A Comprehensive Guide to File Prefix and Suffix Manipulation in Python
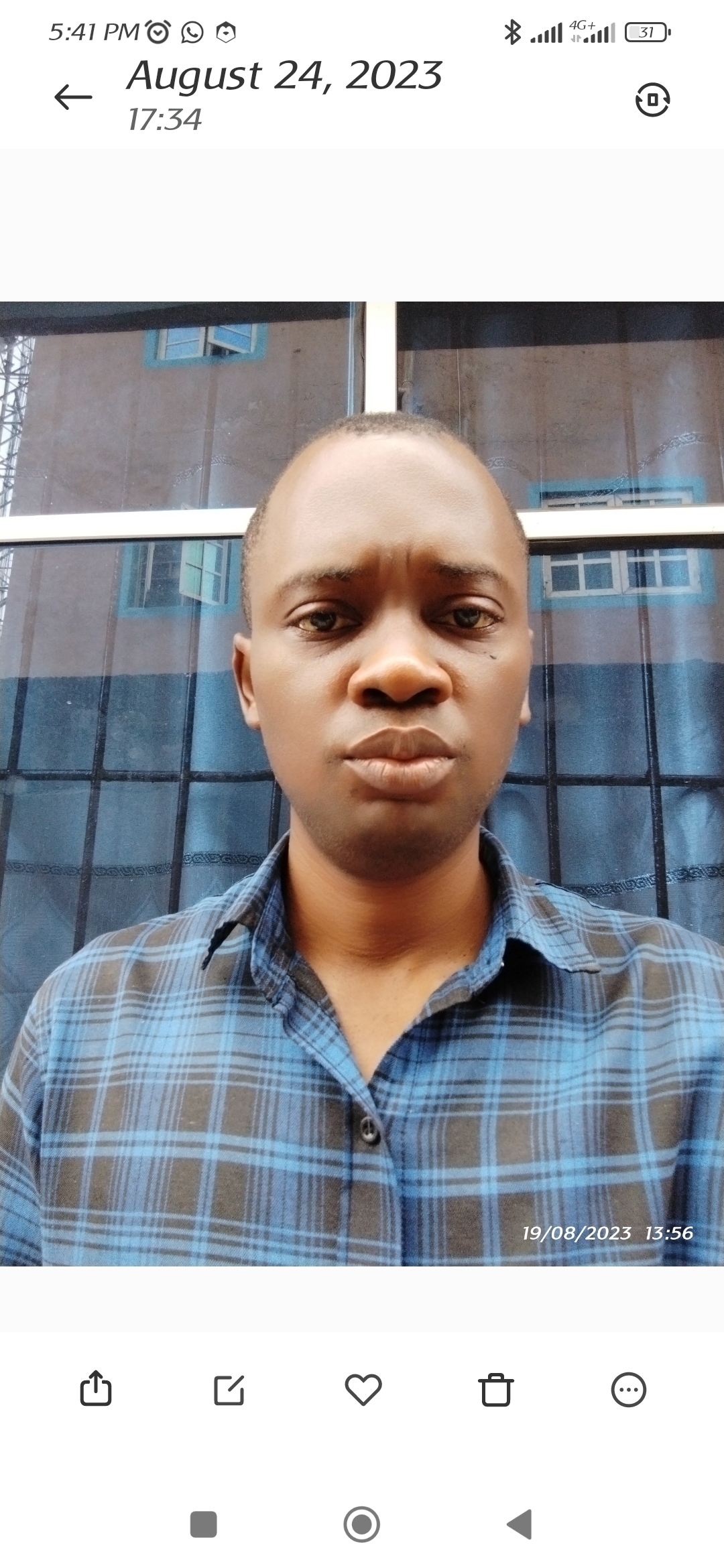
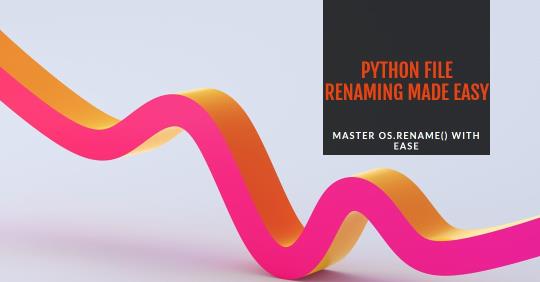
Introduction
File manipulation is a crucial aspect of any programming or automation task. Python offers powerful tools to simplify file operations, including renaming files. In this article, we'll explore the use of Python scripts to add and remove prefixes and suffixes from filenames. We'll discuss how each script works, provide practical examples, and demonstrate how to apply them effectively.
Script 1: Adding a Prefix to Filenames
#!/usr/bin/python3
import os
for file in os.listdir('.'):
old_name = file
new_name = 'movies-' + file
if file.endswith('txt'):
os.rename(old_name, new_name)
This script adds the prefix "movies-" to all text files in the current directory. Here's how it works:
It iterates through all files in the current directory using
os.listdir('.')
.For each file, it checks if it ends with the ".txt" extension.
If the file meets this condition, it renames it by prepending "movies-" to its original name.
Script 2: Adding a Suffix to Filenames
#!/usr/bin/python3
import os
for file in os.listdir('.'):
if file.endswith('.txt'):
old_file = os.path.join('.', file)
new_file = os.path.join('.', os.path.splitext(file)[0] + '-cartoon' + os.path.splitext(file)[1])
os.rename(old_file, new_file)
This script adds the suffix "-cartoon" to the base name (without the extension) of all text files in the current directory. Here's how it works:
It iterates through all files in the current directory using
os.listdir('.')
