Infosys Interview: React + Node.js Questions & Answers.
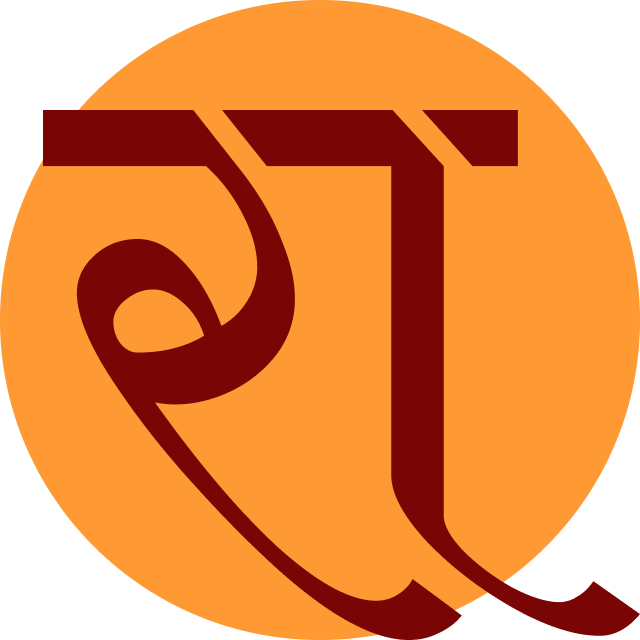
Table of contents
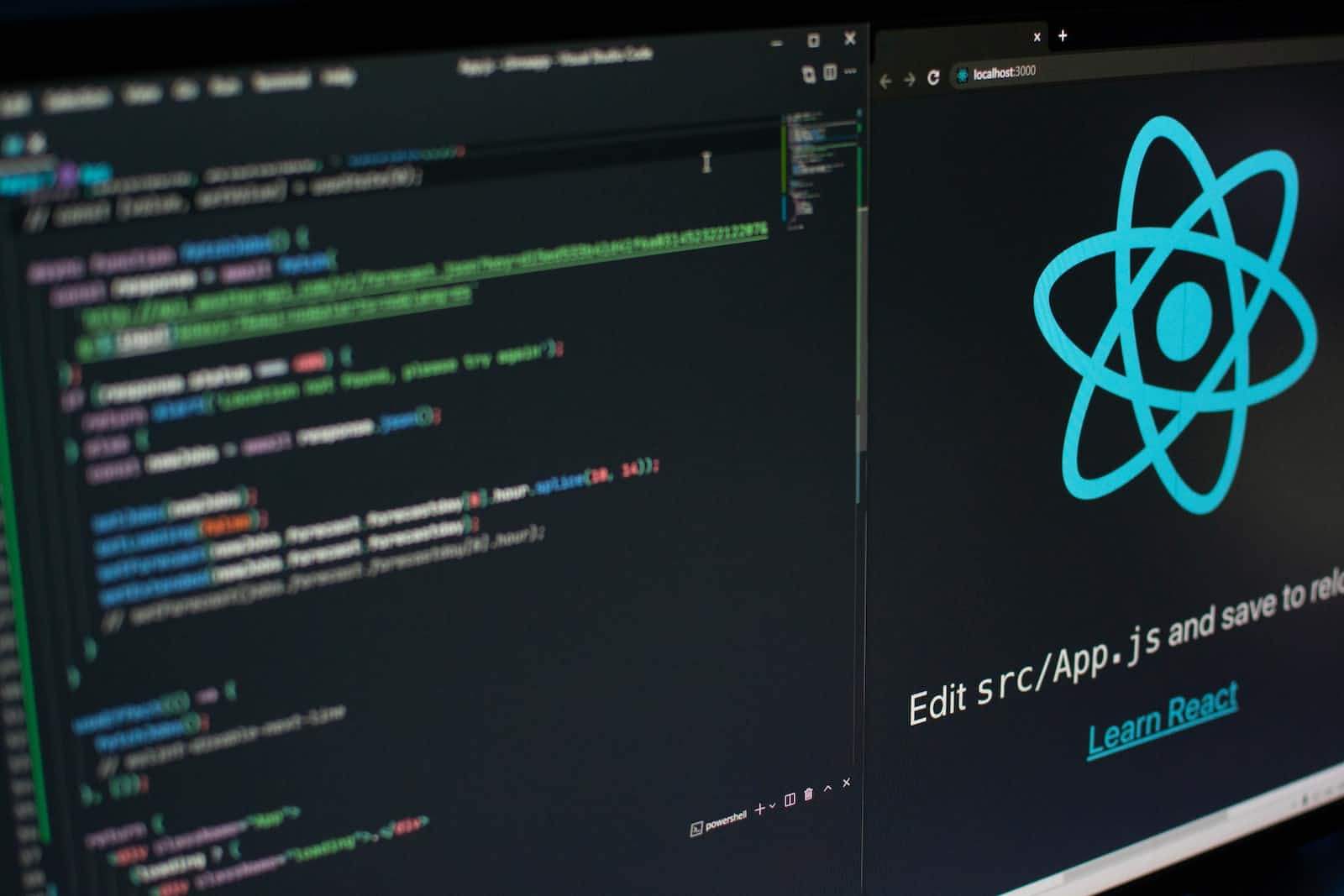
For preparing to react + node.js interview in Infosys. Here is the list of questions I could find from various sources...
