Top Swift iOS Development Interview Questions - Beginner to Advanced Level
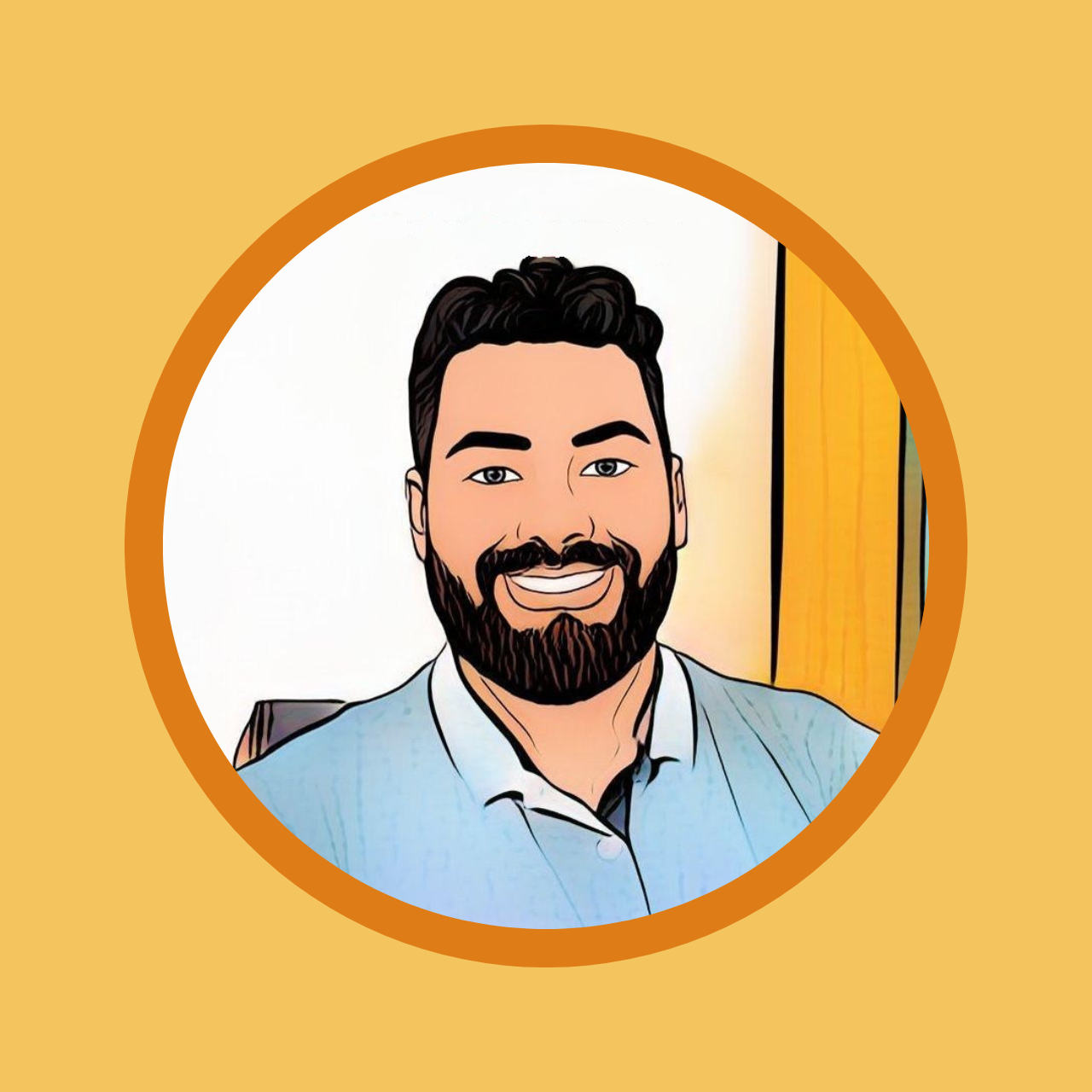
Table of contents
- 1. What are the features of Swift Language?
- 2. What is Optional Binding vs Optional Chaining?
