Spread and Rest Operator in JS

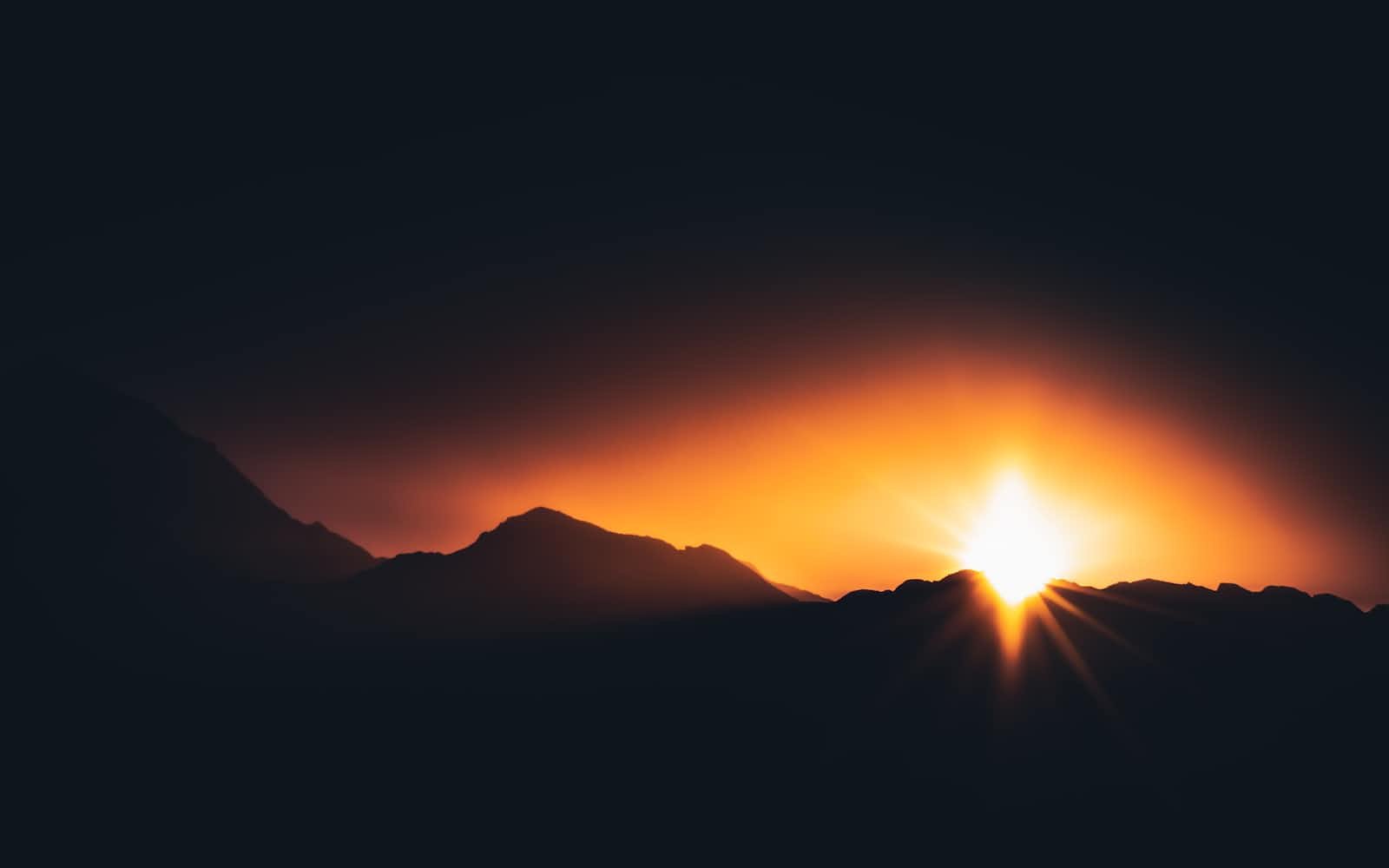
Spread Operator (...obj)
Just like the sun rays spreading in the above image, the spread operator is used to spread the values of iterables. Denoted by three consecutive dots (...), this operator is used on iterables to spread its values and either store it in a new array/object or pass it as a copy to another iterable variable. It is a very useful operator which has proven to be versatile in many situations. With the launch of the most significant version of ECMA (i.e. ES6) in June 2015 this operator also came into existence, and it helps in manipulating and creating arrays and objects.
