Day 1 of 60 Days DSA Challenge - Striver's SDE Sheet

5 min read
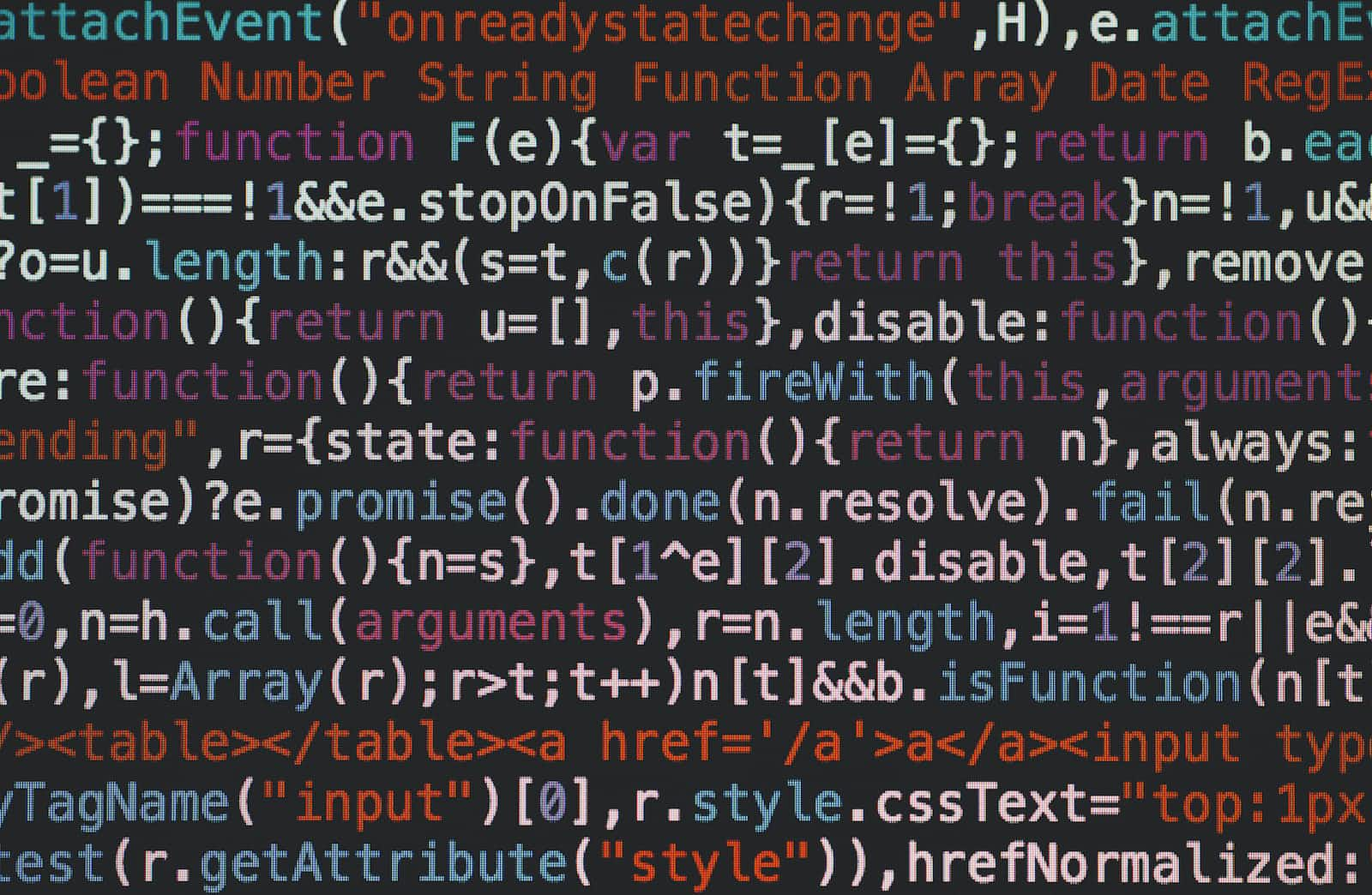
Patterns Problems:
No of Rows === No of Columns ==5
/* Pattern : N= 5 * * * * * * * * * * * * * * * * * * * * * * * * * */ class Main { static void pattern1(int N) { // This is the outer loop which will loop for the rows. for (int i = 0; i < N; i++) { // This is the inner loop which here, loops for the columns // as we have to print a rectangular pattern. for (int j = 0; j < N; j++) { System.out.print("* "); } // As soon as N stars are printed, we move to the // next row and give a line break otherwise all stars // would get printed in 1 line. System.out.println(); } } public static void main(String[] args) { // Here, we have taken the value of N as 5. // We can also take input from the user. int N = 5; pattern1(N); } }
Right Angled triangle with increasing N and left aligned.
