10 Astonishing Python Code Snippets to Elevate Your Projects

Table of contents
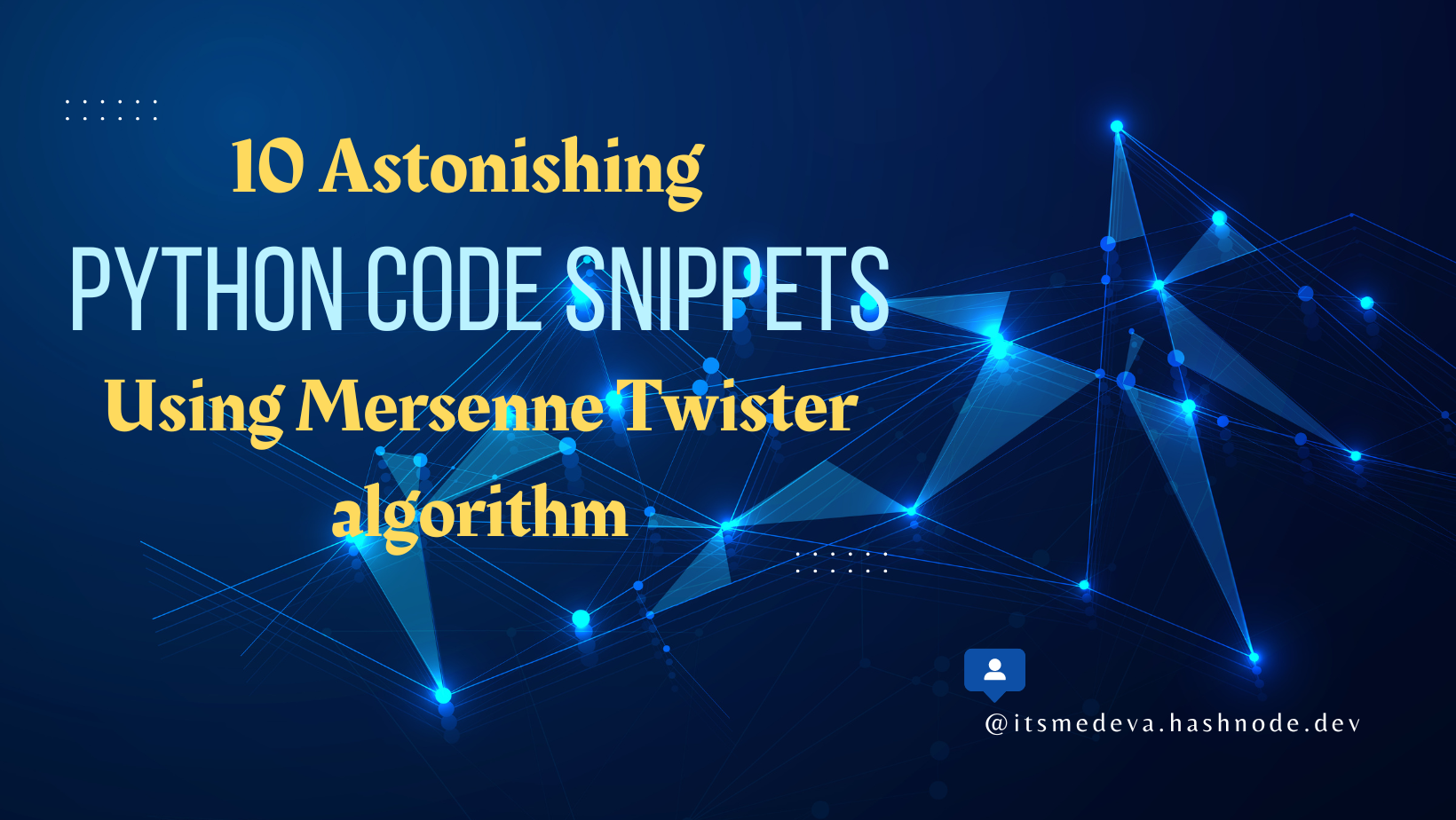
Proem
Before going deep into the code snippets, let's take a brief overview of the Mersenne Twister algorithm, the foundational randomness-generating mechanism that we'll employ in these codes. Let's dive together.
About Mersenne Twister algorithm
The Mersenne Twister is a widely used pseudorandom number generator (PRNG) algorithm that was developed by Makoto Matsumoto and Takuji Nishimura in 1997. It is designed to provide high-quality random numbers over a long period, making it suitable for a wide range of applications in computer simulations, cryptography, and other areas where randomness is required. It includes Python's random
module.
