Java Classes, Objects, and Methods: A Beginner's Guide
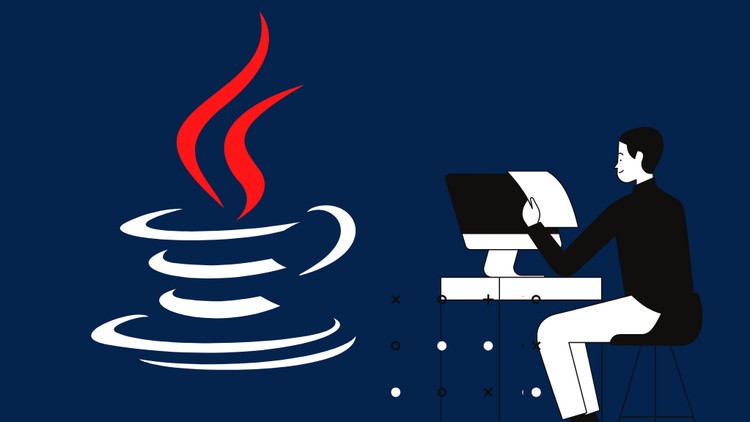
Objective :
This blog aims to introduce beginners to essential Java concepts: classes, objects, and methods. By explaining these concepts with clear examples, it helps readers understand how Java programs are structured.
Introductions of Class & Objects:
Java Classes:
In Java, a class is like a blueprint for creating something called an object. This blueprint defines what the object can do and what it knows. A class created using the keyword class
followed by className and a pair of curly braces {}
to define the class body, which contains its attributes and methods.
class ClassName{
//Attributes
//methods
}
Java Objects:
An object, on the other hand, is an instance of a class and exists in memory when your program runs. It represents a real entity with data and behavior, as defined by the class. An object is created using the new
keyword followed by the class name and parentheses ()
to call the class's constructor. This initializes the object and allocates memory for it.
syntax: ClassName ref = new ClassName();
Now let's see a small code example
public class Calculator{
int add(int a, int b){
return a+b;
}
int mult(int a, int b){
return a*b;
}
int sub(int a, int b){
return a-b;
}
}
In the above code Calculator class contents methods. Methods are nothing but behavior. Now an object of this class can perform/execute the behavior of that class.
public class Main{
public static void main(String[] a){
//Object creation
Calculator ob = new Calculator();
int addition = ob.add(2,3);
System.out.println(addition);
int multiply = ob.mult(2,3);
System.out.println(multiply);
int subtraction = ob.sub(2,3);
System.out.println(subtraction);
}
}
Each object of a class has its own copy of the state (attributes or member variables) and behavior (methods) defined by that class. This means that changes made to one object's state do not affect the state of other objects of the same class. Each object maintains its uniqueness in terms of data. Let's see a small code syntax of two objects of the same class performing the same task with different inputs as parameters.
//object1
Calculator ob1 = new Calculator();
ob1.add(1,6);
//object2
Calculator ob2 = new Calculator();
ob2.add(2,3);
Attributes:
In Java, an attribute is often referred to as a "field" or "member variable." It is a data member of a class that holds values associated with instances of that class. Attributes define the characteristics or properties of objects created from a class.
Let's see one more code to show that one object's state does not affect the state of another object of the same class.
class Student{
//Attributes
String name;
//Methods
public void hello(){
System.out.println("My name is "+name);
}
}
public class Main
{
public static void main(String[] args) {
Student s1 = new Student();
s1.name = "Dhanjeet";
s1.hello();
Student s2 = new Student();
s2.name = "Chaitanya";
s2.hello();
}
}
Output:
My name is Dhanjeet
My name is Chaitanya
Java Methods:
A method is a block of code enclosed within curly braces {}
. It contains a set of statements that perform a specific task or operation. Methods are used to achieve code reusability by writing the code once and calling or invoking the method multiple times. It Enhances readability and maintainability by organizing code into modular, named functions. It is executed only when it is invoked by an instance of a class(object). A method must be declared within a class. It is defined with the name of the method, followed by parentheses ().
A method in Java has several components:
Method Signature: It includes the method name and parameter list.
Access Specifier: It defines who can access the method (public, private, protected, or default).
Return Type: It specifies what the method will return (e.g., a data type or "void" if nothing is returned).
Method Name: A unique name for the method.
Parameter List: The data type and variable names of any input the method receives.
Method Body: The actual code enclosed in curly braces that defines what the method does.
Methods are of two types
Predefined Methods: These methods are already defined in the class library, both by Oracle and third-party libraries created by various entities and open-source communities. For example, in Java, the
String class
comes with predefined methods such asequals()
,length()
,isEmpty()
,charAt()
,toUpperCase()
,toLowerCase()
and more. Predefined methods are also referred to as 'built-in methods' or 'standard library methods'. Let's see a code examplepublic class Main{ public static void main(String[] args){ String s1 = " Java World"; System.out.println("The String s1 :"+s1); //Let's use a predefined method to convert string all uppercase String s2 = s1.toUpperCase(); //here toUpperCase() is predefined method of String class. System.out.println("The Uppercase s2 :"+s2); } }
Output : The String s1 : Java World The Uppercase s2 : JAVA WORLD
User-defined Method: These methods are defined by the user or programmer based on the requirement.
public class Main{ //User-defined Method void helloMsg(){ System.out.println("Hello World"); } public static void main(String[] args){ Main ob = new Main(); ob.helloMsg(); //invoking the user-defined method } }
output: Hello World
Conclusion:
This blog explored Java's core concepts: classes (blueprints for objects), objects (representing real-world entities), and methods (code blocks for tasks). It provided practical examples to illustrate these concepts, emphasizing their importance as the foundation of Java programming
Subscribe to my newsletter
Read articles from Dhanjeet Kumar Thakur directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Dhanjeet Kumar Thakur
Dhanjeet Kumar Thakur
I'm Dhanjeet Kumar Thakur, a dedicated backend developer skilled in Core Java, Spring frameworks, Hibernate, and web tech like HTML, CSS, and JavaScript. With a sharp eye for detail, I create strong backend solutions for seamless user experiences. Git and GitHub are my allies for effective collaboration and code integrity. Always learning, I'm on a mission to elevate my skills and make an impact in Java backend development.