Building a Todo API with Django and GraphQL: A Comprehensive Guide

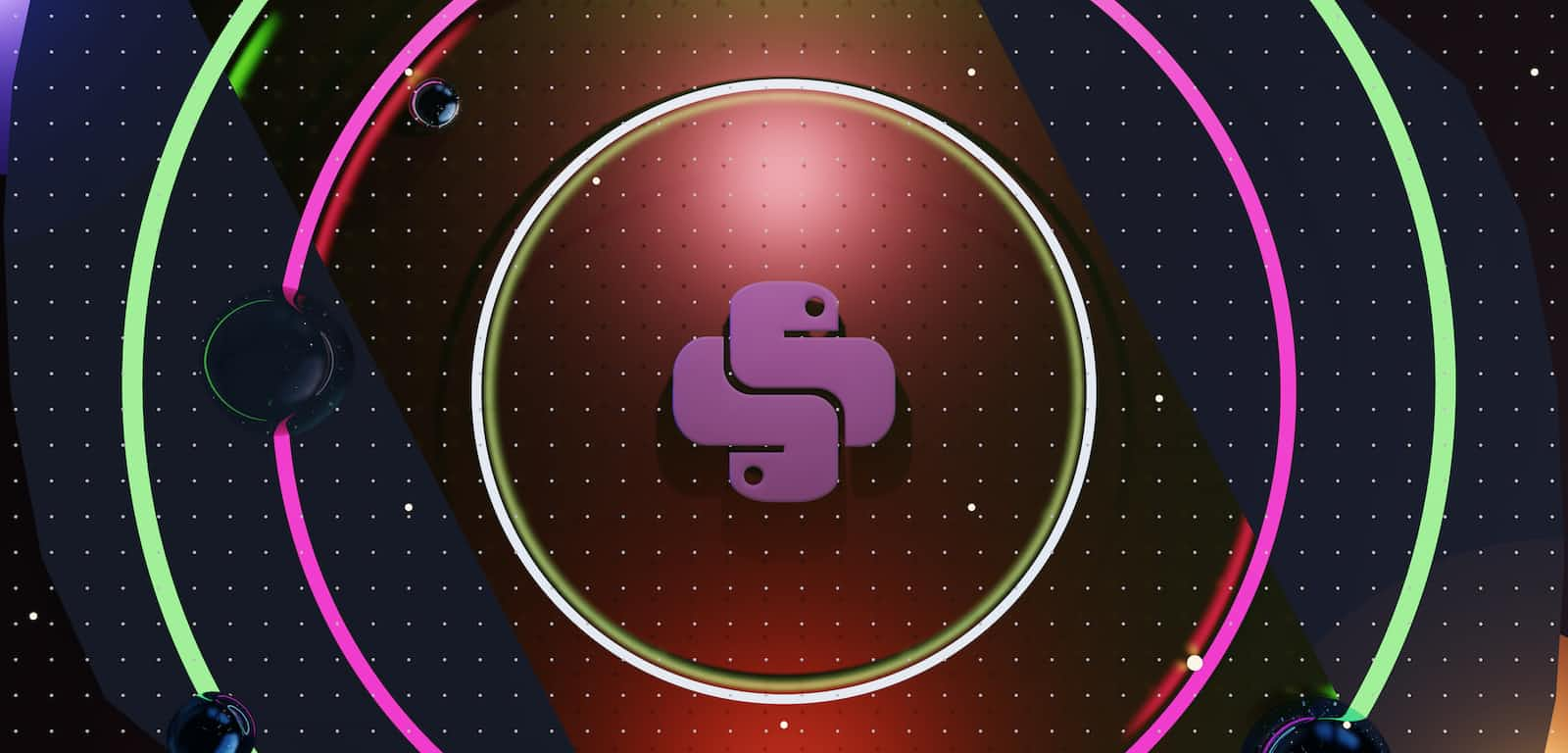
Introduction
In the digital landscape, Application Programming Interfaces (APIs) play a pivotal role in enabling seamless communication between different software components. They serve as bridges that allow applications to interact and share data efficiently. One emerging star in the API world is GraphQL – a query language for APIs that offers flexibility and efficiency in data retrieval.
At the heart of this technological synergy lies Django, a widely acclaimed web framework. Renowned for its simplicity and scalability, Django provides an ideal foundation for constructing robust APIs. With its powerful features and built-in tools, Django simplifies the API development process and accelerates the creation of sophisticated backend systems.
In this era of productivity, the ability to manage tasks and projects efficiently is paramount. A dynamic Todo API built on Django and GraphQL not only streamlines task management but also offers a playground for understanding the intricate dance between modern technologies. By blending Django's prowess with GraphQL's agility, we unlock a world of possibilities for crafting a ToDo API that meets the demands of today's fast-paced digital landscape.
Setting Up Your Django Project
Creating a solid foundation for your Django project is crucial for a smooth development journey. Let's dive into the steps of setting up your new project.
Run the following command to create a new Django project named "TodoApi":
django-admin startproject TodoApi
Virtual environments are essential to keep your project's dependencies isolated from your system's global Python environment. Create a virtual environment within your project directory:
cd TodoApi
python -m venv venv
Activate the virtual environment:
On Windows:
venv\Scripts\activate
On macOS and Linux:
source venv/bin/activate
With your virtual environment active, you can install the required packages using pip
. For your Django project, you'll need packages like Django itself and Graphene for GraphQL integration:
pip install django graphene-django
Your Django project's structure will look something like this:
TodoApi/
├── manage.py
├── TodoApi/
│ ├── __init__.py
│ ├── asgi.py
│ ├── settings.py
│ ├── urls.py
│ └── wsgi.py
└── venv/
manage.py
: Command-line utility for interacting with your project.TodoApi/
: This is your project's Python package.__init__.py
: Marks the directory as a Python package.asgi.py
: ASGI entry point for running your application.settings.py
: Configuration settings for your project.urls.py
: URL patterns for routing requests to views.wsgi.py
: WSGI entry point for running your application.
venv/
: Your virtual environment.
