Unveiling the Power of RPC: Simplifying Distributed Communication
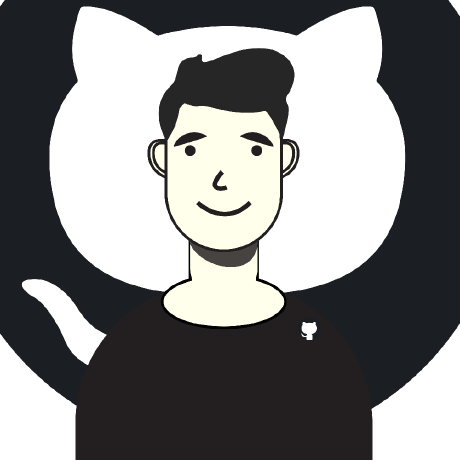
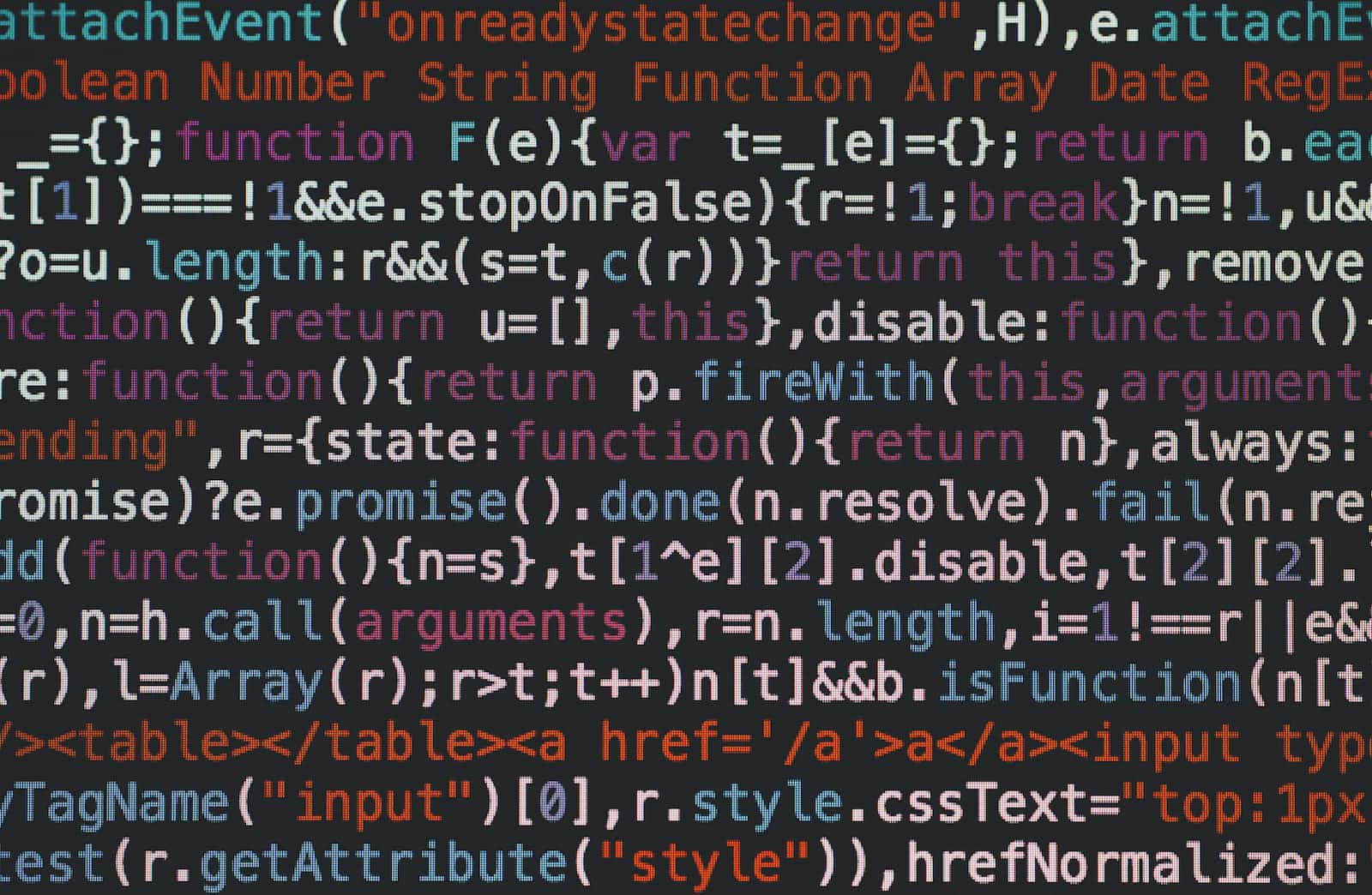
In the ever-expanding realm of modern software development, efficient communication between different parts of a distributed system is nothing short of essential. Picture this: you have multiple computers or software components, scattered across the globe, each with its unique tasks and responsibilities. They need to work together seamlessly, share data, and execute functions as if they were all part of the same program.