- 3. What is the difference between Value type and reference type? Or Class vs Struct.
- 4. What are the practical use cases where struct can be used instead of class?
- 5. How is memory management done in Class and Struct?
- 6. What is Copy-on-write in Swift? How are Array and String using Copy-on-write in Swift?
- 7. What are the types of access modifiers available in Swift?
- 8. What are the ways Read-only properties are created in Swift?
- 9. Let vs. Var?
- 10. What is Private(set)?
- That's Not It !!

Are you gearing up for a Swift iOS development interview and want to ensure you're well-prepared to showcase your skills and knowledge? Look no further! In this comprehensive guide, we're diving into the most essential Swift iOS interview questions and providing you with clear and concise answers to help you ace your interview. Whether you're a seasoned developer or just starting your journey into iOS app development, these questions cover a wide range of topics, from language features to memory management, access modifiers, and much more. So, let's delve into the world of Swift iOS development and get you ready to impress potential employers with your expertise.
1. What are the features of Swift Language?
Type Safe language: Swift enforces type safety, preventing type-related errors at compile time. For instance:
var age: Int = 25 // Trying to assign a string to an integer will result in a compile-time error: age = "Thirty"
Type inference: Swift can automatically infer the data type based on the assigned value. For instance:
let name = "John" // Swift infers that `name` is of type String
Null safety using Optional: Swift uses Optionals to handle the absence of values. For example:
var email: String? = "john@example.com" if let unwrappedEmail = email { print("Email: \(unwrappedEmail)") } else { print("Email is nil") }
Powerful Enumeration: Swift's enums can have associated values and methods, enhancing their flexibility. For instance:
enum Status { case success(message: String) case error(code: Int, message: String) } let result = Status.success(message: "Operation completed successfully")
Protocol-oriented programming: Swift encourages the use of protocols for code reusability. Example:
protocol Vehicle { func start() func stop() } struct Car: Vehicle { func start() { print("Car started") } func stop() { print("Car stopped") } }
Use of value and reference types: Swift has value types (structs, enums) and reference types (classes). Example:
struct Point { var x: Int var y: Int } class Person { var name: String init(name: String) { self.name = name } }
Collection types: Swift offers arrays, sets, and dictionaries. Example:
var colors = ["Red", "Green", "Blue"] colors.append("Yellow") var ages: Set<Int> = [25, 30, 22] var studentGrades = ["John": 95, "Emily": 88]
Generics: Swift's generics enable writing flexible, reusable functions and types. Example:
func swap<T>(_ a: inout T, _ b: inout T) { let temp = a a = b b = temp } var x = 5, y = 10 swap(&x, &y)
Memory management: Swift uses Automatic Reference Counting (ARC) to manage memory. Example:
class Person { var name: String init(name: String) { self.name = name } } var person1: Person? = Person(name: "John") var person2 = person1 person1 = nil // Memory deallocated when no references remain
2. What is Optional Binding vs Optional Chaining?
Optional Binding: Unwraps an optional and binds its value to a new variable using
if let
orguard let
. Example:var website: String? = "www.example.com" if let unwrappedWebsite = website { print("Website: \(unwrappedWebsite)") } else { print("No website available") }
Optional Chaining: Safely accesses properties and methods of an optional that might be nil. Example:
struct Address { var street: String var city: String } class Person { var address: Address? } let person = Person() let cityName = person.address?.city // No crash even if address is nil
3. What is the difference between Value type and reference type? Or Class vs Struct.
Value types (structs, enums) are copied when assigned or passed, creating distinct instances.
Reference types (classes) are passed by reference, allowing multiple variables to refer to the same instance.
struct Point {
var x: Int
var y: Int
}
class Person {
var name: String
init(name: String) {
self.name = name
}
}
var p1 = Point(x: 10, y: 20)
var p2 = p1 // p2 is a copy of p1
p2.x = 30 // p1.x remains 10
var person1 = Person(name: "John")
var person2 = person1 // Both person1 and person2 refer to the same object
person2.name = "Alice" // Changes person1's name as well
4. What are the practical use cases where struct can be used instead of class?
When you need lightweight data structures.
