A Beginner's Guide to Quantum Differential Programming with PennyLane AI
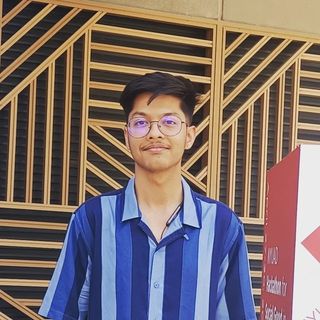
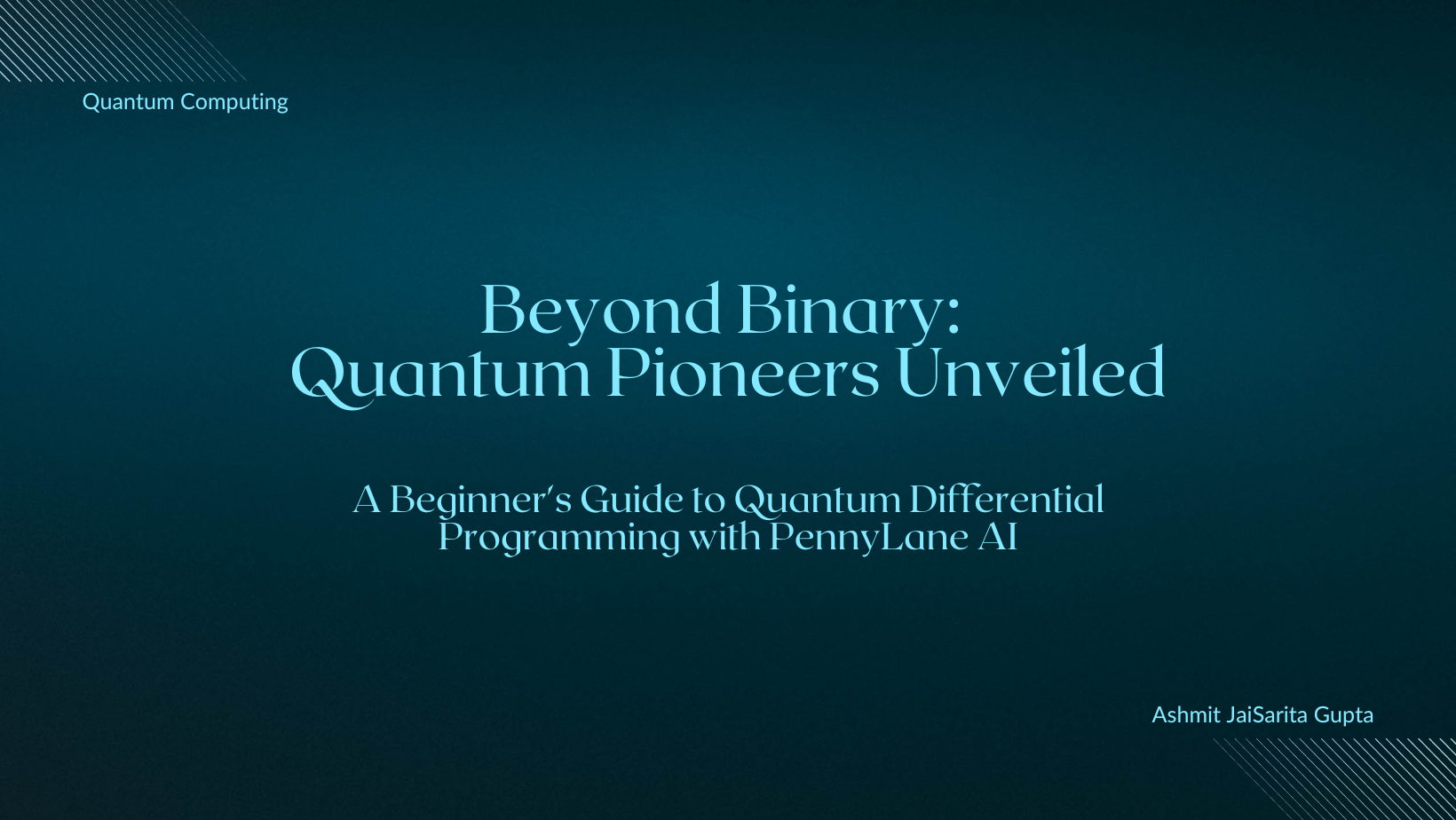
Quantum computing has gained significant attention in recent years due to its potential to solve complex problems more efficiently than classical computers. In the last blog, I gave an Introduction to Pennylane AI and Quantum Differential Programming. This blog is part of a series of blogs to understand Quantum Differential Programming: Beyond Binary: Quantum Pioneers Unveiled. In this blog, we will delve deep into the concept of Quantum differential programming. Quantum differential programming is a fascinating subfield that combines quantum computing with the principles of differentiation, allowing us to optimize quantum algorithms and solve real-world problems. In this comprehensive guide, we will explore quantum differential programming using PennyLane AI, a versatile quantum machine learning library. We'll start with the basics of differentiation in programming and gradually delve into the automatic differentiation of quantum computations.