Enter RPC or Remote Procedure Call, a fundamental concept that acts as the glue holding this distributed ecosystem together. RPC empowers these distant entities to interact, request services, and exchange information, making them cooperate harmoniously, even when separated by vast distances.
In this blog, we'll embark on a journey to demystify RPC, exploring its core principles, real-world applications, benefits, and challenges. Whether you're an experienced developer looking to deepen your understanding of distributed systems or a newcomer curious about how software components communicate over networks, this exploration of RPC promises valuable insights.
So, fasten your seatbelt as we delve into the world of RPC, unraveling its secrets and unveiling its power to streamline communication in distributed environments. By the end of this journey, you'll have a firm grasp of what RPC is, how it works, and why it's a game-changer in the realm of modern software development.
Let's get started.
What is RPC?
Imagine you're developing a software application, and you need to use a specific function or utility that exists on a different computer, perhaps even on the other side of the internet. This is where RPC, or Remote Procedure Call, comes into play.
RPC is a technology that acts as a bridge, allowing your program to seamlessly communicate with utilities, functions, or services that reside on remote computers or servers. It's like having a magical telephone line that lets your code reach out and ask for help from distant resources.
Here's how it works:
Caller (Your Program): Your program initiates an RPC, just like making a phone call. It says, "Hey, I need to use a specific function or service, but it's not here. Can you do it for me?"
Message (Function Request): Your program tells the remote computer what it wants to do. It's like sending a message that says, "I need you to perform this task for me."
Execution (Remote Procedure): The remote computer receives your request and carries out the task you asked for. It's as if a skilled assistant on the other end follows your instructions.
Response (Result): After the task is completed, the remote computer sends back the result to your program. It's like receiving a reply to your message with the answer or outcome.
RPC is incredibly versatile. It allows your software to tap into a wide range of services and functions, even if they're hosted on different machines or servers. This technology is the backbone of modern distributed systems, enabling everything from web services to cloud computing.
So, next time your program needs a helping hand from a distant resource, remember that RPC is the magic telephone line that makes it all possible, connecting your code with utilities and services over the network.
Example:
In this example, we'll create a basic client-server application where the client requests the server to perform a remote procedure, i.e., adding two numbers.
