Python Control Flow Statements - Chapter : 4
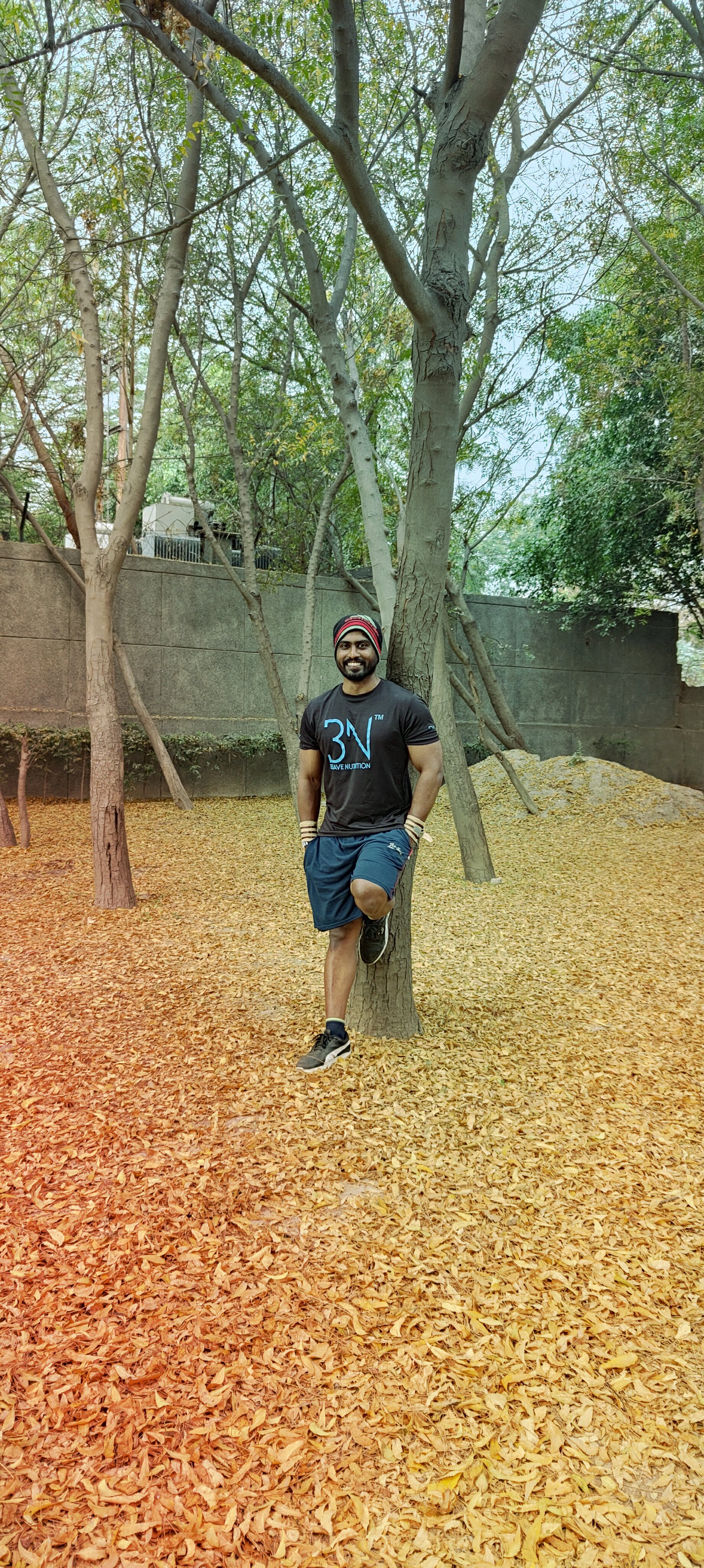
Table of contents
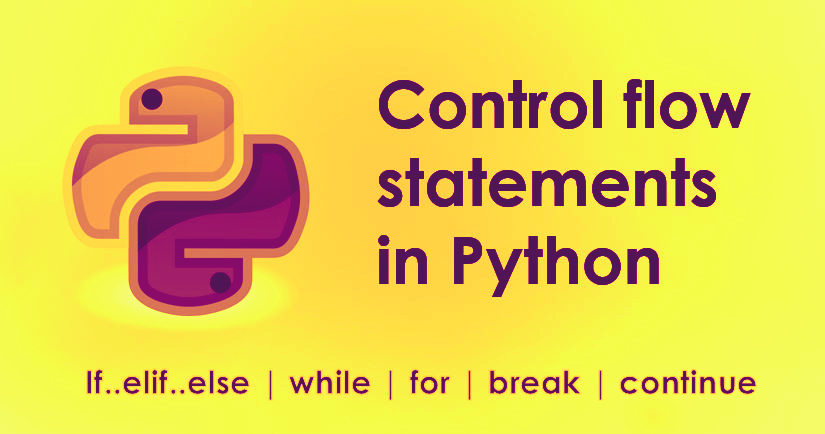
Flow of Execution:
Execution in a Python program begins with the very first statement. How the statements are executed defines the flow of execution in a Python program, which is categorized as follows:
1) Sequential Statements: Sequential statements in Python are a series of instructions executed one after another in the order they appear in the code. They form the basis of any program and define the logical sequence of actions to be performed. Here are some examples of sequential statements in Python:
In the above examples, the statements are executed one by one in the order they appear in the code. Python's sequential execution is the fundamental way to achieve specific tasks and build more complex programs by combining sequential statements with flow control structures like conditionals and loops.
2) Selection / Conditional Statements: Conditional statements in Python allow you to make decisions based on certain conditions. They control the flow of the program by executing different blocks of code depending on whether a specified condition is true or false. The primary conditional statements in Python are:
--> If: The simplest form is the if statement. If the condition is true, the indented block of code under the if
statement is executed.
Syntax:
Eg:
a) If the condition is True, then the indented statement gets executed. However, it will not do anything if the condition is evaluated as False.
b) The indented statement implies that its execution is dependent on the condition.
Note: Do not forget to place a colon ( : ) after the if condition or if statement as shown in the given syntax.
--> if-else: The if-else