When you want value semantics.
When you want copy-on-write behavior.
When working with immutable data.
For example, modeling geometric shapes:
struct Point {
var x: Double
var y: Double
}
struct Circle {
var center: Point
var radius: Double
}
5. How is memory management done in Class and Struct?
Classes: Use Automatic Reference Counting (ARC). Memory is managed by increasing and decreasing reference counts.
Structs: Use copy-on-write. Copies are made only when necessary, avoiding unnecessary copying.
6. What is Copy-on-write in Swift? How are Array and String using Copy-on-write in Swift?
Copy-on-write is a memory optimization technique where data is only copied when changes are about to be made. Both Array
and String
in Swift use copy-on-write to improve memory efficiency.
var array1 = [1, 2, 3]
var array2 = array1 // No actual copying yet, both point to the same data
array2.append(4) // Now, a copy is made for array2
// array1 remains [1, 2, 3]
var str1 = "Hello"
var str2 = str1 // No copying yet
str2 += ", World!" // Now, a copy is made for str2
// str1 remains "Hello"
7. What are the types of access modifiers available in Swift?
Default:
internal
(accessible within the same module).Open vs Public:
open
allows subclassing and overriding in other modules, whilepublic
doesn't allow subclassing/overriding outside the module.Private vs Fileprivate:
private
limits access to the current scope,fileprivate
restricts access to the current source file.
8. What are the ways Read-only properties are created in Swift?
Read-only properties can be created using let
constants, computed properties with only a getter, and using the private(set)
modifier in conjunction with a public getter.
struct Circle {
let radius: Double // Read-only property using let
var diameter: Double {
return radius * 2 // Read-only computed property
}
private(set) var area: Double = 0.0 // Read-only property with private setter
}
9. Let vs. Var?
let
is used to declare constants whose values cannot be changed after assignment.var
is used to declare variables whose values can be changed after assignment.
let pi = 3.14159
var count = 10
count = 20 // Valid, because count is a variable
pi = 3.14 // Invalid, because pi is a constant
10. What is Private(set)?
Private(set)
is an access control modifier that restricts write access to a property. It allows the property to be read from any scope but only modified within the same scope or context it's defined in.
class BankAccount {
private(set) var balance: Double = 0.0
func deposit(amount: Double) {
balance += amount
}
// Cannot directly modify `balance` outside of this class
}
let account = BankAccount()
account.deposit(amount: 100.0)
print(account.balance) // Read access is allowed
account.balance += 50.0 // This would result in a compile-time error
That's Not It !!
This is just the beginning of our journey into the world of Swift iOS development interview preparation. We've covered 10 essential questions that delve into various aspects of the Swift language, memory management, access control, and more. However, the world of iOS development is vast, and there's so much more to explore.
Stay tuned as we continue to expand this resource with additional interview questions, covering topics like design patterns, asynchronous programming, UIKit and SwiftUI frameworks, networking, and much more. Our aim is to provide you with a comprehensive toolkit that empowers you to excel in your Swift iOS development interviews and showcase your expertise with confidence.
So, bookmark this page, subscribe to our updates, and keep an eye out for new questions and answers that will take your interview preparation to the next level. We're here to help you succeed and thrive in the dynamic realm of Swift iOS development interviews. Happy coding and interview prepping!
