Day 3 || Creating a Dockerfile in Docker: A Step-by-Step Guide
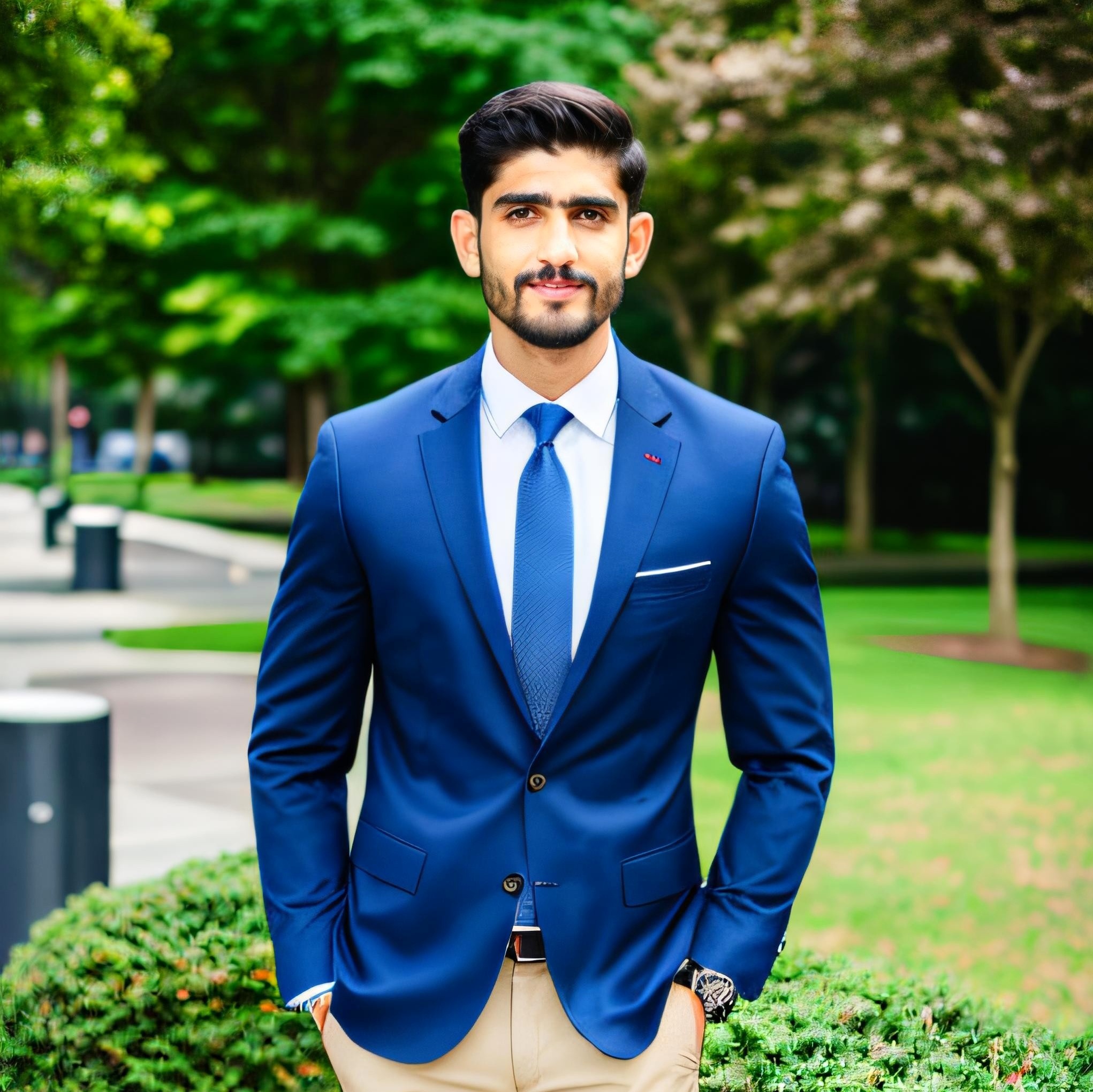
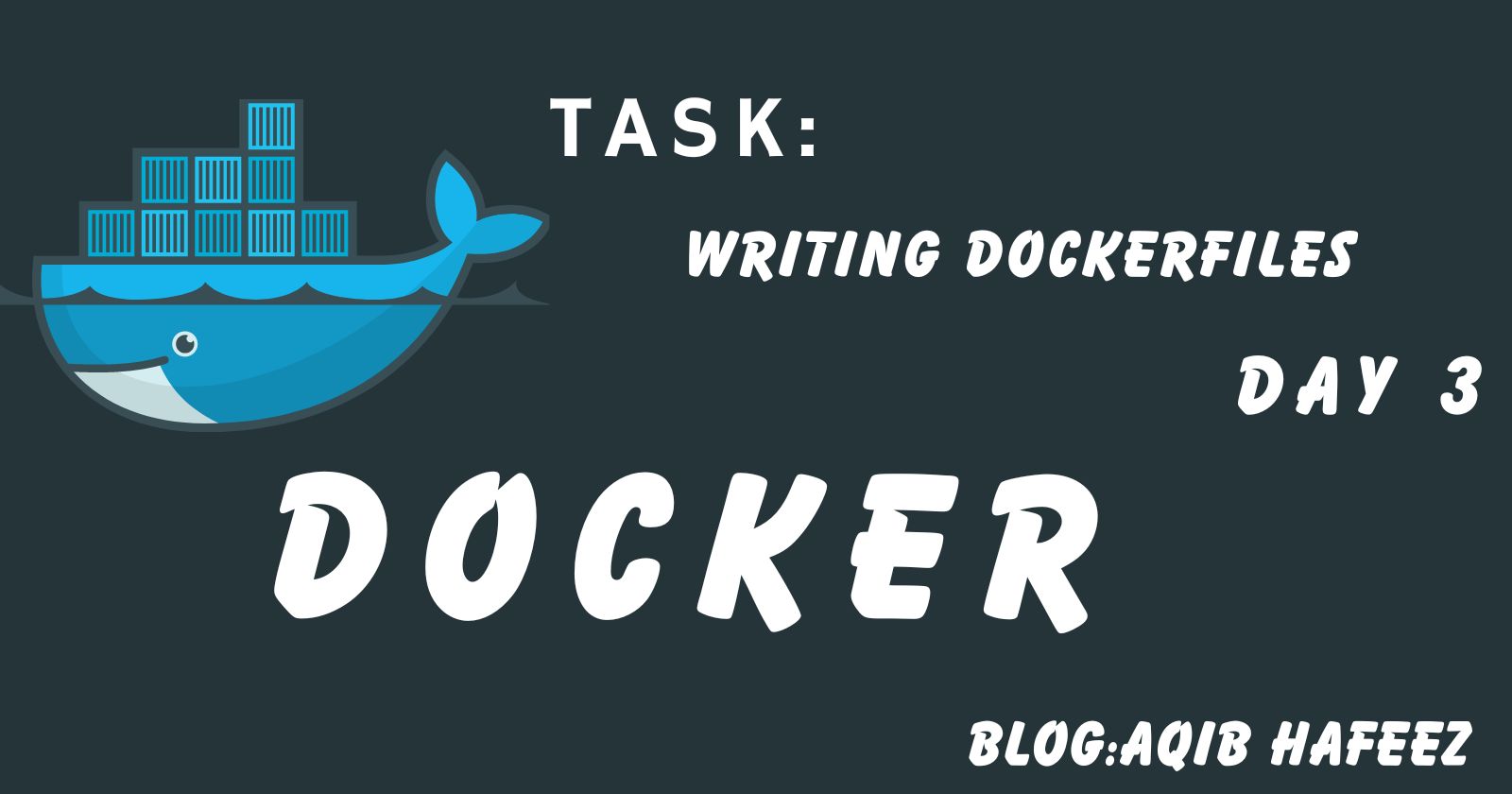
Introduction:
Docker is a powerful tool for containerizing applications, making them portable and easy to deploy across different environments. One of the key components of using Docker is creating a Docker file. In this blog post, we'll walk you through the process of creating a Dockerfile, explain its essential components, and provide a simple example to get you started.
Creating a Dockerfile:
1. Dockerfile Overview:
Description: Learn about the purpose and importance of a Dockerfile in containerization.
2. Base Image Selection:
- Description: Choose an appropriate base image for your application, such as a specific Linux distribution or a pre-configured development environment.
. FROM
Instruction:
Description: The
FROM
instruction specifies the base image that your Docker image will be built upon. It provides the starting point for your application's container.Example: You can choose a base image from the Docker Hub or another container registry. For example, to use an official Python 3.8 image as a base:
FROM python:3.8-slim
2. WORKDIR
Instruction:
Description: The
WORKDIR
instruction sets the working directory for any subsequent instructions in the Dockerfile. This is where your application's files and code will be located inside the container.Example: Setting the working directory to
/app
:
WORKDIR /app
3. COPY
Instruction:
Description: The
COPY
instruction copies files and directories from your host machine (the system where you are building the Docker image) into the container's file system.Example: Copying the current directory's contents into the
/app
directory in the container:
COPY . /app
4. RUN
Instruction:
Description: The
RUN
instruction executes commands during the image build process. It can be used to install packages, run scripts, and perform other setup tasks within the container.Example: Installing Python packages from a
requirements.txt
file:
RUN pip install --trusted-host pypi.python.org -r requirements.txt
5. EXPOSE
Instruction:
Description: The
EXPOSE
instruction specifies which ports the container should listen on at runtime. It does not actually publish the ports; it is more of a documentation mechanism.Example: Exposing port 80 for web traffic:
EXPOSE 80
6. ENV
Instruction:
Description: The ENV
instruction sets environment variables that can be accessed by processes running inside the container. It's often used to configure aspects of the application's behavior.