Table of Contents:
Introduction to Differentiation in Programming
Symbolic Differentiation
Numerical Differentiation
Automatic Differentiation
Examples in Python and PyTorch
Automatic Differentiation of Quantum Computations
Mathematical Foundations
PennyLane: Quantum Machine Learning Library
Quantum Gradients and Parameter Shift Rule
Quantum Gradient Descent
Quantum Differentiation Example with PennyLane
Introduction to Differentiation in Programming
In all the examples below we will be differentiating f(x)=x^2+2x+1 which can also be easily calculated manually as:
$$\frac{df}{dy} = \frac{d}{dy}x^2 + \frac{d}{dy} 2x + \frac{d}{dy} (1)$$
$$\frac{df}{dy}=2x+2$$
Symbolic Differentiation
Symbolic differentiation involves computing derivatives symbolically, which means expressing the derivative as a formula or an expression. This method is precise but can be computationally expensive for complex functions.
Example in Python using SymPy:
import sympy as sp
x = sp.Symbol('x')
f = x**2 + 2*x + 1
f_prime = sp.diff(f, x)
print(f_prime) # Output: 2*x + 2
Numerical Differentiation
Numerical differentiation approximates derivatives by calculating the finite difference between function values at nearby points. It is less accurate than symbolic differentiation but computationally faster. The differentiation of any function can be easily calculated using the finite difference formula:
$$f'(x) \simeq \frac{f(x + h) - f(x)}{h}$$
Example in Python using NumPy:
import numpy as np
def f(x):
return x**2 + 2*x + 1
x = 2.0
h = 1e-5
f_prime_approx = (f(x + h) - f(x)) / h
print(f_prime_approx) # Output: 6.00001000027
Automatic Differentiation
Automatic differentiation is a middle-ground approach that combines the accuracy of symbolic differentiation with the computational efficiency of numerical differentiation. It automatically computes derivatives by applying the chain rule, step by step. You can study Chain Rules from this Lesson on Chain Rules by Khan Academy or any source on the internet.
Example in Python using PyTorch:
import torch
x = torch.tensor(2.0, requires_grad=True)
f = x**2 + 2*x + 1
f.backward()
f_prime = x.grad
print(f_prime.item()) # Output: 6.0
Automatic Differentiation of Quantum Computations
Mathematical Foundations
Before we dive into quantum differentiation, let's understand the basic mathematical concepts involved. In quantum computing, we represent computations as quantum circuits, which consist of quantum gates and qubits. To compute derivatives of quantum circuits, we rely on the principles of matrix calculus.
Quantum Circuits and Parameters
In a quantum circuit, we have parameters that represent angles or values associated with quantum gates. These parameters can be adjusted to optimize the circuit's behavior for a specific task, such as solving a quantum chemistry problem or training a quantum machine learning model. Let's denote these parameters collectively as θ, and our quantum circuit as U(θ).
Quantum Expectation Values
Quantum algorithms often involve calculating expectation values of quantum observables, which are represented as Hermitian operators. Let's denote an observable as O. The expectation value of O in the quantum state produced by U(θ) is given by:
$$\langle O \rangle = \langle \psi(\boldsymbol{\theta}) | O | \psi(\boldsymbol{\theta}) \rangle$$
Where ∣ψ(θ)⟩ represents the quantum state generated by the circuit U(θ).
Parameter Shift Rule
The parameter shift rule is a fundamental concept in quantum automatic differentiation. It allows us to compute the gradient of an expectation value with respect to a parameter. Let's assume we have a parameter θi in our circuit:
- We first calculate the expectation value of the observable O for the original parameter θi:
$$E_1 = \langle O \rangle(\boldsymbol{\theta})$$
- Next, we compute the expectation value for the circuit with a slight shift in the parameter θi:
$$E_2 = \langle O \rangle(\boldsymbol{\theta} + \Delta \theta_i)$$
- The derivative of the expectation value with respect to θi can be approximated using the finite difference:
$$\frac{dE}{d\theta_i} \approx \frac{E_2 - E_1}{\Delta \theta_i}$$
The parameter shift rule effectively quantifies how a small change in a parameter affects the expectation value of the quantum observable. This is essential for optimizing quantum circuits during training and variational quantum algorithms.
