super() / super(props) in React Class Components and Why?
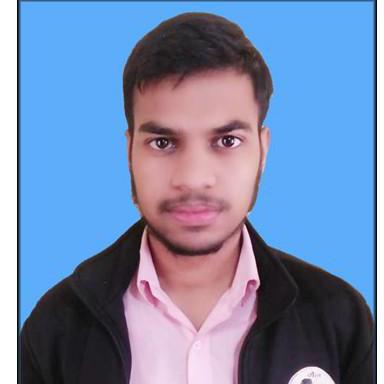
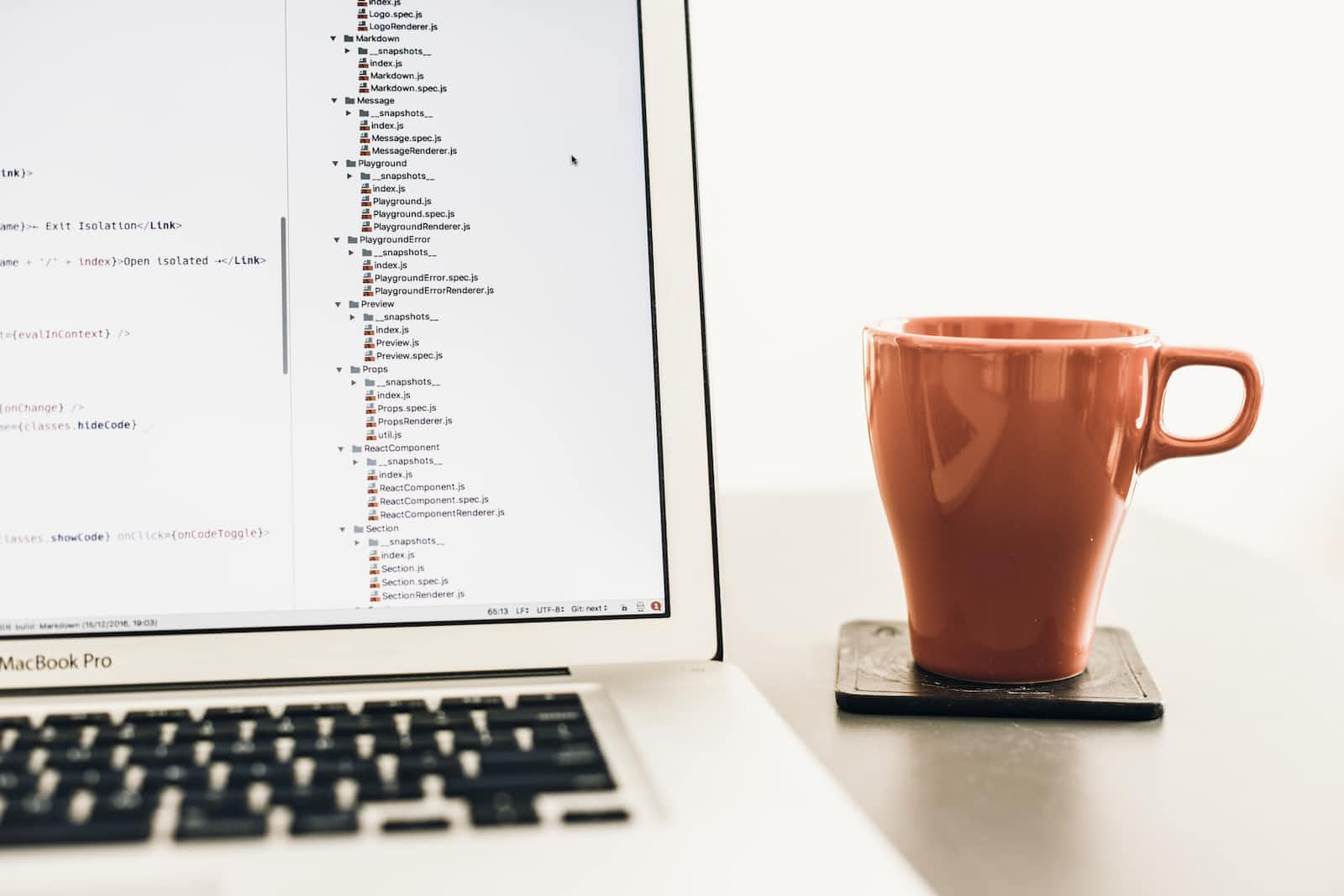
If you have this question, then you should, first of all, visit the basics of inheritance in Javascript and get the answer.
Let's look at the example
Create a parent class with a constructor and a few functions.
class parent{
val = 4;
constructor() {
this.val = 8;
}
printval1 = function() {
console.log(this.val)
}
printval2 = function() {
this.printval1()
}
}
Create a child class which tries to access a variable in the parent class.
class child extends parent{
printval3 = function() {
console.log(this.val)
}
}
Creating an instance of child and invoking printval3 will print '8' as expected.
But if you have a constructor in the child class with nothing in it, it will result in undefined with this code.
class child extends parent{
constructor(){
}
printval3 = function() {
console.log(this.val)
}
}
Because when we write a constructor, the class is constructed according to whatever we write in this method. So to retrieve all the powers of the base class javascript provides a method called super and writing super in the constructor (and it is necessary otherwise js will throw an error) of the child class will result in invoking the constructor of the parent class and thus providing all the functionality of the base class.
class child extends parent{
constructor(){
super()
}
printval3 = function() {
console.log(this.val)
}
}
And the output will turn to '8'.
This will answer why we need to write super() in every class component - to extract all the functionalities of the React.Component in our current component.
But why should we provide it with props?
Consider another example with a tweak -
class parent{
val = 4;
constructor(v) {
this.val = v;
}
printval1 = function() {
console.log(this.val)
}
printval2 = function() {
this.printval1()
}
}
class child extends parent{
constructor(props){
super(props)
}
printval3 = function() {
console.log(this.val)
}
}
var c = new child(10);
c.printval3();
Writing super(props) will result in logging 10 and writing super() will result in undefined in the console.
Because in the implementation of React.Component constructor, it expects a props parameter and assigns the same value to base class props. So you can use this.props anywhere in the code.
To sum up -
class Comp extends React.Component{
constructor(props){
super(props)
}
render(){
return (
<div>
{
this.props.prop1 // defined value
}
</div>
)
}
}
class Comp extends React.Component{
constructor(props){
// empty here - error in console
// super() - this.props as undefined
// super(props) - this.props as defined
}
}
Anything else about this topic should be put in comments so, every doubt is cleared.
Subscribe to my newsletter
Read articles from Akash Bansal directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
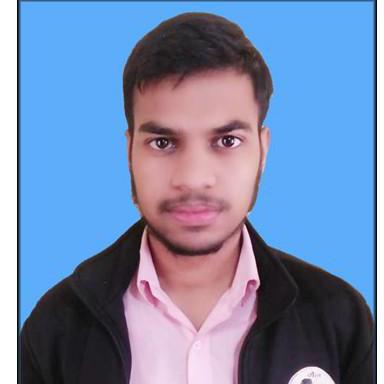