Creating Text with Ellipsis in HTML and CSS
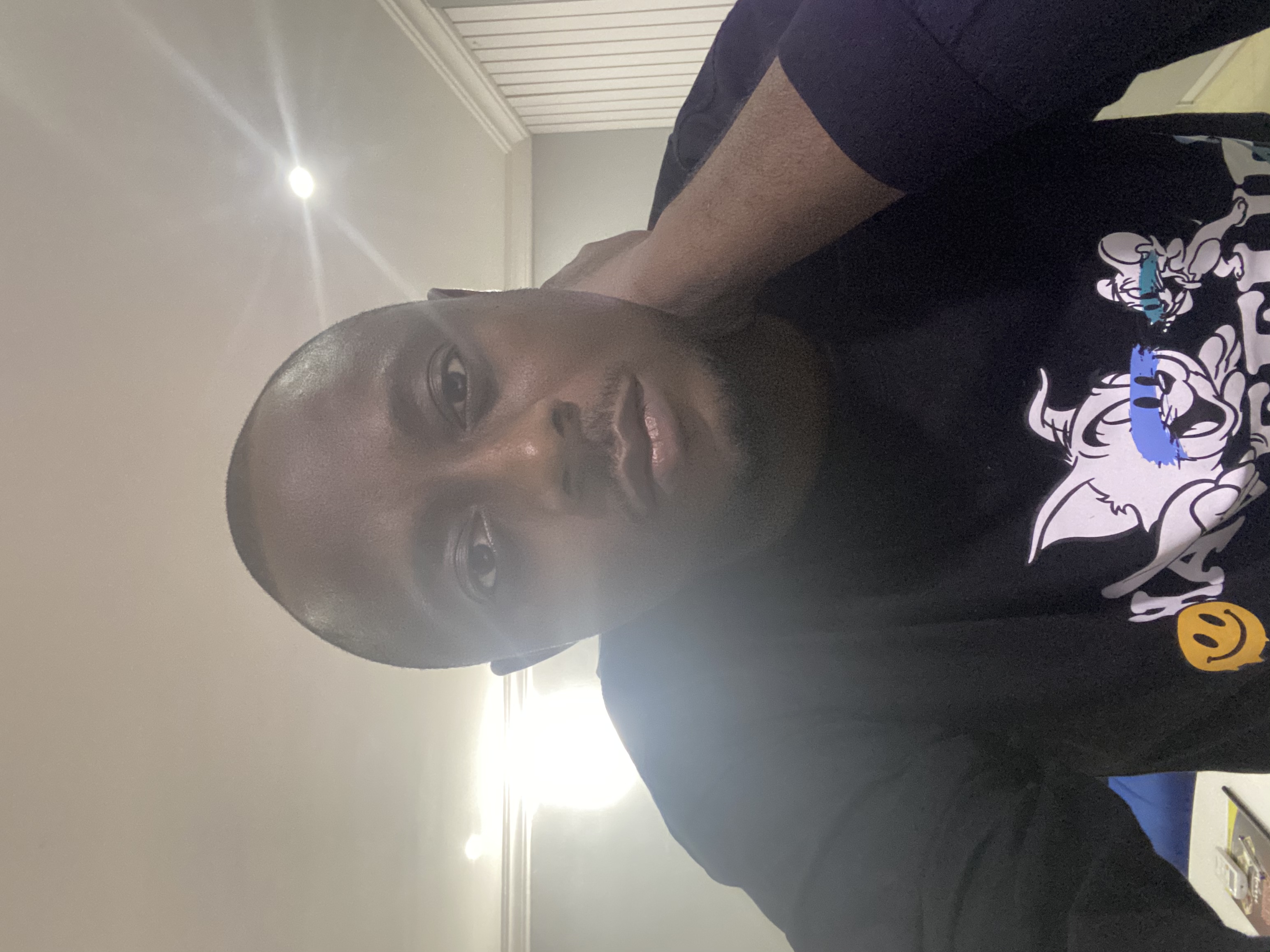
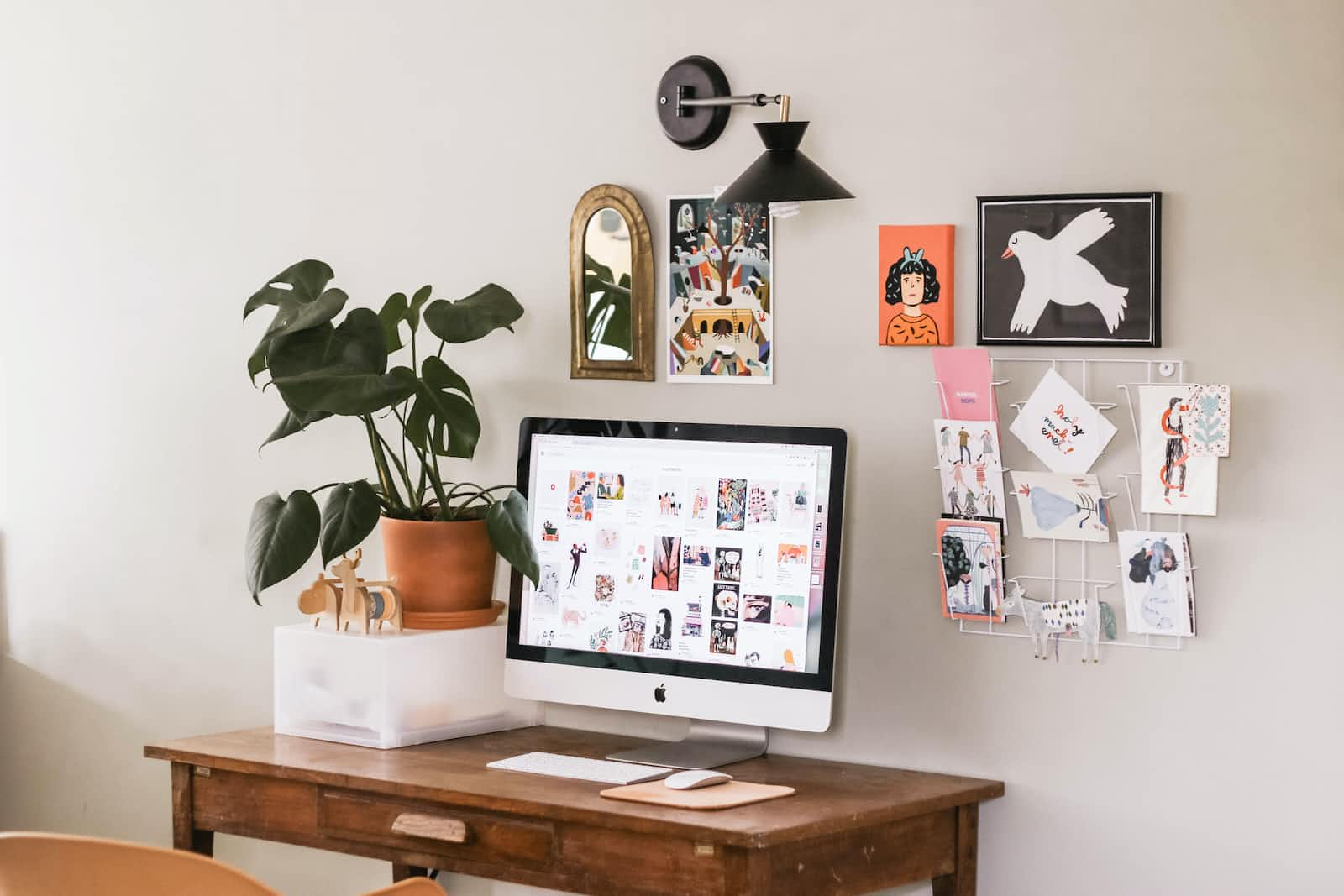
Text truncation with ellipsis is a common technique used in web design to handle situations where text content is too long to fit within a fixed width container. This technique allows users to see a snippet of the text with an ellipsis (...) at the end, indicating that there is more content that is not visible. In this blog post, we will walk through how to achieve this effect using HTML, CSS, and a touch of JavaScript.
HTML Structure
Let's start by creating the HTML structure for the content that we want to display with ellipsis. For this example, we'll assume you have a container with multiple rows, each containing three columns: "Game," "User," and "Payout." The text in these columns may vary in length, and we want to ensure that it is properly truncated with an ellipsis when it exceeds the width of the container.
