Swift Language
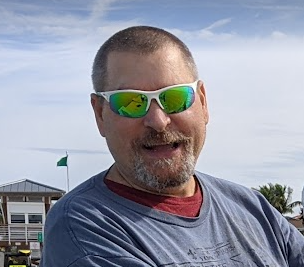
Table of contents
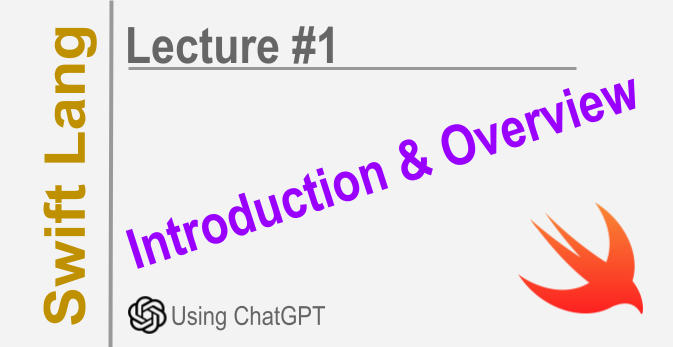
Swift is a general-purpose programming language developed by Apple Inc. It was originally introduced in 2014 at the Apple WWDC as a replacement for Objective-C. Some key points about Swift:
Swift is designed to work with Apple's Cocoa and Cocoa Touch frameworks and the large body of existing Objective-C code written for Apple products.
It is a simple, modern language with object-oriented features, protocols and generics.
It avoids many common programming mistakes like null pointers and provides memory safety and type safety.
Code is more readable and concise due to features like string interpolation, optional types, range operators, etc.
It has high-level features like closures and automatic reference counting.
Swift compiles code down to native Apple machine instructions for optimal performance.
It is fully open-source and free to use.
Swift is mainly used to develop software for Apple platforms like iOS, watchOS, tvOS and macOS. Some popular apps built with Swift are Instagram, Uber, Pinterest, LinkedIn, Spotify, etc.
Swift Platforms
Swift currently does not run natively on Linux or Windows platforms. It has been designed specifically to work with Apple's SDKs and frameworks. However, there are some community efforts to port Swift to run on other platforms:
There is a project called Swift for TensorFlow which allows using Swift as a TensorFlow frontend on Linux. This allows Swift to define and train machine-learning models on Linux.
There are projects like SwiftPM, SwiftSh, and CSwift that aim to implement the core parts of Swift like the compiler and standard library to allow running Swift on other platforms. But these are still in the early stages.
There are some experimental efforts to create a Linux port of the official Swift compiler but nothing official from Apple yet.
So in summary, while Swift currently only runs natively on Apple platforms, there are some community efforts underway to port Swift to run on Linux and Windows as well. But an official cross-platform version of Swift from Apple is still lacking.
The main hurdle is that Swift uses many Apple-specific APIs and frameworks which would need alternatives on other platforms. So a fully cross-platform Swift would require significant work from Apple to make it possible.
Running Swift
You can run Swift code on replit.com. Replit is an online IDE (integrated development environment) that supports many programming languages, including Swift.
To run Swift on Replit, you can do the following:
Go to replit.com and sign up for a free account.
Click the "New Repl" button and select "Swift" from the language dropdown.
You'll see a new Repl with a Swift template code. You can modify this code and add your own Swift files.
Replit provides a simulator so you can run and test your Swift code directly in the browser.
You can also run Swift command line tools on Replit. It has a built-in terminal for that.
Replit supports features like version control, collaborating with others, and deploying your Swift code to various environments.
So in summary, yes Replit is a great option to write, run and test Swift code directly in your browser - without having to install Xcode or any other IDE locally. It's a perfect choice for learning Swift or prototyping Swift projects.
The main benefits of using Replit for Swift development are:
You can code directly in the browser from any device
It's free and easy-to-use
You get an online iOS simulator
Supports collaboration and version control
Allows deploying to various environments
Code Example
Here is a simple Swift code example with comments:
// Function to calculate the average of an array of integers
func calculateAverage(_ numbers: [Int]) -> Int {
// Variable to store the sum of all numbers
var sum = 0
// Loop through each number in the array
for number in numbers {
// Add the number to the sum
sum += number
}
// Return the sum divided by the number of elements
return sum / numbers.count
}
// Test the function
let numbers = [1, 3, 5, 7]
let average = calculateAverage(numbers)
print(average) // Prints 4
This code defines a function calculateAverage() that takes an array of integers and calculates the average.
It does the following:
It declares a sum variable to store the total sum of all numbers.
It uses a for-in loop to iterate through each number in the array.
It adds each number to the sum variable.
Finally, it returns the sum divided by the number of elements in the array, which gives us the average.
We then test the function by passing in a sample array of integers and printing the result.
The main highlights of the code are:
A function declaration with a parameter and return type
Variable declaration and initialization
for-in loop to iterate an array
Arithmetic operators like += and /
return statement
Calling the function and printing the result
Conclusion
While you do not currently have a Mac laptop or desktop, you can still start learning the Swift programming language now. Swift is a powerful and intuitive programming language created by Apple for building iOS, macOS, watchOS and tvOS apps. Even though you cannot yet create full native Mac apps without a Mac computer, there are still many benefits to learning Swift:
• Swift has great documentation, tutorials and online courses that are freely available. You can learn the basics of the language like syntax, data types, functions, classes, optionals and other concepts using these online resources.
• You can write and test small Swift programs in online code editors like Repl.it or CodeSandbox. This will help you gain familiarity with the language and build programming skills.
• Learning Swift will prepare you to start creating native Mac apps once you have access to a Mac computer. The knowledge you gain now will be directly applicable then.
• Understanding Swift will give you a head start in your career as an iOS or Mac app developer. You can highlight your Swift skills when applying for relevant jobs.
• Swift is a general-purpose language that shares similarities with other languages like JavaScript, Java and C#. The concepts you learn in Swift can be transferred to other languages as well.
However, to effectively use Swift to create native apps for the Mac platform, having access to a Mac laptop or desktop is essential. This is because you need Xcode - Apple's official integrated development environment for creating Mac software - which is only available on Mac computers.
So in summary, while you cannot yet build full-fledged Mac apps, learning Swift now will still be highly beneficial. Once you have access to a Mac, you will be able to immediately apply your Swift knowledge towards creating native Mac software.
Disclaim: This article was created with Rix (an AI chatbot). My effort was to ask the questions. I will continue asking questions about Swift, to learn this language properly then I will decide if I'm going to use it or not.
Subscribe to my newsletter
Read articles from Elucian Moise directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
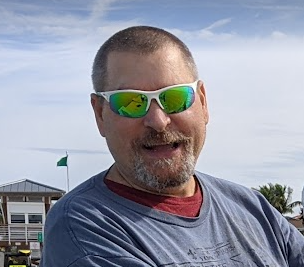
Elucian Moise
Elucian Moise
Software engineer instructor, software developer and community leader. Computer enthusiast and experienced programmer. Born in Romania, living in US.