Finding the Most Common Element in a List - A Python Example

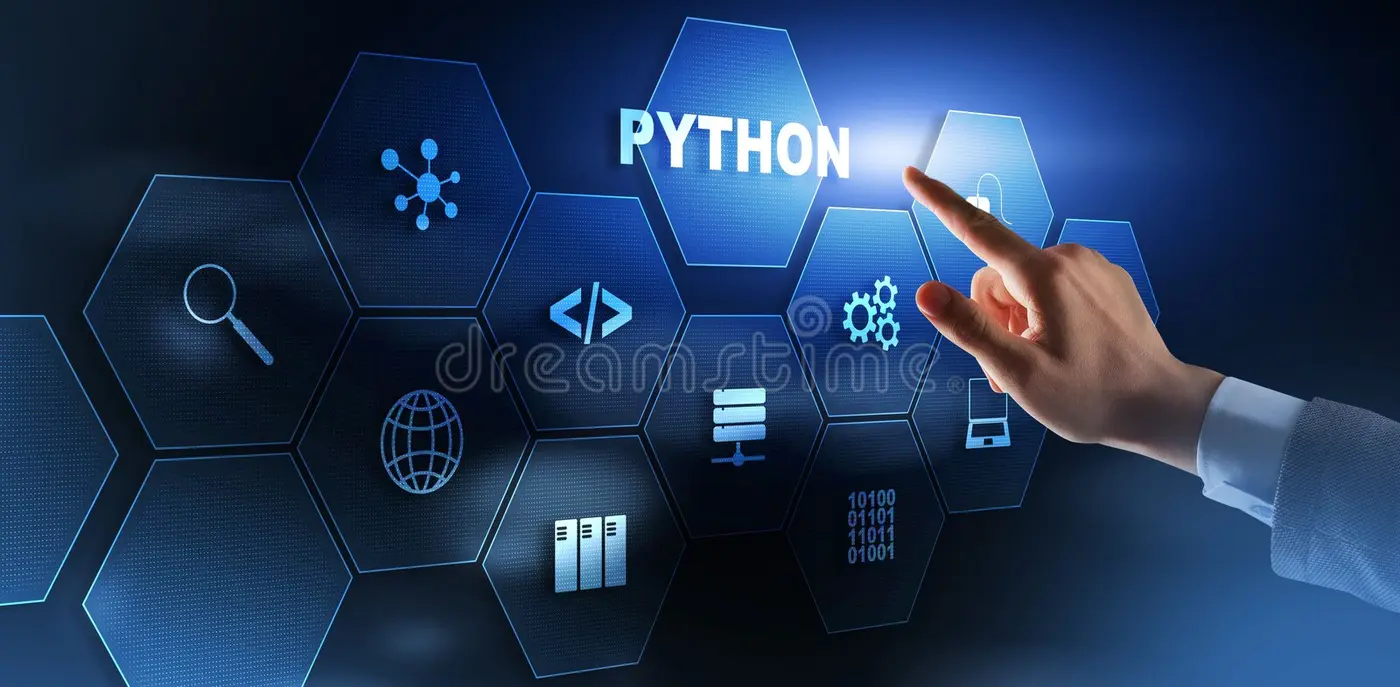
When working with data in Python, it's often necessary to analyze and manipulate lists. One common task is finding the element that appears the most frequently in a given list. In this blog post, we'll explore a simple Python code snippet that accomplishes this task efficiently.
Let's consider the following list:
list = [1, 2, 3, 1, 4, 1, 5, 6, 1]
The element that appears the most frequently in this list is the one we are looking for. We'll combine many built-in Python functions to do this by streamlining the process.
list = [1, 2, 3, 1, 4, 1, 5, 6, 1]
most = max(set(list), key=list.count)
Let's break down this code step by step to understand what each part does.
Step 1: Converting the List to a Set
In the first step, we convert the original list into a set using the set()
function. The set data structure automatically removes duplicate elements, leaving us with a collection of unique values. In our example, set(list)
becomes {1, 2, 3, 4, 5, 6}
.
Step 2: The Key Function
A key
parameter in the max()
function allows us to specify a custom function that calculates a value for each element. In our case, we use the list.count
function as the key. This function counts how many times an element appears in the original list.
Step 3: Finding the Most Frequent Element
The max()
function then iterates through the set and applies the list.count
function to each element. It determines the maximum element based on the count returned by the key function. In other words, it finds the element with the highest frequency in the original list.
Result and Conclusion
After executing the code, the variable most
will hold the value of the element that appears most frequently in the list. In our example, the value of most
will be 1
since it appears three times in the list.
This code demonstrates a simple and elegant way to find the most common element in a list using Python's built-in functions. It's a great example of how Python's concise syntax and powerful functions can be combined to solve real-world problems efficiently.
Next time you need to determine the most frequent element in a list, you can use this code as a reference to streamline your process and save time.
Subscribe to my newsletter
Read articles from fakhir hassan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

fakhir hassan
fakhir hassan
Student at Comsats Islamabad Will be completing my degree in 2026 here you will find all my daily learnings