Creating a Simple Python Thermostat Control System
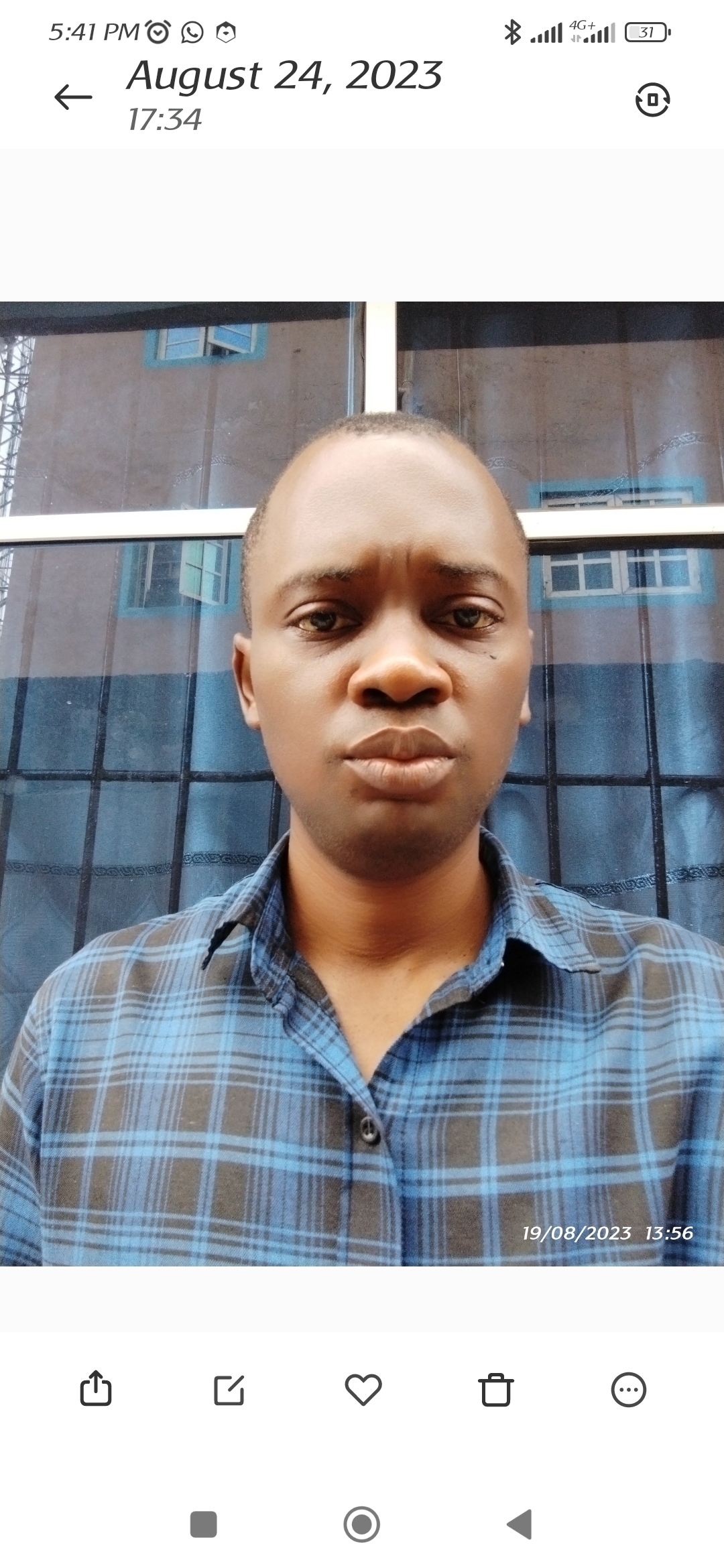

Introduction
In today's modern world, automation and smart control systems have made their way into our homes, making our lives more comfortable and efficient. One such example is a thermostat control system. In this article, we'll explore a simple Python script that mimics the behavior of a thermostat to maintain a desired temperature range.
The Thermostat Script
#!/usr/bin/python3
import time
def thermostat(temp, low_temp, high_temp):
direction = 1
while True:
if temp < low_temp:
print("Min Temp reached. Heating...")
direction = 1
elif temp > high_temp:
print("Max Temp reached. Cooling...")
