Building Reusable Components in React: A Beginner's Guide to Organizing Your Project for Efficiency
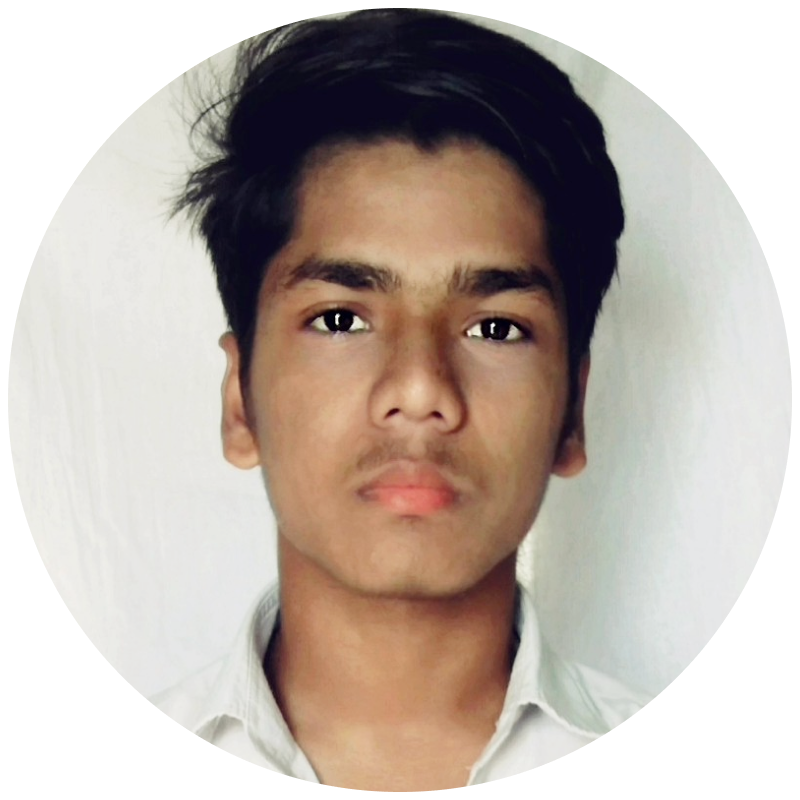
In this guide, I'm going to explain how you can master the art of using common modules for building reusable components in React.
Learning this skill offers a multitude of benefits. You'll be able to create apps faster, reduce bugs, and maintain your codebase with greater ease. By the end of this guide, you'll unlock the ability to create efficient and organized React projects that are set up for success.
Unfortunately, despite the advantages, many developers shy away from diving into components.
The primary reason for this hesitation is the misconception that reusable components are complex and time-consuming to implement.
Here are a few other reasons people hesitate to learn and implement reusable components:
Fear of Complexity
Lack of Understanding
Resistance to Change
Time Constraints
Despite these hurdles, there's hope! I'm here to show you exactly how can overcome these challenges and become proficient in creating and utilizing reusable components in React.
Why We Need a Common Module
Think of reusable code as puzzle pieces. We have components (like building blocks), custom hooks (useful tools), and logic (smart thinking) that we use in different parts of our project.
They need a home where they can stay together and show us how useful they are across the project. That's the goal of a common module – to keep all these puzzle pieces organized and let us know they're important everywhere.
Different Types of Reusable Pieces
Reusability in React comes in many shapes and sizes:
Everyday Components: These are simple things like buttons, text boxes, and boxes for holding content. They're like tools we use all the time.
Special Components: Sometimes, we need special tools for special jobs. Like charts for showing data in apps that analyze things.
Make-It-Your-Way Components: Imagine being able to change how the text looks in an article. These kinds of tools are like that – they're flexible and can fit into different situations.
Helpers and Shortcuts: These are little helpers that save us time. They can do small jobs or big jobs, depending on what we need.
Why a Common Module is Cool:
Making a common module is like cleaning up our room. When we put all our toys in one place, it's easier to find them. Here's why it's great:
One Big Happy Family: All our reusable stuff lives together. This keeps things neat and stops us from copying the same things all over the place.
Easy Sharing: Imagine showing your friends where your toys are – that's what a common module does for your code. It's like saying, "Look, here are all the cool things we can use!"
