Dictionary Comprehension in Python
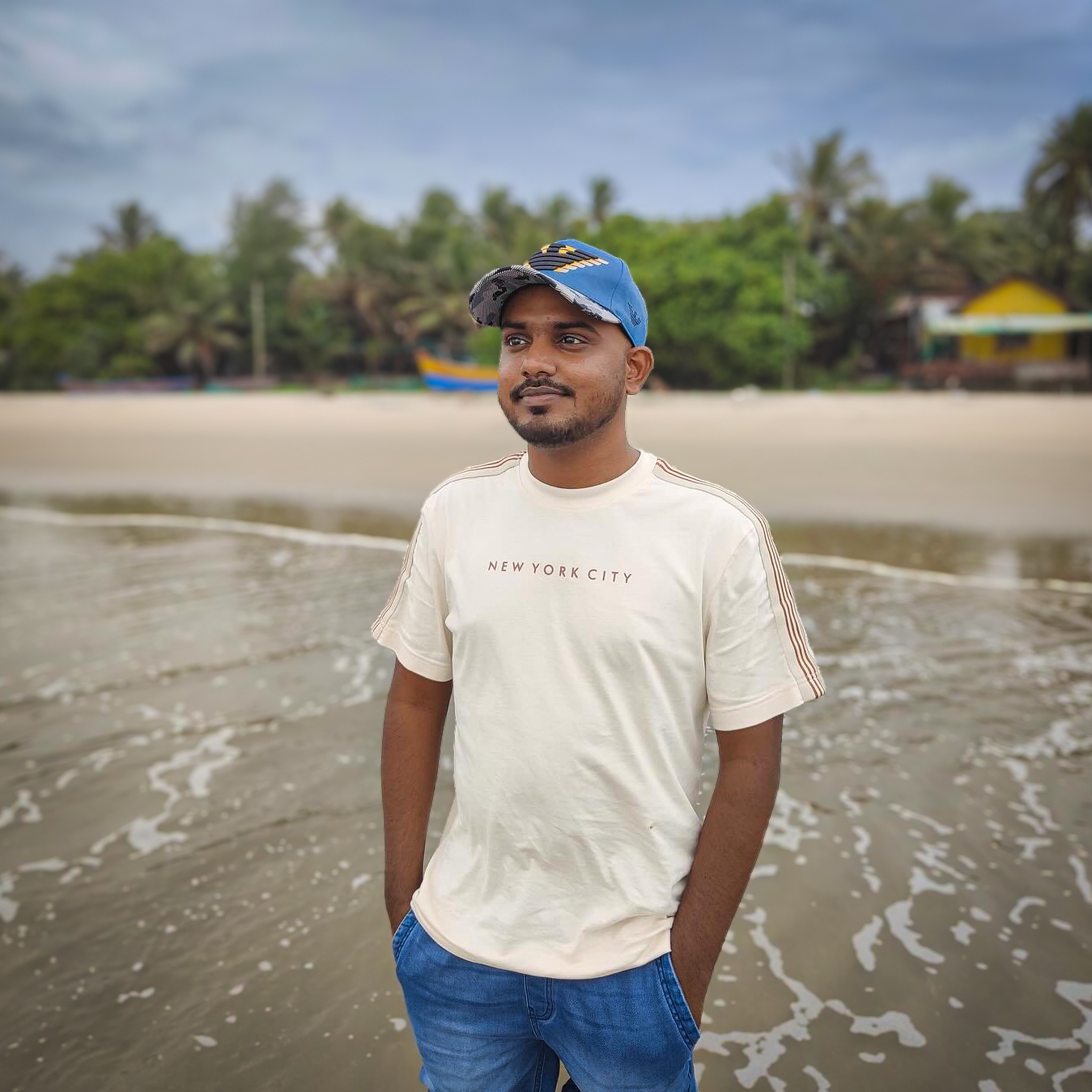
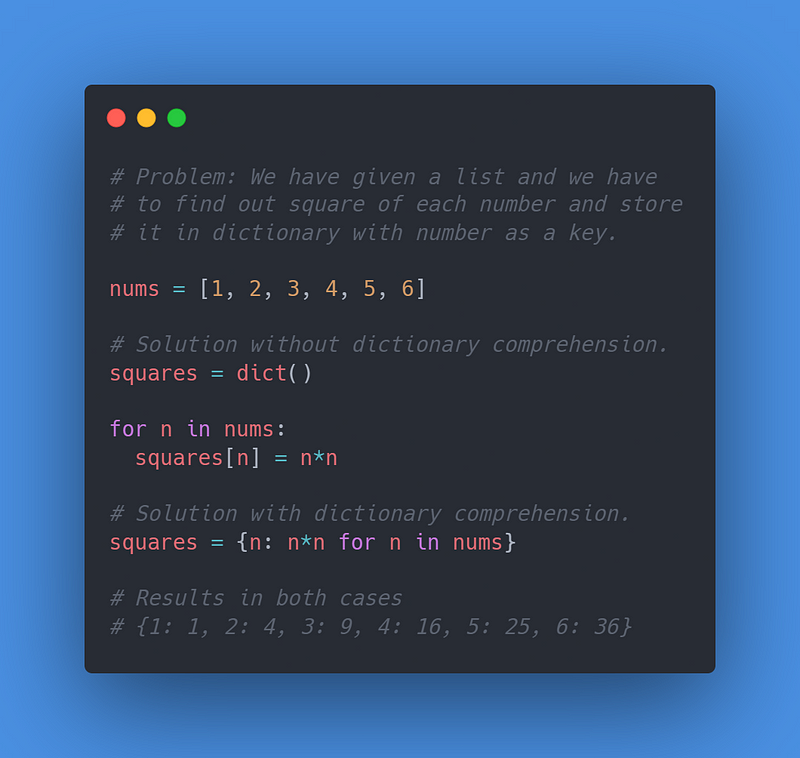
In my previous article, I wrote about List comprehension. So let’s explore dictionary comprehension in this article. Yes, you heard it right. There’s something called dictionary comprehension as well in Python.
Let’s look at a problem first and then we will solve it with Dictionary Comprehension.
Problem: We have given a list and we have to find out the square of each number and store it in the dictionary with the number as a key.
solution with and without dictionary comprehension
In this example, We have solved the problem with two different approaches.
Without dictionary comprehension
With dictionary comprehension.
For the first solution, we declared a blank dictionary, then we have iterated items in the list, and then populated our dictionary. In the second approach, we used the same logic but implemented it in just one line. That’s the advantage of dictionary comprehension.
Syntax:
{key_expression: value_expression for item in iterable if condition}
Syntax for dictionary comprehension
So before diving deep into it, Let’s list out components that we need for dictionary comprehension.
For loop
Condition to check (condition)
Key expression, to get the key from the current item
Value expression, to get value from the current item which is assigned to key in the dictionary
In the above example, We haven’t used the condition part which can be omitted if not required. We used the current item as a key, then an expression that calculates the square of the current item which is used as a value in a dictionary.
Let’s look at a few more examples.
- Make a dictionary from a list and check item is an even number. Use the number as the key and True/False value as a number. Exclude any number which is a multiple of 5.
check even numbers and exclude multiples of 5
In the above example, we have used the if condition where we are checking if a number is not multiple of 5 and including numbers in a dictionary if the condition is true.
2. We have a list of words, we have to make a dictionary with a word as a key and the number of letters in the word as a value. All keys should be in upper case.
In the above example, We are using an expression for key to capitalize all letters in a word as well as for value to calculate the number of letters in a word.
Use an existing dictionary to build a new dictionary
Use an existing dictionary to build a new dictionary
In this example, We are iterating over items of the current dictionary and including items where the value is greater than 3. We are also changing the key to lowercase. This way, just like list comprehension we can use dictionary comprehension to make a smaller dictionary.
One more example is where we are interchanging key, and value in a dictionary.
Interchange key, value in dictionary
There are so many situations where you can take full advantage of dictionary comprehension. If you have any questions or suggestions, please drop a reply.
Follow me on Twitter for more daily Python tips. Let’s code and explore new things in Python 😉.
