Clearing Away the Clutter with Proxy Objects
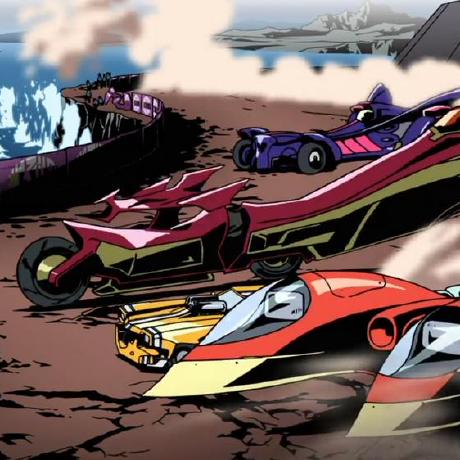
Modern web apps let users view data across multiple tabs. Product filters, selections, and more become isolated little sandboxes. Now imagine clearing filters in one tab also wiped out the others! Without care, state can become tangled across UI components. Proxy objects elegantly isolate state to prevent such headaches.
Let’s look at an example app. We’re building an ecommerce site that shows products in a grid. Users can open the catalog in multiple tabs and apply filters - but we need these selections kept separate.
Our first attempt stores filters in a shared object:
// Filters from all tabs
const filters = {
tab1: {size: 'small'},
tab2: {brand: 'Acme'}
};
This works initially. But as more tabs open, it becomes unwieldy. And we’ll need messy logic to isolate filter clearing.
Enter proxy objects to cut the strings! Proxies allow intercepting operations like setting properties. We can use them to encapsulate tab state.
Here is a refactor with proxies:
// Track open tabs
const tabs = [];
// Open tab
function openTab() {
// Create proxy for this tab
const filters = createFiltersProxy();
// Add to tabs list
tabs.push({
id: Date.now(),
filters
});
}
// Tab filter proxy
function createFiltersProxy() {
return new Proxy({size: ''}, {
set(target, key, value) {
// Logic to isolate filters
}
});
}
When a new tab opens, we create a proxy to manage its filters. The proxy intercepts filter changes, letting us isolate logic per tab.
Now clearing filters only affects one tab:
function clearTabFilters(tabId) {
const tab = tabs.find(id === tabId);
tab.filters.size = '';
}
The proxy encapsulates the change. Other tabs remain intact.
Under the hood, proxies override operations like setting properties. When we update a filter:
The proxy handler runs
We override the behavior
The change is isolated to one tab
No tangled conditional logic!
Benefits of the Proxy Pattern
Encapsulate state per component
Limit effects of changes
Avoid convoluted code
Dynamic proxy creation
Scale as complexity increases
So when state gets out of hand across UI elements, reach for a proxy. Proxies elegantly isolate behaviors to prevent tangled dependencies. They are an invaluable tool for web developers.
Subscribe to my newsletter
Read articles from Kshitij Kakade directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
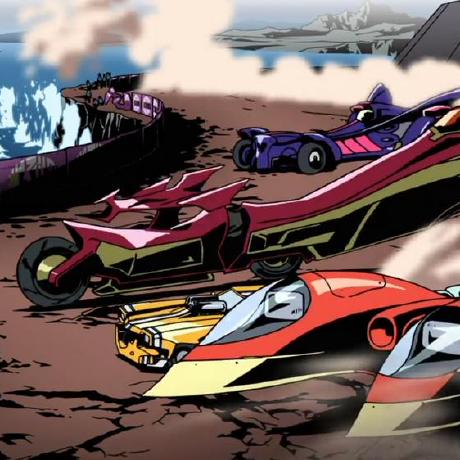