Understanding Git: A Comprehensive Guide
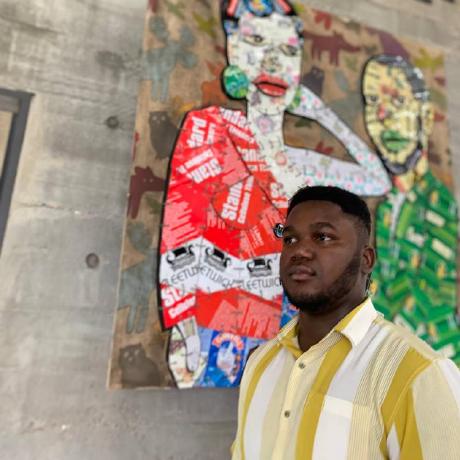
Introduction to Version Control
What is source code management?
Source code management (SCM), also known as version control or revision control, is the practice of tracking and managing changes to a collection of files, typically related to software development. It provides a systematic way to organize, collaborate on, and maintain different versions of source code, documents, and other files within a project. This is incredibly important when developing software because it addresses several critical challenges that arise during the development process.
SCM tools help developers work together efficiently by allowing multiple team members to work on the same codebase simultaneously. Without SCM, coordinating changes from various contributors could quickly become chaotic, leading to confusion and conflicts. By providing a structured framework for collaboration, SCM ensures that everyone's work can be seamlessly integrated without disrupting the overall progress.
Another key aspect of SCM is maintaining a history of changes. Imagine you're writing a book and want to revert to a previous chapter because your recent edits didn't work out. In software development, mistakes can happen, and code can break unexpectedly. SCM acts as a safety net by enabling developers to revert to earlier versions of the code that were known to work properly. This is invaluable for troubleshooting and ensuring that a project doesn't come to a halt due to unforeseen issues.
Additionally, SCM supports the concept of branching and merging. This means that developers can work on new features or fixes in isolation without affecting the main codebase. Once their changes are thoroughly tested and reviewed, they can be merged back into the main codebase. This isolation and controlled integration prevent incomplete or buggy code from negatively impacting the overall project.
In essence, source code management tools offer a structured way to collaborate, maintain a history of changes, work on different features simultaneously, and recover from mistakes—fundamental aspects of software development. By providing these capabilities, SCM contributes to smoother teamwork, better organization, and the overall success of software projects. Let me not bore you with a lot of talks, let's see some key concepts which are more exciting.
Key concepts and benefits of source code management include:
Version Tracking: SCM systems keep a detailed record of changes made to every file, allowing developers to view the history of modifications, including who made each change and when.
Example: You write a program to calculate grades. You start with a basic version, and over time, you add new features like calculating averages and handling different subjects. Version tracking helps you see how your program evolved step by step.
Collaboration: Multiple developers can work on the same codebase simultaneously. Changes made by different developers can be managed, merged, and tracked to avoid conflicts.
Branching and Merging: SCM systems enable developers to create branches, which are separate lines of development. These branches can be worked on independently and later merged back together to incorporate changes.
Example: A team is building a website. One person creates the homepage, another works on the contact page, and someone else handles the products page. SCM ensures that when these pieces are combined, they fit together seamlessly.
Revert and Rollback: If a mistake is made or undesirable changes are introduced, developers can revert to a previous version of the code, effectively "rolling back" to a known state.
Backup and Recovery: With an SCM system, even if data is lost or corrupted, previous versions of the code can be recovered, as long as they were committed to the repository.
Code Reviews and Collaboration: SCM facilitates code reviews, where developers can provide feedback on proposed changes before they are integrated into the main codebase.
Getting started with Git
What is Git?
When I first started using Git, it was quite an interesting journey. Coming from a background with limited familiarity with command-line environments, the initial experience felt both unusual and rather complex. However, with dedicated learning and consistent practice, I was able to gradually overcome the challenges and ultimately become proficient in using Git. It's a testament to the fact that while Git might appear daunting at first, it becomes more accessible and manageable as you invest time and effort into understanding and applying its principles.
