How React Works

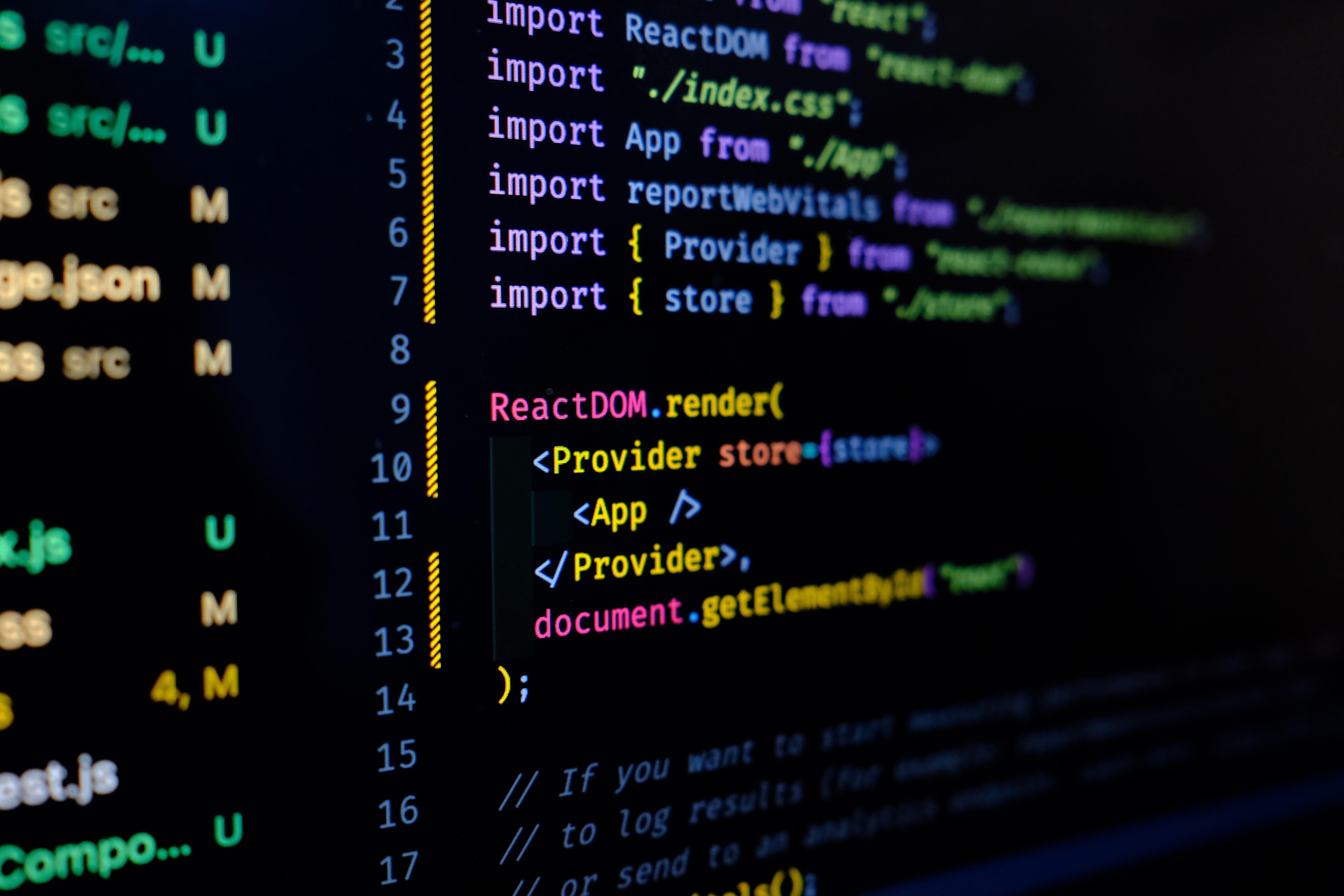
Table of Contents
Introduction to React
Importance of React in modern web development
Virtual DOM: Enhancing performance and rendering efficiency
Components: The building blocks of React applications
Setting Up a React Environment
How to install Node.js and NPM on Windows
Using react_scripts
Starting the Server
Introduction
What comes to your mind when you hear the word React.js?