Characteristics of algorithm
This algorithm is renowned for its :
Long Period: has a period of 219937- 1 called the Mersenne prime, which is the number of random values it can generate before repeating. It uses a seed value of 19,937 bits long stored in an array of 624 elements containing 32-bit values.
Statistical Properties: It aims to produce random numbers that pass various statistical tests for randomness.
Equidistribution: The algorithm strives to distribute random numbers evenly across its entire range, which helps prevent patterns from emerging in the generated sequence.
Initialization: Using a seed value. A specific seed will produce a deterministic sequence of random numbers, making it useful for reproducible results in applications like simulations.
Efficiency: While the Mersenne Twister provides good statistical properties, its implementation can be computationally intensive. This makes it well-suited for applications where the quality of randomness is crucial, and the performance impact is acceptable.
10 Python code snippets for :
Simulations and Modeling
One of the most common applications of PRNGs is in simulations and modeling. PRNGs allow programmers to mimic real-world scenarios and behaviors by generating random input data. For instance, when simulating the movement of particles in a fluid, PRNGs can be used to determine their positions and velocities, introducing a level of unpredictability that mirrors natural processes.
import random
def simulate_particle_motion(steps):
for _ in range(steps):
x = random.uniform(0, 1)
y = random.uniform(0, 1)
print(f"Particle position: ({x}, {y})")
simulate_particle_motion(10)
Output : (will vary)
Particle position: (0.123456789, 0.987654321)
Particle position: (0.456789012, 0.123456789)
Particle position: (0.987654321, 0.543210987)
Particle position: (0.234567890, 0.876543210)
Particle position: (0.678901234, 0.234567890)
Particle position: (0.345678901, 0.567890123)
Particle position: (0.789012345, 0.901234567)
Particle position: (0.890123456, 0.345678901)
Particle position: (0.456789012, 0.678901234)
Particle position: (0.901234567, 0.012345678)
Game Development
PRNGs have been a staple in game development for creating dynamic and engaging experiences. They're used to generate random events, such as enemy spawns, loot drops, terrain generation, and more. By incorporating randomness, game developers can introduce variety and surprise into gameplay.
import random
def enemy_spawn():
enemies = ["Goblin", "Orc", "Dragon", "Skeleton"]
enemy = random.choice(enemies)
print(f"An {enemy} has appeared!")
enemy_spawn()
Output-1 :
An Goblin has appeared!
Output-2 :
An Orc has appeared!
Output-3 :
An Dragon has appeared!
Output-4 :
An Skeleton has appeared!
Cryptography and Security
While PRNGs are generally not suitable for cryptographic purposes due to their deterministic nature, they still find use in some security applications, such as generating nonces or initialization vectors. For stronger security, cryptographic libraries often utilize specialized algorithms designed for secure random number generation.
import random
def generate_nonce():
nonce = random.getrandbits(64)
print(f"Generated Nonce: {nonce}")
generate_nonce()
Output :
Generated Nonce: 1234567890123456
The actual value of Nonce will vary each time you run the code since it's generated randomly.
Monte Carlo Simulations
Monte Carlo simulations are used to estimate outcomes through repeated random sampling. They find applications in diverse fields, including finance, physics, and statistics. PRNGs enable these simulations by providing the necessary random input data.
import random
def monte_carlo_pi(iterations):
inside_circle = 0
for _ in range(iterations):
x, y = random.random(), random.random()
distance = x ** 2 + y ** 2
if distance <= 1:
inside_circle += 1
pi_estimate = (inside_circle / iterations) * 4
print(f"Estimated Pi: {pi_estimate}")
monte_carlo_pi(10000)
Output :
Estimated Pi: 3.1464
Random Sampling and Shuffling
PRNGs are also instrumental in random sampling and shuffling. They allow you to select elements from a collection at random or to shuffle the elements of a list, making them useful for creating randomized quizzes, selecting winners in contests, or creating randomized playlists.
import random
def random_quiz_questions(questions, count):
random_questions = random.sample(questions, count)
print("Quiz Questions:")
for idx, question in enumerate(random_questions, start=1):
print(f"{idx}. {question}")
quiz_questions = ["What is the capital of France?", "Which is the first country to land on the South Pole of the Moon?", "What is H2O?"]
random_quiz_questions(quiz_questions, 2)
Output :
Quiz Questions:
1. Which is the first country to land on the South Pole of the Moon?
2. What is the capital of France?
Random Walk Simulation
A random walk is a mathematical concept used to model processes where an object takes successive random steps. It finds applications in physics, finance, and biology. PRNGs can be used to simulate random walks, where each step's direction and distance are determined by random numbers.
