Introduction To EJS-Embedded Javascript
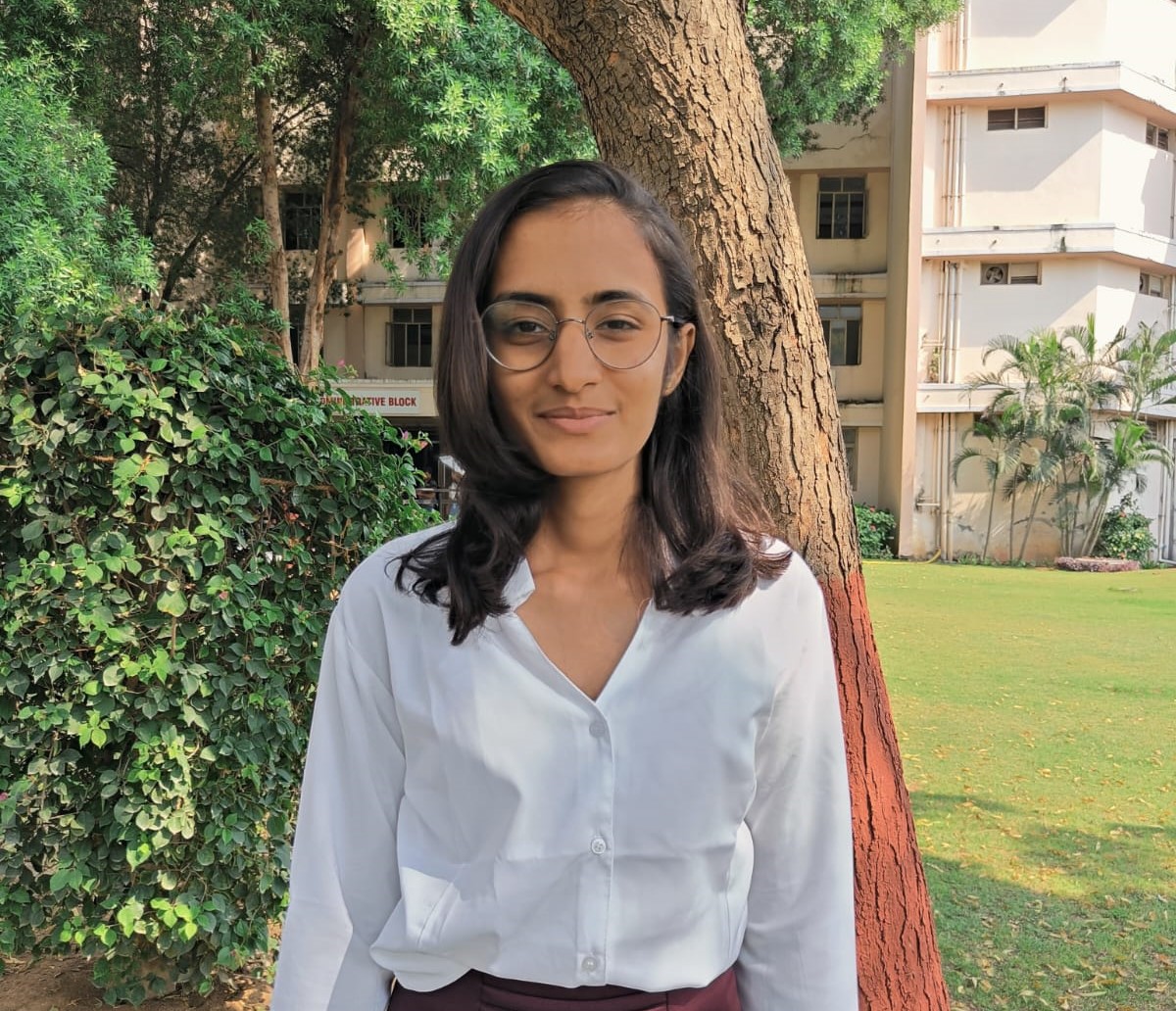
EJS stands for Embedded Javascript. Node.js makes use of this Templating engine. The template engine makes it possible to generate an HTML template with minimal coding. It can also inject data into a client-side HTML template and generate the final HTML. EJS is a basic templating language that uses plain JavaScript to build HTML markup. It is also beneficial to embed JavaScript into HTML sites.