React is used for developing swift and flexible interfaces. It's one of the popular JavaScript frameworks used. React is used to build many of the best websites in a range of sectors. React (also known as ReactJS or React.js) is a popular free and open-source front-end Javascript library for developing UI components and user interfaces. It is maintained by Meta and a group of individual developers and corporations. With frameworks such as Next.js, React may be used to create single-page, mobile, or server-rendered applications. Meta, Netflix, Uber, and Airbnb are among the most well-known firms that make use of the framework.
As Facebook's Ad app gained traction, its code began to encounter an inflow of new upgrades, which proved burdensome and difficult to handle after a while. Jordan Walke built FaxJS, a prototype that made this process more efficient, in an attempt to assist in solving this problem. This was the beginning of what would later become the version of React that we all know and love today. React was first launched in 2013, and it has since become one of the most popular JavaScript libraries for creating user interfaces.
The Importance of React in Modern Web Development
React is particularly efficient at managing complicated user interfaces. React can assist you in creating a responsive and smooth user experience if your online application contains a lot of dynamic and interactive features, such as real-time updates or data visualization.
1. Component-Based Architecture
React.js helps component-based architecture, which allows developers to divide user interfaces into reusable and modular components. Component-based design can help you develop efficient apps that respond to changing market needs for your application. The best part is that you don't have to hire expensive app developers to achieve it!
For companies looking to grow their front-end development operations, component-based architecture has become known as the go-to strategy. The shift in perception enables businesses to take advantage of the benefits realized by streamlining and scaling CSS across numerous products and page layouts. Nowadays, high-performing front-end applications are all about providing tailored, coherent experiences.
Component-based programming helps organizations to quickly harness these performance and functionality advantages. Component-based development is a software development strategy that focuses on creating reusable components. Consider a web page with elements such as a header, search bar, content body, and so on. When contemplating component-based architecture, all of these are independent of one another and are referred to as components.
2. Rapid Development
Component-based methods can help teams produce high-quality software up to 65% faster than non-component-based methodologies. Teams can avoid starting from scratch with their program by generating components from reusable libraries that are always accessible. They can choose directly from this library without regard for non-functional concerns like security, usability, or performance.
3. Simpler Maintenance
One of the most important benefits of component-based design is that each component is self-contained and reusable. It aids in the decomposition of the front-end monolith into smaller and more manageable components, making any upgrade or modification a breeze. Instead of changing the code every time, you only need to change the required components once.
4. Independent Teams
The cross-functional component teams accept the design-language system as a single source of truth and develop components independently without interference or help from outside sources. The components in this instance are independent, but they have no impact on the system.
5. Improved Reusability
There are many advantages to reuse, one of which is developing less code for commercial applications. When working with a component-based framework, developers can concentrate on core functionality rather than continually registering the same lines of code. Then they can use these same elements in additional apps that might cater to different purposes or be executed across a variety of platforms.
6. More Consistent UX
If you use an unsupervised front-end development technique, you run the danger of giving your customers uneven and confused experiences. However, if you use a component-based architecture, all the components made in the design document will automatically have a uniform user interface.
7. Enhanced Scalability
It is likely that the system will need to be prepared for expansion (and scalability) if a product is new and customers are joining up. Purpose-built components can fit together like jigsaw pieces thanks to component-based development.
Virtual DOM and Performance
The virtual DOM (VDOM) is a programming concept in which a library like ReactDOM maintains a "virtual" or "ideal" representation of a user interface in memory and keeps it in sync with the "real" DOM. Reconciliation is the name given to this procedure. When re-rendering the UI, React employs the virtual DOM as a method to compute the fewest possible DOM actions. It does not outperform or move more quickly than the actual DOM.
To help us write more predictable code, the virtual DOM offers a method that abstracts manual DOM manipulations away from the developer. A virtual DOM object has the same properties as a real DOM object, but it is unable to directly alter the content of the screen like a genuine DOM object can. The DOM takes time to manipulate.
Because nothing is drawn on the screen, manipulating the virtual DOM is much quicker. The Virtual DOM makes web applications faster and more effective by preventing needless updates and re-rendering the user interface each time a change is made. By lowering latency, the Virtual DOM enhances the user experience of an online application.
Because React updates the DOM automatically to match your render output, your components won't frequently need to modify it. To concentrate on a node, scroll to it, or determine its size and position, for example, you might occasionally need access to the DOM elements that React manages. Direct access to DOM objects is how traditional DOM manipulation is often carried out, usually in reaction to a user action like a button click.