<div class="mbody6">
<div class="mbody6head">
<div class="sport1 active">All Bets</div>
<div class="sport2">High Rollers</div>
<div class="sport3">Lucky Wins</div>
<div class="sport4">My Bets</div>
</div>
<div class="mbodybody">
<div class="bodyhead">
<div>Game</div>
<div>User</div>
<div>Payout</div>
</div>
<div id="sport1-content">
<div class="bodybody-container">
<div class="bodybody">
<div class="ellipsis">
<span> rhapsodory flame</span>
</div>
<div class="ellipsis">
<span>Hidden</span>
</div>
<div class="ellipsis cgreen">
<i class="fa fa-dollar"></i>
<span> 179.20</span>
</div>
</div>
<div class="bodybody">
<div class="ellipsis">
<span> Fishing Position</span>
</div>
<div class="ellipsis">
<i class="fa fa-hourglass-start"></i>
<span> Masnond</span>
</div>
<div class="ellipsis cgreen">
<i class="fa fa-dollar"></i>
<span> 45.20</span>
</div>
</div>
<div class="bodybody">
<div class="ellipsis">
<span> rhapsodory flame</span>
</div>
<div class="ellipsis">
<i class="fa fa-hourglass-start"></i>
<span> Masnondshjdhdjssd</span>
</div>
<div class="ellipsis cgreen">
<i class="fa fa-dollar"></i>
<span> 179.20</span>
</div>
</div>
<div class="bodybody">
<div class="ellipsis">
<span> rhapsodory flame</span>
</div>
<div class="ellipsis">
<i class="fa fa-hourglass-start"></i>
<span> Masnondshjdhdjssd</span>
</div>
<div class="ellipsis cgreen">
<i class="fa fa-dollar"></i>
<span> 179.20</span>
</div>
</div>
</div>
</div>
<div id="sport2-content">
<div class="bodybody-container">
<div class="bodybody">
<div class="ellipsis">
<span> rhapsodory flame</span>
</div>
<div class="ellipsis">
<i class="fa fa-hourglass-start"></i>
<span> Masnondshjdhdjssd</span>
</div>
<div class="ellipsis cgreen">
<i class="fa fa-dollar"></i>
<span> 179.20</span>
</div>
</div>
<div class="bodybody">
<div class="ellipsis">
<span> rhapsodory flame</span>
</div>
<div class="ellipsis">
<i class="fa fa-hourglass-start"></i>
<span> Masnondshjdhdjssd</span>
</div>
<div class="ellipsis cgreen">
<i class="fa fa-dollar"></i>
<span> 179.20</span>
</div>
</div>
<div class="bodybody">
<div class="ellipsis">
<span> rhapsodory flame</span>
</div>
<div class="ellipsis">
<i class="fa fa-hourglass-start"></i>
<span> Masnondshjdhdjssd</span>
</div>
<div class="ellipsis cgreen">
<i class="fa fa-dollar"></i>
<span> 179.20</span>
</div>
</div>
<div class="bodybody">
<div class="ellipsis">
<span> rhapsodory flame</span>
</div>
<div class="ellipsis">
<i class="fa fa-hourglass-start"></i>
<span> Masnondshjdhdjssd</span>
</div>
<div class="ellipsis cgreen">
<i class="fa fa-dollar"></i>
<span> 179.20</span>
</div>
</div>
</div>
</div>
<div id="sport3-content">
<div class="bodybody-container">
<div class="bodybody">
<div class="ellipsis">
<span> rhapsodory flame</span>
</div>
<div class="ellipsis">
<i class="fa fa-hourglass-start"></i>
<span> Masnondshjdhdjssd</span>
</div>
<div class="ellipsis cgreen">
<i class="fa fa-dollar"></i>
<span> 179.20</span>
</div>
</div>
<div class="bodybody">
<div class="ellipsis">
<span> rhapsodory flame</span>
</div>
<div class="ellipsis">
<i class="fa fa-hourglass-start"></i>
<span> Masnondshjdhdjssd</span>
</div>
<div class="ellipsis cgreen">
<i class="fa fa-dollar"></i>
<span> 179.20</span>
</div>
</div>
<div class="bodybody">
<div class="ellipsis">
<span> rhapsodory flame</span>
</div>
<div class="ellipsis">
<i class="fa fa-hourglass-start"></i>
<span> Masnondshjdhdjssd</span>
</div>
<div class="ellipsis cgreen">
<i class="fa fa-dollar"></i>
<span> 179.20</span>
</div>
</div>
</div>
</div>
<div id="sport4-content">
<div class="bodybody-container">
<div class="bodybody">
<div class="ellipsis">
<span> rhapsodory flame</span>
</div>
<div class="ellipsis">
<i class="fa fa-hourglass-start"></i>
<span> Masnondshjdhdjssd</span>
</div>
<div class="ellipsis cgreen">
<i class="fa fa-dollar"></i>
<span> 179.20</span>
</div>
</div>
<div class="bodybody">
<div class="ellipsis">
<span> rhapsodory flame</span>
</div>
<div class="ellipsis">
<i class="fa fa-hourglass-start"></i>
<span> Masnondshjdhdjssd</span>
</div>
<div class="ellipsis cgreen">
<i class="fa fa-dollar"></i>
<span> 179.20</span>
</div>
</div>
</div>
</div>
</div>
</div>
Styling the Container
Before we proceed to style the individual rows of content, let's style the container and header section to provide a cohesive look for our content display.
