Topics to Cover on Learning Java Basics
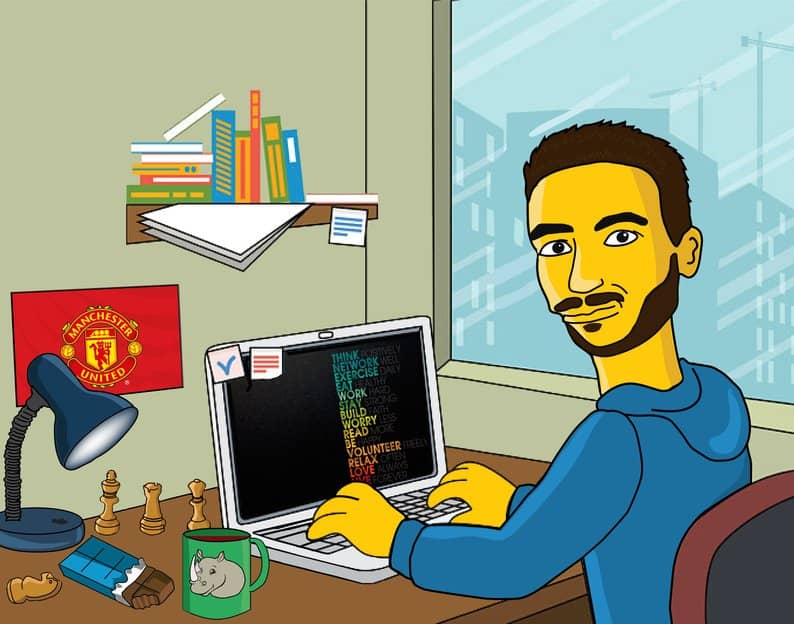
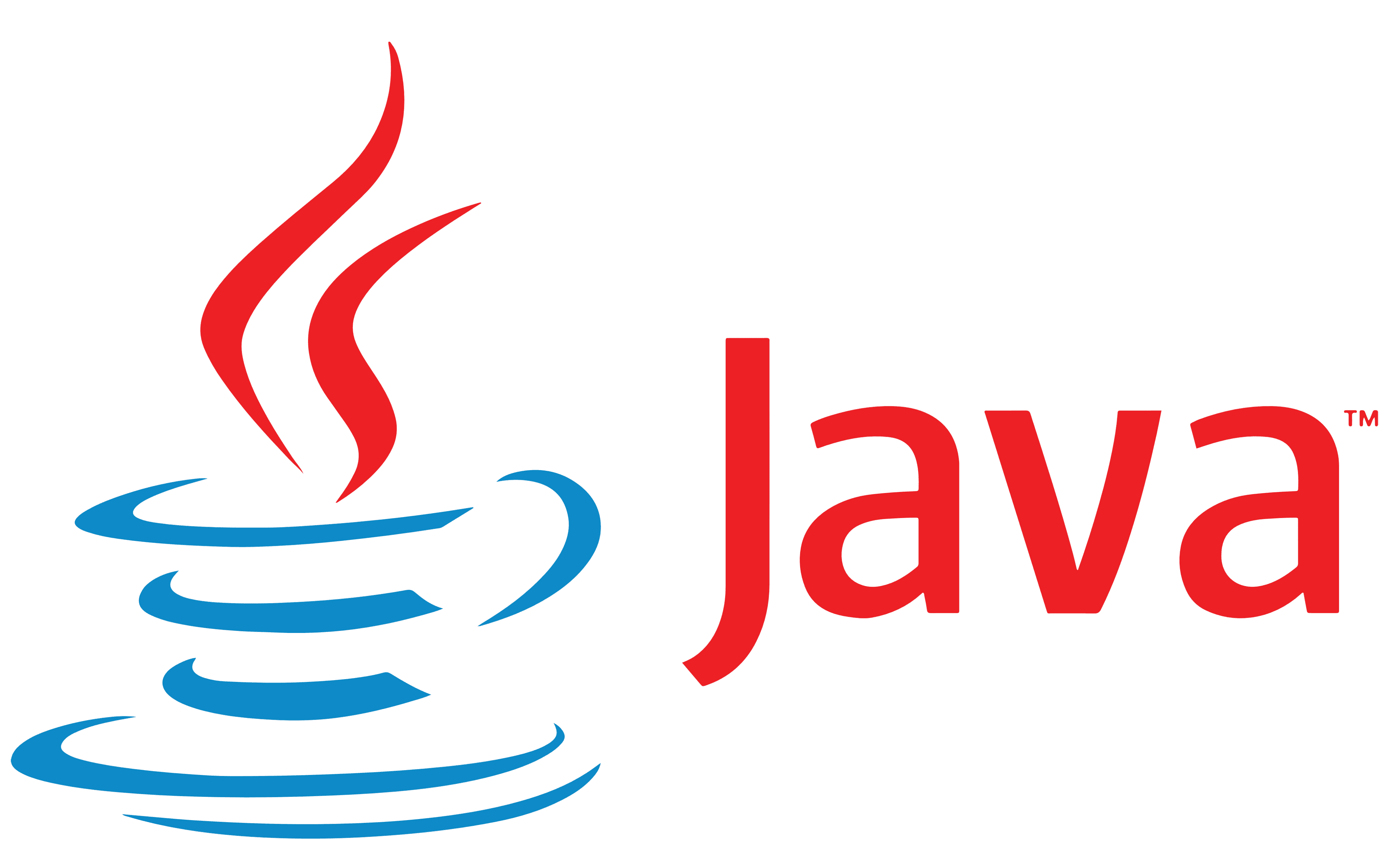
1. Introduction to Java:
What is Java and its significance
Java's platform independence
2. Setting Up Development Environment:
Installing JDK (Java Development Kit)
Choosing an IDE (Integrated Development Environment)
3. Java Syntax and Structure:
Basic syntax rules
Comments
Semicolons
4. Data Types and Variables:
Primitive data types: int, double, char, boolean, etc.
