Introduction to python variables
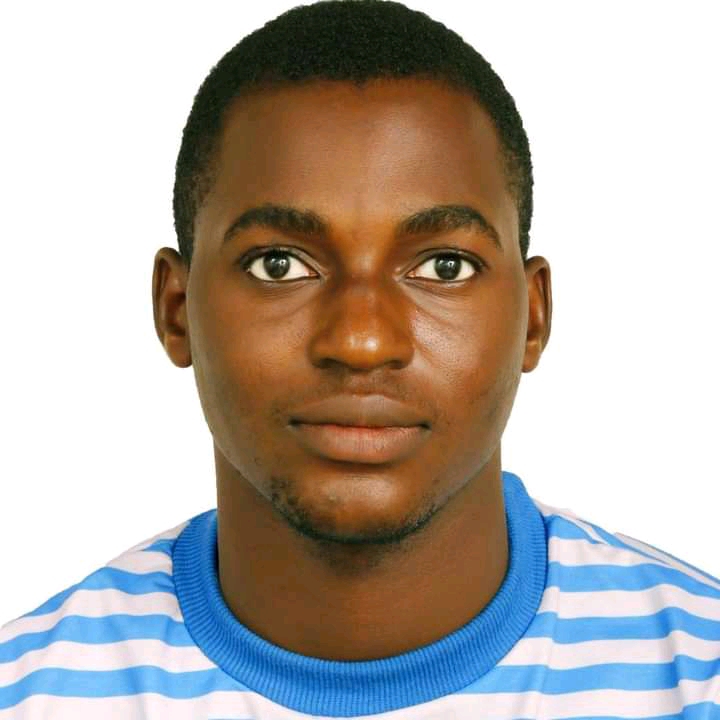
Table of contents
- 1. Introduction to Python Variables
- 2. Naming Conventions for Variables in Python
- 3. Difference between Local and Global Variables in Python
- 4. Mutability and Immutability in Python Variables
- 5. Python Built-in Data Types and Variables
- 6. Variable Assignment and Initialization in Python
- 7. Scope of Variables in Python
- 8. Variables as Containers for Storing Data in Python
- 9. Understanding Data Types and Type Conversion in Python Variables
- 10. Memory Allocation and Management for Python Variables
- 11. Tips for Effective Variable Usage in Python Programming
- 12. Best Practices for Naming and Using Variables in Python
- 13. Assigning Multiple Values to Multiple Variables in Python
- 14. Augmented Assignment Operators for Variables in Python
- 15. Variable Unpacking in Python
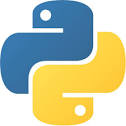
Objectives: Below is what you should expect from this article.
1. Introduction to Python variables: Understand the concept of variables in Python and their importance in programming.
2. Naming conventions for variables in Python: Learn the recommended practices for naming variables in Python to enhance code readability.
3. Difference between local and global variables in Python: Understand the distinctions between local and global variables and how they are used within the context of Python.
4. Mutability and immutability in Python variables: Explore the concepts of mutability and immutability in Python variables and their implications in programming.
5. Python built-in data types and variables: Discover the built-in data types in Python and how they are utilized when working with variables.
6. Variable assignment and initialization in Python: Learn different techniques for assigning and initializing variables in Python.
7. Scope of variables in Python: Understand the scope of variables and how their visibility and accessibility are determined in Python.
8. Variables as containers for storing data in Python: Explore how variables act as containers for storing and manipulating data in Python.
9. Understanding data types and type conversion in Python variables: Gain knowledge about Python's data types, their characteristics, and techniques for converting between them.
10. Memory allocation and management for Python variables: Learn about the memory allocation and management mechanisms related to variables in Python.
11. Tips for effective variable usage in Python programming: Discover practical tips and techniques for using variables efficiently and effectively in Python programming.
12. Best practices for naming and using variables in Python: Get insights into the best practices for naming variables and utilizing them appropriately in Python code.
13. Assigning multiple values to multiple variables in Python: Learn how to assign multiple values to multiple variables simultaneously in Python.
14. Augmented assignment operators for variables in Python: Explore the augmented assignment operators in Python and how they make variable manipulation more concise.
15. Variable unpacking in Python: Understand the concept of variable unpacking and how it can be utilized to assign values from sequences to multiple variables in Python.
Table of contents
1. Introduction to Python variables
2. Naming conventions for variables in Python
3. Difference between local and global variables in Python
4. Mutability and immutability in Python variables
5. Python built-in data types and variables
6. Variable assignment and initialization in Python
7. Scope of variables in Python
8. Variables as containers for storing data in Python
9. Understanding data types and type conversion in Python variables
10. Memory allocation and management for Python variables
11. Tips for effective variable usage in Python programming
12. Best practices for naming and using variables in Python
13. Assigning multiple values to multiple variables in Python
14. Augmented assignment operators for variables in Python
15. Variable unpacking in Python
1. Introduction to Python Variables
Variables are one of the fundamental concepts in programming, and they play a crucial role in Python as well. In Python, a variable is simply a name that represents a value. It acts as a container to store data that can be referenced and manipulated throughout a program.