GraphQL Configuration:
You can specify GraphQL-specific settings in settings.py
. For example, you can set the GraphQL endpoint URL and enable CSRF protection:
GRAPHENE = {
'SCHEMA': 'todos.schema.schema', # Path to your GraphQL schema
'CSRF_COOKIE_SECURE': True, # Enable CSRF protection for GraphQL
}
Defining the Data Model:
In Django, models represent the structure of your data and define how it will be stored in the database. Create a new app within your project to manage your ToDo items:
python manage.py startapp todos
Add your todos
app to the INSTALLED_APPS
list in settings.py
:
INSTALLED_APPS = [
# ...
'todos',
]
Open todos/models.py
and define your ToDo model:
from django.db import models
class ToDoItem(models.Model):
title = models.CharField(max_length=200)
description = models.TextField()
created_at = models.DateTimeField(auto_now_add=True)
completed = models.BooleanField(default=False)
def __str__(self):
return self.title
Creating the Database Schema:
After defining your model, you need to apply these changes to the database to create the necessary tables. Run the following commands:
python manage.py makemigrations todos
python manage.py migrate
These commands generate the database schema based on your model's structure.
Creating GraphQL Schema
GraphQL revolutionizes how we interact with APIs, offering advantages over traditional REST APIs. Let's delve into creating a GraphQL schema for your ToDo API using Graphene.
Graphene is a Python library that simplifies the creation of GraphQL schemas. Open todos/schema.py
and define your GraphQL types, Queries and Mutations:
import graphene
from graphene_django import DjangoObjectType
from .models import Todo
class TodoType(DjangoObjectType):
class Meta:
model = Todo
class AddTodoMutation(graphene.Mutation):
class Arguments:
title = graphene.String(required=True)
description = graphene.String(required=True)
todo = graphene.Field(TodoType)
@classmethod
def mutate(cls,root,info,title,description):
todo = Todo(title=title,description=description)
todo.save()
return AddTodoMutation(todo=todo)
class UpdateTodoMutation(graphene.Mutation):
class Arguments:
id = graphene.ID()
title = graphene.String(required=True)
description = graphene.String(required=True)
completed = graphene.Boolean()
todo = graphene.Field(TodoType)
@classmethod
def mutate(cls,root,info,id,title,description,completed):
todo = Todo.objects.get(id=id)
todo.title = title,
todo.description = description,
todo.completed = completed
todo.save()
return UpdateTodoMutation(todo=todo)
class DeleteTodoMutation(graphene.Mutation):
class Arguments:
id = graphene.ID()
todo = graphene.Field(TodoType)
@classmethod
def mutate(cls,root,info,id):
todo = Todo.objects.get(id=id)
todo.delete()
class Query(graphene.ObjectType):
todos = graphene.List(TodoType)
todo = graphene.Field(TodoType,id=graphene.Int())
def resolve_todos(root,info):
return Todo.objects.all()
def resolve_todo(root,info,id):
return Todo.objects.get(pk=id)
class Mutation(graphene.ObjectType):
add_todo = AddTodoMutation.Field()
update_todo = UpdateTodoMutation.Field()
delete_todo = DeleteTodoMutation.Field()
schema = graphene.Schema(query=Query,mutation=Mutation)
Routing (urls.py):
Define your URL patterns for the GraphQL API in TodoApi/urls.py
:
from django.urls import path
from graphene_django.views import GraphQLView
from .schema import schema
urlpatterns = [
path('graphql/', GraphQLView.as_view(graphiql=True, schema=schema)),
]
Here, we're using GraphQLView
from graphene_django.views
to handle GraphQL queries and mutations. The graphiql=True
parameter enables the interactive GraphiQL interface for testing your API.
Examples of CRUD Operations:
With your mutations in place, you can use tools like GraphiQL to interact with your API. For example, you can create a new ToDo item:
mutation {
addTodo(title: "Sample Title", description: "Sample Description") {
todo {
id
title
description
}
}
}
Advantages of a Flexible API:
GraphQL's flexibility shines here. Clients can request just the fields they need, reducing over-fetching. Complex queries can be handled in a single request. Additionally, as your data model evolves, you can update your schema without affecting existing clients, providing robust backward compatibility.
Conclusion
In this journey, we embarked on the path of crafting a powerful ToDo API using Django and GraphQL. We explored the synergy of Django's web framework and the flexibility of GraphQL to create a dynamic and efficient API for managing tasks and projects. lving data structures, GraphQL seamlessly adapted to our requirements.
Our journey doesn't end here. It's a stepping stone for further exploration and enhancement. From refining authentication and testing strategies to optimizing performance, your ToDo API can continue to evolve and thrive in the ever-changing landscape of software development.
As you embark on your journey to build APIs, remember that Django and GraphQL are your allies, offering a powerful combination that empowers you to bring your ideas to life. The world of APIs is vast and exciting and armed with the knowledge you've gained, you're ready to shape it according to your vision. Happy coding!
Subscribe to my newsletter
Read articles from Johanan Oppong Amoateng directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Johanan Oppong Amoateng
Johanan Oppong Amoateng
I am a self taught backend and mobile developer. I use Django,Flask or Node for my backends and for mobile i use either Jetpack Compose or Flutter. I love watching anime