statement is used to execute one block of code if the condition is true and another block if the condition is false.
Syntax:
Eg:
--> if-elif-else: The if-elif-else
statement is used when you have multiple conditions to check. It allows you to execute different blocks of code based on various conditions.
Syntax:
Note:
a) else is optional
b) The number of elif is dependent on the number of conditions
c) If the first condition is False, the next is checked, and so on. If one of the conditions is True, the corresponding statement(s) execute, and the statement ends.
Eg:
--> Nested if: You can nest if
statements inside each other to create more complex decision-making scenarios.
Syntax:
3) Iterative / Looping Statements: Iterative or looping statements in Python are used to repeat a block of code multiple times, based on a condition. The statements in a loop are executed again and again as long as a particular logical condition remains true. There are two main types of loops in Python: for loops and while loops.
--> for loop: The for
loop is used to iterate over a sequence (like a list, tuple, string, etc.) or other iterable objects. It executes a block of code for each item in the sequence.
The for statement is used to iterate/repeat itself over a range of values or a sequence one by one. The for loop is executed for each of these items in the range.
With every iteration of the loop, the control variable checks whether each of the values in the range has been traversed or not. When all the items in the range are exhausted, the body of the loop is not executed any more; the control is then transferred to the statement immediately following the for loop.
Syntax:
Note:
1. The sequence may be a list, tuple, string, etc.
2. 'else' statement will be executed after all the iterations of the for loop, if provided.
