Authenticating apps via Client Credentials in .NET
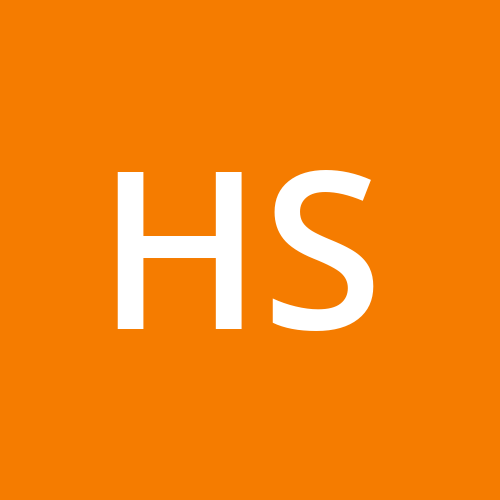
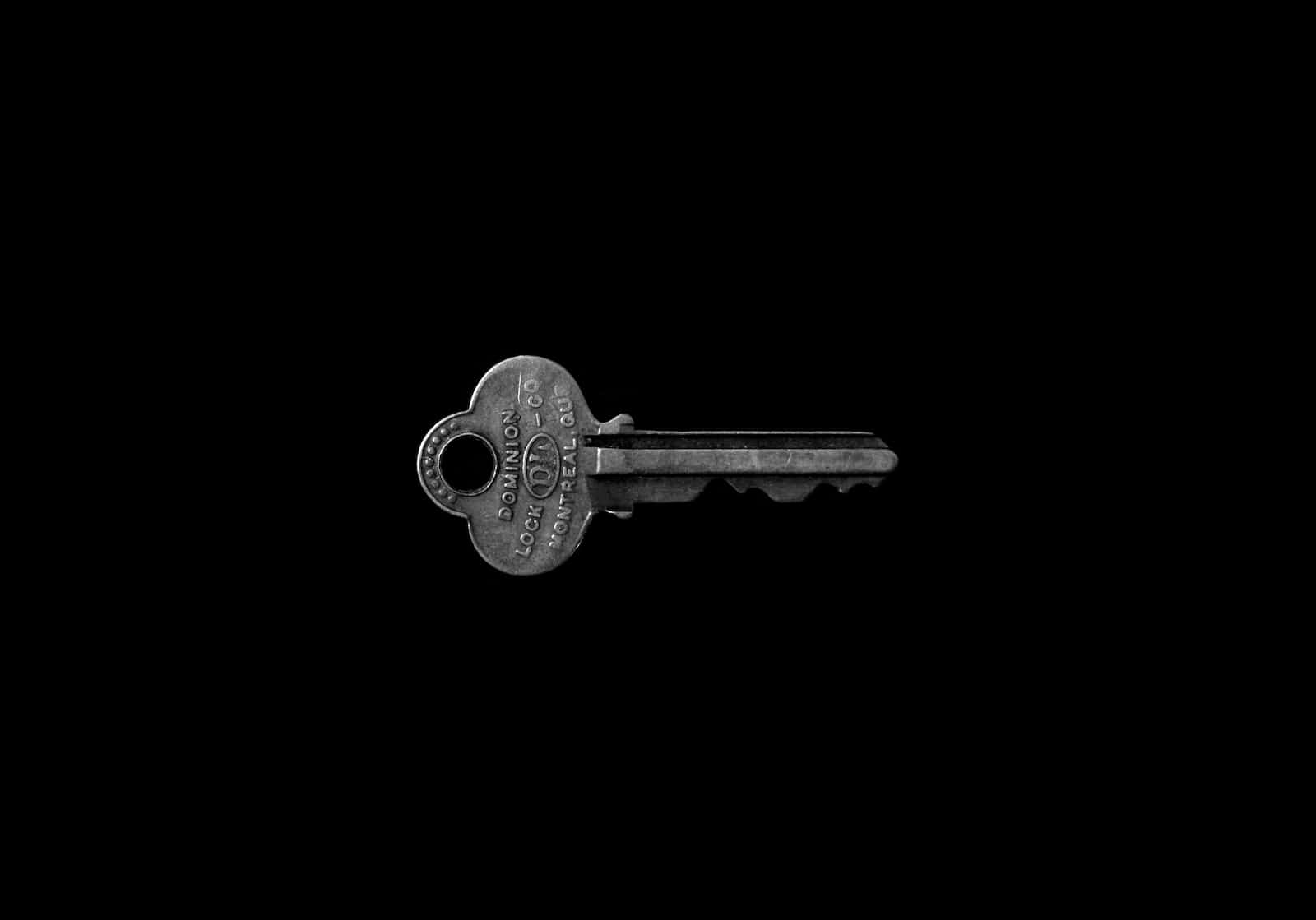
Authentication is a critical aspect of securing APIs and ensuring that only authorized clients can access the resources they are entitled to. One common method of authentication is using client credentials, where the client (usually another application or service) presents its credentials to the API to prove its identity. In this article, we will explore how to implement client credential authentication in a C# Web API.
