Mastering Game Flow: Pygame's Secret Sauce for Seamless State Transitions!
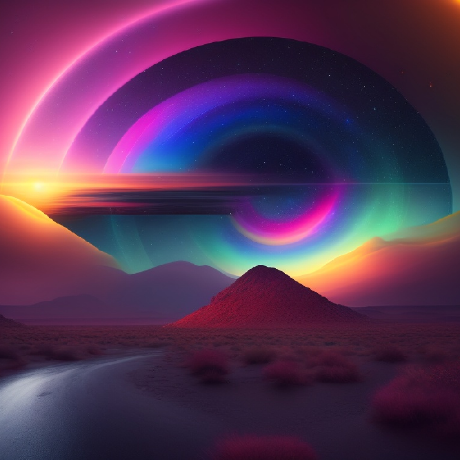
Table of contents
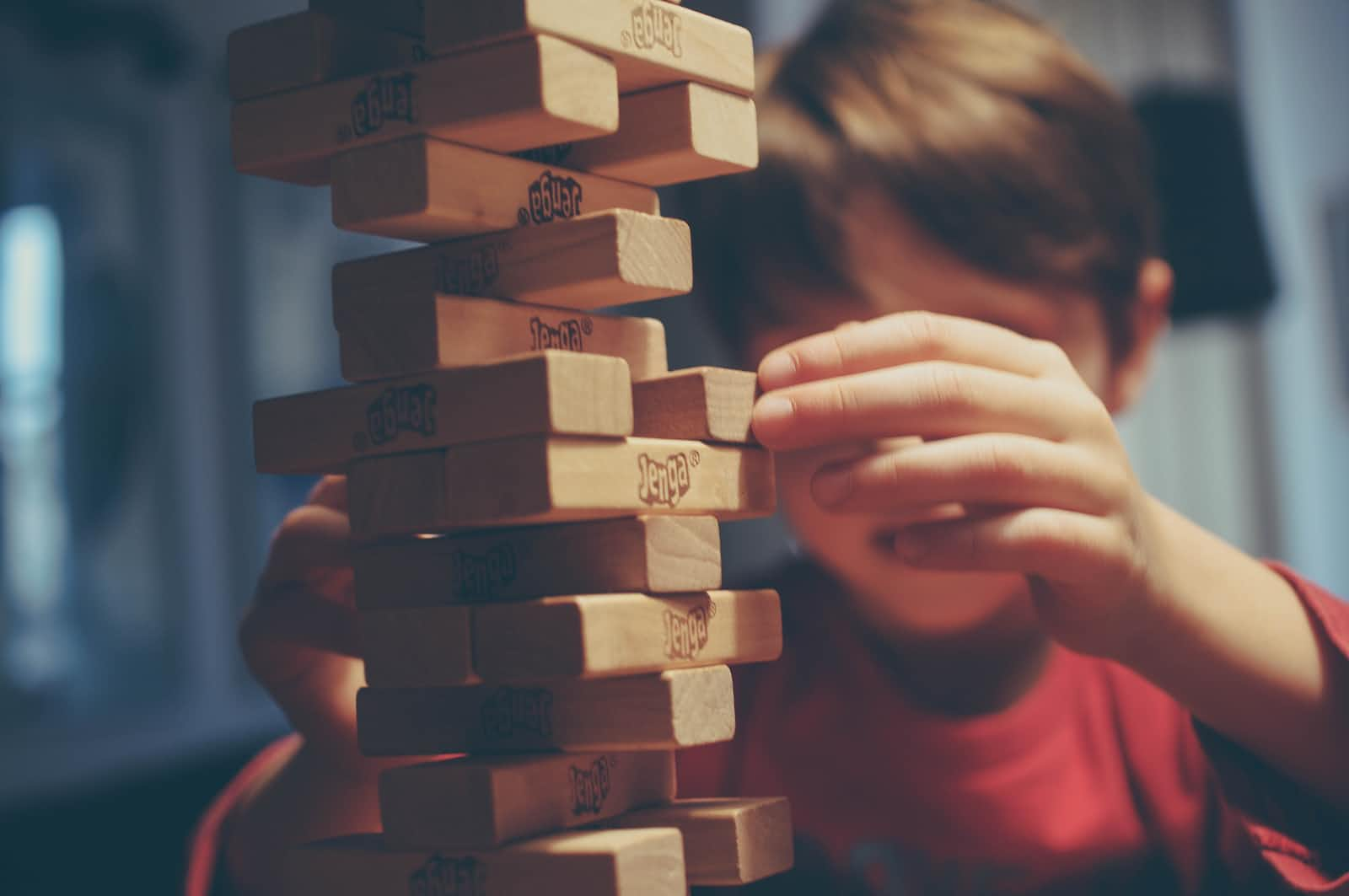
In Pygame, a popular Python library for creating 2D games, game states and transitions are important concepts that help you organize and manage different parts of your game. Game states represent different stages or screens of your game, such as the main menu, gameplay, pause menu, game over screen, etc. Transitions involve moving between these states smoothly to create a coherent gaming experience.
