What are Props and state in React Js?
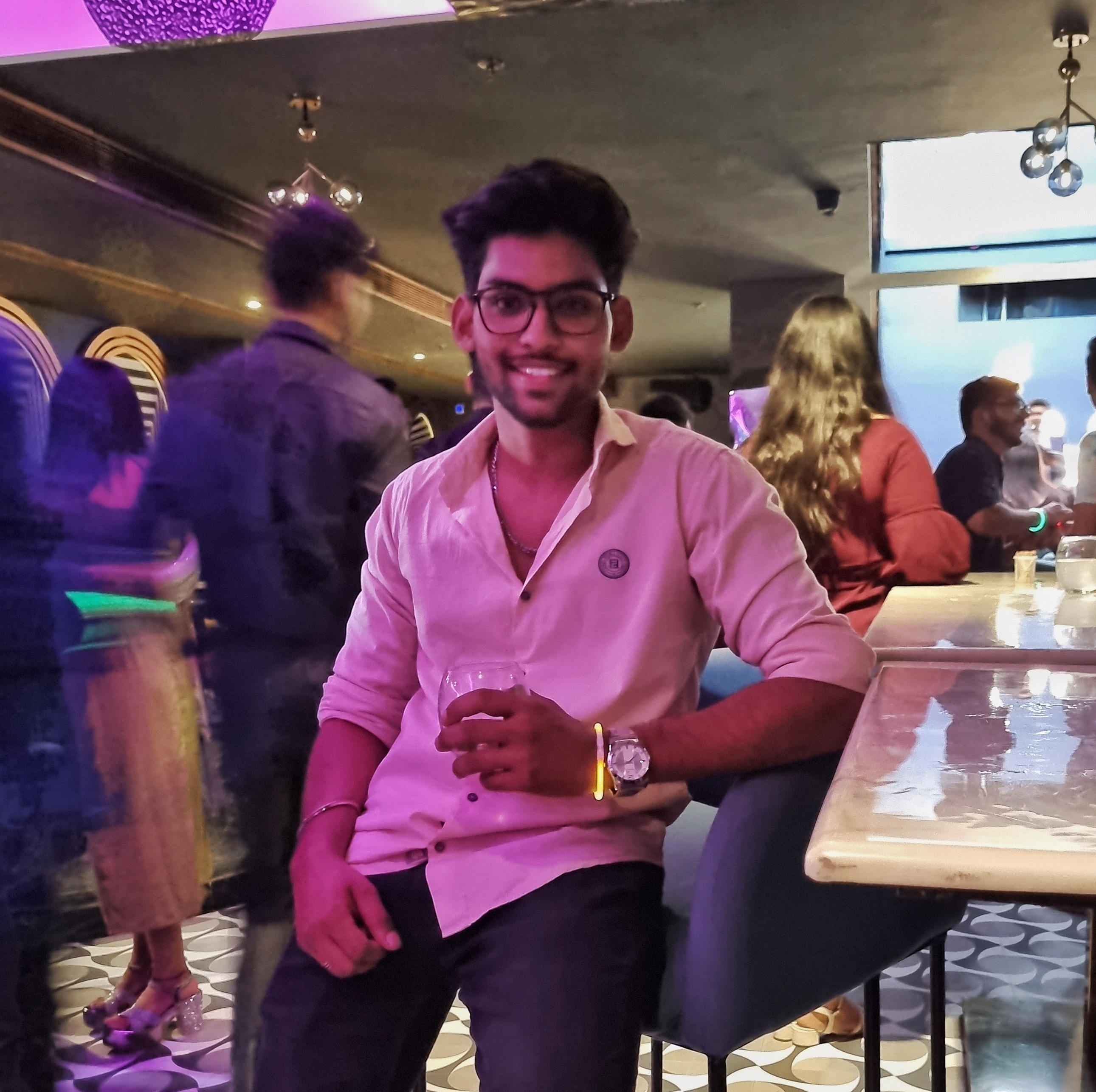
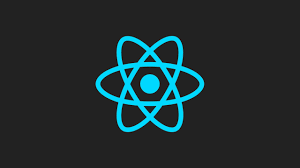
React State and Props
React state and props are two of the most important concepts in React. They are used to manage the data in your React components.
State
State is the data that is managed within a component. It is mutable, meaning that it can be changed. State is used to store data that changes over time, such as the number of items in a shopping cart or the current user's location.
To use state, you need to declare a state variable in your component. This is done by using the state
property.
const [counter, setCounter] = state({
counter: 0,
});
The first argument to the state
function is the initial value of the state variable. The second argument is a function that is called whenever the state variable changes.
To change the state variable, you call the setCounter
function. This function takes a new value for the state variable as its argument.
setCounter(1);
Props
Props are the data that is passed from a parent component to a child component. Props are immutable, meaning that they cannot be changed. Props are used to pass data from one component to another, such as the title of a blog post or the image to be displayed in a card.
