Finding, Fixing, and Preventing N+1 Queries in Ruby on Rails
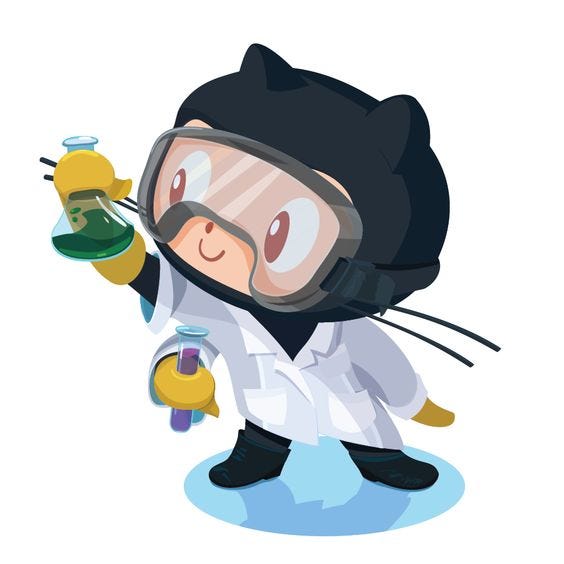
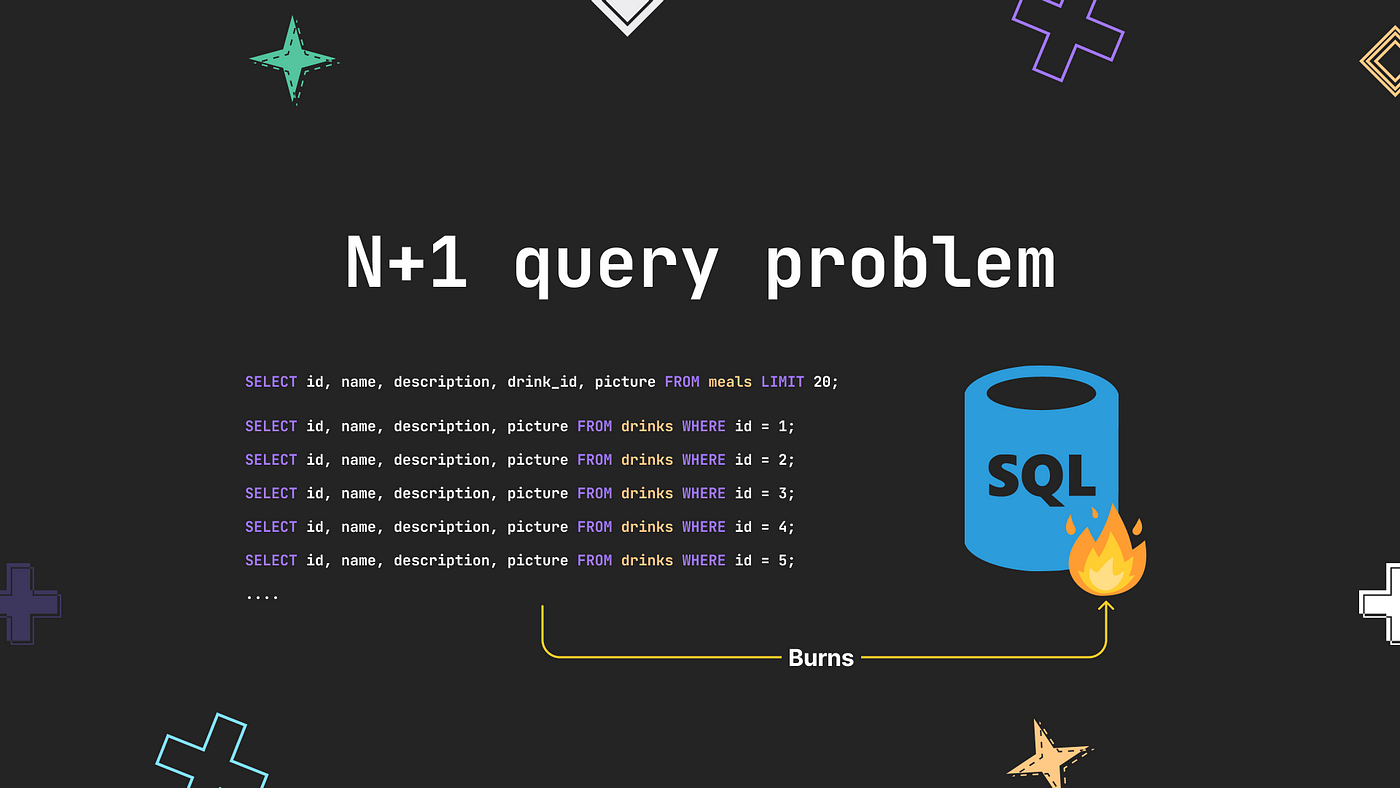
One of the most common performance bottlenecks in Ruby on Rails applications is the N+1 query problem. It's a scenario where you fetch a collection of records and then, for each record, perform an additional database query. This can lead to a significant increase in the number of database queries, slowing down your application. In this blog post, we'll explore how to find, fix, and prevent N+1 queries in your Rails application.
