Diving into Docker Compose: Simplifying Multi-Container Deployments

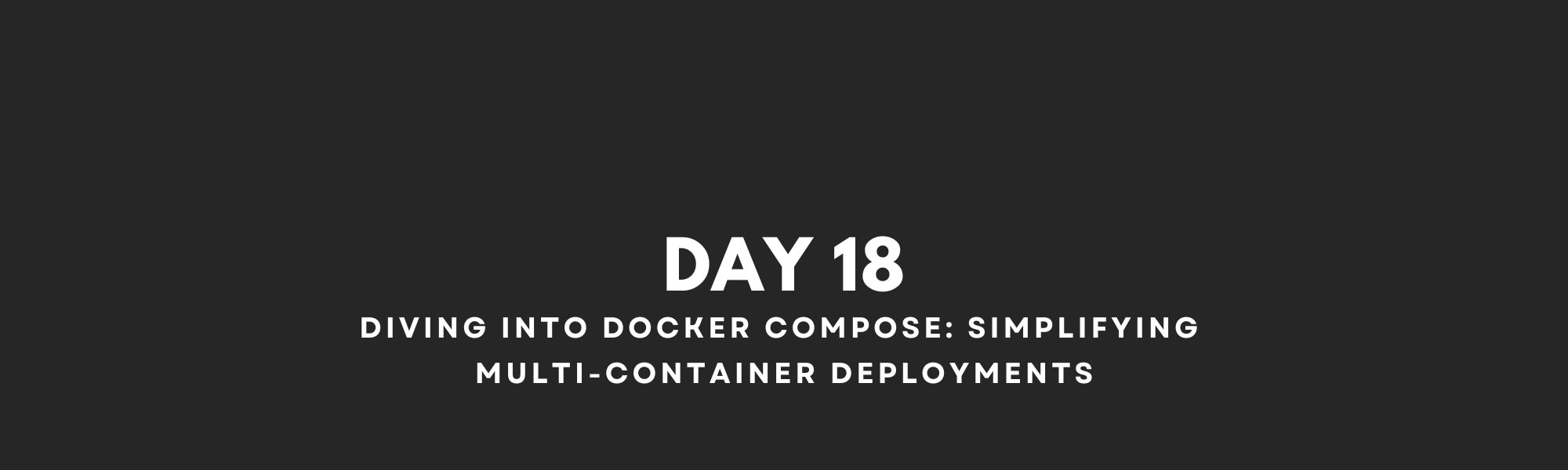
YAML:
YAML (short for "YAML Ain't Markup Language" or sometimes "Yet Another Markup Language") is a human-readable data serialization format. It is often used for configuration files and data exchange between languages with different data structures. YAML files use indentation to represent data hierarchies, making it easy for humans to read and write, while also being machine-readable.
Here are some key features of YAML:
Human-Readable: YAML is designed to be easily readable by humans. It uses indentation and minimal punctuation, such as colons and dashes, to define data structures, which makes it visually clear.
Whitespace Sensitive: YAML uses indentation (typically spaces or tabs) to indicate nesting and hierarchy, similar to how Python uses indentation. Proper indentation is crucial for the correct interpretation of YAML documents.
Data Types: YAML supports various data types, including strings, numbers, booleans, arrays (lists), and dictionaries (maps). It allows you to represent complex data structures and their relationships.
Comments: YAML allows for comments, which start with the
#
character. Comments are used for adding explanatory notes within the configuration file.Inclusion of Other Files: YAML supports the inclusion of other YAML files, which can be helpful for modularizing configurations.
Here's a basic example of YAML syntax:
# This is a YAML comment
key1: value1
key2: value2
nested_key:
- item1
- item2
nested_dict:
sub_key1: sub_value1
sub_key2: sub_value2
In this example:
key1
andkey2
are key-value pairs.nested_key
is a list containing two items.nested_dict
is a dictionary containing key-value pairs.
YAML is commonly used for configuration files in software development, such as Docker Compose files, Kubernetes configuration files (YAML manifests), and configuration files for various programming languages and tools. It's also used in data serialization formats like JSON and XML but with a more human-friendly syntax.
Overall, YAML's simplicity and human-readable nature make it a popular choice for configuration files and data exchange formats in many applications.
Docker Compose:
Docker Compose is a tool for defining and running multi-container Docker applications. It allows you to define your application's services, networks, and volumes in a single, easy-to-read YAML file (docker-compose.yml
). With Docker Compose, you can start and manage multiple containers as a single application, making it easier to develop, test, and deploy complex, multi-container applications.
Here's a detailed breakdown of the Docker Compose file structure:
1. Version: The first line of the docker-compose.yml
file specifies the version of the docker-compose file format being used. It determines which features and options are available. For example:
version: '3.8'
2. Services: The services
section is where you define the containers that make up your application. Each service is a separate container or a group of containers. Here's an example of defining two services: web
and db
:
services:
web:
# Configuration for the web service
db:
# Configuration for the database service
3. Service Configuration: Within each service block, you can configure various aspects of the container:
Image: Specifies the Docker image to use for the service. It can be an official image from Docker Hub or a custom image.