Client Credentials
Client credentials refer to the authentication credentials used by an application or client to prove its identity when accessing resources or services from an authorization server. Client credentials are a way for an application to assert its identity to a server or an authorization service. This is commonly used in server-to-server communication or when an application needs to access an API without involving a user.
Client credentials typically consist of two components:
Client ID: A unique identifier assigned to the client application by the authorization server during the registration process. The client ID is usually a string value and is used to identify the client when making authentication requests.
Client Secret: A secret key shared between the client application and the authorization server. The client secret is known only to the client and the authorization server. It is used to prove the client's identity and establish a level of trust between the client and the server.
Here's a typical flow for using client credentials to authenticate an application in OAuth 2.0:
The client application registers with the authorization server and receives a client ID and a client secret. The process of generating client credentials involves creating a unique client ID and a corresponding client secret that the client application uses to authenticate itself with the authorization server.
When the client application needs to access a protected resource, it sends a request to the authorization server with its client ID and client secret.
The authorization server validates the client credentials and issues an access token if the credentials are valid.
The client application uses the received access token to access the protected resource by presenting it in the request headers.
Steps to Implement Client Credential Authentication on Client Side
Follow these steps to implement client credential authentication in your C# Web API:
Step 1: Create a New Web API Project
Open Visual Studio and create a new ASP.NET Core Web API project. You can use the Visual Studio wizard to create a new project.
Step 2: Configure Authentication
In the Startup.cs
file, you need to configure authentication for your Web API. In this case, we'll use the OAuth 2.0 framework to implement client credentials flow.
Add the necessary using statement at the top of the file:
using Microsoft.AspNetCore.Authentication.JwtBearer;
using Microsoft.IdentityModel.Tokens;
Inside the ConfigureServices
method, configure the authentication:
public void ConfigureServices(IServiceCollection services)
{
// ... other configurations
services.AddAuthentication(JwtBearerDefaults.AuthenticationScheme)
.AddJwtBearer(options =>
{
options.Authority = "https://your-auth-server"; // Replace with your auth server URL
options.TokenValidationParameters = new TokenValidationParameters
{
ValidateAudience = false // If not validating audience
};
});
// ... other configurations
}
Replace "
https://your-auth-server
"
with the actual URL of your authorization server. Make sure you also adjust any other parameters according to your setup.
Step 3: Secure Your API Endpoints
To secure your API endpoints, you can use the [Authorize]
attribute on your controllers or actions that require authentication. This will ensure that only authenticated clients can access those endpoints.
[ApiController]
[Route("api/[controller]")]
[Authorize]
public class MyController : ControllerBase
{
// Your API actions
}
Step 4: Testing the Client Credential Flow
To test the client credential authentication flow, you need to obtain an access token from your authorization server. This access token is then sent in the Authorization
header of your API requests.
Here's a sample code snippet that demonstrates how a client application could obtain an access token using the HttpClient
class:
var client = new HttpClient();
var tokenEndpoint = "https://your-auth-server/token"; // Replace with your token endpoint URL
var tokenResponse = await client.RequestClientCredentialsTokenAsync(new ClientCredentialsTokenRequest
{
Address = tokenEndpoint,
ClientId = "your-client-id",
ClientSecret = "your-client-secret",
Scope = "your-api-scope"
});
if (tokenResponse.IsError)
{
Console.WriteLine(tokenResponse.Error);
}
else
{
var accessToken = tokenResponse.AccessToken;
// Use the access token in your API requests
}
Replace "
https://your-auth-server/token
"
with the actual token endpoint URL, "your-client-id"
and "your-client-secret"
with your client credentials, and "your-api-scope"
with the desired scope.