3. control_variable is a variable that takes a new value from the range each time the loop is executed.
range(): In Python, the range()
function is often used in conjunction with loops to generate a sequence of numbers. It's a built-in function that creates a range object representing a sequence of integers, which can be used in various looping scenarios. The range()
function has three different forms:
range(stop)
: Generates a sequence of integers from 0 up to (but not including) the specified stop value.
range(start, stop)
: Generates a sequence of integers starting from the specified start value up to (but not including) the stop value.
range(start, stop, step)
: Generates a sequence of integers starting from the specified start value up to (but not including) the stop value, with the specified step size.
Nested Loops: Loop inside another loop, which are known as nested loops. Each time the outer loop runs, the inner loop completes its full iteration. Nested loops are used when you want to iterate over a collection of items and perform a certain action for each combination of items.
- while loop: The
while
loop in Python is used to repeatedly execute a block of code as long as a specified condition remains true. It's especially useful when you don't know the exact number of iterations needed beforehand. The loop continues executing as long as the condition evaluates toTrue
. Here's the syntax and an example of using thewhile
loop:
Syntax:
while is a reserved word
condition to be checked for true/false value
code block of while consists of single or multiple statements or even an empty statement, i.e, pass statement
else is optional and is executed when the test condition evaluates to false.
Eg:
Infinite Loops:
5) Transfer Statements: In Python, transfer statements are used to control the flow of execution in your program. They allow you to change the normal flow of execution by transferring control to a different part of the program. The main transfer statements in Python are:
--> break: The break
statement is used to exit a loop prematurely, regardless of whether the loop condition has been satisfied completely.
--> continue: The continue
statement is used to skip the rest of the code inside the current iteration of a loop and move to the next iteration.
--> pass: The pass
statement in Python is a placeholder statement that does nothing when executed. It's used as a syntactic requirement in situations where some code is expected but not yet implemented or necessary. The pass
statement essentially acts as a no-op and is used to avoid syntax errors when a statement is needed but you don't want to execute any code yet.
Function or Class Definition: When you're creating a function or class but haven't yet implemented the body, you can use