Identifying N+1 Queries
Before we can fix N+1 queries, we need to identify them. Rails provides some built-in tools to help us spot these inefficiencies.
1. Bullet Gem
Bullet is a gem that helps you detect N+1 query issues. To use it, add it to your Gemfile:
gem 'bullet', group: 'development'
And then run:
bundle install
In your config/environments/development.rb
, enable Bullet:
config.after_initialize do
Bullet.enable = true
Bullet.rails_logger = true
end
With Bullet enabled, it will log N+1 query issues in your Rails application's log. When you see a message like N+1 Query Detected
, it means you have an N+1 query problem.
2. Active Record Log
Rails itself provides some information about database queries in the log. Look for queries that appear frequently, especially within loops. If you see a query that's repeated for each item in a collection, that's a potential N+1 issue.
Fixing N+1 Queries
Once you've identified N+1 queries in your application, it's time to fix them. Let's go through common scenarios and how to address them.
1. Eager Loading
Eager loading is the most common solution for N+1 query problems. It allows you to load associated records along with the main record in a single query.
Example 1: Loading Associated Records
Suppose you have a Post
model that has_many
comments, and you want to load all posts with their comments:
# Inefficient: N+1 queries
@posts = Post.all
@posts.each { |post| @comments = post.comments }
To fix this, use eager loading:
# Efficient: 2 queries (1 for posts, 1 for comments)
@posts = Post.includes(:comments).all
Example 2: Nested Associations
If you have deeply nested associations, you can use a hash to specify which associations to preload:
@posts = Post.includes(:comments => [:user, :likes]).all
Preventing N+1 Queries
While fixing N+1 queries is essential, preventing them in the first place is even better. Here are some strategies to avoid N+1 queries from the beginning.
1. Use joins
for Complex Queries
When you have complex queries that involve multiple tables, consider using joins
to fetch the required data in a single query.
Example: Joining Tables
Suppose you want to fetch all authors with their associated books and genres. Use joins
to combine the necessary tables:
authors = Author.joins(:books => :genres).distinct
2. Review and Optimize Code
Regularly review your code for potential N+1 query issues. Pay attention to loops and iterations over collections of records. Ensure that you load associated records efficiently using eager loading.
3. Database Indexing
Optimize your database by adding appropriate indexes to columns that are frequently used in queries. Indexing can significantly speed up database operations.
4. Pagination
Implement pagination for large collections of records. Fetching all records at once can lead to performance issues. Use tools like kaminari
or will_paginate
to paginate your data.
5. Caching
Cache frequently accessed data to reduce database queries. Use Rails' caching mechanisms to store and retrieve data efficiently.
Conclusion
Identifying, fixing, and preventing N+1 query problems in your Ruby on Rails application is essential for maintaining good performance. With the right tools and strategies, you can ensure that your application remains responsive and efficient, even as your data grows.
By using tools like Bullet, understanding eager loading, and optimizing your code, you can keep N+1 queries at bay and deliver a smooth user experience.
Remember, database performance is a crucial aspect of your application's overall performance, and addressing N+1 query issues is a big step towards achieving that goal.
Subscribe to my newsletter
Read articles from sivalaxman directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
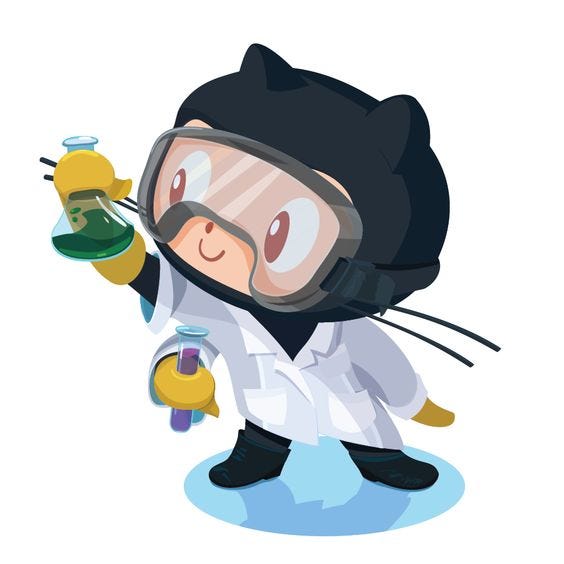
sivalaxman
sivalaxman
Dedicated and Skilled Full Stack Developer with experience in building and optimizing user-centric web applications. Proficient in a wide range of technologies, including front-end frameworks such as React.js and Next.js, as well as back-end technologies like Kotlin with Micronaut, Ruby on Rails, and Node.js. Experienced in working with databases, RESTful APIs, and third-party integrations, with a strong commitment to delivering high-quality software solutions.