Git is a distributed version control system (DVCS) that helps developers track changes to their code over time. Think of it as a digital time machine for your code. It allows you to take snapshots of your project at different stages, preserving the history of every change you make. This is incredibly useful because it enables you to work on new features, fix bugs, and experiment with confidence, knowing that you can always go back to a previous state if needed.
Git works locally on your computer, meaning you have a full copy of the entire project's history on your machine. This allows you to work offline and perform various operations quickly. Additionally, Git's branching and merging capabilities let you create separate lines of development (branches) that can later be combined (merged) back together. This is especially helpful for collaborative projects where multiple people are working on different features at the same time.
What is GitHub?
When I was first introduced to GitHub, it carried a mix of intrigue and intimidation. I realized that this platform held significant importance for anyone aiming to become a developer. At the initial encounter, I was a bit apprehensive about making any accidental mistakes that could potentially disrupt the codebase. There was a slight fear that even a small misstep might result in major disruptions to GitHub's entire code repository, funny right :). However, as time progressed, my perception changed. I gradually gained familiarity with GitHub's user-friendly interface, which stands in stark contrast to Git's command-line nature. Now, I'm able to navigate and manipulate GitHub with ease, having grown comfortable enough to handle it almost instinctively. This shift in perspective demonstrates how learning and adaptation can transform what once appeared daunting into something as familiar as second nature.
GitHub is a web-based platform that provides hosting for Git repositories. It acts as a central hub where developers can store their Git repositories, collaborate on code, and manage their projects. While Git primarily deals with version control locally, GitHub takes it a step further by offering a way to share, collaborate, and contribute to open-source projects with ease.
GitHub provides a user-friendly interface for managing Git repositories, making it accessible to both technical and non-technical users. It offers features like issue tracking, pull requests (a way to propose changes and collaborate on them), and wikis (for project documentation). GitHub's social features also foster a community of developers who can contribute to each other's projects.
What is the difference between Git and GitHub?
The main difference between Git and GitHub lies in their roles and functionalities:
Git is a version control system that focuses on tracking changes to code and managing its history on your local machine. It provides the core functionality for version control, branching, and merging.
GitHub is a web-based platform built around Git. It offers a centralized location to host Git repositories, enabling collaboration, code sharing, and project management. GitHub enhances Git by providing features like issue tracking, pull requests, and collaboration tools.
In summary, Git is the tool that handles version control locally on your computer, while GitHub is an online platform that extends Git's capabilities by providing a collaborative environment for hosting, sharing, and managing Git repositories. Together, they form a powerful ecosystem that revolutionizes how software is developed, shared, and maintained.
Getting Started with Git
Installing Git on Different Platforms
Before you can use Git, you need to install it on your computer. Here's how to do it on various platforms:
Windows
Download the Git installer for Windows from the official Git website: Git for Windows.
Run the installer and follow the installation instructions.
During installation, you can choose the default settings or customize them according to your preferences.
Open a new command prompt or Git Bash window to start using Git.
macOS
Git is usually pre-installed on macOS. To check if Git is installed, open the Terminal and type:
git --version
If Git is not installed, you'll be prompted to install the Xcode Command Line Tools. Follow the prompts to complete the installation.
Linux
On most Linux distributions, Git can be installed using the package manager.
For Debian-based systems (e.g., Ubuntu), open the terminal and run:
sudo apt-get update sudo apt-get install git
For Red Hat-based systems (e.g., Fedora), use:
sudo yum install git
Configuring user information
Configuring User Information
After installing Git, it's important to configure your user information. This information is associated with your commits and helps identify who made the changes. Use the following commands to set your username and email:
git config --global user.name "Your Name" git config --global user.email "youremail@example.com"
Replace "Your Name" with your actual name and "youremail@example.com" with your email address.