2. Naming Conventions for Variables in Python
While naming variables in Python, it is essential to follow certain conventions to improve code readability and maintainability. Variable names should be descriptive, indicating the purpose of the data they store. They can consist of letters, numbers, and underscores, but must start with a letter or underscore. Python is case-sensitive, so variables "myVariable" and "myvariable" would be considered separate entities.
3. Difference between Local and Global Variables in Python
In Python, variables can be classified into two types: local and global variables. Local variables are defined within a specific scope, such as inside a function, and can only be accessed within that scope. On the other hand, global variables are declared outside functions, making them accessible from anywhere in the program. It's important to note that modifying a global variable within a function requires the 'global' keyword to indicate the intention.
4. Mutability and Immutability in Python Variables
In Python, variables can be either mutable or immutable. Mutable variables can be modified after creation, while immutable variables cannot be changed once assigned. Examples of mutable data types in Python include lists and dictionaries, while integers and strings are immutable. The distinction between mutable and immutable variables is crucial when it comes to understanding how data is stored and manipulated in Python.
5. Python Built-in Data Types and Variables
Python provides several built-in data types, each with its purpose and functionality. These include integers, floating-point numbers, strings, booleans, lists, tuples, sets, and dictionaries. Variables can be assigned to any of these data types based on the requirements of the program.
6. Variable Assignment and Initialization in Python
In Python, variables are assigned using the "="
operator. Initialization refers to the process of providing an initial value to a variable.
For example,
"age = 25"
assigns the value 25 to the variable 'age'
. Variables can also be reassigned to different values during the execution of a program.
7. Scope of Variables in Python
The scope of a variable in Python refers to the region of a program where the variable is accessible. Python supports four levels of variable scope: local, enclosing (for nested functions), global, and built-in. Local variables can only be accessed within the function they are defined in, while global variables are accessible throughout the program.
8. Variables as Containers for Storing Data in Python
Variables serve as containers for storing and manipulating data in Python. They allow programmers to assign values to names, making the code more readable and manageable. By using variables, data can be reused, modified, and easily referenced throughout the program, improving overall code efficiency.
9. Understanding Data Types and Type Conversion in Python Variables
Python is a dynamically typed language, which means that variables can hold different types of data at different points in the program. Understanding the various data types and their conversion methods is vital in Python programming. Python provides built-in functions like 'int()', 'str()', and 'float()' to convert variables from one type to another.
10. Memory Allocation and Management for Python Variables
Python handles memory allocation and management automatically using a process called garbage collection. It frees up memory occupied by variables that are no longer in use, reducing the risk of memory leaks. The Python interpreter takes care of allocating memory when variables are created and releasing it when they are no longer referenced.
11. Tips for Effective Variable Usage in Python Programming
To improve the efficiency and readability of code, it is essential to follow some best practices when using variables in Python. These include using descriptive and meaningful names, avoiding using reserved keywords as variable names, and ensuring that variables are properly initialized before use.
12. Best Practices for Naming and Using Variables in Python
Following standardized naming conventions can greatly enhance the readability and understandability of code. Variables should have meaningful and descriptive names, using lowercase letters and underscore to separate words (e.g., 'user_name' instead of 'usrnm'). It is also recommended to avoid using single-letter variable names unless used in short loops.
13. Assigning Multiple Values to Multiple Variables in Python
Python allows the simultaneous assignment of multiple values to multiple variables in a single line. This technique is called tuple unpacking.
For example,
name, age, city = 'John', 25, 'New York
assigns the value 'John' to the variable 'name', 25 to 'age', and 'New York' to 'city'. This feature provides a concise way of assigning multiple values at once.
14. Augmented Assignment Operators for Variables in Python
Python provides augmented assignment operators to perform operations on variables and assign the result back to the same variable. For instance, "+="
adds a value to a variable and assigns the result to the same variable. Similarly, "-="
, "*="
, "/="
, and "%="
are used for subtraction, multiplication, division, and modulo operations, respectively.
15. Variable Unpacking in Python
Variable unpacking in Python refers to extracting values from iterable objects like tuples, lists, and dictionaries, and assigning them to individual variables. This process is beneficial when dealing with functions that return multiple values. For example, "x, y = (1, 2)" assigns 1 to 'x' and 2 to 'y'. Variable unpacking can simplify code and make it more concise.