Advantages of react over other angular/vue
react is a javascript library that is used to build UI. It is easier to work with react than it is with vanilla JS. React only deals with how to manipulate DOM using a technique called Virtual DOM manipulation, which makes it easier and faster than it is to work with Vanilla JS.
It is different from angular that React only deals with DOM. while angular is a complete framework for designing frontend web apps. it has its own router, state management and many more. You can create complete web app with react but that would require tools & they are opinionated. so user have choice to use whatever they want. React deals with DOM manipulation & it does it efficiently and best way possible.
Vue, on the other hand, is similar to react. It also uses Virtual DOM to keep track of UI state. But it is not that popular. So there are not many options when using tools/libraries.
What is Virtual DOM?
DOM stands for Document Object Model. It is structured representation of actual DOM. since it is present in memory and not in webapp. so it is called virtual. DOM is represented as Tree data structure where each node is an UI element. when any state changes then the new virtual dom is calculated efficiently of the supposed UI component. then the changes reflects in actual UI. it also gives us the flexibility to control the rendering of ui thus making it more performant than to work with vanilla JS.
Lifecycle method of react class Components?
There are 3 phases of a component rendering:
Mounting
constructor()
: states, and functions bindings are done.getDerivedStateFromProps():
state and functions bindings are done from outside of classrender()
: Virtual DOM is created using JSX structure & logiccomponentDidMount()
: place where we do other side stuffs, here we have access to real DOM.
Updating
getDerivedStateFromProps()
: states are again initialised based on the previous state, similarly bindings are performed. receivesprops
&states
as arguments.shouldComponentUpdate()
: for a normal component (extended fromReact.Component
, it returnstrue
by default. Here we decide whether we should let the component re-render or not.render()
: Here Virtual DOM is created based on JSX syntax & logic.getSnapshotBeforeUpdate(props, states)
: Here we still have access to previousprops
&states
even though states & props has changed. last place to know previous state, used for logging.componentDidUpdate()
: this place is used to perform side effects. HTML DOM is updated.
Unmounting
componentWillUnmount()
: called when component is about to be unmounted, removed from DOM. component is also unmounted before updating.
What is a pure component ?
A pure components is used for optimizing a react app. It differs from regular component that a regular component doesn't implement shoudComponentUpdate
method. they by default returns true
for this. hence a regular will always update if its props
or states
changes. on the other hand. a pure component implements shouldCompoenntUpdate
method. shouldComponentUpdate
method receives nextProp
and nextState
as arguments. and it does shallow comparison & returns true
if any change is detected.
What are promises? What is use of async & await?
a promise is a representation of eventual completion(or failure) of an asynchronous operation and its resulting value. A promise in one of these states:
pending: initial state, when promise is created, means task is undergoing execution
fulfilled: the task at hand completed successfully.
rejected: the task at hand failed.
the eventual state of a promise can either be fulfilled or rejected as pending is a temporary state.
async/await is just a syntactical sugar for promises introduced in es6. it makes whole async code writing like we are writing a normal code, where we use try/catch block to catch errors.
What is the difference between let, const & var?
All three are used to declare a variable in javascript. variables creatad using var are stored as key-value pair on the window object. and it is globally available as windows
object is globally available. if the variable is changed anywhere along the execution. be it deeply nested, the new value will reflect from there.
A variable created using let
are lexically(block) scoped. means it is present only within that block. It is also stored in block memory so it is local. if it is defined inside a block then it can't be accessed outside of that block.
A variable created using const
behaves totally like a variable created using let
. the only difference is that once a variable is assigned a value, it can't be changed. if the variable holds an object, we can only add key-value pair into it. if that variable holds an array/list then we can only add/remove element from the array.
Describe how promises work.
Promises are used to carry out asynchronous tasks which generally take long time. the idea is that we let the asynchronous task execute in the background then once that is completed & output is returned, that output should be passed to the next function which can be a promise or another function. this whole execution should not block any other code execution. so it is essentially simplification of passing callbacks to another functions.
for creating a promise, we use Promise
constructor which takes an function as an argument. the function has two arguments which are basically actions : resolve & reject. resolve
is executed when the task defined inside promise is successfully completed. if any error occured,then we execute reject
const promise = new Promise((resolve, reject) => {
const num = Math.random();
if (num >= 0.5) {
resolve("Promise is fulfilled!");
} else {
reject("Promise failed!");
}
});
What is closure?
