Browser History Manipulation
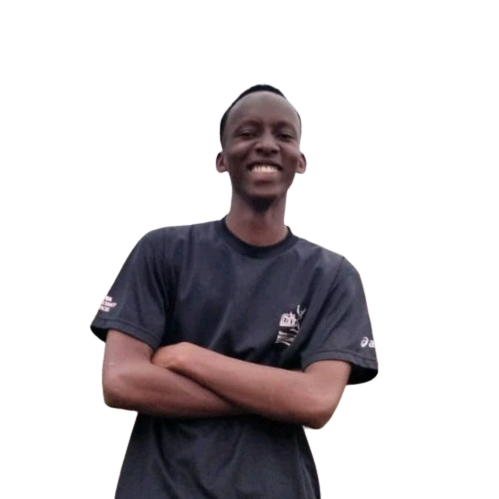
In modern web development, managing browser history is crucial for creating seamless user experiences. One of the fundamental components that enable this is the set of functions provided in the code snippet you've shared. These functions are responsible for manipulating the browser's history stack, tracking navigation, and allowing for smooth client-side routing. Let's dive deep into how these functions work and their significance.
Section 1: Introduction
The provided code snippet consists of four functions, namely: pushState
, replaceState
, onPopState
, and onPushState
. Together, they allow developers to control the browser's history stack, listen for navigation events, and trigger custom actions based on changes in the application's state.
Section 2: pushState
and replaceState
The pushState
Function
export const pushState = (state, title, url) => {
window.history.pushState(state, title, url);
};
The pushState
function is a core part of HTML5 history manipulation. It allows you to add a new entry to the browser's history stack without triggering a full page refresh. Let's break down its parameters:
state
(Object): This is an arbitrary JavaScript object that represents the state associated with the new history entry. This state can be used to store additional data related to the page's state.title
(String): Although ignored by most browsers, this parameter represents the title of the history entry.url
(String): The URL to be pushed to the history stack. This URL can be relative or absolute.
When you call pushState
, it adds a new history entry with the provided state and URL to the stack, making it appear as if the user has navigated to a new page. However, the page content doesn't actually change until you update the view manually.
The replaceState
Function
export const replaceState = (state, title, url) => {
window.history.replaceState(state, title, url);
};
The replaceState
function is similar to pushState
, but with a crucial difference. Instead of adding a new entry to the history stack, it replaces the current history entry with a new one. This can be particularly useful for situations where you want to update the URL without adding a new navigation point to the stack. Its parameters are the same as those of pushState
.
Section 3: Event Listeners
The onPopState
Function
export const onPopState = (handler) => {
window.addEventListener('popstate', handler);
};
The onPopState
function sets up an event listener for the 'popstate' event. This event is triggered whenever the user navigates backward or forward in the browser's history, either by using the back/forward buttons or programmatically. When such an event occurs, the provided handler
function is executed, allowing you to respond to changes in the history stack.
The onPushState
Function
export const onPushState = (handler) => {
window.addEventListener('pushstate', handler);
};
While not a native browser event, the onPushState
function lets you define custom behavior when a 'pushstate' event occurs. This event can be triggered programmatically using the pushState
function. By setting up an event listener using onPushState
, you can execute specific actions when you want to simulate a navigation event without relying on user interaction.
Section 4: Practical Use Cases
These functions are instrumental when implementing client-side routing in single-page applications (SPAs). They enable developers to create dynamic, responsive web applications that load content asynchronously without full page refreshes. Additionally, they make it possible to maintain clean and meaningful URLs that reflect the application's state.
Conclusion
Understanding how to manipulate the browser's history stack and respond to navigation events is a valuable skill in modern web development. Whether you're building SPAs, enhancing user experiences, or implementing advanced routing mechanisms, these functions provide a powerful toolset for controlling and managing the browser's history.
By mastering these techniques, you can create web applications that feel more like native applications, providing users with a seamless and enjoyable browsing experience.
Get the full code here on my Github Repository - Better Programming.
Feel Free to collaborate.
If you are happy with my content I'll be happy to connect with you on Linkedin
Happy coding!
Subscribe to my newsletter
Read articles from Titus Kiplagat directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
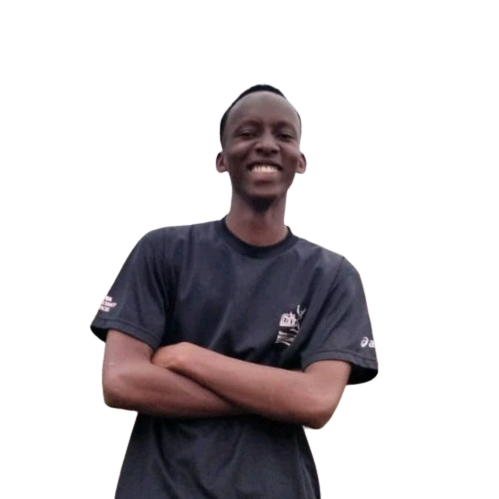
Titus Kiplagat
Titus Kiplagat
Passionate Software Developer with 3+ years of experience specializing in Python programming, AI-driven solutions, and automation workflows. Proficient in integrating APIs, implementing machine learning models, and developing scalable backend systems. Dedicated to leveraging technology to solve real-world problems efficiently and effectively.