How to Get Human-Readable Variable Type Names in C++
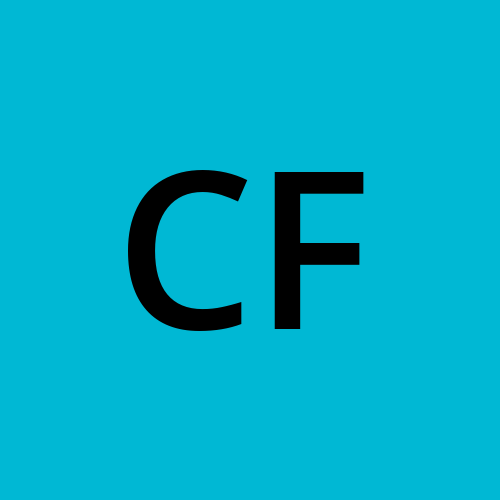
Using the abi::__cxa_demangle
Function for Type Demangling in C++
Introduction
When working with C++ code, especially in debugging or reflection scenarios, it's often useful to know the human-readable name of a type. The typeid
operator can provide this information, but the output is often mangled to a non-readable format that is hard to understand. This article focuses on how to use the abi::__cxa_demangle
function to convert these mangled type names to a human-readable form.
Prerequisites
- A C++ compiler (GCC or compatible is required for
abi::__cxa_demangle
) - Basic knowledge of C++ syntax and type system
What is Name Mangling?
Name mangling is a technique used by C++ compilers to generate unique names for variables, functions, and types that may not be unique in the original source code. This is necessary because C++ supports function overloading and other features that may introduce naming collisions.
The typeid().name()
Pitfall
Using typeid().name()
can provide you with a type's name, but the string returned is a mangled, compiler-dependent version of the name. For example:
#include <iostream>
#include <typeinfo>
int main() {
auto x = 42;
std::cout << typeid(x).name() << std::endl; // Output: "i" for GCC
}
Demangling with abi::__cxa_demangle
The abi::__cxa_demangle
function is provided by the GCC C++ ABI library and can be used to demangle mangled names into their human-readable string representations.
How to Use
Here's an example demonstrating how to use it:
#include <iostream>
#include <typeinfo>
#include <cxxabi.h>
std::string demangle(const char* name) {
int status = -1;
char* demangled_name = abi::__cxa_demangle(name, nullptr, nullptr, &status);
std::string result((status == 0) ? demangled_name : name);
free(demangled_name);
return result;
}
int main() {
auto x = 42;
std::cout << "Type of x: " << demangle(typeid(x).name()) << std::endl;
}
Steps to Integrate in Your Code
Include Headers: Add
#include <typeinfo>
fortypeid
and#include <cxxabi.h>
forabi::__cxa_demangle
.Define the Demangling Function: Create a function like
demangle()
as shown in the example above.Use the Function: Call
demangle(typeid(variable).name())
wherever you need the human-readable type name.Linking: Make sure you link against the C++ ABI library. With GCC, this is usually automatic. If not, you may need to add
-lstdc++
to your linker flags.
Conclusion
Name demangling is an important task when dealing with type information at runtime in C++. The abi::__cxa_demangle
function provides an effective way to get human-readable type names, improving code readability and aiding in debugging. Note that this function is specific to GCC and compatible compilers.
Subscribe to my newsletter
Read articles from Carlo Fornari directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
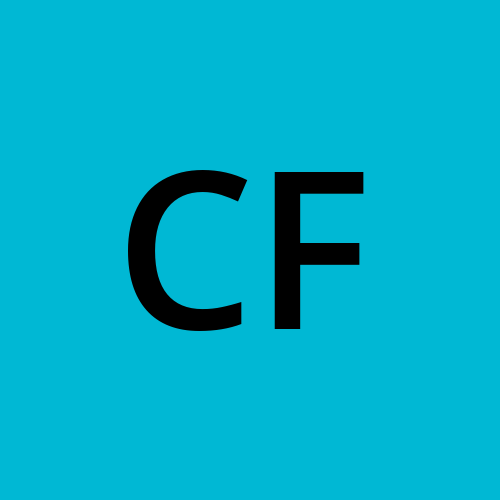