.mbody6 {
height: 500px;
border: 1px solid red;
border-radius: 3px;
display: flex;
flex-direction: column;
margin-top: 30px;
margin-bottom: 30px;
}
.mbody6head {
height: 50px;
border-bottom: 1px solid rgb(90, 123, 90);
display: flex;
justify-content: space-between;
align-items: center;
padding: 0 10px;
font-size: 15px;
background-color: rgba(6, 6, 10, 0.961);
color: white;
}
.mbodybody {
display: flex;
flex-direction: column;
gap: 2px;
padding: 5px 5px;
}
.bodyhead {
height: 35px;
background-color: rgba(53, 53, 65, 0.961);
display: flex;
justify-content: space-between;
align-items: center;
padding: 0 13px;
font-size: 14px;
color: white;
}
.bodybody-container {
max-height: 400px;
/* Set a maximum height for scrolling */
overflow-y: auto;
/* Enable vertical scrolling if content overflows */
}
.bodybody {
height: 35px;
background-color: rgba(27, 27, 40, 0.961);
display: flex;
justify-content: space-between;
align-items: center;
padding: 0 13px;
font-size: 14px;
margin-bottom: 5px;
color: white;
}
.bodybody div {
display: flex;
align-items: center;
border: 0px solid red;
width: 33%;
padding: 5px 15px;
white-space: normal; /* Allow text to wrap */
white-space:nowrap;
overflow: hidden;
}
.bodybody div i{
margin-right: 5px;
}
Adding Ellipsis with JavaScript
At this point, our CSS styling ensures that the text content is truncated when it exceeds the width of the column. However, we need JavaScript to dynamically add the ellipsis (...) to the text when it's truncated.
<script>
const ellipsisDivs = document.querySelectorAll('.ellipsis');
ellipsisDivs.forEach((div) => {
const textElement = div.querySelector('span');
if (div.scrollWidth > div.clientWidth) {
textElement.textContent = textElement.textContent.trim();
while (div.scrollWidth > div.clientWidth && textElement.textContent.length > 0) {
textElement.textContent = textElement.textContent.slice(0, -1);
}
textElement.textContent += '...';
}
});
</script>
This JavaScript code checks each .ellipsis
element to see if its content exceeds its container's width. If it does, the content is trimmed and ellipsis is added.
Wrapping Up
In this blog post, we explored how to create text with ellipsis using HTML, CSS, and JavaScript. By combining proper styling with dynamic truncation, we can create a visually appealing and user-friendly way to display potentially long text content within fixed-width containers.
By following the steps outlined in this blog post and using the provided code snippets, you can easily implement text truncation with ellipsis in your own web projects. This technique is particularly useful when you have limited space and need to prioritize content visibility while maintaining a clean and organized layout.