To use props, you need to declare a props variable in your component. This is done by using the props
property.
const { title, image } = props;
The props
object contains all of the props that were passed to the component. You can access the props by their name.
When to Use State and Props
State should be used to store data that changes over time. Props should be used to pass data from one component to another.
Here is a table that summarizes the differences between state and props:
Feature | State | Props |
Mutability | Mutable | Immutable |
Scope | Within the component | From parent to child |
Use cases | Storing data that changes over time | Passing data from one component to another |
Conclusion
State and props are two of the most important concepts in React. They are used to manage the data in your React components. By understanding the difference between state and props, you can use them effectively to create dynamic and interactive React applications.
I hope this blog post was helpful. If you have any questions, please feel free to leave a comment below.
Subscribe to my newsletter
Read articles from Gaurav directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
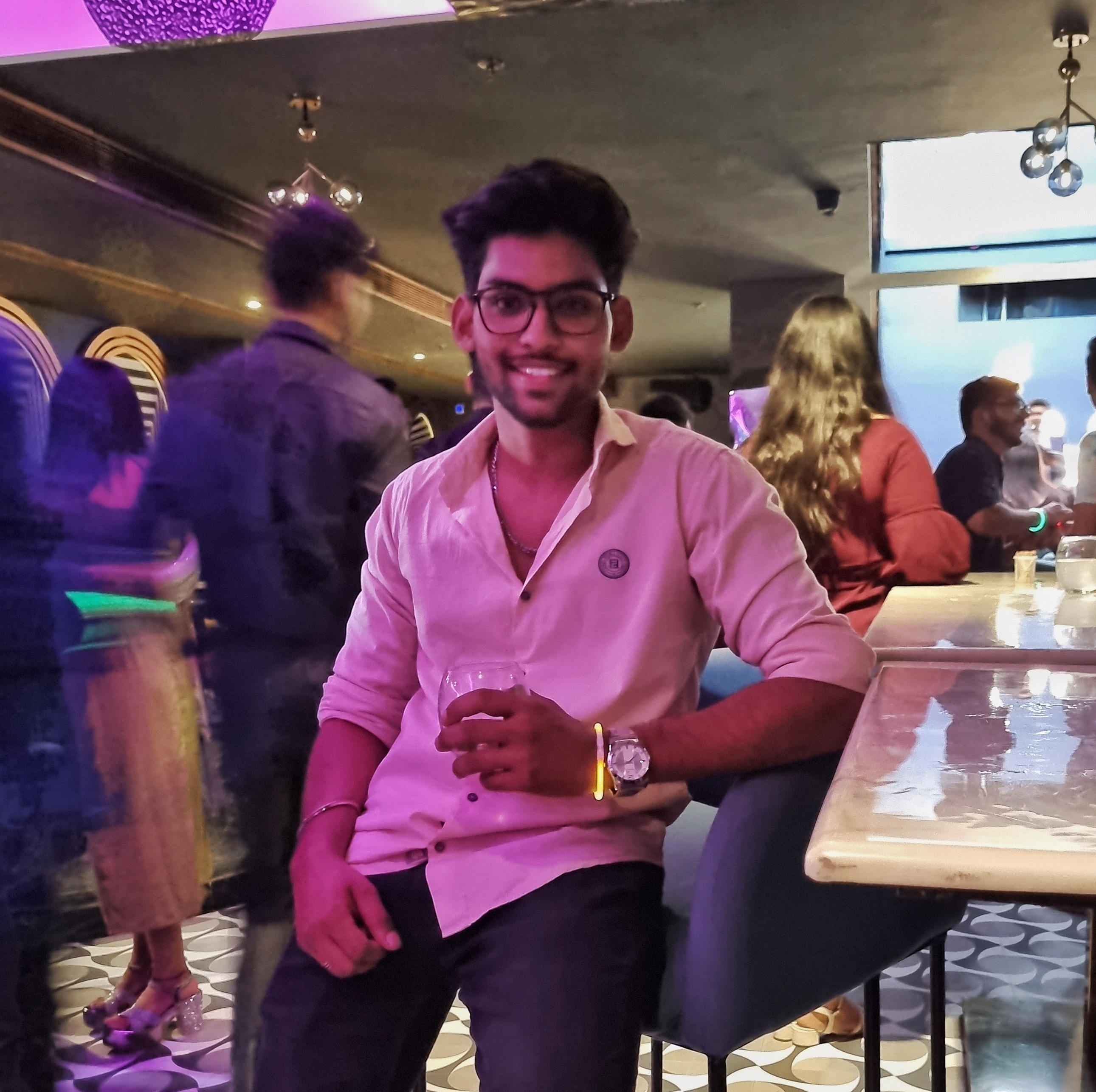
Gaurav
Gaurav
Front-end Developer, UI/UX designer