Quantum Gradients
The gradient of an expectation value with respect to all parameters θ is a vector of partial derivatives:
$$\boldsymbol{\nabla}\langle O \rangle = \left[\frac{dE}{d\theta_1}, \frac{dE}{d\theta_2}, \ldots, \frac{dE}{d\theta_n}\right]$$
This gradient vector provides valuable information about how each parameter influences the expectation value. By adjusting the parameters in the direction that minimizes or maximizes the cost function, we can optimize quantum circuits for specific tasks.
PennyLane: Quantum Machine Learning Library
PennyLane is an open-source Python library that integrates seamlessly with popular quantum computing frameworks like Qiskit and Cirq. PennyLane provides a high-level interface for building and optimizing quantum circuits, making it an excellent choice for quantum differential programming.
Quantum Gradients and Parameter Shift Rule
To compute gradients of quantum circuits, we use the parameter shift rule. This rule allows us to calculate the gradient of an expectation value with respect to a parameter by applying two slightly different quantum circuits and taking the difference in their measured values.
Quantum Gradient Descent
With quantum gradients in hand, we can use optimization algorithms like gradient descent to find optimal parameters for quantum circuits. This is crucial for solving real-world problems efficiently using quantum computers.
Quantum Differentiation Example with PennyLane
Let's put our knowledge into practice with a simple example in PennyLane. We'll differentiate a quantum circuit representing a variational quantum eigensolver (VQE) for a quantum chemistry problem. You can study VQE in detail on this amazing blog by Michał Stęchły on Musty Thoughts.
import pennylane as qml
import numpy as np
# Define a quantum device
dev = qml.device("default.qubit", wires=2)
# Create a quantum circuit
@qml.qnode(dev)
def circuit(params):
qml.RX(params[0], wires=0)
qml.RY(params[1], wires=1)
qml.CNOT(wires=[0, 1])
return qml.expval(qml.PauliZ(0))
# Initialize parameters
params = np.array([0.1, 0.2], requires_grad=True)
# Define the cost function
def cost(params):
return circuit(params)
# Compute the gradient
grad_fn = qml.grad(cost)
gradient = grad_fn(params)
print(gradient)
In this example, we've defined a quantum circuit, initialized some parameters, and computed the gradient of the circuit's expectation value with respect to those parameters using PennyLane's automatic differentiation capabilities. This example demonstrates how PennyLane simplifies the process of differentiating quantum circuits, making it accessible for quantum machine learning tasks and quantum optimization problems.
In summary, the automatic differentiation of quantum computations using PennyLane empowers researchers and developers to explore the full potential of quantum computing by efficiently optimizing quantum circuits for various applications. It combines the power of quantum computing with the convenience of automatic differentiation, opening up new possibilities in the world of quantum differential programming.
Conclusion
Quantum differential programming is an exciting field that merges quantum computing with differentiation techniques. It enables us to optimize quantum algorithms for various applications, from quantum chemistry to machine learning. With the help of PennyLane and the principles of automatic differentiation, even beginners can dive into this cutting-edge field and start exploring the limitless possibilities of quantum computing.
Know the Author
I am Ashmit JaiSarita Gupta, an engineering physics undergraduate at the National Institute of Technology Hamirpur. I am passionate about Quantum Computing, Machine Learning, UI/UX, and Web Development. About two years ago, when I first discovered the field of Web Development and Quantum Computing, it totally amazed me and I have been dedicating my education to them ever since. Over the past two years, I have dedicated a considerable amount of time and effort to learning and developing skills in these fields by taking various online courses, reading different articles, making several projects, and being involved in various research internships and mentorship programs. Fast forward to today, I am currently researching QUBO Relaxation Parameter Optimisation using a Learning Surrogate Solver (QROSS). Visit my portfolio website to learn more about me, my previous projects, and the places I have worked. Feel free to connect with me on Twitter, LinkedIn, or GitHub.
Subscribe to my newsletter
Read articles from Ashmit JaiSarita Gupta directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
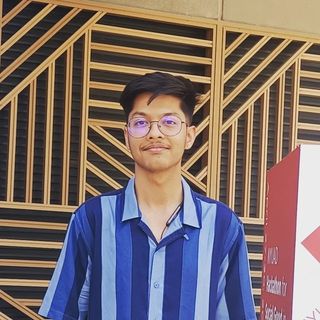
Ashmit JaiSarita Gupta
Ashmit JaiSarita Gupta
I am an engineering physics undergraduate passionate about Quantum Computing, Machine Learning, UI/UX, and Web Development. About two years ago, when I first discovered the field of Web Development and Quantum Computing, it totally amazed me and I have been dedicating my education to them ever since. Over the past two years, I have dedicated a considerable amount of time and effort to learning and developing skills in these fields by taking various online courses, reading different articles, making several projects, and being involved in various research internships and mentorship programs. Fast forward to today, I am currently working on a modern streaming platform and researching QUBO Relaxation Parameter Optimisation using Learning Surrogate Solver (QROSS).