Pseudorandom Number Generators

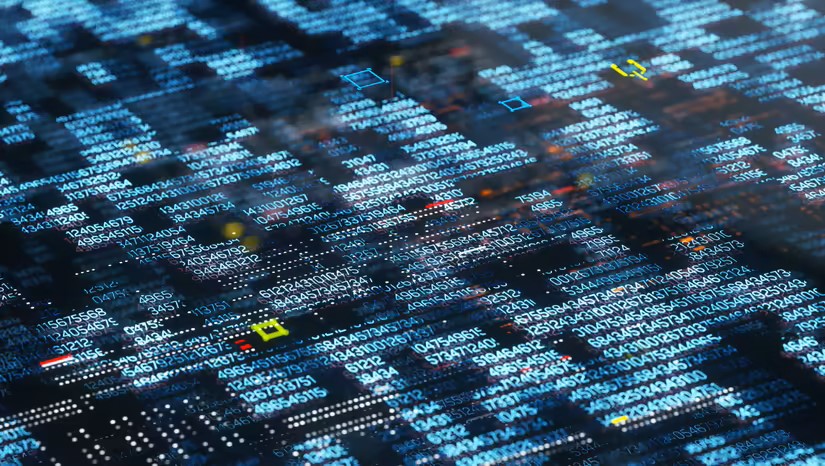
Proem
In the realm of computer science and programming, randomness is often a useful and sometimes essential feature. Whether it’s for simulations, cryptography, game development, security purpose or any application that requires unpredictable values, PseudoRandom Number Generators (PRNGs) play a significant role. Python, a popular programming language, offers a versatile set of tools for generating pseudorandom numbers.
Let’s delve into the concepts of pseudorandom number generators in Python.
Understanding the concept of PRNGs
A pseudorandom number generator is an algorithm that generates a sequence of numbers that appear to be random but are actually deterministic. This means that given the same initial conditions, a PRNG will always produce the same sequence of numbers. PRNGs are widely used due to their speed and repeatability. However, they should not be used for security-critical applications, such as cryptographic systems, as their predictability makes them vulnerable to certain attacks.
Python offers several built-in functions for generating pseudorandom numbers, primarily through the random module. The random module provides functions to generate random floating-point numbers, integers and even make choices from sequences.
How do PRNGs work?
Pseudo-random number generators (PRNGs) work by using a mathematical formula that takes an initial value called a seed and generates a sequence of numbers. Each generated number becomes the seed for the next iteration. These algorithms aim to produce sequences that appear random based on the seed, even though they are deterministic in nature. The key steps are:
Seed Initialization: The PRNG starts with an initial seed value, usually provided by the user or system.
Number Generation: The PRNG applies a mathematical formula to the seed to generate the first pseudo-random number.
Updating Seed: The generated number becomes the new seed for the next iteration.
Repeating the Process: The process is repeated, generating a new number using the updated seed, and the cycle continues.
The Role of 'Seeds'
Seeds are crucial elements for generating pseudo-random numbers. Look at this picture below.
Of course not, the above picture is only for reference that just as a seed is a starting point for the growth of a plant, a seed in a PRNG is a starting point for the generation of a sequence of numbers, basically the initial value. By setting the seed, we can ensure reproducibility. If we use the same seed value, we will obtain the same sequence of pseudorandom numbers every time we run the program.
Generating pseudorandom numbers using the random Module in Python
Python’s random module is a powerful tool for generating pseudorandom numbers. Let’s explore some of the key functions within this module:
random()
: This function generates a random float between 0 and 1 (including 0 but excluding 1).
import random
random_float = random.random()
print(random_float)
Output :
0.5212009010991806
randint(a, b)
: This function generates a random integer between a and b (inclusive).
random_integer = random.randint(1, 10)
print(random_integer)
Output :
7
uniform(a, b)
: This function generates a random float between a and b (including both).
random_uniform = random.uniform(2.5, 5.5)
print(random_uniform)
Output :
4.947899634681125
seed(a=None)
: This function initializes the random number generator with the provided seed. If no seed is provided, the current system time is used as the seed.
seed_value = 42
random.seed(seed_value)
Reproducibility and Experimentation
Seeds are invaluable when you want to reproduce the same sequence of random numbers. This is particularly useful when debugging or sharing code with others, ensuring that everyone works with the same random data. By setting a seed at the beginning of the code, we can create a controlled environment for experimentation.
seed_value = 123
random.seed(seed_value)
for _ in range(5):
print(random.random())
Now, the output will be something like :
0.7687850531442355
0.6219695842280818
0.15355509331210202
0.1306523867616743
0.8225775534392175
Note: These outputs might differ if you run the codes on a different system.
Conclusion
Pseudorandom number generators are essential concepts in computer programming, offering controlled randomness for various applications. While pseudorandom numbers are not truly random, they are valuable for simulations, testing, and other scenarios where true randomness isn’t necessary.
Python’s random module simplifies the process of generating pseudorandom numbers and using seeds enhances reproducibility, making it easier to debug and share code. Remember, while PRNGs have their limitations, they remain a fundamental tool in the programmer’s toolbox.
As this article draws to a close, let's embrace the promise of tomorrow. Just as a seedling evolves into a towering tree, so too will our understanding of this topic continue to flourish. In future explorations, I hope to unveil even more layers, revealing their beauty and significance to you. Dare to code !!
Subscribe to my newsletter
Read articles from Girija Shankar Panda directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Girija Shankar Panda
Girija Shankar Panda
Hey everyone, I am Girija, a developer by passion and an undergrad student at Institute of Technical Education and Research. With a penchant for innovation and a thirst for knowledge, I'm embarking my journey on @Hashnode and eager to share my experiences and insights. Through my blog, I am offering fellow developers and enthusiasts a platform to learn and grow. Join me on this exciting endeavor as we explore the fascinating realm of programming together.