As Mr. Hitesh Choudhary has said in his JS playlist on YT channel, think of the spread operator as dropping a glass on the floor, What will happen? It's simply going to get spread on the ground. Similarly, the operator is going to work here in the code.
In Array
- Merging two Arrays:-
let arr1 = [10,11,12,13,14]
let arr2 = [2,3,4]
let newArray = [...arr1, ...arr2, 50, 60]
//in newArray each element of arr1 and arr2 is spreaded and added
console.log(newArray)
Output:-
[
10, 11, 12, 13, 14,
2, 3, 4, 50, 60
]
Copying Array:-
The spread operator helps in making a shallow copy of the original array. Deep cloning concepts require additional techniques that will be covered in upcoming articles.
let array1 = ["Ronaldo", "Modric", "Benzema"]; let cpy = [...array1]; console.log(array1)// prints the original array cpy.push("Xavi"); //This will push the element at the last index console.log(array1)// the original array is not going to be affected console.log(cpy)//prints the modified array "Xavi" at last index
Output:-
[ 'Ronaldo', 'Modric', 'Benzema' ] [ 'Ronaldo', 'Modric', 'Benzema' ] [ 'Ronaldo', 'Modric', 'Benzema', 'Xavi' ]
In the above example if we would have assigned the array1 to cpy like this:-
let cpy = array1;
In this case, if we alter the copied array then it is going to change the original one too, which was not intended. Hence spread acts as a helping hand in this situation.
In Objects
Copying Objects:-
In the case of objects also this operator will create a shallow copy of the original one.let object1 = { prop1: "something", prop2: 2 }; console.log(object1); let cpy = {...object1}; console.log(cpy);
Output:-
{ prop1: 'something', prop2: 2 } { prop1: 'something', prop2: 2 }
Object Spread:-
Spread Operator not only helps in creating a shallow copy but also allows you to add some more properties at the time of copying. Let's take the same previous example.let object1 = { prop1: "something", prop2: 2 }; console.log(object1); let cpy = {...object1, prop3: "something more to be added"}; console.log(object1); //printing here to show that it does not affect the orginal object console.log(cpy);
Output:-
{ prop1: 'something', prop2: 2 } { prop1: 'something', prop2: 2 } { prop1: 'something', prop2: 2, prop3: 'something more to be added' }
Chai aur Code example reference link is given below:-
Chai aur Code spread operator in JS playlist
Rest Operator (...arg)
Denoted with three consecutive dots (...) the rest "parameter" / "operator" is used in the function parameter list to collect multiple or indefinite numbers of arguments into an array. This is an improvised version of handling function parameters in JS. This parameter was included with the ES6 launch.
Taking Multiple Function Params:-
function restParam(...args) { //inside args we can place indefinites number of parameters console.log(args); //printing the entire array of parameter console.log(args[0]); // extracting the 0th index param console.log(args.length); //this shows that we can use all the properties of Array on this args param } restParam(1, 2, 3, 4); restParam(1,2); // Pass as many arguments you require
Output:-
[ 1, 2, 3, 4 ] 1 4 [ 1, 2 ] 1 2
Taking Remaining Function Params:-
function UnderstandingRestParam(arg1, arg2, ...restArgs) { console.log("First argument:", arg1); console.log("Second argument:", arg2); console.log("Rest of the arguments:", restArgs); } UnderstandingRestParam(1, 2, 3, 4, 5, "Hello", {a: 1});
Output:-
First argument: 1 Second argument: 2 Rest of the arguments: [ 3, 4, 5, 'Hello', { a: 1 } ]
In this article, by now we have understood almost all types of cases that come under the spread and rest operator. But hold onto your curiosity, there is a lot more to discover in JS and I'll be bringing new ways of delivering the concepts of JS. Stay connected.
Subscribe to my newsletter
Read articles from Syed Kumail Rizvi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Syed Kumail Rizvi
Syed Kumail Rizvi
Learner | Student | Trainee | Interested In Tech related stuff. | Writes about JS, Java, Node, Spring, ORM. |