We would try to understand the basics of EJS with the help of this example.
Prerequisite:-
Install the node on your local device.
EJS Installation:-
Firstly, we need to initialize a node.js project or package and then install express and body-parser. Just type in the commands in your favorite command line tool.
npm init -y
npm i express
npm i body-parser
File Structure:
The following files would be created in your project after installation and by making the index.js and index.html files:
Sending response to server:-
We will be sending Hello with the user name as shown below to the server and display it on the page as below:
1. First start the server by importing the express module in the index.js file
//index.js
const express = require('express');
const app = express();
app.listen(3000, function () {
console.log("Server is started on port 3000");
});
2. Make the index.html file for making a form in which the user will enter the username and as soon as the user clicks on submit they will get a response as Hello + their name.
The action of the form will be set to "/" (route handler for root URL) and the method will be set to post.
<!-- index.html -->
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<form action="/" method="post">
<input type="text" name="name"/>
<button type="submit" name="submit">Submit</button>
</form>
</body>
</html>
3. Now in the index.js file
//index.js
//import body-parser package
const bodyParser = require('body-parser');
// urlencoded:- by using urlencoded we can get access to form data.
// body-parser:- by using body-parser we can able to parse the http request that we get.
app.use(bodyParser.urlencoded({extended: true}));
//get function will let us define a route handler for GET request
//below when it is call, it will receive an HTTP GET request to / (index.html)
app.get("/", function(req, res) {
//res.send() :- function of Express.js used to send files to the client
res.sendFile(__dirname + "/index.html");
//__dirname:- global variable in Node.js that represents the directory name of the current module
//index.html:- to send as a response on server
});
app.post("/", function(req, res){
//Extract name parameter which user have given
var name = req.body.name;
//sends a response back to the client.
//It creates an HTML response which has a name value given by user
res.send(`<h1>Hello ${name} </h1>`);
});
app.get(): When a user accesses the root URL in their web browser, this code will be executed
res.sendFile(__dirname + "/index.html"): It sends a file in response to the client.
Now run the below command in terminal
node index.js
4. After running it the server will start on the given port.
Now type the name you will get a response as Hello your name.
Why to use EJS in above example?
The above res.send thing will work for only simple/small tasks. But what if we want to send a whole HTML page as a response then we have to write the whole HTML code inside res.send then index.js file clustered. All of the HTML files related to the structure are now being embedded into a javascript file which is our server-side code that is meant to deal with functions that are not good so to avoid that we will use Separate of Concern.
Separate of Concern:-
We can separate HTML (Structure), CSS (Style) and Javascript (Functionality) that is we can separate Frontend and Backend.
This Separate Concern is more important than starting building complex project. It will be nice to work on one small thing at a time and not to hold the whole complexity of our website.
To do Separate of Concern we use Templating Language.
Templating Language:-
There are many templating languages and they are used for different language too. Some are listed below:-
pug
jinja:- use for python
twig:- use for PHP
EJS:- commonly used with express and node
In EJS, there will be a filename with extension .ejs which includes a html file which has some EJS tags too.
Thank you for reading.
We will dicuss more about EJS in next part.
Keep Learning.