You can also configure other settings, such as your preferred text editor and merge tool:
git config --global core.editor "your-text-editor" git config --global merge.tool "your-merge-tool"
Replace "your-text-editor" with the command to open your preferred text editor and "your-merge-tool" with the name of your preferred merge tool.
By configuring user information, you ensure that your commits are attributed to the correct author and provide a consistent experience across your Git projects.
With Git installed and your user information configured, you're ready to start using Git for version control and collaborating on projects.
How to create a repository.
Creating a repository is a fundamental step in using version control, especially with Git. A repository is like a folder that contains your project's files along with the entire history of changes. Here's how you can create a Git repository:
Local Repository Creation:
a. Command Line:
Open your terminal or command prompt and navigate to the directory where you want to create your repository.
cd /path/to/your/project/folder
Initialize a new Git repository using the
git init
command.git init
Your project folder is now a Git repository.
b. Git GUI:
If you prefer a graphical interface, you can use a Git GUI tool like GitKraken, Sourcetree, or GitHub Desktop. Most of these tools have an option to create a new repository. Just open the tool, look for a "New Repository" or "Initialize Repository" button, and follow the prompts.
Remote Repository (Optional - GitHub):
While your local repository contains your project's history, you might also want to host your repository online for collaboration and backup. GitHub is a popular platform for this purpose. Here's how to create a repository on GitHub:
a. Create a GitHub Account:
If you don't already have a GitHub account, sign up for one at github.com.
b. Create a New Repository:
Log in to your GitHub account.
Click the "+" icon in the top right corner and choose "New repository."
Give your repository a name, and optional description, and choose other settings.
Click "Create repository."
c. Connecting Remote and Local Repositories:
After creating a remote repository on GitHub, you need to connect it to your local repository:
On your GitHub repository page, you'll see a set of instructions under the heading "…or push an existing repository from the command line."
Follow those instructions in your terminal to link your local repository to the remote one.
Now you have a local Git repository on your computer, and if you've created a remote repository on GitHub, you also have an online copy for collaboration.
What is a README?
A README is a text file that provides essential information about a project. It is typically placed in the root directory of the project's repository and serves as the first point of contact for anyone exploring the project. READMEs are used to explain what the project does, how to set it up, and how to use it. They're crucial for providing context and instructions to both contributors and users.
A well-written README includes:
A project description and its purpose.
Installation instructions to set up the project.
Usage examples and explanations.
A brief overview of the project's structure.
Contribution guidelines for developers.
Licensing information.
Contact details for support.
How to write good READMEs.
Writing an effective README involves clarity and conciseness. Follow these tips:
Clear and Brief: Keep it concise while covering the essential information. Avoid lengthy paragraphs.
Sections: Use headings for different sections like "Introduction," "Installation," "Usage," "Contributing," "License," etc.
Formatting: Use bullet points, code snippets, and formatting to make it easy to read.
Include Visuals: Screenshots, GIFs, and diagrams can help users understand your project.
Usage Examples: Include practical examples of how to use your project.
Installation: Provide clear steps to set up your project.
Contributing: Explain how others can contribute, if applicable.
How to commit.
Committing in Git involves saving your changes in a local repository. Follow these steps:
Make Changes: Edit files in your project.
Stage Changes: Use
git add
to stage the changes for commit.git add filename
Commit Changes: Use
git commit
to commit the staged changes.git commit -m "Your commit message here"
Repeat: Continue editing, staging, and committing until your changes are complete.
How to write helpful commit messages?
Writing clear and informative commit messages is essential for understanding the purpose of changes. Follow these tips:
Be Specific: Clearly state what you changed or added.
Summarize: Keep the message short (around 50 characters) for the summary.
Use Imperative Mood: Write messages as commands, like "Add feature" or "Fix bug."
Elaborate if Needed: After the summary, you can add a more detailed explanation on a new line.
Reference Issues: If your commit relates to an issue, mention it by adding the issue number.
