Demystifying Functional Interfaces in Java: A Comprehensive Guide
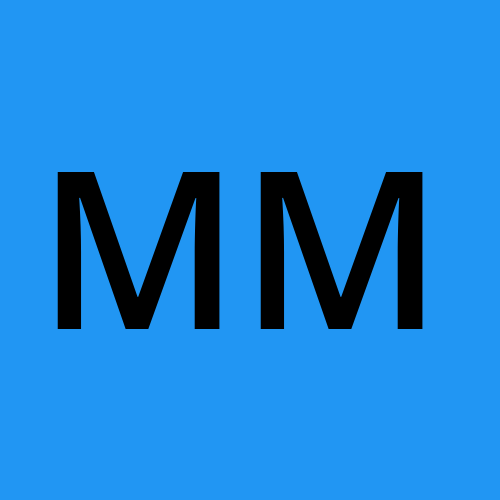
Welcome back to our journey through the world of Java, where we continue our exploration of lambda expressions and functional interfaces. In our previous blog(here), we delved into the fascinating realm of lambda expressions and learned how they can simplify our code and make it more expressive. Today, we'll take a closer look at functional interfaces, which are essential in unleashing the power of lambdas. Whether you're a seasoned Java developer or just starting your programming journey, this guide is for you!
Types of Functional Interfaces
Functional interfaces in Java come in various flavours, each designed to serve a specific purpose. Let's explore four fundamental types:
1. Function
The Function
type functional interface is your go-to choice when you need to process input and produce a result. It takes a single argument, performs some operations, and returns a value. This type of functional interface is particularly handy for tasks like searching for a value in a map based on a user-provided key.
Syntax:
@FunctionalInterface
public interface Function<T, R> {
R apply(T t);
}
@FunctionalInterface
annotation ensures that the interface is functional.T
represents the input type.R
is the return type of the abstract methodapply()
.
2. Predicate
When you need to determine if a condition is true or false, the Predicate
functional interface comes to the rescue. It takes a single argument and returns a boolean value, making it ideal for filtering values from collections. In essence, Predicate
is a specialization of Function
tailored for boolean results.
Syntax:
public interface Predicate<T> {
boolean test(T t);
}
The abstract method
test()
returns a boolean value.It takes a parameter of type
T
.
3. Consumer
The Consumer
interface is all about receiving input and performing an action without returning any value. In Java 8 and beyond, this interface is part of the java.util.function
package, introduced to embrace functional programming.
Functions in Consumer
Interface:
accept()
: This method takes one parameter and does not return any value.
Syntax:
void accept(T t)
andThen()
: This default method allows you to chain twoConsumer
operations together.
Syntax:
default Consumer<T> andThen(Consumer<? super T> after)
4. Supplier
The Supplier
interface is your go-to choice when you need to obtain a value without supplying any arguments. It produces a result of type T
and is particularly useful when you don't need to provide input but still require an output.
Functions in Supplier
Interface:
get()
: This method doesn't take any arguments but produces a value of typeT
.
Syntax:
T get()
Now that we've uncovered the four essential types of functional interfaces in Java, you're well-equipped to harness the power of lambda expressions and simplify your code. Whether you're filtering collections, processing data, or obtaining values, these functional interfaces are your trusted allies on your programming journey.
So, whether you're a beginner eager to dive into the world of functional programming or an experienced developer looking to sharpen your skills, remember that mastering functional interfaces in Java opens up a world of possibilities and cleaner, more expressive code.
Happy learning!
Subscribe to my newsletter
Read articles from Minal Madhur directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
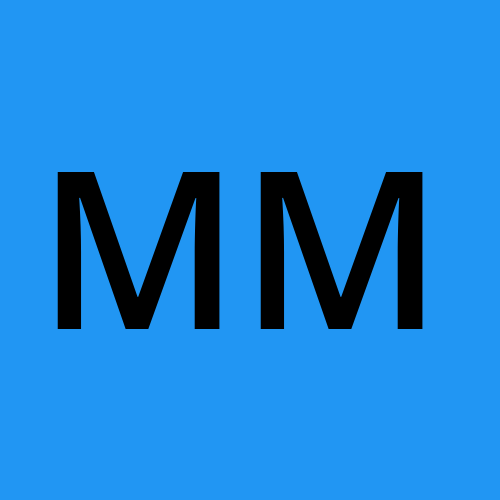