Mastering File Renaming in Python with os.rename()
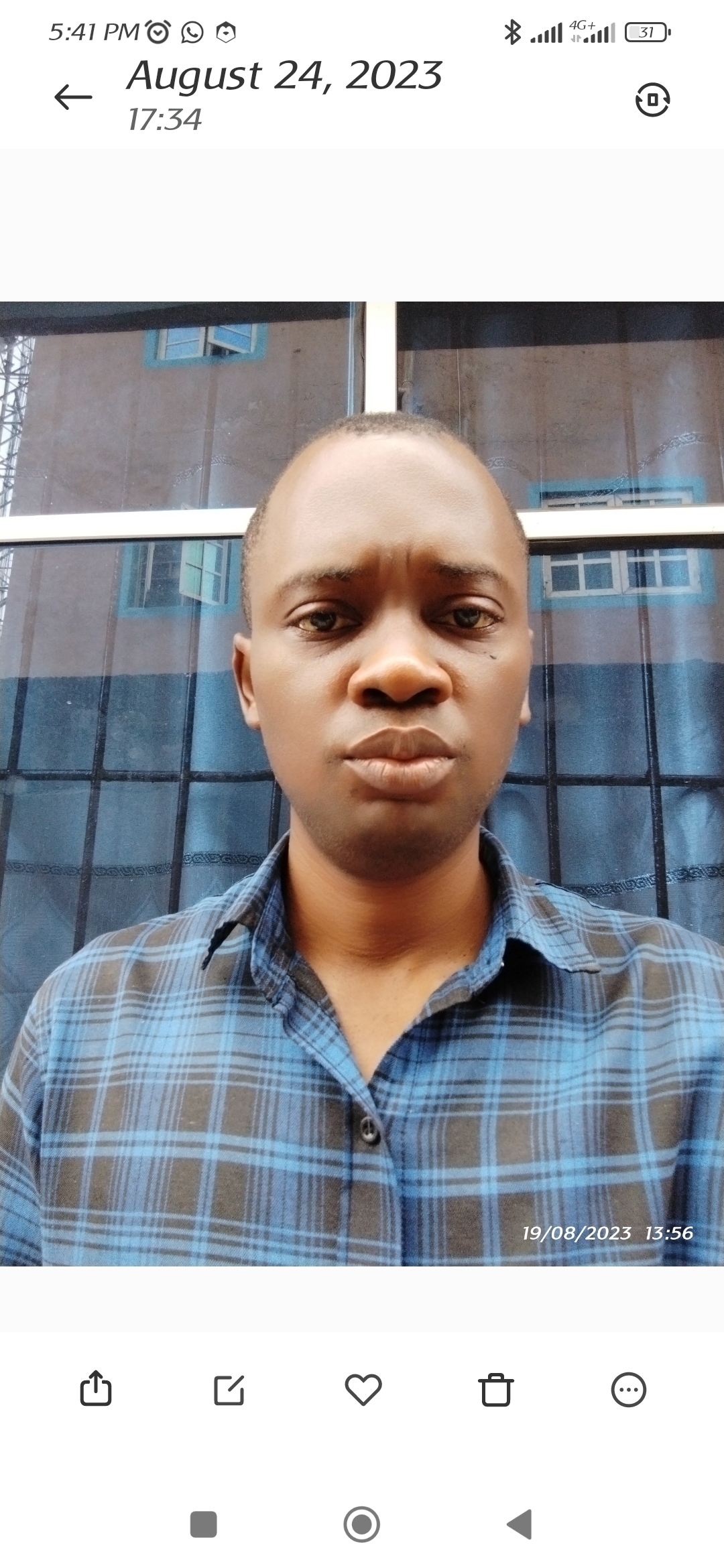
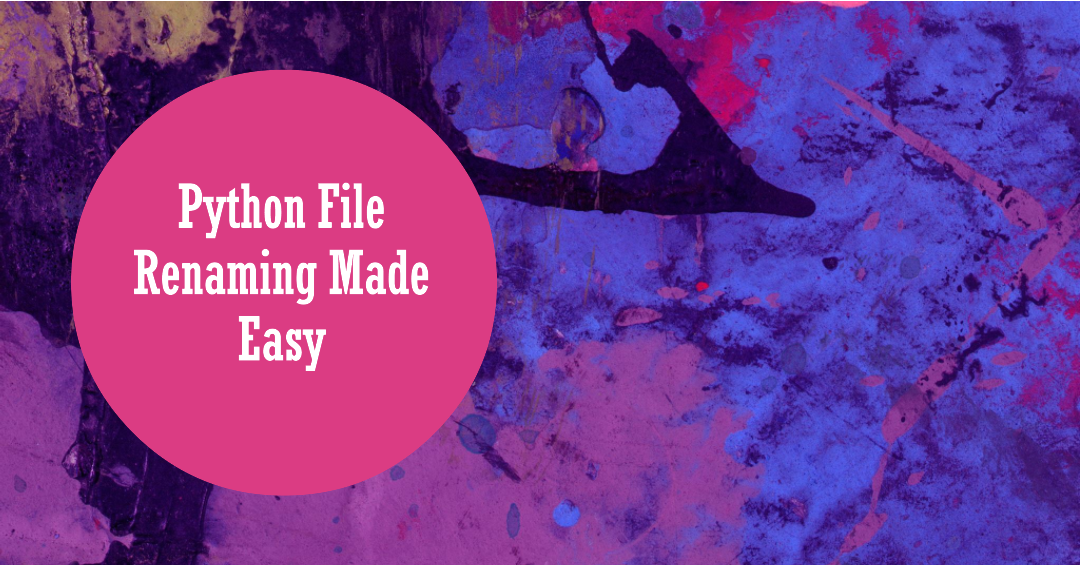
Introduction
File management is a common task in programming, and Python offers a versatile set of tools to help you manipulate files and directories. In this article, we'll explore the os.rename()
function, a powerful tool for renaming files. We'll discuss its usage, examine practical applications, and provide examples to illustrate its capabilities.
Understanding os.rename()
The os.rename()
function is part of Python's built-in os
module, designed to interact with the operating system. This function allows you to change the name of a file or directory by providing the current name and the desired new name. Here's the basic syntax:
import os
current_name = "old_name.txt"
new_name = "new_name.txt"
os.rename(current_name, new_name)
In this code snippet:
We import the
os
module to gain access to its functions.We define the
current_name
variable, which holds the current name of the file we want to rename.We set the
new_name
variable to the desired new name for the file.Finally, we use
os.rename(current_name, new_name)
to perform the renaming operation.
Applications of os.rename()
The os.rename()
function has various applications in file management and automation, including:
Bulk File Renaming: You can use it to rename multiple files in a directory at once, following a specific pattern or criteria.
Data Processing: When processing files in batch, you might need to rename them to maintain a structured naming convention.
File Versioning: It helps maintain different versions of files by renaming them accordingly.