Example of a good commit message:
Add user authentication feature
This commit adds user authentication using OAuth 2.0 for secure logins.
By writing meaningful commit messages, you make it easier for collaborators to understand your changes and track the project's history effectively.
How to push code.
Pushing code in Git involves sending your committed changes from your local repository to a remote repository, such as one hosted on GitHub. Here's how you can push your code:
Commit Your Changes:
Before you push your code, make sure you've committed your changes using the
git commit
command. This prepares your changes to be sent to the remote repository.git commit -m "Your commit message here"
Check Remote:
Ensure that your local repository is linked to a remote repository. You can verify this using the
git remote -v
command. It should display the URL of the remote repository.Push to Remote:
Use the
git push
command followed by the name of the remote (often "origin") and the branch name you want to push. The common default branch is "main" or "master."git push origin main
If you're pushing to a branch with the same name as your local branch, you can use a shortcut:
git push origin
This pushes the changes from your local branch to the corresponding remote branch.
Authentication:
If it's your first time pushing to the remote repository or if you're not logged in, Git might prompt you to provide your credentials, such as your username and password or a personal access token. This is to ensure you have the necessary permissions to push to the repository.
View Changes Online:
After pushing, you can visit your remote repository on platforms like GitHub to see your changes reflected online. This is useful for collaboration and sharing your work with others.
Remember that pushing code is about sharing your work with others and keeping the remote repository up to date with your local changes. It's a way to contribute to a collaborative project or back up your code in a remote location.
How to pull updates.
Pulling updates in Git involves getting the latest changes from a remote repository into your local repository. This is important to keep your local copy of the code in sync with any changes made by you or others in the project. Here's how you can pull updates:
Check Remote:
Ensure that your local repository is linked to the correct remote repository. You can verify this using the
git remote -v
command. It should display the URL of the remote repository.Fetch Remote Changes:
First, fetch the latest changes from the remote repository using the
git fetch
command. This command retrieves the latest changes from the remote but does not apply them to your working directory.git fetch origin
View Remote Changes:
You can use the
git log
command to see a list of the latest changes on the remote branch. This helps you understand what changes you're about to pull into your local repository.git log origin/main
Merge or Rebase:
After fetching the remote changes, you have two options to integrate them into your local repository:
Merge: Use
git merge
to combine the fetched changes with your local branch. This creates a new merge commit.git merge origin/main
Rebase: Use
git rebase
to move your local changes to the top of the latest fetched changes. This results in a cleaner history.git rebase origin/main
Resolve Conflicts:
If there are conflicting changes between your local branch and the fetched changes, Git will alert you. You'll need to resolve these conflicts manually by editing the affected files, marking conflicts, and then committing the resolved changes.
Push Changes (If Needed):
After pulling and resolving any conflicts, you might need to push your local changes to the remote repository if you've made new commits during the process.
git push origin main
Remember that pulling updates is a way to stay up to date with the latest changes in the project and ensure smooth collaboration with others. It's a crucial step in maintaining the integrity and consistency of the codebase.
How to create a branch?
Creating a branch in Git allows you to work on new features, fixes, or experiments without affecting the main codebase. It's like creating a parallel version of your project to make changes in isolation. Here's how you can create a new branch:
Check Current Branch:
To see which branch you're currently on, use the
git branch
command. The current branch will have an asterisk (*
) next to it.git branch
Create a New Branch:
Use the
git branch
command followed by the name of the new branch you want to create. For example, let's create a branch named "feature/add-login."git branch feature/add-login
Switch to the New Branch:
To start working in the newly created branch, switch to it using the
git checkout
command.git checkout feature/add-login
Alternatively, you can use a single command to create and switch to the branch:
git checkout -b feature/add-login
Work on the Branch:
You're now in the new branch and can start making changes specific to the feature or fix you're working on. Commit your changes as usual.