Subscribe to my newsletter
Read articles from Ogunuyo Ogheneruemu B directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
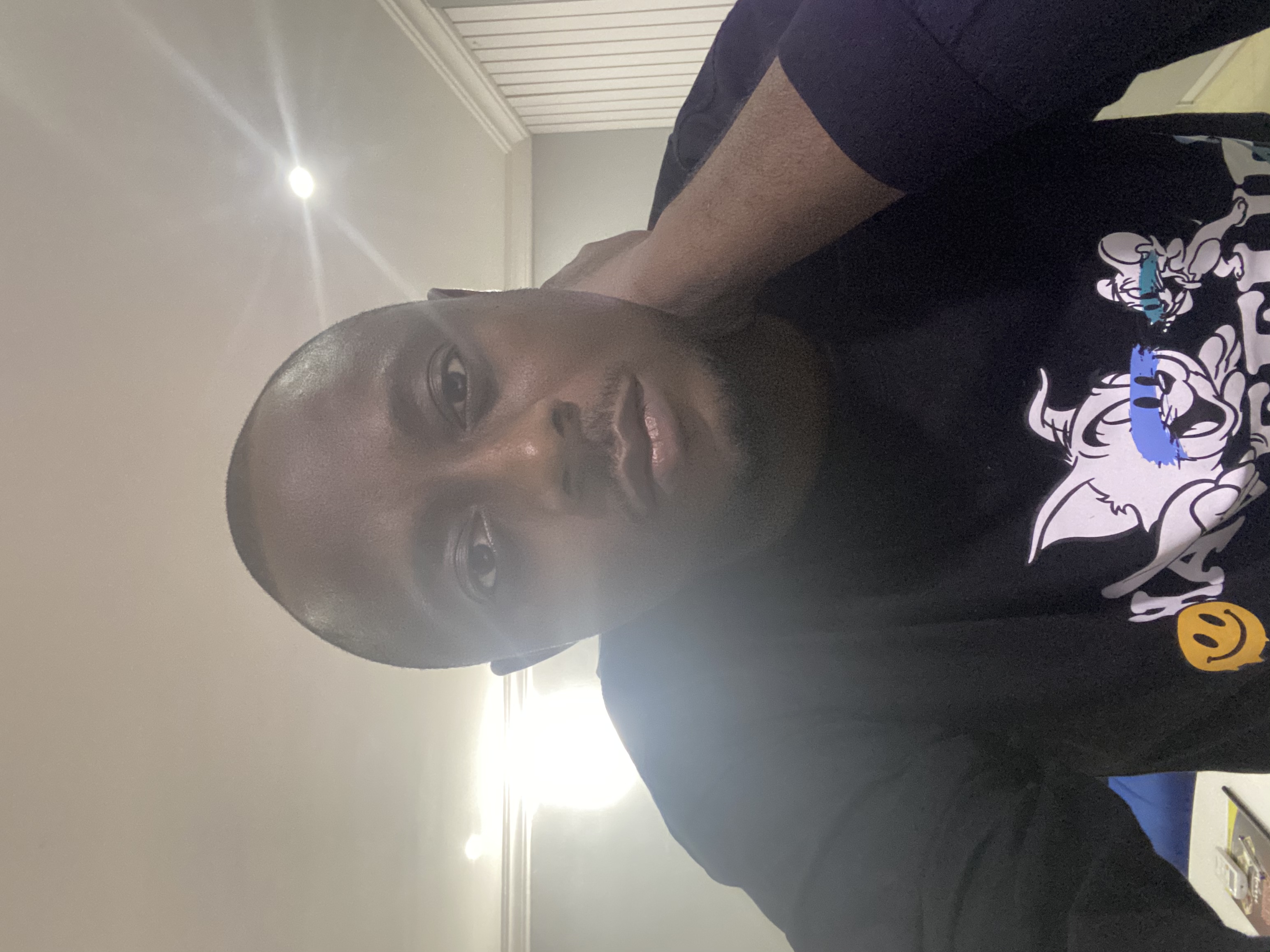
Ogunuyo Ogheneruemu B
Ogunuyo Ogheneruemu B
I'm Ogunuyo Ogheneruemu Brown, a senior software developer. I specialize in DApp apps, fintech solutions, nursing web apps, fitness platforms, and e-commerce systems. Throughout my career, I've delivered successful projects, showcasing strong technical skills and problem-solving abilities. I create secure and user-friendly fintech innovations. Outside work, I enjoy coding, swimming, and playing football. I'm an avid reader and fitness enthusiast. Music inspires me. I'm committed to continuous growth and creating impactful software solutions. Let's connect and collaborate to make a lasting impact in software development.