Large and Active Community: The React.js community is active and supportive, which helps the library develop and advance. This community has made it easier for developers to learn and use React.js by making a wealth of documentation, tutorials, and open-source packages available.
Extensive Adoption by Prominent Companies: Thanks to the extensive use of React.js by well-known organizations like Facebook, Instagram, Airbnb, and Netflix, it is simple for React.js developers to find employment. Many businesses have adopted React.js because it meets the requirements of large-scale apps and enables the creation of user interfaces that are fast and feature-rich. Because React.js is compatible with other frameworks and libraries, developers may include it in already-existing applications or use it in conjunction with other tools like GraphQL or TypeScript.
The idea of components aids React in upholding the single-responsibility paradigm. React's building blocks are components. A component is a chunk of reusable code that corresponds to a certain user interface element. Complex user interfaces can be made using components that can be reused throughout an application. Components should be viewed as reusable parts of our application, each of which should (ideally) carry out just one duty or task. Simply put, a component is a function that specifies how data should be displayed in the UI and receives data (props).
Components: The building blocks of React applications
Depending on the component, it may be class-based or function-based.
Class-based Components
Stateful components or container components are other names for class-based components. These components are constructed as follows and have state and lifecycle methods:
Function-based Components
Functions that return React elements are known as functional components. These are less complicated parts that don't have lifecycle or state methods. They are JavaScript functions, hence the name "function-based." These components are also known as stateless or representational components (originally!) because their sole purpose is to display user interfaces.
Even though it doesn't look like it, everything in React is actually JavaScript (JS), so keep that in mind as we talk about it!
You must agree that the above variable declaration neither resembles HTML nor JS.
This is due to the use of JSX, a syntax extension for JS, and Babel (a compiler for next-generation JS), which compiles everything into JS code in the end.
This is due to the use of JSX, a syntax extension for JS, and Babel (a compiler for next-generation JS), which compiles everything into JS code in the end. So, by enclosing any JS expression in curly braces, such as variables, getting object properties, or even calling functions, we can use it in JSX code.
As you can see in the sample above, the getAddress function is called within the JSX code, and the curly braces are utilized to retrieve the address as a string type.
By batching several updates together, the Virtual DOM minimizes the need for layout calculations and repaints. Performance is enhanced as a result of the rendering process becoming more effective. Except for the fact that it is a lightweight copy, a virtual DOM object is identical to a real DOM object. This implies that it is unable to control on-screen objects. Additionally, it just updates the relevant nodes and not the entire tree when a property is changed.
The Virtual DOM makes web applications faster and more effective by preventing needless updates and re-rendering the user interface each time a change is made. By lowering latency, the Virtual DOM enhances the user experience of an online application. It is therefore a quick and effective substitute.
Setting up a React Environment
Node.js with npm (Node Package Manager) installation
A Javascript program can be run in Node.js because it includes all the necessary components. Programs are used to render the content on the server before it is sent to a web browser.
A tool and repository for creating and exchanging JavaScript code is called Node Package Manager, or NPM.
I'll show how to set up Node.js, NPM, and other helpful Node.js commands on a Windows computer.
Basic Requirements
a user account having access to download and install software or administrative rights
a user profile with administrative rights, such as the capacity to download and set up software
Access to the Windows command line (search > cmd > right-click > run as administrator) OR Windows PowerShell (Search > Powershell > right-click > run as administrator)
How to Install Node.js and NPM on Windows
Step 1: Download Node.js Installer
Go to https://nodejs.org/en/download/ via a web browser. Use the Windows Installer option to obtain the most recent default version. Version 10.16.0-x64 was the most recent version at the time this article was written. The Node.js installer comes with the NPM package manager.
Step 2: Download and install NPM and Node.js
1. Open the installer after it has finished downloading. Click the file after opening the downloads link in your browser. Alternatively, you can double-click the file by navigating to the location where you stored it to start it.
2. Click Run when the system prompts you to run the program.
3. After being welcomed, click Next to see the Node.js Setup Wizard.
4. Examine the license agreement on the next screen. If you are ready to install the software and accept the terms, click Next.
5. The installer will ask you where you want to install it. Click Next after selecting the default location unless you have a reason to install it somewhere else.