Subscribe to my newsletter
Read articles from RaiTech Labs directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
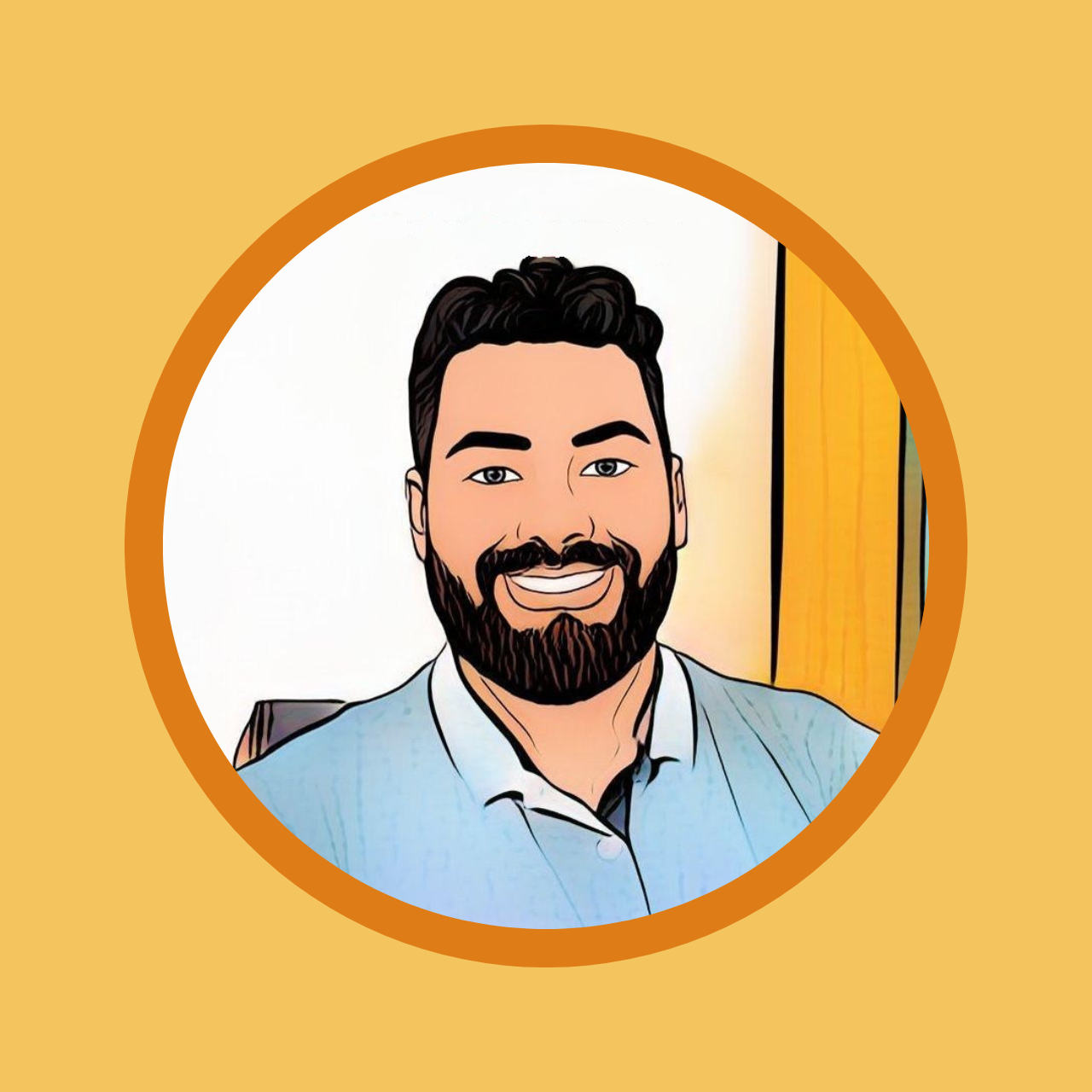
RaiTech Labs
RaiTech Labs
๐ Welcome to RaiTech Labs! I'm Keshu Rai, a passionate Mobile App Developer with 6+ years of experience. Join me on this exciting channel as we explore the world of programming and mobile app development. ๐ฑ๐ก Whether you're a seasoned coder or a beginner, RaiTech Labs is the place for you. I'm here to share my knowledge, insights, and tips to empower you on your coding journey. ๐ฏ From Swift to SwiftUI and Flutter, we'll cover a wide range of topics to help you become a pro developer. Get ready for tutorials, real-world examples, and coding best practices. ๐ Our mission is to build a community of ambitious developers, eager to grow and create amazing apps. Let's unlock your coding potential together! ๐ Join RaiTech Labs now and let's embark on this coding adventure. Remember, with passion and dedication, you can achieve anything in the world of coding! Happy coding! ๐ค