.For each file with the ".txt" extension, it constructs the new name by appending "-cartoon" to the base name (without the extension).
It then renames the file using
os.rename()
.
Script 3: Removing a Prefix from Filenames
#!/usr/bin/python3
import os
prefix = "movies"
[os.rename(file, file.replace(prefix, "")) for file in os.listdir('.') if file.startswith(prefix)]
This script removes the specified prefix ("movies") from all filenames in the current directory. Here's how it works:
It defines the
prefix
variable with the value to be removed.It uses a list comprehension to iterate through all files in the current directory (
os.listdir('.')
) that start with the specified prefix.For each file with the prefix, it renames the file by removing the prefix using the
str.replace()
method.
Script 4: Removing a Suffix from Filenames
#!/usr/bin/python3
import os
suffix = "-cartoon"
for file in os.listdir('.'):
old_file = os.path.join('.', file)
new_file = file.replace(suffix, '')
os.rename(old_file, new_file)
This script removes the specified suffix ("-cartoon") from all filenames in the current directory. Here's how it works:
It defines the
suffix
variable with the value to be removed.It iterates through all files in the current directory using
os.listdir('.')
.For each file, it constructs the new name by removing the suffix using the
str.replace()
method.It then renames the file using
os.rename()
.
Conclusion
Python's os
module provides powerful tools for renaming files with ease. By understanding and utilizing scripts like the ones presented in this article, you can efficiently manipulate filenames in your programming and automation tasks. Whether you need to add or remove prefixes and suffixes, these scripts provide valuable techniques for managing your files effectively.
Feel free to access the codebase by cloning the Git repository, which is available at the following Github URL
Subscribe to my newsletter
Read articles from Omini Okoi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
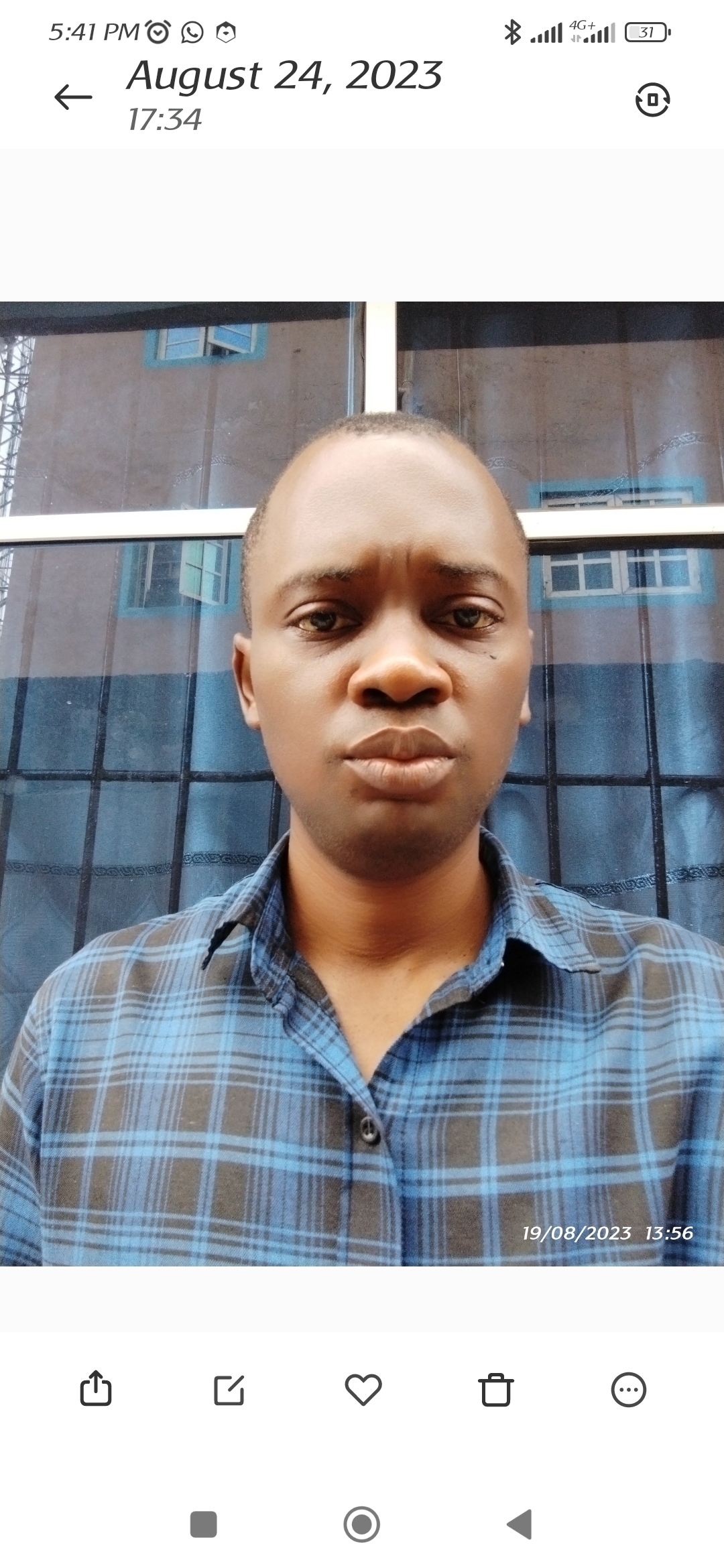
Omini Okoi
Omini Okoi
I am a web developer with experience in back-end engineering and cloud computing. I enjoy mentoring and training others in coding and technology. I’m always open to collaboration and building stuff that can make a positive impact.