File Sorting: You can use
os.rename()
to organize files into different folders based on certain criteria.
Examples of os.rename()
Usage
Let's dive into some practical examples to demonstrate how os.rename()
can be applied in various scenarios.
Example 1: Basic File Renaming
import os
current_name = "old_name.txt"
new_name = "new_name.txt"
os.rename(current_name, new_name)
This code renames a file named "old_name.txt" to "new_name.txt."
Example 2: Bulk File Renaming
import os
directory_path = '/path/to/your/directory'
for filename in os.listdir(directory_path):
if filename.endswith('.jpg'):
new_name = filename.replace('.jpg', '_new.jpg')
os.rename(os.path.join(directory_path, filename), os.path.join(directory_path, new_name))
In this example, we iterate through a directory, find all files with the ".jpg" extension, and add "_new" to their names.
Example 3: Organizing Files into Folders
import os
directory_path = '/path/to/your/directory'
for filename in os.listdir(directory_path):
if filename.startswith('doc_'):
new_folder = 'documents'
os.rename(os.path.join(directory_path, filename), os.path.join(directory_path, new_folder, filename))
This code sorts files that start with "doc_" into a "documents" folder within the same directory.
Conclusion
The os.rename()
function is an essential tool for file management and automation tasks in Python. Its versatility allows you to perform basic file renaming or tackle more complex operations like bulk renaming, file sorting, and versioning. By mastering os.rename()
, you gain the ability to efficiently organize and manage files, making your programming and automation tasks more efficient and structured.