Push the Branch (Optional):
If you want to collaborate with others or back up your branch on a remote repository, you can push the new branch using the
git push
command. This command creates the branch on the remote repository.git push origin feature/add-login
Creating branches is a fundamental part of Git's workflow, allowing you to work on different tasks simultaneously without interfering with each other. Once your changes in the new branch are ready, you can merge or rebase the branch back into the main branch or another target branch.
How to merge branches?
Merging branches in Git involves combining the changes from one branch into another. This is typically done to incorporate new features, bug fixes, or changes from a separate branch back into the main branch. Here's how you can merge branches:
Switch to Target Branch:
First, make sure you're on the branch into which you want to merge changes. For instance, if you want to merge changes from a feature branch into the main branch, switch to the main branch using the
git checkout
command.git checkout main
Merge Changes:
Use the
git merge
command followed by the name of the branch you want to merge. In this example, we're merging the "feature/add-login" branch into the "main" branch.git merge feature/add-login
Git will try to automatically merge the changes. If there are no conflicts, the changes will be applied, and a new merge commit will be created.
Resolve Conflicts:
If there are conflicting changes between the two branches (changes in the same lines of code), Git will alert you. You'll need to manually resolve these conflicts by editing the affected files, marking conflicts, and then committing the resolved changes.
Commit the Merge:
After resolving any conflicts, commit the merged changes using the
git commit
command.git commit -m "Merge feature/add-login into main"
Push Changes (If Needed):
If you've made new commits during the merge process, you might need to push the merged changes to the remote repository.
git push origin main
Merging branches is a powerful way to integrate changes from separate development paths into a common branch. It's important to handle conflicts carefully and ensure that the merged code remains functional and error-free.
How to work as collaborators on a project?
Working as collaborators on a Git project involves multiple people contributing to the same codebase. Git provides features that facilitate collaboration and help manage changes made by different team members. Here's how you can effectively work as collaborators on a project:
Clone the Repository:
Every collaborator starts by cloning the repository to their local machine using the
git clone
command. This creates a copy of the project's code and history on their computer.git clone <repository_url>
Create and Switch Branch:
Collaborators should create a new branch for their work. This helps keep their changes separate from the main codebase. Use the
git checkout -b
command to create and switch to a new branch.git checkout -b feature/new-feature
Work on the Branch:
Collaborators can now make changes to the code in their branch. Commit changes regularly to capture their progress.
Fetch and Pull Updates:
Regularly fetch and pull updates from the remote repository to stay in sync with changes made by other collaborators. Use
git fetch
to fetch remote changes andgit pull
to incorporate them into your branch.git fetch origin git pull origin main
Resolve Conflicts:
If there are conflicting changes between your branch and the remote updates, resolve them as explained earlier.
Push Changes:
When the collaborator's changes are ready, push them to the remote repository using
git push
.git push origin feature/new-feature
Open Pull Requests (Optional):
For more structured collaboration, collaborators can open pull requests (PRs) if the project is hosted on a platform like GitHub. A pull request is a request to merge your changes into the main codebase. It allows for code review and discussion before the changes are merged.
Review and Merge Pull Requests:
Other collaborators or maintainers of the project can review the changes in the pull request, provide feedback, and eventually merge the changes into the main branch if everything looks good.
Repeat and Communicate:
Continue the cycle of branching, making changes, fetching updates, and collaborating. Effective communication among collaborators helps ensure a smooth workflow.
Collaboration in Git involves keeping code changes organized and structured, while also being responsive to updates from other contributors. Following Git's branching and merging capabilities along with clear communication helps maintain a high-quality codebase, even when multiple people are working on it.
Which files should and which files should not appear in your repo?
Managing the files in your Git repository is essential to maintain a clean and efficient codebase. Here's a breakdown of which files to include and exclude:
Files to Include:
Source Code: Include all the source code files that are required for your project to function. These are the files that you and other contributors will work on.
Configuration Files: Include configuration files necessary for setting up your project. These might include
.env
files, configuration templates, or files required for deployment.Documentation: Include documentation files that help users and contributors understand your project. This could be in the form of READMEs, documentation files, and guides.
