bring our ideas to life online. Think of it as crafting something cool with a digital toolkit!
In this first part, we'll start with the basics. We'll set things up, kind of like getting the foundation ready for a house. It might sound a bit technical, but I promise to explain it all in simple terms. We'll take it slow and steady, making sure we don't miss any important steps.
But wait, there's more to this adventure! We're not just stopping at the beginning. We're going to keep going until we've got our blog up and running for everyone to see. It's like putting the finishing touches on a masterpiece and then displaying it for the world to enjoy, thanks to a special place called Vercel.
As we move forward, we'll learn about designing our blog, adding cool features, and making it work really well. It's going to be a mix of creativity and technology, and I'm here to help you every step of the way.
So, let's jump right in and start with the very first chapter of our journey: getting things ready for our Next.js and Keystatic-powered blog. Get ready for a fun ride filled with learning and creating!
Prerequisites
Before we dive into the exciting world of building your own blog website with Next.js and Keystatic, there are a couple of things you should be familiar with. Don't worry, I'll keep it simple!
Basic Understanding of React: Having a grasp of the fundamentals of React, a popular JavaScript library for building user interfaces, will be really helpful. If you've played around with components, state, and props, you're on the right track.
Introduction to Next.js: It's good to have a bit of familiarity with Next.js, a framework that works with React and simplifies the process of building web applications. If you know how Next.js handles routing and server-side rendering, you'll be in a great position.
If those prerequisites sound a bit intimidating, don't fret! I'll explain concepts along the way and provide references for you to brush up on if needed. Remember, this journey is about learning and growing. So, if you're ready to embark on this adventure of creating your own blog site, let's get started!
What is Next.js?
Imagine Next.js as a super helper for creating websites. It's like a magic box filled with tools that make building websites easier. Instead of just showing plain pages, Next.js can make your pages load faster and look better. It's like making sure your guests at a party have a super comfy chair to sit on before they even arrive!
What is Keystatic?
Keystatic is a content management system (CMS) that works seamlessly with Next.js. Think of it as the engine that powers the content of your website. With Keystatic, you can easily manage and update your blog posts, images, and other content without diving into complex code. It helps you separate content creation from coding, making your life as a developer and content creator much easier.
Why use Next.js and Keystatic to create a blog website?
Choosing Next.js and Keystatic for your blog website comes with several advantages:
Faster Loading: Next.js's server-side rendering speeds up page load times, improving user experience and SEO.
Efficient Development: Next.js simplifies complex tasks, letting you focus more on creating features and less on setup.
Great User Experience: Faster-loading pages and smooth transitions create a better browsing experience for your readers.
Easy Content Management: Keystatic's intuitive CMS interface empowers you to manage your blog content effortlessly.
Flexibility: Next.js allows you to build static sites, server-rendered apps, or a mix of both, giving you flexibility in how you create your website.
SEO Benefits: Server-side rendering and proper metadata handling in Next.js contribute to better search engine visibility.
Community and Support: Both Next.js and Keystatic have active communities, ensuring you can find help and resources when needed.
By combining the power of Next.js with the content management capabilities of Keystatic, you'll be equipped to create a blog website that's not only visually appealing but also high-performing and easy to manage. It's a dynamic duo that empowers you to bring your creative vision to life without getting lost in technical complexities. In the upcoming sections of this series, we'll explore how to set up and integrate these tools step by step.
Next.Js Setup - with Tailwindcss
Start by creating a new Next.js project if you don’t have one set up already.
npx create-next-app@latest
As you can see from the above image, I have selected Yes for Tailwind CSS which we will use for our styling.
Next will be to enter your project directory and start the server by running the command npm run dev
. This will start the Next.js project on http://localhost:3000.
Adding Keystatic to the Next.js project
NB - From keystatic documentation.
We're going to need to install a few packages to get Keystatic going.
Let's install two packages from npm:
npm install @keystatic/core @keystatic/next
Creating a Keystatic config file
Keystatic needs a config file. This is where you can connect a project with a specific GitHub repository and define a content schema.
Let's create a file called keystatic.config.ts
in the root of the project:
// keystatic.config.ts
import { config, fields, singleton } from '@keystatic/core'
export default config({
storage: {
kind: 'local',
},
singletons: {
homepage: singleton({
label: 'Homepage',
path: 'src/content/_homepage',
schema: {
headline: fields.text({ label: 'Headline' }),
},
}),
},
})
We export a config object wrapped in the config
function imported from @keystatic/core
.
For now, we set the storage
strategy to local
, and we create a “homepage” singleton
which contains one text field: headline
.
With that, we are done with all configuration Keystatic needs to start managing content.
Now, let's display the Keystatic Admin UI on our site!
Adding Keystatic Admin UI pages
The Keystatic Admin UI runs in NextJS' app directory.
In your src/app
directory, we want every route within the /keystatic
segment to become a Keystatic Admin UI route.
Create a keystatic
folder in your src/app
directory, and add the following files to help with our keystatic admin layout.
// src/app/keystatic/keystatic.ts
"use client";
import { makePage } from "@keystatic/next/ui/app";
import config from "../../keystatic.config";
export default makePage(config);
// src/app/keystatic/layout.tsx
import KeystaticApp from "./keystatic";
export default function RootLayout() {
return (
<html>
<head />
<body>
<KeystaticApp />
</body>
</html>
);
}
To ensure all routes in the keystatic segment work as expected, we can leverage Next.js' Optional Catch-all Segments to match file paths of any depth.
Let's create a new folder called [[...params]]
, and add a page.tsx
file with the following:
// src/app/keystatic/[[...params]]/page.tsx
export default function Page() {
return null;
}
Before we can actually start reading and writing content via the Keystatic Admin UI, we need to create some API routes
for Keystatic.
Adding Keystatic API Routes
Create a new file at app/api/keystatic/[...params]/route.ts
Once again, we use Next.js's dynamic route segments here.
// src/app/api/keystatic/[...params]/route.ts
import { makeRouteHandler } from '@keystatic/next/route-handler';
import config from '../../../../../keystatic.config';
export const { POST, GET } = makeRouteHandler({
config,
});
We should be all set to go for our Keystatic Admin UI now.
Try and visit the /keystatic
page in the browser one more time, and click on the “Homepage” singleton:
In our Keystatic config file, we have set the storage
kind to local
. For our homepage
singleton, we set the path
property to the following:
path: 'src/content/_homepage',
If you edit the Headline and click create then look in the src
directory, a new content
folder should have been created.
And with that, we are done with the base setup we need.
Resources:
https://github.com/marville001/nextjs-keystatic-blog
In the next part, we will check on displaying Keystatic content on the Next.js front-end.
That's all for part 1.
Keep coding :)
🤖
Subscribe to my newsletter
Read articles from Martin Mwangi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
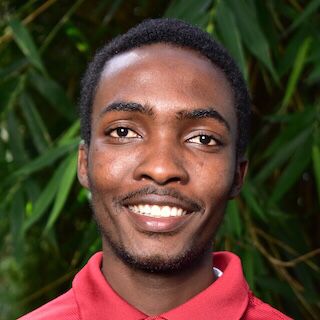
Martin Mwangi
Martin Mwangi
Hello, World :). I am Martin, a software developer. I am passionate about web development and my favorite stack is React.js and Node.js. When I am not coding I love reading books, and articles and exploring the internet (mostly dribbble).