- Example: Defining an environment variable:
ENV NAME World
7. CMD
Instruction:
Description: The
CMD
instruction specifies the default command to run when the container starts. This is typically the command that starts your application.Example: Running a Python script as the default command:
CMD ["python", "app.py"]
Here's a complete example of a Dockerfile for a Python web application, combining all these components:
DockerfileCopy code# Use an official Python runtime as a parent image
FROM python:3.8-slim
# Set the working directory to /app
WORKDIR /app
# Copy the current directory contents into the container at /app
COPY . /app
# Install any needed packages specified in requirements.txt
RUN pip install --trusted-host pypi.python.org -r requirements.txt
# Make port 80 available to the world outside this container
EXPOSE 80
# Define environment variable
ENV NAME World
# Run app.py when the container launches
CMD ["python", "app.py"]
This Dockerfile creates an image for a Python web application, copying its files, installing dependencies, exposing port 80, setting an environment variable, and running the application when a container is started from this image.
Fiverr:
Subscribe to my newsletter
Read articles from AQIB HAFEEZ directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
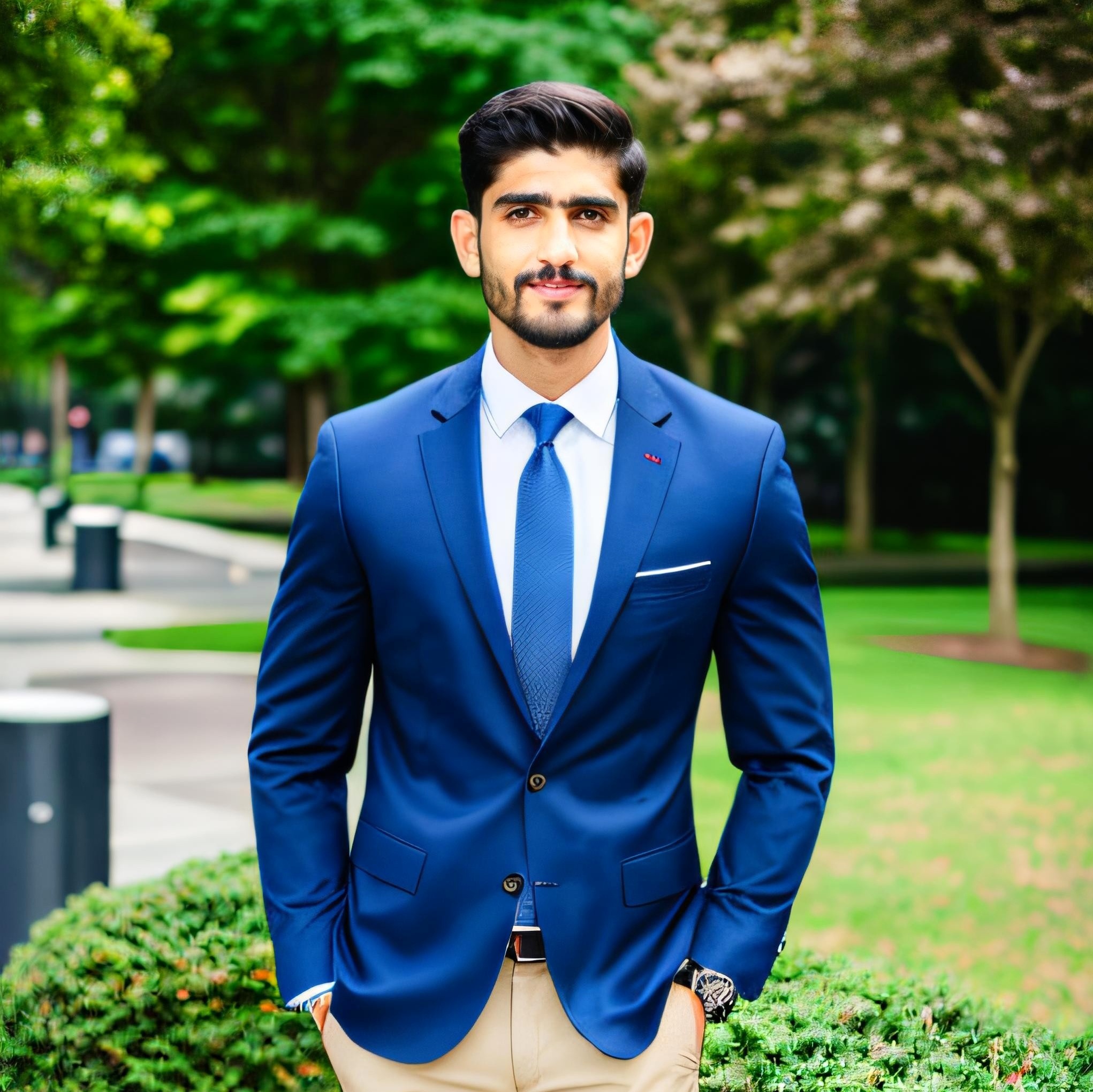
AQIB HAFEEZ
AQIB HAFEEZ
DevOps Engineer, Linux, Git, GitHub, GitLab, CI/CD pipelines, Jenkins, Docker, Kubernetes, Ansible & AWS. Practical experience in these DevOps tools enhances my passion for streamlined workflows, automated processes, and holistic development solutions. Proficient in digital and Facebook marketing, aiming to merge technical acumen with marketing finesse.