A closure is a function along with its lexical scope. Lexical scope being the area where that function is accessible or parent scope. so basically when a variable/function is accessed inside a function then its defintion is first located inside the function where it is used. if not found, then it is located in function's parent block, again not found then that block's parent block, all the way upto global scope. so whenever a function is pushed into callstack, it is in the form of a closure so that whatever the function needs, it is with it.
What is JSX?
JSX stands for JavaScript XML. It is a syntax extension of javascript, which allows HTML to be directly written inside javascript code. It is syntactical sugar for defining structure of a virtual DOM. It is faster than normal JavaScript as it performs optimizations while translating to regular JavaScript. It is not mendatory to use it while creating html structure, the same can be achieved by using React.createElement()
. but JSX provides simplicity. it also provides optimizations.
What is useEffect()
hook in react?
This hook is used only in functional component. it won't work in class components. it is equivalent of componentDidMount()
, componentDidUpdate()
& componentWillUnmount()
in different scenrios. This hook is used to do some side stuffs when functional component is mounted. we can peform all sorts of sync/async kind of stuffs here. even DOM manipulation.
useEffect function takes two arguments:
a callback function that needs to do some particular stuffs
an array of dependencies.
When the empty array is passed, then it behaves like a componentDidMount
as it will be called only once. when some state/prop dependencies are passed then it behaves like componentDidUpdate()
when we return a callback from the first callback function then returned callback will be called when the component is unmounted.
What are hooks?
hooks are functions that let you "hook into" React state & lifecycle features from the function components. hooks don't work inside class components.
Some examples are: useState()
, useEffect()
and useContext()
What is context
in react?
Context in react is a way to pass data from a parent component to deeply nested children(grandchildren) components. The parent component makes data available to the whole children tree through context. When a child at any depth has to use that data, then the children can simply take from context. which otherwise would require passing data down from parent to all its children who subsequently passed them to their children.
What is redux? what is the use of redux?
redux is a state management library which can be used with any JS library. but mainly we use it with react as other framework generally has their state management libraries. redux takes the responsibility of managing global state away from react and provides some actions. which component in react tree uses for its storage & manipulation of state in redux store.
Similarities & difference between context & redux.
Similarities:
both provide a way to manage states & a way to manipulate them
both provide state globally, if we consider root element as parent, then all children have access to states.
Difference of | React context | Redux |
Purpose | Share data between components | Centralized state management |
Component | Provider and Consumer | Store, Actions, and Reducers |
State Updates | Updates can happen frequently | State updates are predictable |
State Changes | State changes can be made anywhere | State changes are only made through actions and reducers |
Time-Travel Debugging | Not supported | Supported |
Size of Application | Suitable for small and medium-sized applications | Suitable for small and medium-sized applications |
Difference between useMemo & useCallback?
useCallback and useMemo are both React Hooks that help optimize the performance of a React application by memoizing values. They both accept a function as an argument and return a memoized version of the function.
useCallback is a hook that returns a memoized callback.
useMemo is a hook that returns a memoized value.
Subscribe to my newsletter
Read articles from Rajesh Kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
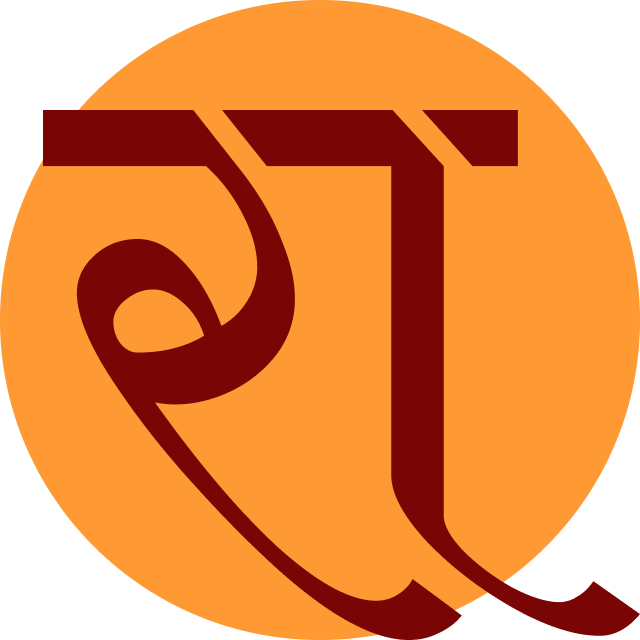
Rajesh Kumar
Rajesh Kumar
I am developer from Madhepura, Bihar, Graduated from Assam university