Destructuring Arrays in React
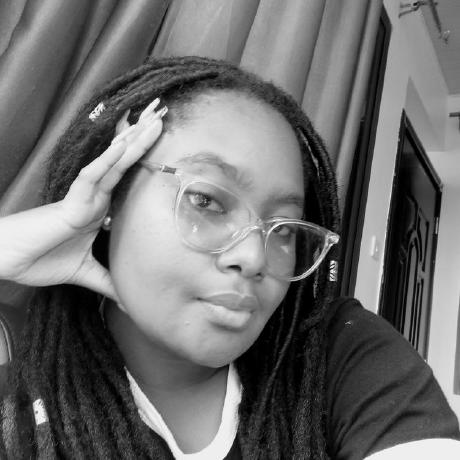
Table of contents
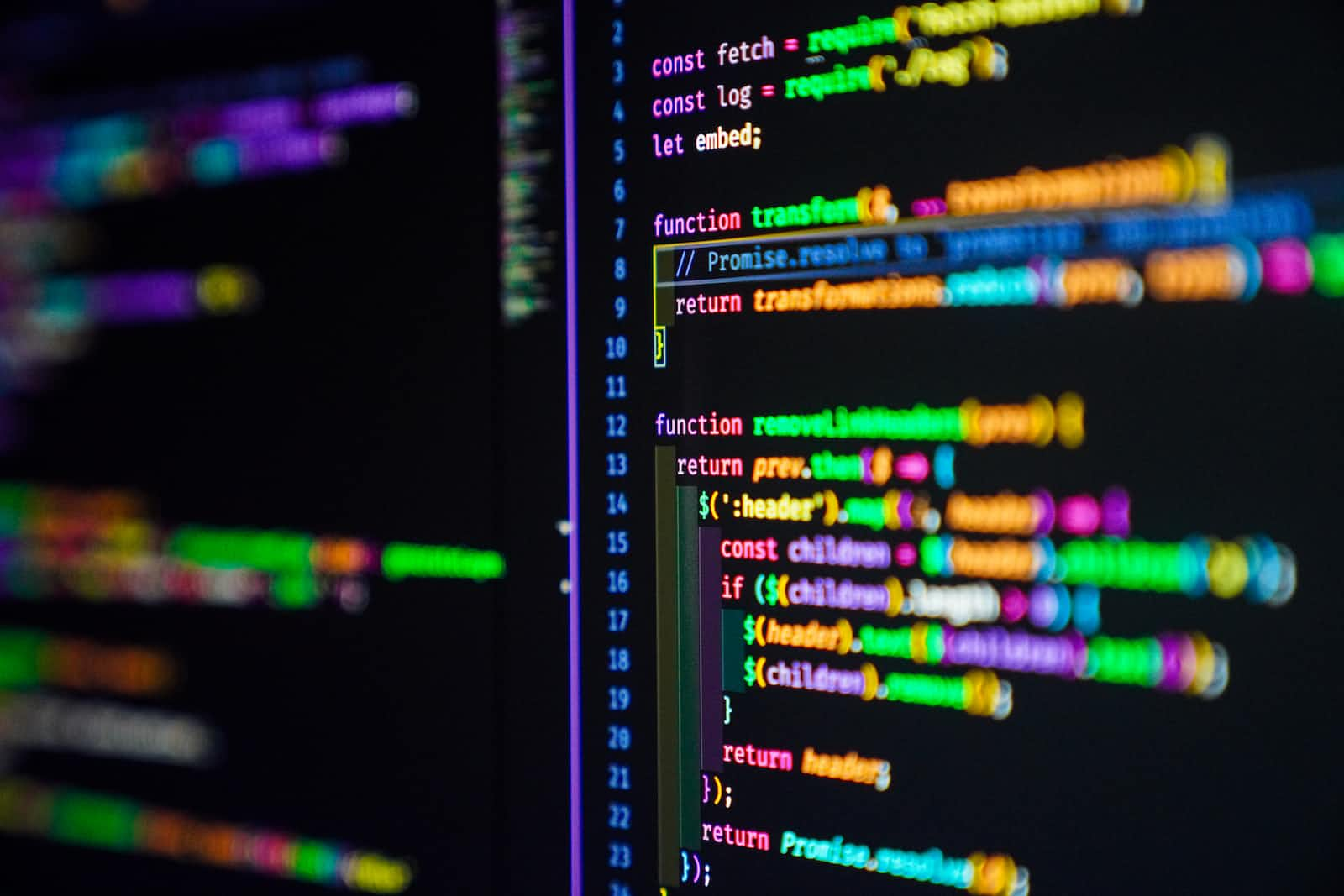
I was working on something recently and I reached a point where I had to destructure an array. Since I am aiming to get better at React, I decided to revisit the concept. This article contains information that I learned about destructuring arrays in JavaScipt.