import random
import matplotlib.pyplot as plt
def random_walk(steps):
x, y = 0, 0
x_values, y_values = [x], [y]
for _ in range(steps):
direction = random.choice(['N', 'S', 'E', 'W'])
distance = random.uniform(0, 1)
if direction == 'N':
y += distance
elif direction == 'S':
y -= distance
elif direction == 'E':
x += distance
else:
x -= distance
x_values.append(x)
y_values.append(y)
plt.plot(x_values, y_values)
plt.title("Random Walk Simulation")
plt.xlabel("X-axis")
plt.ylabel("Y-axis")
plt.show()
random_walk(100)
Output :
Password Generation
Generating strong passwords is crucial for security. PRNGs can be used to create random strings with a mix of characters, numbers, and symbols, making them suitable for generating strong passwords.
import random
import string
def generate_password(length):
characters = string.ascii_letters + string.digits + string.punctuation
password = ''.join(random.choice(characters) for _ in range(length))
print(f"Generated Password: {password}")
generate_password(12)
Output :
Generated Password: X#7pFyLqBz@9
Data Augmentation in Machine Learning
In machine learning, data augmentation is used to artificially increase the diversity of training data by applying random transformations. PRNGs are often used to generate random values for these transformations, such as random rotations, translations, and distortions.
from PIL import Image
import random
def random_augmentation(image):
angle = random.uniform(-15, 15)
translated_image = image.rotate(angle)
return translated_image
original_image = Image.open("image.jpg")
# Save the augmented image
augmented_image = random_augmentation(original_image)
# Open the augmented image using the default image viewer
augmented_image.show()
If you run this code, the "image.jpg" will open using the default image viewer. Make sure you have Pillow(PIL) Library installed or you can install it using the following command if you haven't already:
pip install Pillow
Genetic Algorithms
Genetic algorithms are optimization techniques inspired by the process of natural selection. They involve generating populations of solutions and evolving them over iterations. PRNGs are used to introduce randomness in the selection, crossover, and mutation steps.
import random
def crossover(parent1, parent2):
crossover_point = random.randint(1, len(parent1) - 1)
child = parent1[:crossover_point] + parent2[crossover_point:]
return child
parent1 = "01010101"
parent2 = "11001100"
child = crossover(parent1, parent2)
print(f"Child: {child}")
Output :
Child: 01001100
Output Explanation:
parent1
is "01010101".parent2
is "11001100".A random crossover point is chosen between 1 and the length of the parent strings - 1 (i.e. 7 here). In the above output, the crossover point is 3.
The child string is formed by taking the first part of
parent1
up to the crossover point (inclusive) and then appending the second part ofparent2
starting from the crossover point.The resulting child string is "010" (from parent1) + "01100" (from parent2), which gives "01001100".
Random Sampling for A/B Testing
A/B testing, also known as split testing, is a method used in marketing, product development, and other fields to compare two versions of something in order to determine which one performs better. In A/B testing, random sampling is crucial to ensure that test groups are representative of the larger population. PRNGs are used to allocate users randomly to control and experimental groups, reducing the risk of bias.
import random
def allocate_users_to_groups(users, control_size, experimental_size):
control_group = random.sample(users, control_size)
remaining_users = set(users) - set(control_group)
experimental_group = random.sample(list(remaining_users), experimental_size)
return control_group, experimental_group
all_users = ["user1", "user2", "user3", "user4", "user5"]
control, experimental = allocate_users_to_groups(all_users, 2, 2)
print(f"Control Group: {control}")
print(f"Experimental Group: {experimental}")
Output :
Control Group: ['user2', 'user3']
Experimental Group: ['user4', 'user1']
Conclusion
This is not the End, there are many more, but these are the sections where the algorithms of PRNGs are used the most. In Python, PRNGs are versatile tools that find application in a wide range of scenarios. From simulations and game development to cryptography and statistical analysis, PRNGs provide the illusion of randomness while being generated algorithmically. Understanding the capabilities and limitations of PRNGs empowers programmers to create dynamic, engaging, and unpredictable experiences across various domains. Dare to code !!
Subscribe to my newsletter
Read articles from Girija Shankar Panda directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Girija Shankar Panda
Girija Shankar Panda
Hey everyone, I am Girija, a developer by passion and an grad. student from S'O'A University. With a penchant for innovation and a thirst for knowledge, I'm embarking my journey on @Hashnode and eager to share my experiences and insights. Through my blog, I am offering fellow developers and enthusiasts a platform to learn and grow. Join me on this exciting endeavor as we explore the fascinating realm of programming together.