pass
to satisfy the syntax requirements until you add the actual code later.Empty Loop or Conditional Block: In cases where you want to define a loop or conditional block that doesn't have any immediate code to execute, you can use
pass
to avoid syntax errors.In Mocking or Testing: When you're writing tests or placeholders for testing purposes, you can use
pass
to define test stubs that you intend to implement later.In all these cases, the
pass
statement ensures that your code is syntactically correct while allowing you to leave certain parts of the code empty until you're ready to fill them in with actual functionality.Previous Chapter: Chapter - 3
Subscribe to my newsletter
Read articles from Vimal Girija V directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
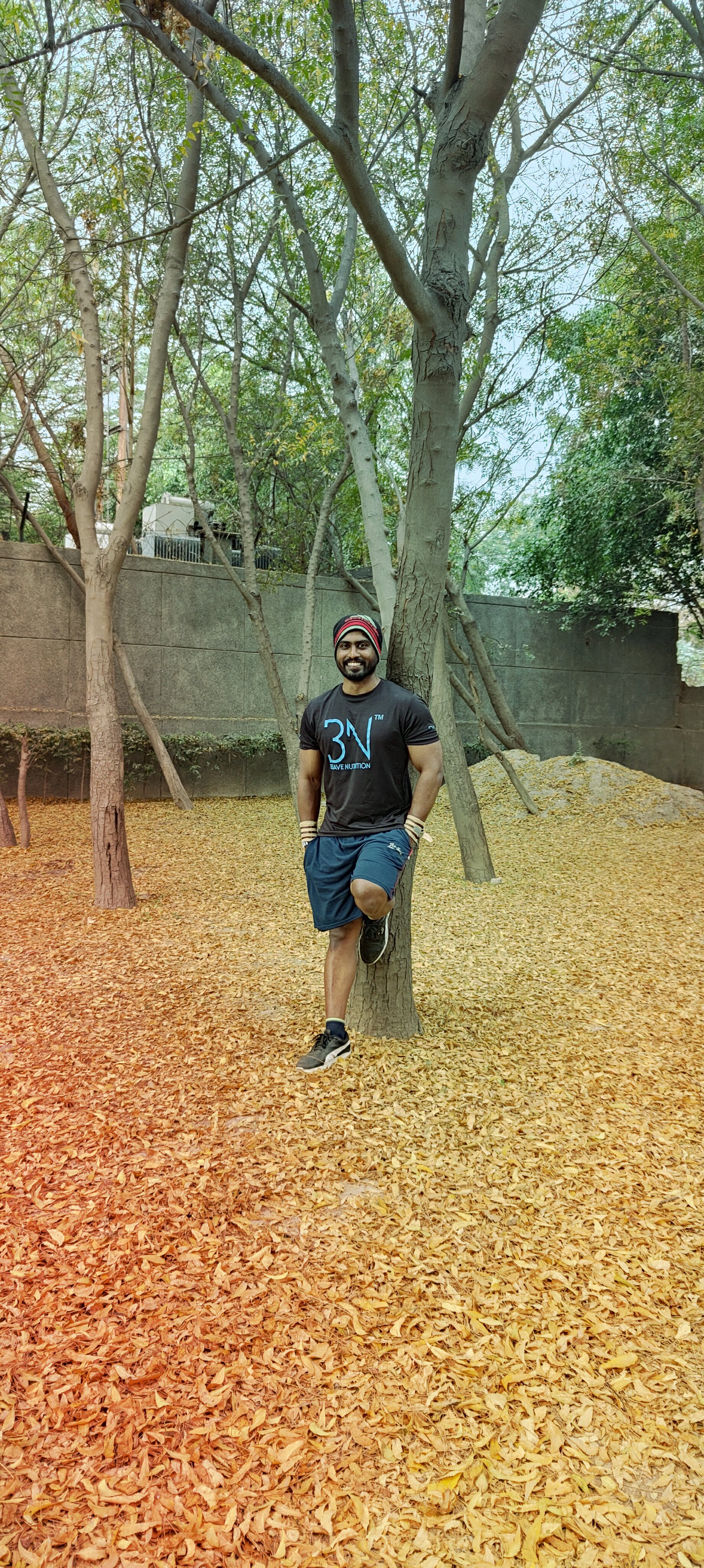
Vimal Girija V
Vimal Girija V
Hey there! I'm a passionate tech enthusiast with a diverse skill set in software development, DevOps, and cybersecurity. I love building robust APIs with Python Django and creating interactive user interfaces with React.js. My journey has taken me through the fascinating world of IoT, where I've tinkered with Arduino and Rasberry Pi devices, and I've also delved into the exciting realm of ethical hacking and penetration testing. Technologies I Know: Python Django Framework for API: I have hands-on experience in building APIs using the powerful Python Django framework, enabling me to create scalable and efficient web applications. React.js for Frontend Development: With proficiency in React.js, I craft engaging and responsive user interfaces, making the web experience seamless and enjoyable. Python Automation Testing with Selenium, Pytest, and Appium: My expertise in automation testing using Selenium, Pytest, and Appium ensures that software projects are thoroughly tested, reducing manual effort and improving the overall quality of the code. AWS DevOps with Git, Jenkins, Docker, SonarQube, Terraform, Ansible, Kubernetes, and Maven: I've mastered various DevOps tools and practices on the AWS platform, streamlining the development and deployment process. Git, Jenkins, Docker, SonarQube, Terraform, Ansible, Kubernetes, and Maven are among the tools I'm proficient in. IoT with Arduino and Raspberry Pi: Exploring the Internet of Things world, I've successfully worked with Arduino and Raspberry Pi to build exciting and innovative projects that leverage sensor data and real-world interactions. Certified Ethical Hacking and Penetration Testing: My passion for cybersecurity has driven me to become a Certified Ethical Hacker. I conduct penetration testing to identify and fix security vulnerabilities, ensuring systems and applications are protected against potential threats.