Declaration and initialization of variables
Naming conventions for variables
5. Operators:
Arithmetic operators (+, -, *, /, %)
Comparison operators (>, <, ==, !=, etc.)
Logical operators (&&, ||, !)
6. Control Structures:
Conditional statements: if, else-if, else
Switch statement
Loops: while, for, enhanced for (foreach)
Using break and continue statements
7. Methods and Functions:
Declaring and defining methods
Method parameters and return types
Method overloading
8. Object-Oriented Programming (OOP) Basics:
Classes and objects
Instance variables and methods
Constructors
Access modifiers (public, private, protected)
9. Inheritance and Polymorphism:
Creating subclasses and superclasses
Method overriding and dynamic method dispatch
Polymorphism and its benefits
10. Packages and Imports:
Organizing classes into packages
Importing classes from other packages
11. Arrays and Lists:
Declaring and initializing arrays
Accessing array elements
Java ArrayList for dynamic lists
12. Basic Input and Output:
Using the Scanner class for user input
Output with System.out
Formatting output using printf()
13. Exception Handling:
Understanding exceptions
Using try-catch blocks
Handling exceptions gracefully
14. Basic String Manipulation:
Creating and manipulating strings
String concatenation
Commonly used string methods
15. Type Casting and Conversion:
Implicit and explicit type casting
Converting between data types
16. Basic File I/O:
Reading from and writing to files
Using FileReader, FileWriter, BufferedReader, BufferedWriter
17. Java Standard Library:
Exploring Java's built-in classes and methods
java.lang, java.util, java.io, and more
Subscribe to my newsletter
Read articles from Karun directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
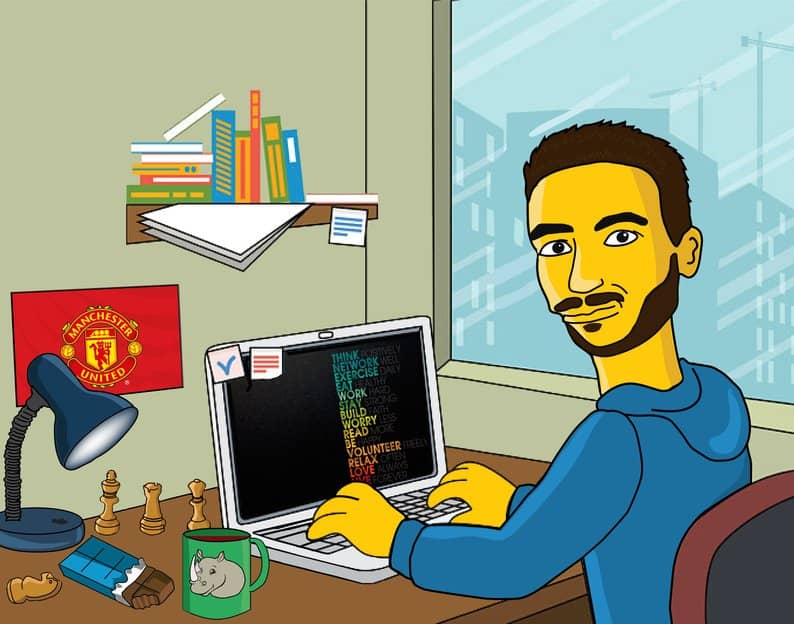
Karun
Karun
๐ Passionate Python Enthusiast | Educator | Blogger ๐ Welcome to my Python playground! ๐ I'm here to share my love for Python programming and help you master this versatile language. Whether you're just starting your coding journey or looking to level up your Python skills, you're in the right place. ๐ Dive into my tutorials and learn Python from scratch, one step at a time. From basic syntax to complex projects, I've got you covered. ๐ As an educator, I believe in the power of sharing knowledge. Let's learn, grow, and conquer coding challenges together. ๐ Got a question or a cool project idea? Don't hesitate to reach out. Remember, in the world of programming, a little indentation goes a long way. Happy coding, Pythonistas! ๐๐ป ๐ karun.hashnode.dev ๐ธ https://twitter.com/karunakarhv