Tests: Include test files that ensure your code works correctly. These files are essential for maintaining code quality and catching bugs.
Assets: Include static assets like images, stylesheets, and fonts that are part of your project.
Dependency Files: Include files like
requirements.txt
,package.json
, orGemfile.lock
that lists project dependencies. This helps others recreate your development environment accurately.
Files to Exclude:
Compiled Output: Exclude compiled binaries, executables, or other build artifacts. These files can be generated from source code and don't need to be stored in the repository.
Temporary Files: Exclude temporary files, cache files, and backup files. These files can clutter your repository and don't contribute to the project's functionality.
User-Specific Files: Exclude user-specific configuration files or personal settings that do not apply to other contributors.
IDE/Editor-Specific Files: Exclude IDE-specific or editor-specific files and folders, like
.idea
or.vscode
. These can cause conflicts and are not relevant to other collaborators.Sensitive Information: Exclude files containing sensitive information such as passwords, API keys, and private credentials. Use environment variables or configuration files for such data.
Logs: Exclude log files generated by your application. Logs can be large and are typically generated during runtime.
To manage which files to include or exclude, you can create a .gitignore
file in your repository's root directory. This file lists patterns of files and directories that Git should ignore when tracking changes. Using a .gitignore
file ensures that unwanted or sensitive files are not accidentally included in your commits and pushed to the repository.
Troubleshooting and Tips
Recovering lost commits or branches
Accidentally lost some commits or branches? Don't panic! Git's got your back. You can use the
git reflog
command to view the history of your recent actions and find the commit or branch that seemingly disappeared. Once you've located it, you can easily recover it using various Git commands.Understanding Git's internal structure
Ever wondered what's cooking inside Git's magical pot? Git's internal structure is like a well-organized closet of your coding history. Dive into concepts like objects, blobs, trees, and commits. Understanding how Git stores your project's snapshots helps demystify the Git wizardry.
Useful tips for a smoother Git experience
Branch Naming: Be descriptive in your branch names. "feature/user-authentication" is much clearer than "new-feature."
Interactive Add: Use
git add -i
orgit add -p
to interactively stage changes. It's like creating a custom buffet of commits!Alias Magic: Save time by creating custom Git aliases for frequently used commands. "git st" instead of "git status" is like a secret handshake.
Visual Aids: Leverage GUI tools like Sourcetree or GitKraken for a more visual approach to Git. Sometimes a picture is worth a thousand commits.
Commit Early, Commit Often: Embrace small, frequent commits instead of massive code dumps. It's like saving your progress in a game – you won't lose as much if things go awry.
Branch Protection: On collaborative projects, protect main branches to prevent accidental pushes. Trust me, you don't want your masterpiece turned into spaghetti code.
Conclusion
Congratulations, you've embarked on a journey through the Git wonderland! From understanding the essence of version control to mastering the art of collaboration and troubleshooting, you've gained insights into an essential tool for modern development. Armed with this knowledge, you're ready to confidently navigate the coding seas, equipped with Git's powerful capabilities and a bag of handy tricks. Happy coding! 🚀
Subscribe to my newsletter
Read articles from Tetteh Henry directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
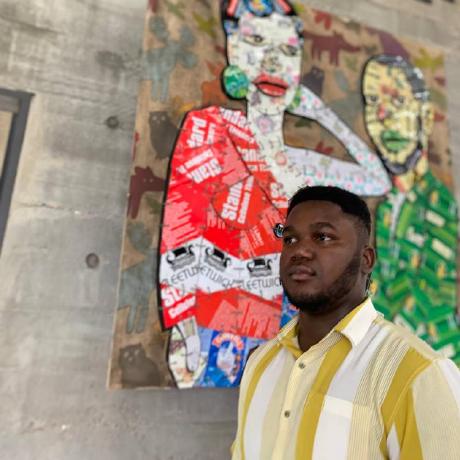