Subscribe to my newsletter
Read articles from Rohit Patil directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
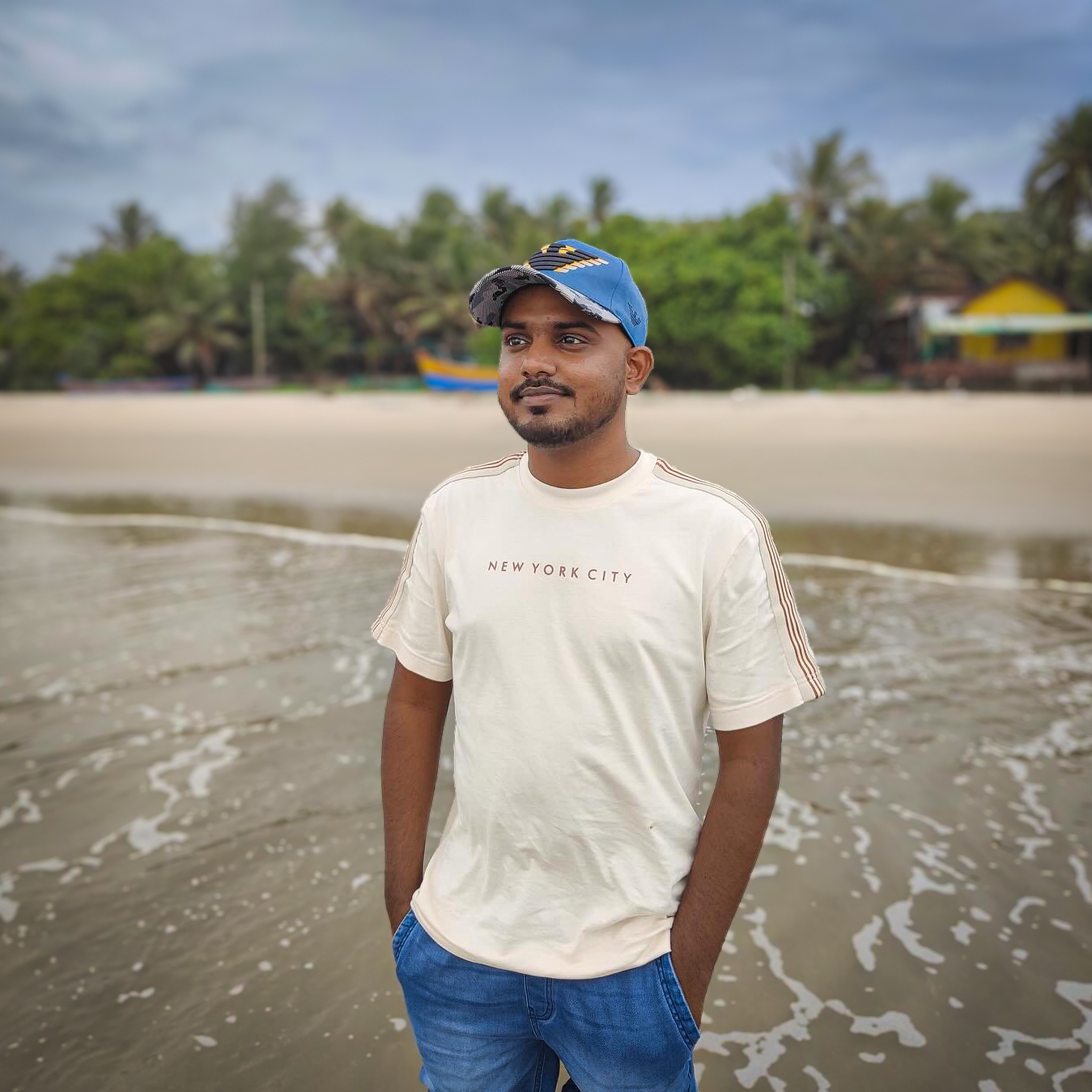
Rohit Patil
Rohit Patil
I'm a software engineer with nearly five years of experience specializing in Python, Golang, DevOps, and Cloud technologies. As a former Tech Lead, I have led diverse teams and worked on eCommerce, Banking, Real Estate, and Machine Learning/AI projects. I excel in collaborating with clients to develop scalable, high-performance solutions.