Pic Reference:
1. https://dev.to/efecollins/blog-template-made-with-ejs-nodejs-expressjs-d38
Subscribe to my newsletter
Read articles from Kansariwala Hetashri Dhavalkumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
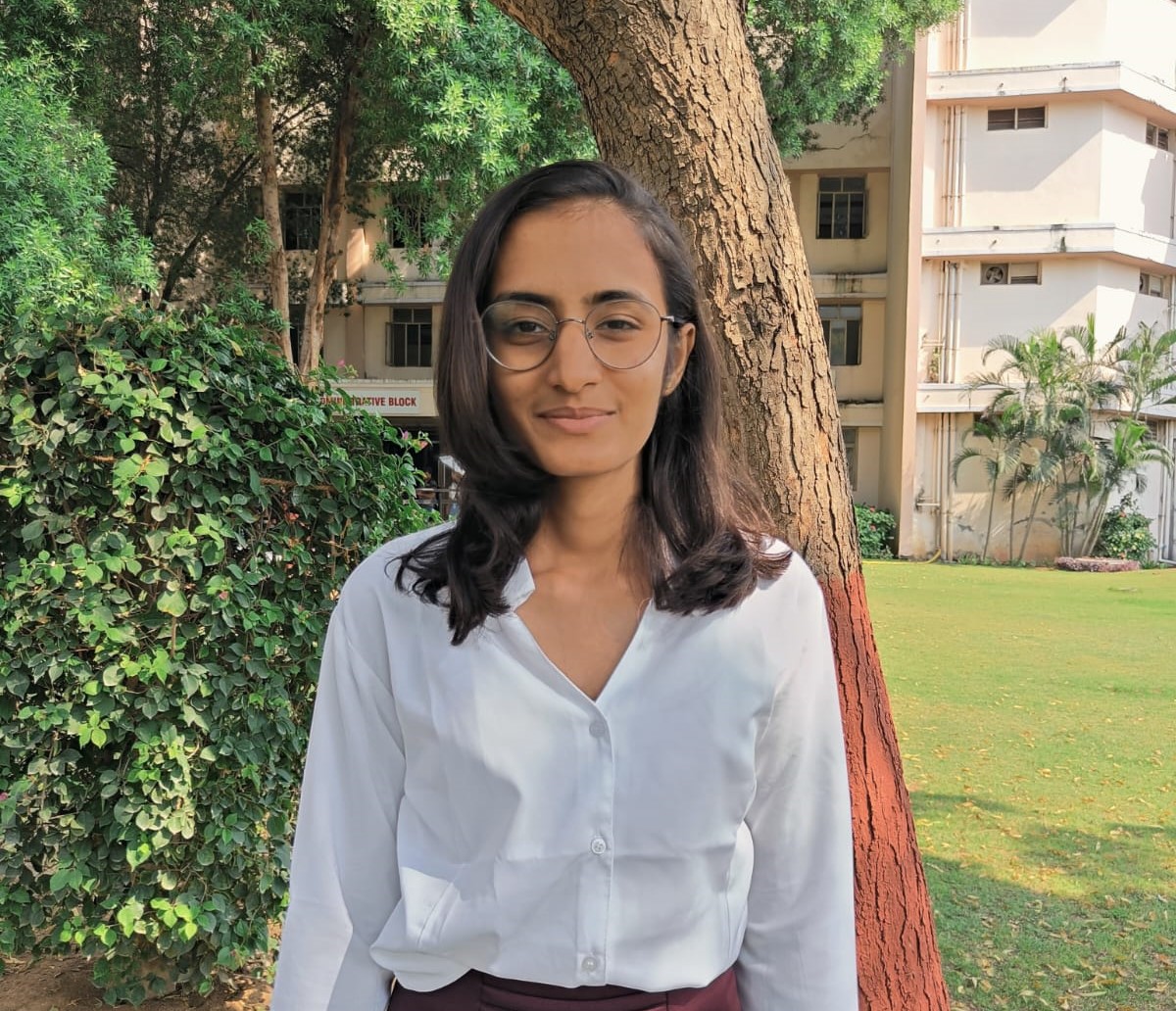
Kansariwala Hetashri Dhavalkumar
Kansariwala Hetashri Dhavalkumar
I am a Front-End Developer, Open Source Contribution.