Ports: Defines the ports to map from the container to the host system. For example, to expose port 80 on the container as port 8080 on the host:
ports:
- "8080:80"
- Environment Variables: Allows you to set environment variables for the container. For example:
environment:
MYSQL_ROOT_PASSWORD: mysecretpassword
- Volumes: Specifies the volumes to mount into the container. Volumes allow you to persist data and share files between the host and container. For example:
volumes:
- ./data:/app/data
- Networks: Connects the container to specific Docker networks. This enables communication between containers on the same network. For example:
networks:
- mynetwork
4. Networks: The networks
section allows you to define custom Docker networks that your services can connect to. This can be useful for isolating containers or specifying communication rules.
networks:
mynetwork:
driver: bridge
Some common Docker Compose commands:
docker-compose up
: Build and start containers as defined in thedocker-compose.yml
file.docker-compose up -d
: Build and start containers in the background (detached mode).docker-compose down
: Stop and remove containers, networks, and volumes defined in thedocker-compose.yml
file.docker-compose build
: Build images for services defined in thedocker-compose.yml
file.docker-compose start
: Start containers that are already defined in thedocker-compose.yml
file.docker-compose stop
: Stop containers that are already defined in thedocker-compose.yml
file.docker-compose ps
: List running containers defined in thedocker-compose.yml
file.docker-compose logs
: Display logs from containers defined in thedocker-compose.yml
file.docker-compose exec
: Execute a command in a running container.docker-compose run
: Run a one-off command in a new container.docker-compose pull
: Pull images from the registry as defined in thedocker-compose.yml
file.docker-compose images
: List images used by the services in thedocker-compose.yml
file.docker-compose config
: Validate and view the final configuration after variable substitution in thedocker-compose.yml
file
📝Make sure to run these commands where the docker-compose.yml
file is present.🖊
How to install Docker and run commands without sudo?
1. Install Docker:
You can install Docker on various Linux distributions using the package manager specific to your distribution. Here are examples of some common Linux distributions:
sudo apt-get update
sudo apt-get install docker.io
2. Add Your User to the Docker Group:
To run Docker as a non-root user, you need to add your user to the docker
group. This group is automatically created during Docker installation.
# Add your user to the docker group
sudo usermod -aG docker $USER
If you do not add the user to the docker group, you will get the below error:
After running this command, you'll need to log out and then log back in for the group changes to take effect. You can also use newgrp docker
to activate the group membership without logging out.
3. Reboot the system and Start and Enable the Docker Service:
To ensure Docker starts automatically upon system boot, you can enable and start the Docker service:
# reboot the system to apply the changes
sudo reboot
# Enable the Docker service
sudo systemctl enable docker
# Start the Docker service
sudo systemctl start docker
4. Test Docker as a Non-Root User:
You can verify that you can run Docker as a non-root user by running a simple Docker command without using sudo
:
docker --version
You should see the Docker version information without any permission errors.
TASK 1:
Learn how to use the docker-compose.yml file, to set up the environment, configure the services and links between different containers, and also to use environment variables in the docker-compose.yml file.
Step 2: - Write a docker-compose file.
---
version: "3.3"
services:
web:
image: nginx:latest
ports:
- 80:80
depends_on:
- db
db:
image: mysql
ports:
- 3306:3306
# Replacing the environment: with env_file:
# environment:
# - MYSQL_ROOT_PASSWORD=test@123
env_file:
- ./.env
Here, in this scenario, we have used .env
(hidden file) with a password of the user root of the MySQL database to keep it a secret and we have used that path of file in env.
The file is explained below for more clarity,
version: "3.3": Specifies the version of the Docker Compose file format being used.
Services: This section defines the different services (containers) in your application.
web: This service is defined with the nginx:latest image, which pulls the latest version of the Nginx image from Docker Hub. It maps port 80 on the host machine to port 80 in the container, allowing you to access the Nginx web server.
DB: This service uses the mysql image, which is the official MySQL image from Docker Hub. It maps port 3306 on the host to port 3306 in the container. Additionally, we have created a file with .env in which we have set the password for the root user, which will be used as the root password for the MySQL database and that path of file is added in the compose file.
NOTE: Once the docker-compose.yml
is created you can verify using the yamllint online YAML Validator - https://www.yamllint.com/
Step 3: - Run the docker-compose file
To run the compose file, first of all need to install docker-compose in the system.
sudo apt install docker-compose -y
Once the docker-compose is installed. Run the YAML file as below.
It will pull the latest image which creates a container, which we can check on the web. Once the container is created, we can check it on the web by accessing its port.
This example demonstrates how you can use Docker Compose to define and manage a multi-container application environment.
TASK 2:
Pull a pre-existing Docker image from a public repository (e.g. Docker Hub) and run it on your local machine. Run the container as a non-root user
By using this command, we can pull the image from the docker hub, and run it.
Inspect the container's running processes and exposed ports using the docker inspect command.
Use the docker logs command to view the container's log output.
Use the docker stop and docker start commands to stop and start the container. To Stop container: -
To start the container: -
Use the docker rm command to remove the container when you're done.
To remove the container, first, we need to Kill it, if it is running. Once it is killed, then it can be removed.
Thanks for reading !!
Subscribe to my newsletter
Read articles from Ajay Gite directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