6. You can choose which components to include or leave out of the installation using the wizard. Again, click Next to accept the settings unless you have a compelling need to change them.
7. To launch the installer, click the Install option. Click Finish when it's finished.
8 When you're finished, be sure to check the installation.
To execute a remote script, you will construct a new application in this step using the npm package manager. The script will install all prerequisites and copy the required files into a new directory.
A package management program named npm was also deployed along with Node. In addition to installing JavaScript packages in your project, npm will also keep track of project information. Npm also comes with a program named npx that can execute packages. That implies that you won't download the project before running the Create React App code.
The create-react-app installation will be carried out by the executable package in the directory that you choose. It will begin by creating a new project in a directory with the name "remireact" for the sake of this tutorial. Again, the executable package will create this directory for you; it is not necessary for it to already exist. Additionally, npm install will be launched by the script inside the project directory, downloading any additional dependencies.
Run the following command to set up the base project:
npx create-react-app remireact
With this command, a build process will start, downloading the base code and all of its dependencies.
You will receive a success message that reads as follows when the script is finished:
A set of npm commands that you may use to execute, build, launch, and test your application will also be displayed. In the section after this, you'll learn more about these.
Your project has now been configured in a new directory. Enter the fresh directory:
cd remireact
You are now at the project's foundation. You have now added each dependency to a new project that you have established. However, you haven't done anything to manage the project. You will execute unique scripts to build and test the project in the following part.
Using React-scripts
You will discover more about the many react-scripts installed with the repository in this phase. To run the test code, you must first run the test script. The build script will then be executed to produce a minified version. You'll examine the eject script's ability to provide you with total customization power in the last section.
Take a look around the project directory now that you are inside of it. You have two options: open the entire directory in your text editor, or use the following command on the terminal to list the files:
ls -a
The -a flag ensures that the output also includes hidden files.
Either way, you will see a structure like this:
Let's examine this:
The application's external JavaScript libraries are all located in the directory node_modules/. You won't frequently need to use it.
There are some basic HTML, JSON, and image files in the public/ directory. These form the foundation of your endeavor.
Your project's src/ directory contains the React JavaScript code. You'll be working primarily in that directory.
The node_modules directory is one of the default directories and files that git, your source control, will ignore. These directories and files are listed in the gitignore file. Larger folders or log files that are unnecessary for source control are frequently among the ignored items. Additionally, it will contain a few directories that you'll make using a few of the React scripts.
There is a lot of helpful information about Create React App in the markdown file README.md, which also includes a list of commands and links to more complex configurations. It's better to keep the README.md file exactly how it is for the time being. You will replace the default information as your project develops with more thorough information.
The package manager in your computer uses the final two files. In addition to creating the base project when you did the initial npx command, you also installed the additional dependencies. A package-lock.json file was produced when the dependencies were installed. To make sure that the packages match exact versions, npm uses this file.
You can check that your project's dependencies are the same if someone else installs it in this manner. Since this file is generated automatically, you won't often directly change it.
A package.json file is the final one. This includes information about your project's metadata, including the title, version, and dependencies. You can utilize the scripts in it to manage your project.
Starting the Server.
You will start a local server in this stage and launch the project in your browser.
You run another npm script to launch your project. This script does not require the run command, similar to npm test. When you run the script, a local server is started, the project code is executed, a watcher that monitors code changes is started, and the project is opened in a web browser.
In the root of your project, use the following command to launch the project. The remireact directory is the project root for this lesson. you prevent this script from continuing indefinitely, be sure you open it in a different terminal or tab:
npm start
Before the server starts up, you'll see some placeholder text with the following output:
giving this output:
The script will launch the project in your browser window and switch the focus from the terminal to the browser if you are running it locally.
If that doesn't happen, you can view the site in action by going to http://localhost:3000/. It's okay if you already have another server operating on port 3000. The server will be started using the next accessible port that Create React App will detect. That is to say, if a project is currently operating on port 3000, the new project will begin on port 3001.
Without any further configuration, you can still see your site if you are running this from a distant server. It will have the URL http://your_server_ip:3000. You must open the port on your remote server if your firewall is set up.
You will see the following React template project in the browser:
Subscribe to my newsletter
Read articles from Oluwaremilekun Adebowale directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