Here's a general guideline on how to implement game states and transitions in Pygame:
Game State Management: You can define different classes or modules for each game state. Each class/module should handle the logic and rendering for its specific state. For example, you might have classes like
MainMenuState
,GameState
,PauseState
, andGameOverState
.State Initialization: Each state class should have an initialization function (
__init__
) where you set up the necessary variables, load resources, and perform any initializations specific to that state.Input Handling: Depending on the state, you might want to handle input differently. For example, the main menu state might respond to "start" or "quit" commands, while the gameplay state would respond to player movement and actions.
Update and Render: Each state should have an update method that processes logic and an associated render method that draws the state's visuals onto the screen.
State Transitions: To transition between states, you need to manage which state is active at any given time. One common approach is to use a state manager or a stack to keep track of active states. When you want to switch states, you push the new state onto the stack and pop the previous state off.
State Switching: Implement methods within your state manager to switch between states. For example, you might have methods like
push_state
,pop_state
, andset_state
.Main Game Loop: In your main game loop, you'll repeatedly update and render the current active state. This loop ensures that the game logic and visuals are continuously processed.
Here's a simple code outline that demonstrates this concept:
import pygame
import sys
class MainMenuState:
def __init__(self):
# Initialize menu resources and variables
def handle_input(self):
# Handle menu-specific input
def update(self):
# Update menu logic
def render(self, screen):
# Render menu visuals onto the screen
class GameState:
def __init__(self):
# Initialize game resources and variables
def handle_input(self):
# Handle gameplay-specific input
def update(self):
# Update gameplay logic
def render(self, screen):
# Render gameplay visuals onto the screen
# Initialize Pygame
pygame.init()
# Create a screen
screen = pygame.display.set_mode((800, 600))
# Initialize states and state manager
states = [MainMenuState(), GameState()]
current_state = 0 # Index of the active state
# Game loop
while True:
# Handle events
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
# Handle input, update, and render the active state
states[current_state].handle_input()
states[current_state].update()
states[current_state].render(screen)
pygame.display.flip()
pygame.time.Clock().tick(60) # Cap the frame rate
Remember that this is just a basic outline. Depending on the complexity of your game, you might need to add more features and details to the state management system. It's also a good practice to organize your code into separate files or modules for each state to keep your project well-structured and maintainable.
Subscribe to my newsletter
Read articles from Yusuf Adeagbo directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
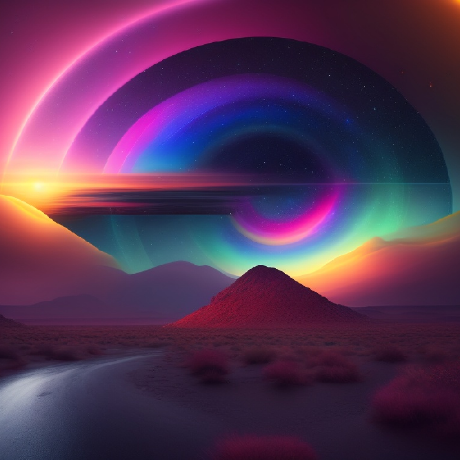
Yusuf Adeagbo
Yusuf Adeagbo
Frontend dev HTML/ CSS/ JS | TailwindCSS, SvelteKit | Git/GitHub | 🌱 Python.