Creating a Basic Telegram Bot using Python and Telebot
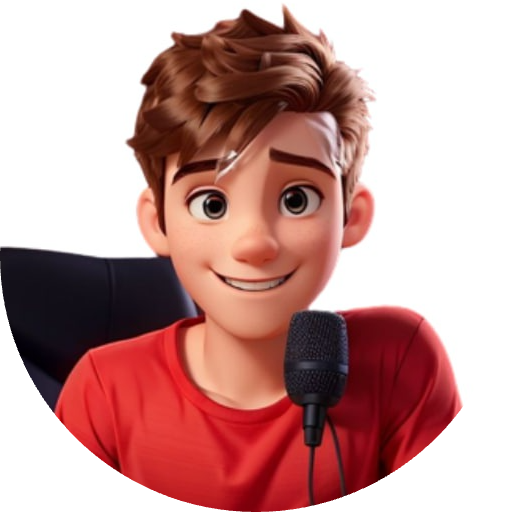

In this tutorial, we'll walk you through the process of creating a basic Telegram bot using Python and the Telebot library. The bot will respond to user messages and provide a welcome message upon the /start
command. Let's get started!
Prerequisites
Telegram Account: You'll need a Telegram account to create a bot and obtain its token.
Python Installed: Ensure that Python is installed on your system.
Step 1: Getting Your Bot Token
Open the Telegram app and search for BotFather.
Start a chat with BotFather by sending the
/start
command.Use the
/newbot
command to create a new bot. Set a name and username for your bot.Copy the token provided by BotFather.
Step 2: Writing the Python Code
import telebot
bot = telebot.TeleBot("your_bot_token_here") # Replace with your bot token
# /start command for your bot
@bot.message_handler(commands=['start'])
def handle_start(message):
start_message = "<b>Hello, welcome to the bot!</b>"
bot.reply_to(message, start_message, parse_mode='HTML')
# A text message handler
@bot.message_handler(func=lambda message: True)
def handle_text(message):
reply_text = message.text
bot.reply_to(message, reply_text)
# Start the bot
bot.polling()
Running the Bot
Save the Python code in a
.py
file.Open your terminal or command prompt and navigate to the directory containing the
.py
file.Replace
your_bot_token_here
with the actual bot token.Run the bot script by executing the command:
python filename.py.
Interacting with Your Bot
Open your Telegram app and search for your bot's username.
Start a chat with your bot.
Send the
/start
command to receive a welcome message.Send any text message to the bot, and it will respond with the same message.
Conclusion
Congratulations! You've successfully created a basic Telegram bot using Python and the Telebot library. This bot responds to the /start
command with a welcome message and echoes back any text messages you send. This is just a starting point, and you can build more advanced interactions and features using the Telebot library.
Subscribe to my newsletter
Read articles from Karan Coder directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
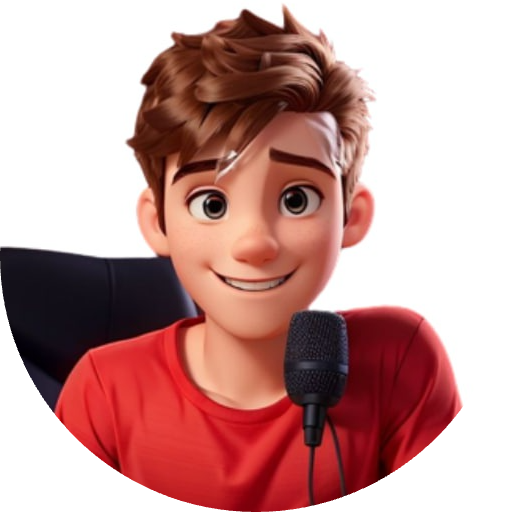