What is Destructuring?
Destructing is a feature that allows you to extract elements from arrays and properties from objects and assign them to distinct variables. Destructuring makes your code readable and concise. It also makes it easier to reference elements in arrays.
Let's create an array that we will destructure.
const Countries = ['Kenya', 'Uganda', 'Tanzania'];
We'll destructure the array Countries
by creating three new variables countryOne
countryTwo
and countryThree
. These three variables will be mapped to the array elements from Countries
. This mapping is in order of the positions of the array elements and the variables created. Thus, countryOne
will have the value Kenya
, countryTwo
will have the value Uganda
and countryThree
will have the value Tanzania
as shown below.
const Countries = ['Kenya', 'Uganda ', 'Tanzania'];
const [countryOne, countryTwo] = Countries;
console.log(countryOne); // Kenya
console.log(countryTwo); // Uganda
If the number of variables created is more than the elements in the array, then the excess variables will not be assigned any value from the array. The value of these excess variables will be undefined.
const Countries = ['Kenya', 'Uganda ', 'Tanzania'];
const [countryOne, countryTwo, countryThree, countryFour] = Countries;
console.log(countryOne); // Kenya
console.log(countryTwo); // Uganda
console.log(countryThree); // Tanzania
console.log(countryFour); // undefined
Swapping variables
The values of these variables can be swapped in a single destructuring assignment. Since we are reassigning values we have to initialize our variables using let. When swapping, the variables are wrapped in []
brackets. In the code block below, we swap the values of countryOne
and countryTwo
.
const Countries = ['Kenya', 'Uganda ', 'Tanzania'];
let [countryOne, countryTwo, countryThree, countryFour] = Countries;
[countryOne, countryTwo] = [countryTwo, countryOne];
console.log(countryOne); // Uganda
console.log(countryTwo); // Kenya
console.log(countryThree); // Tanzania
console.log(countryFour); //undefined
Ignoring values
Destructuring allows values to be ignored during assignment. As shown below, Kenya
and Uganda
are ignored and countryThree
is mapped to the array element Tanzania
. ,
is used to ignore one array element at a time.
const Countries = ['Kenya', 'Uganda', 'Tanzania'];
let [, , countryThree, countryFour] = Countries;
console.log(countryThree); // Tanzania
conole.log(countryFour); // undefined
Default values
If the array element is missing, variables can be assigned default values using the = sign. If the array element is present, the default value will be ignored.
const Countries = ['Kenya', 'Uganda ', 'Tanzania', 'Rwanda'];
const [countryOne, countryTwo, countryThree, countryFour = 'Ethiopia'] = Countries;
console.log(countryOne); // Kenya
console.log(countryTwo); // Uganda
console.log(countryThree); // Tanzania
console.log(countryFour); // Rwanda
Nested arrays.
Destructuring nested arrays applies the same syntax as destructuring regular arrays. However, include nested brackets to match the syntax of the nested arrays.
const Countries = ['Kenya', ['Uganda ', 'Tanzania']];
const [countryOne, [countryTwo, countryThree]] = Countries;
console.log(countryOne); // Kenya
console.log(countryTwo); // Uganda
console.log(countryThree); // Tanzania
Conclusion
Destructuring arrays is simple and kind of fun. Just think of it as a fun way of referencing array elements.
Subscribe to my newsletter
Read articles from Sally Nzungula directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
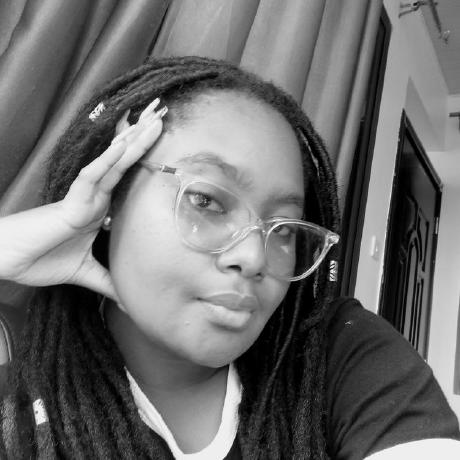
Sally Nzungula
Sally Nzungula
I'm a frontend developer based in Kenya. I enjoy learning and exploring new things in the software engineering universe.