direction = -1
temp += direction
print(f"Current temperature: {temp}°C")
time.sleep(1)
thermostat(24, 18, 26)
Let's break down how this simple thermostat control system works:
Initial Parameters: The script starts by defining three parameters:
temp
(the current temperature),low_temp
(the lower bound of the desired temperature range), andhigh_temp
(the upper bound of the desired temperature range). These parameters are set to initial values.Direction Control: The
direction
variable is introduced to control whether the thermostat needs to heat (direction = 1
) or cool (direction = -1
) the environment.Thermostat Loop: The script enters a
while True
loop, which means it will continuously monitor and adjust the temperature.Temperature Checks: Within the loop, the script checks if the current temperature (
temp
) is below the desired lower bound (low_temp
) or above the desired upper bound (high_temp
). Depending on the situation, it prints messages indicating whether it needs to heat or cool the environment.Temperature Adjustment: The
temp
variable is adjusted according to thedirection
. If the temperature needs to be increased (heating),temp
is incremented by 1. If it needs to be decreased (cooling),temp
is decremented by 1.Current Temperature Display: The script continuously prints the current temperature (
temp
) to provide feedback to the user.Delay: To simulate real-world temperature changes, the script adds a 1-second delay using
time.sleep(1)
between each temperature check and adjustment.Function Call: Finally, the
thermostat
function is called with initial values (24
degrees Celsius as the starting temperature,18
as the lower bound, and26
as the upper bound) to start the thermostat control loop.
Conclusion
This simple Python script provides a basic simulation of a thermostat control system. It can be a starting point for more complex home automation projects where you can integrate actual temperature sensors and control heating or cooling devices based on real-time data. Building upon this foundation, you can create a smart and energy-efficient climate control system for your home.
Subscribe to my newsletter
Read articles from Omini Okoi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
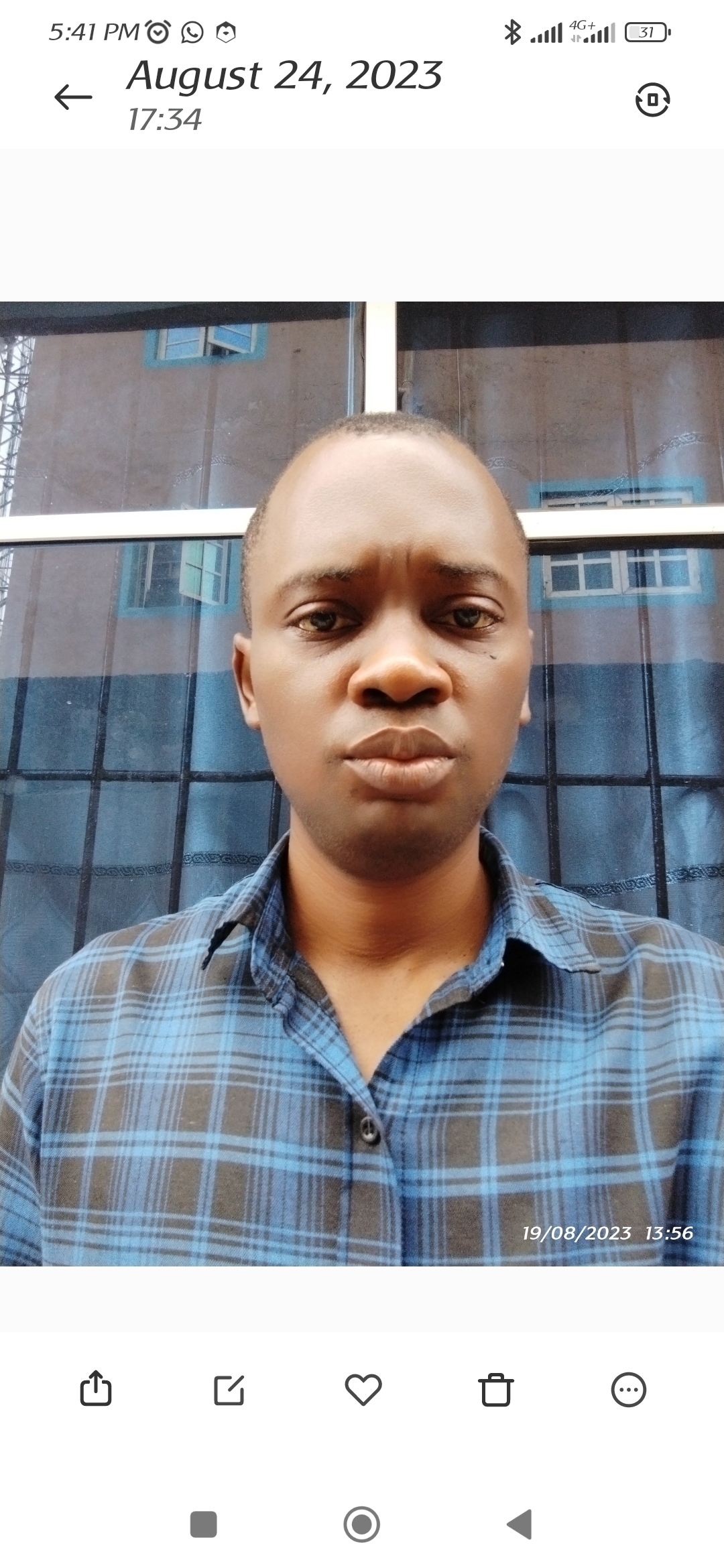
Omini Okoi
Omini Okoi
I am a web developer with experience in back-end engineering and cloud computing. I enjoy mentoring and training others in coding and technology. I’m always open to collaboration and building stuff that can make a positive impact.