Steps to Implement Client Credential Authentication on Auth Server
Assuming you are building the authorization server using ASP.NET Core, here's how you could implement the authentication and token issuance process:
Step 1: Set Up Startup Configuration
In the Startup.cs
of your authorization server project, you would configure the authentication and token issuance process.
public void ConfigureServices(IServiceCollection services)
{
// ... other services and configurations
services.AddAuthentication("Bearer")
.AddJwtBearer("Bearer", options =>
{
options.Authority = "https://your-auth-server"; // URL of the authorization server
options.TokenValidationParameters = new TokenValidationParameters
{
ValidateAudience = false // If not validating audience
};
});
// ... other services and configurations
}
Replace "
https://your-auth-server
"
with the actual URL of your authorization server.
Step 2: Implement Client Credentials endpoint
Creating an endpoint in the authorization server's Web API to generate client credentials involves a few steps. Here's a basic example of how you could implement this in an ASP.NET Core Web API.
Assuming you have a Client
model to represent client information:
public class Client
{
public string Id { get; set; }
public string Secret { get; set; }
// Other properties like redirect URIs, scopes, etc.
}
Here's how you could create an endpoint to generate client credentials:
In this example, the /api/clients/generate
endpoint generates a new client ID and secret, stores the client in-memory (you should use a database or more persistent storage in a real-world scenario), and returns the generated credentials to the caller.
Please note that this example is for demonstration purposes and lacks security measures like authorization checks, secure storage, and input validation. In a production environment, you should implement additional security measures to protect the client credentials and ensure the endpoint is accessible only by authorized personnel.
Step 3: Token Endpoint Implementation
Create a token endpoint in your authorization server's controller. This endpoint would handle the client credential authentication request and issue an access token.
[ApiController]
[Route("token")]
public class TokenController : ControllerBase
{
[HttpPost]
public async Task<IActionResult> IssueTokenAsync()
{
// Perform client authentication (check client credentials)
if (!IsValidClientCredentials(Request.Form["client_id"], Request.Form["client_secret"]))
{
return BadRequest("Invalid client credentials.");
}
// Generate and return an access token
var accessToken = GenerateAccessToken();
return Ok(new { access_token = accessToken, token_type = "bearer" });
}
private bool IsValidClientCredentials(string clientId, string clientSecret)
{
// Logic to validate client credentials against a database or configuration
// Return true if valid, false otherwise
}
private string GenerateAccessToken()
{
// Logic to generate an access token using a library like System.IdentityModel.Tokens.Jwt
// Return the generated access token
}
}
In the above code, the IsValidClientCredentials
method checks if the provided client credentials are valid. The GenerateAccessToken
method generates an access token, which can be implemented using the System.IdentityModel.Tokens.Jwt
library or any other library you prefer.
The IsValidClientCredentials
method in the authorization server's token endpoint would typically involve validating the client credentials against a database or a predefined configuration. Here's a simplified example of how the body of the IsValidClientCredentials
method could look:
private bool IsValidClientCredentials(string clientId, string clientSecret)
{
// In a real-world scenario, you would query a database or some configuration store
// to check if the provided client credentials (clientId and clientSecret) are valid.
// For demonstration purposes, let's assume a predefined list of valid client credentials.
var validClients = new Dictionary<string, string>
{
{ "client1", "secret1" },
{ "client2", "secret2" }
};
if (validClients.TryGetValue(clientId, out var expectedSecret))
{
return clientSecret == expectedSecret;
}
return false;
}
In the example above, the IsValidClientCredentials
method checks if the provided clientId
exists in the validClients
dictionary and whether the provided clientSecret
matches the expected secret associated with the client. This is a simplified representation, and in a real-world scenario, you would likely replace the dictionary with a database query or a more secure and scalable configuration mechanism.
Remember that client secrets should be securely stored and never exposed in the source code or publicly accessible files. In a production environment, you would manage and store client credentials securely, possibly using environment variables, secret management tools, or other secure storage mechanisms.
Subscribe to my newsletter
Read articles from Hemant Singh directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
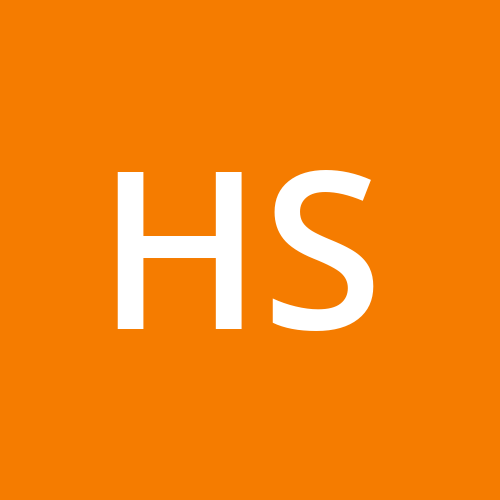