In conclusion, variables are essential components of Python programming, allowing the storage and manipulation of data. By understanding their properties, best practices, and various techniques discussed above, programmers can leverage variables effectively to create efficient and maintainable code.
Leave your question in the comment section and don't just read but practice makes perfect.
Subscribe to my newsletter
Read articles from Lateef Ahmad Adisa directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
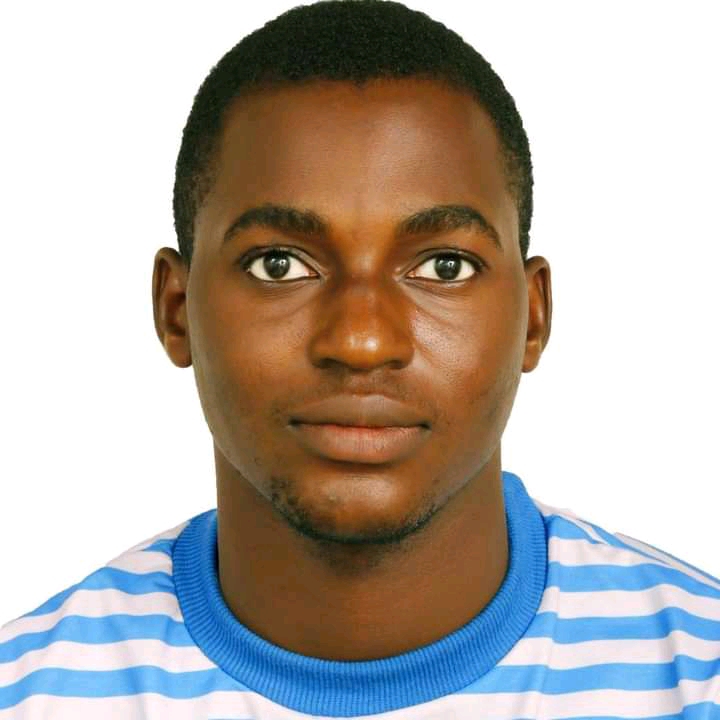
Lateef Ahmad Adisa
Lateef Ahmad Adisa
Title: Lateef Ahmad Adisa: A Full Stack Developer, Python Enthusiast, and Passionate Technical Writer Introduction: Meet Lateef Ahmad Adisa, a highly skilled and dedicated professional in the field of software development, with a focus on full-stack development, Python programming, and technical writing. With an unwavering passion for creating cutting-edge solutions and empowering the developer community, Lateef has become an invaluable asset for companies and individuals alike. Background and Experience: Lateef Ahmad Adisa has honed his skills through years of practical experience and continuous learning. Holding a strong educational background in Computer Science, Lateef's journey began as a fresh graduate, eager to make his mark in the world of technology. After completing his studies, he quickly embarked on a professional career, diving deep into the world of software development. Full Stack Development: Lateef's expertise lies in full-stack development, making him capable of handling both front-end and back-end development with equal proficiency. His extensive knowledge of modern frameworks, such as Angular and React, enables him to create intuitive user interfaces that seamlessly interact with powerful server-side architectures. Python Development: One of Lateef's main areas of specialization is Python development. With Python’s versatility and efficiency, Lateef has embraced the language as his go-to tool for solving complex problems. His skills range from creating web applications using Django and Flask, to utilizing Python in data science projects, scripting, and automation tasks. Technical Writing: In addition to his technical expertise, Lateef is an accomplished technical writer, sharing his knowledge and insights through various online platforms. With a unique ability to simplify complex concepts, Lateef's articles, tutorials, and documentation have been praised by numerous readers for their clarity and practicality. By contributing to the developer community, Lateef aims to inspire and empower others on their learning journeys. Contributions to the Developer Community: Lateef holds a strong belief in the power of open-source software and the importance of giving back. He actively contributes to GitHub repositories, where he shares his own projects and contributes to existing ones. His insightful blog posts and tutorials on platforms like Hashnode have garnered a loyal following, providing aspiring developers with guidance, solutions, and a sense of belonging to a thriving developer community. Passion and Professionalism: Beyond his technical prowess, Lateef Ahmad Adisa is a dedicated professional known for his strong work ethic and commitment to excellence. His ability to blend creativity with precision ensures the delivery of elegant solutions, tailored to meet the unique demands of every project. Lateef's enthusiasm for learning and his dedication to staying up-to-date with the latest industry trends ensures that he consistently delivers high-quality outcomes. Conclusion: Lateef Ahmad Adisa's profound knowledge and expertise in full-stack development, Python programming, and technical writing make him an exceptional asset in today's rapidly evolving tech landscape. Through his numerous contributions to the developer community and his commitment to personal growth, Lateef aims to inspire fellow developers, fuel innovation, and push the boundaries of what is possible in the world of software development.