Level Up Your React Components: A Comprehensive Journey Through Fragments

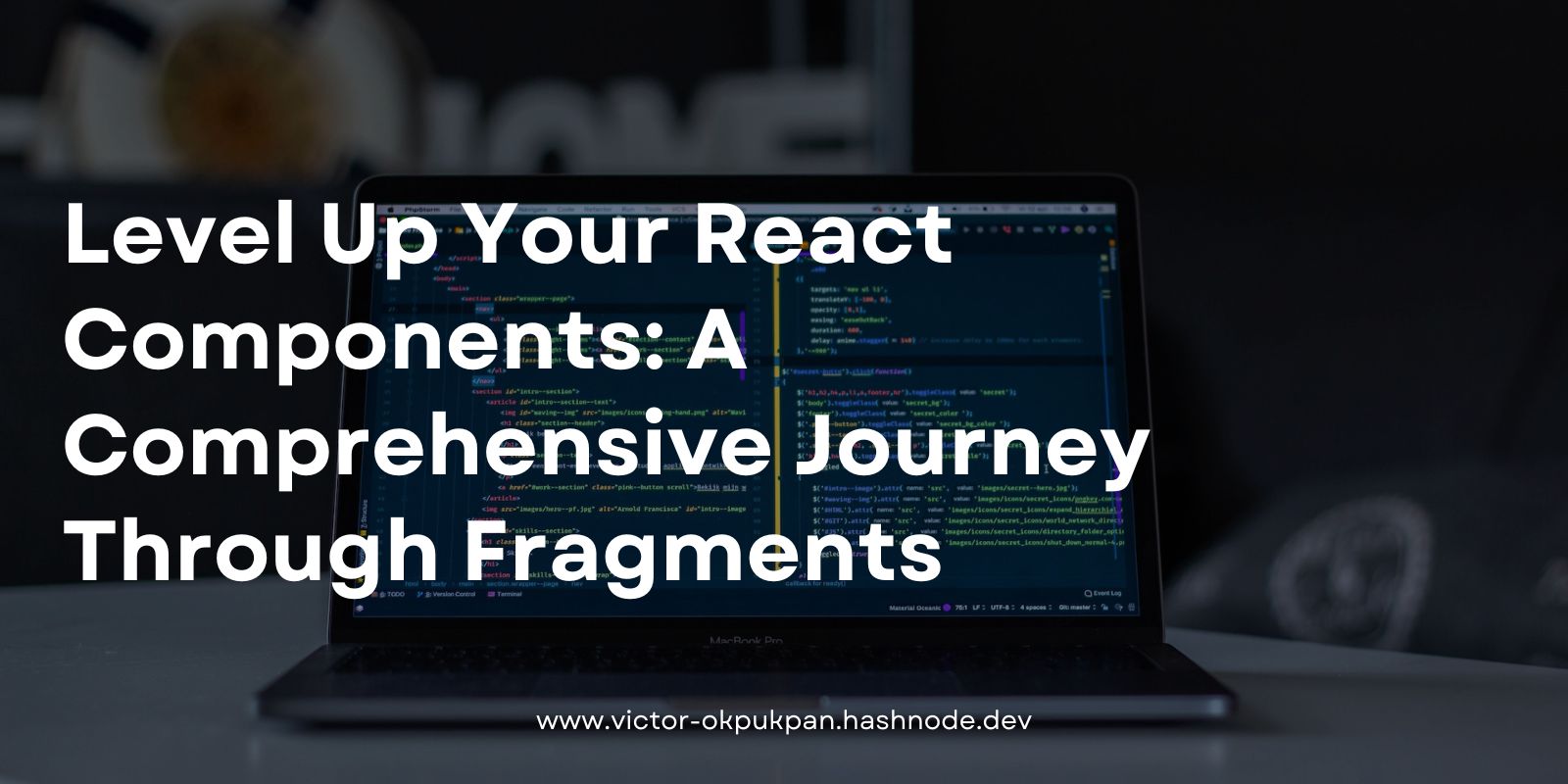
ReactJS has simplified and improved the way we develop user interfaces. As applications become more sophisticated, efficiently managing the Document Object Model (DOM) becomes critical. This is where the concept of "fragments" comes into play, as it is one of the fantastic features of ReactJS.
Understanding Fragments in React
In React, a fragment is a way to group multiple elements without introducing an additional DOM element. It allows you to group components without having to add an extra wrapper div. This is especially useful when you want to avoid unnecessary DOM elements that can affect the structure of your HTML and potentially hinder CSS styling.
Creating Fragments in React
Creating fragments in React is straightforward. You can use the <Fragment> syntax to wrap your elements. However, you can also use the short syntax with <> and </> for a more concise approach. This allows you to enclose multiple components within a fragment without causing any distortion to the HTML structure.
For example:
import React, { Fragment } from "react";
export default const FragmentContainer = () => {
return(
<Fragment>
<div>
<h1>This is a div within a fragment</h1>
</div>
<div>
<h1>This is another div within the same fragment</h1>
</div>
</Fragment>
)
}
In the example above, two divs have been wrapped within a React Fragment using the <Fragment> syntax. Likewise, the above code could also be written as:
import React, { Fragment } from "react";
export default const FragmentContainer = () => {
return(
<>
<div>
<h1>This is a div within a fragment</h1>
</div>
<div>
<h1>This is another div within the same fragment</h1>
</div>
</>
)
}
The Benefits of React Fragments
One of the primary advantages of using React Fragments is that they improve performance and consume less memory. You can reduce the number of actual DOM elements rendered by using Fragments, which results in faster rendering. Furthermore, because you avoid using unnecessary divs, your JSX code remains cleaner and more readable.
In large-scale applications, using fragments becomes critical to effectively managing the DOM and maintaining a smooth user experience. They aid in overcoming common issues that arise when dealing with complex component hierarchies.
Conclusion
ReactJS fragments provide an elegant solution for managing complex component hierarchies without the addition of unnecessary DOM elements. By using fragments, you can keep your JSX codebase clean and efficient, improve rendering performance, and improve the overall user experience. As your applications become more complex, embracing fragments becomes a critical strategy for efficient DOM management.
Subscribe to my newsletter
Read articles from Victor Okpukpan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Victor Okpukpan
Victor Okpukpan
With over a year of hands-on experience as a front-end developer, I've decided to begin sharing my knowledge here on Hashnode to ensure that I continue to grow alongside others. I am also a final-year undergraduate computer science student. As an aspiring beacon in the field of front-end development, I'm motivated by my passion for the craft, which began when I built my first website. Since then, my journey has been a mix of learning, creating, and innovating within the web development landscape. I am a firm believer in the power of web technologies to shape the digital experiences of the future. From creating elegant user interfaces to delving into the complexities of responsive design, I enjoy every aspect of the front-end journey. My blog will serve as a canvas for me to share a wide range of insights, tutorials, and creative musings about the ever-changing world of web development. My goal is to provide fellow developers with both inspiration and practical knowledge, from breaking down complex concepts to capturing the latest trends. But this journey is about us, not just me. I'm here to connect with others who share my enthusiasm for front-end development. Let's learn from one another, grow together, and create a thriving community that pushes the limits of what's possible on the web. I invite you to join me on this exciting journey, whether you're a seasoned developer or just getting started. Let's dive into the world of front-end development and make our shared dreams a reality. Feel free to contact me on X (formerly known as Twitter) or via email at vokpukpan@gmail.com. Let us shape the web's future together, one line of code at a time. I'm looking forward to connecting and learning with everyone!