How to Get Started
When we start building a project, we need to think about reusing stuff. If we don't, things can get messy. So, we make a plan:
Think Ahead: We decide early on how we'll manage our reusable things. If we don't, our project could turn into a big jumble.
Create a Folder: Start by creating a new folder in your React project. You can call it something like "common" or "shared."
Organize Your Code: Inside this folder, you can start organizing your reusable code. Create sub-folders for different types of reusable elements, like "components," "hooks," and "utils."
Add Your Code: Start adding your reusable components, hooks, utility functions, and any other shared logic to their respective folders. Make sure to keep things organized and easy to find.
Export Them: In each file, export the things you want to be reusable. This means other parts of your project can easily use them.
Test and Refine: As you use your Common Module, you might find things that need improvement. That's okay! Just go back to your Common Module, make the changes, and see how it affects different parts of your project.
How to Take Care of the Common Module
While having a Common Module is a great idea, we need to be a little careful. Sometimes, if we put too many things in there, it can become really big and hard to manage.
So, the smart thing to do is to divide the Common Module into smaller parts. Each part can have specific things that are related. This way, it's not like having one big box of tools; it's more like having smaller boxes with specific tools that we need.
Conclusion
When we're working on React projects, creating a Common Module is a pretty big deal. It's like making a special home for all the pieces of code we use again and again.
This home keeps things organized, helps us avoid repeating ourselves, and makes it easier for everyone in the team to work together. But remember, we should be careful not to overload the Common Module with too much stuff.
By keeping things in smaller, well-organized parts, we can make the most of the Common Module's benefits without overwhelming it. So, when you're working on your next React project, don't forget to create that special toolbox – your Common Module!
Just follow the simple steps, and you'll be well on your way to a more efficient and organized coding journey.
Subscribe to my newsletter
Read articles from Mohammad Talim directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
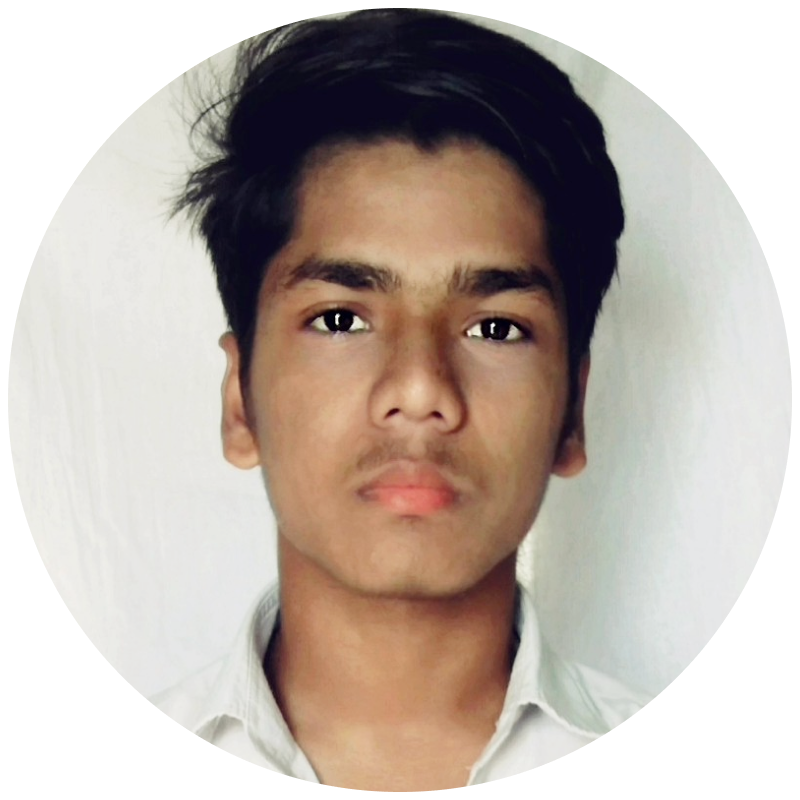
Mohammad Talim
Mohammad Talim
Final year student and front-end developer passionate about building beautiful and user-friendly experiences. Writing about web development and design.