/* Pattern : N= 5 * * * * * * * * * * * * * * * */ class pattern2 { static void pattern2(int N) { // This is the outer loop which will loop for the rows. for (int i = 1; i <= N; i++) { // This is the inner loop which here, loops for the columns // no. of columns = row number for each line here. for (int j = 1; j <= i; j++) { System.out.print("* "); } // As soon as N stars are printed, we move to the // next row and give a line break otherwise all stars // would get printed in 1 line. System.out.println(); } } public static void main(String[] args) { // Here, we have taken the value of N as 5. // We can also take input from the user. int N = 5; pattern2(N); } }
Right angled left aligned triangle with numbers of column no.
/* Pattern : N= 5 1 1 2 1 2 3 1 2 3 4 1 2 3 4 5 */ class pattern3 { static void pattern3(int N) { // This is the outer loop which will loop for the rows. for (int i = 1; i <= N; i++) { // This is the inner loop which loops for the columns // no. of columns = row number for each line here. // Here, we print numbers from 1 to the row number // instead of stars in each row. for (int j = 1; j <= i; j++) { System.out.print(j+" "); } // As soon as N stars are printed, we move to the // next row and give a line break otherwise all stars // would get printed in 1 line. System.out.println(); } } public static void main(String[] args) { // Here, we have taken the value of N as 5. // We can also take input from the user. int N = 5; pattern3(N); } }
Right angled left aligned triangle with numbers of row no.
/* Pattern : N= 5 1 2 2 3 3 3 4 4 4 4 5 5 5 5 5 */ class pattern4 { static void pattern4(int N) { // This is the outer loop which will loop for the rows. for (int i = 1; i <= N; i++) { // This is the inner loop which loops for the columns // no. of columns = row number for each line here. // Here, we print numbers equal to the column number for (int j = 1; j <= i; j++) { System.out.print(i+" "); } // As soon as N stars are printed, we move to the // next row and give a line break otherwise all stars // would get printed in 1 line. System.out.println(); } } public static void main(String[] args) { // Here, we have taken the value of N as 5. // We can also take input from the user. int N = 5; pattern4(N); } }
Right angled linverted eft aligned triangle
/* Pattern : N= 5 5 4 3 2 1 4 3 2 1 3 2 1 2 1 1 */ class pattern6 { static void pattern6(int N) { // This is the outer loop which will loop for the rows. for (int i = N; i >= 1; i--) { // This is the inner loop which loops for the columns // no. of columns = (N - row index) for each line here // as we have to print an inverted pyramid. // (N-j) will give us the numbers in a row starting from 1 to N-i. for (int j = i; j >= 1; j--) { System.out.print(j+" "); } // As soon as N stars are printed, we move to the // next row and give a line break otherwise all stars // would get printed in 1 line. System.out.println(); } } public static void main(String[] args) { // Here, we have taken the value of N as 5. // We can also take input from the user. int N = 5; pattern6(N); } }
pattern 7
/* Pattern : N= 5 1 2 3 4 5 1 2 3 4 1 2 3 1 2 1 */ class pattern7 { static void pattern7(int N) { // This is the outer loop which will loop for the rows. for (int i = N; i >=1 ; i--) { // This is the inner loop which loops for the columns // no. of columns = (N - row index) for each line here // as we have to print an inverted pyramid. // (N-j) will give us the numbers in a row starting from 1 to N-i. for (int j = 1; j<= i; j++) { System.out.print(j+" "); } // As soon as N stars are printed, we move to the // next row and give a line break otherwise all stars // would get printed in 1 line. System.out.println(); } } public static void main(String[] args) { // Here, we have taken the value of N as 5. // We can also take input from the user. int N = 5; pattern7(N); } }
0
Subscribe to my newsletter
Read articles from Ankita Dodamani directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