Feel free to access the codebase by cloning the Git repository, which is available at the following Github URL
Subscribe to my newsletter
Read articles from Omini Okoi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
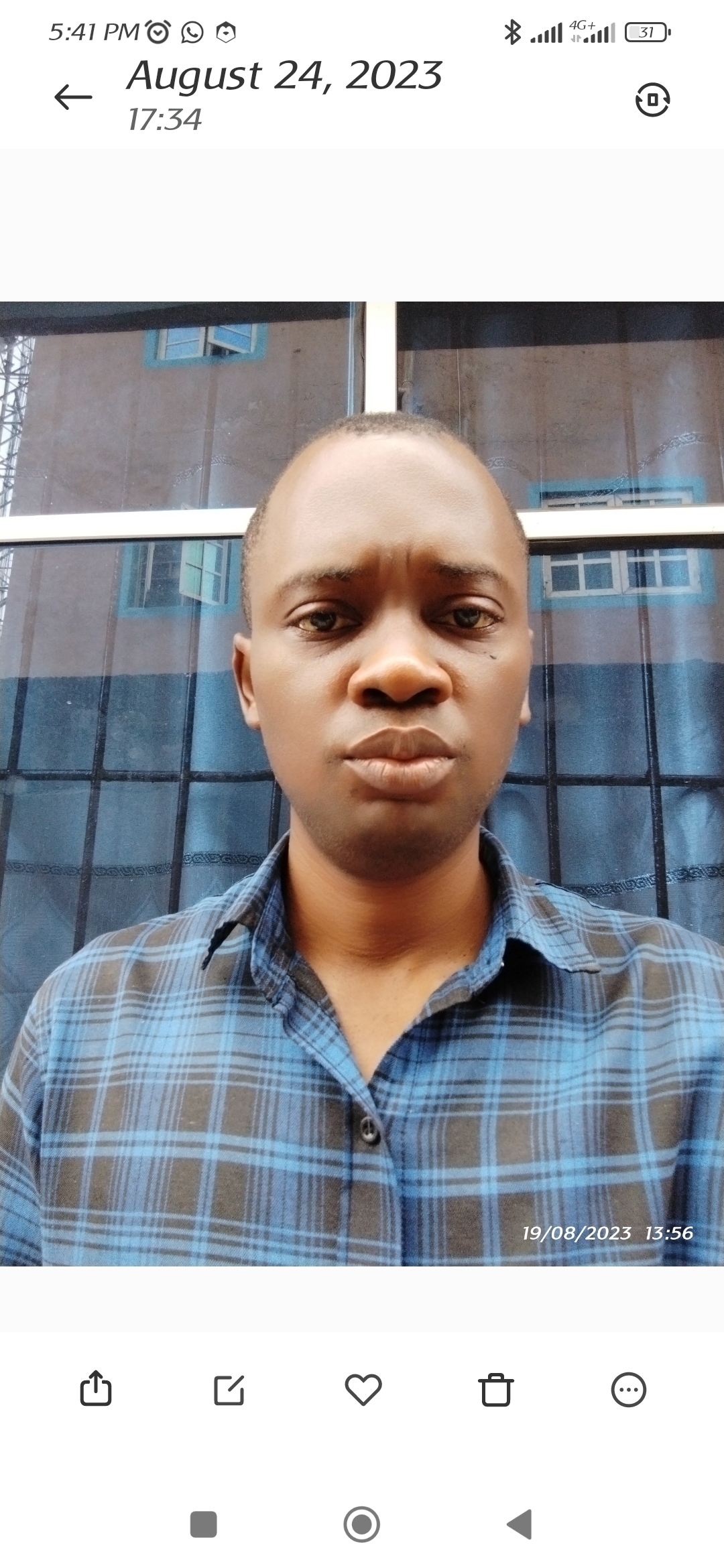
Omini Okoi
Omini Okoi
I am a web developer with experience in back-end engineering and cloud computing. I enjoy mentoring and training others in coding and technology. I’m always open to collaboration and building stuff that can make a positive impact.