Here's the Python code for both the client and server:
Server (RPC Provider) - server.py:
import socket
def add_numbers(x, y):
return x + y
def main():
host = '127.0.0.1'
port = 12345
server_socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
server_socket.bind((host, port))
server_socket.listen(1)
print("RPC Server is listening on {}:{}".format(host, port))
while True:
client_socket, addr = server_socket.accept()
print("Connected by", addr)
data = client_socket.recv(1024).decode()
if not data:
break
# Parse the data as a tuple (function_name, arguments)
function_name, args = eval(data)
if function_name == 'add_numbers':
result = add_numbers(*args)
else:
result = "Unknown function"
client_socket.send(str(result).encode())
client_socket.close()
if __name__ == '__main__':
main()
Client (RPC Caller) - client.py:
import socket
def rpc_call(function_name, args):
host = '127.0.0.1'
port = 12345
client_socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
client_socket.connect((host, port))
data = (function_name, args)
client_socket.send(str(data).encode())
result = client_socket.recv(1024).decode()
client_socket.close()
return result
if __name__ == '__main__':
x = 5
y = 3
result = rpc_call('add_numbers', (x, y))
print("Result of {} + {} is: {}".format(x, y, result))
Working Procedure for RPC Model:
RPC (Remote Procedure Call) is a mechanism for invoking functions or procedures on a remote server or service as if they were local, abstracting away the complexities of network communication. Here's how the process works, including stub generation, call receipt, argument handling, and communication:
- Interface Definition:
- RPC starts with defining the interface for the remote service. This interface specifies the available remote procedures, their inputs, and outputs. This can be defined using technologies like Protocol Buffers (protobuf) or IDL (Interface Definition Language).
- Stub Generation:
The next step is to generate code or stubs from the interface definition. These stubs are used by both the client and server to serialize and deserialize data and handle network communication.
Stub generation tools take the interface definition and generate language-specific code for both the client and server. This code includes serialization and deserialization logic, making it easier to send data between the client and server.
- Client Call:
The client, which uses the generated stub, calls a remote procedure as if it were a local function. The client's code is unaware that the function is remote.
When the client makes this call, the stub takes care of packaging the function name and arguments into a message that can be sent over the network.
- Message Serialization:
- The stub serializes the function name and arguments into a message format that can be transmitted over the network. This often involves encoding the data into a binary or text format, depending on the chosen serialization protocol.
- Network Transmission:
- The serialized message is sent over the network to the remote server. This typically involves opening a network socket connection to the server's address and sending the message over this connection.
- Server Receive:
- On the server side, the server's stub receives the incoming message. This stub understands the message format and can extract the function name and arguments.
- Function Execution:
- The server's stub then calls the actual implementation of the remote procedure (the server-side code). It passes the received arguments to this implementation.
- Argument Handling:
- The server-side code processes the received arguments and performs the intended operation, such as addition, on the arguments.
- Response Serialization:
- After the server-side code has executed the function, it returns the result. The result is then serialized into a response message using the same serialization format used for the request.
- Response Transmission:
- The response message is sent back over the network to the client.
- Client Receive:
- On the client side, the client's stub receives the response message, deserializes it, and extracts the result.
- Function Result:
- Finally, the client's code receives the result of the remote procedure call and can continue its execution as if the function had been called locally.
Advantages and Disadvantages of RPC
Advantages:
Abstraction of Network Communication: RPC abstracts away the complexities of network communication, making it easier for developers to build distributed systems. Developers can invoke remote functions or procedures as if they were local, simplifying the development process.
Language Neutrality: RPC allows different parts of a distributed system to be implemented in different programming languages. As long as both the client and server understand the RPC protocol, they can communicate seamlessly.
Code Reusability: RPC promotes code reusability because developers can encapsulate functionality into remote procedures. These procedures can be reused across different parts of the system, reducing code duplication.
Efficiency: RPC can be highly efficient, especially when using binary serialization formats and optimized communication protocols. This efficiency is crucial for high-performance distributed systems.
Interoperability: RPC can be used to integrate systems and services that use different technologies or platforms. By defining a common RPC interface, disparate systems can communicate with each other, fostering interoperability.
Disadvantages:
Complex Error Handling: Error handling in RPC can be complex, especially when dealing with network failures or remote service unavailability. Developers need to implement robust error handling to ensure system reliability.
Performance Overhead: RPC introduces performance overhead due to the serialization and deserialization of data, as well as network communication. In some cases, this overhead can be significant, impacting system performance.
Tight Coupling: RPC can lead to tight coupling between the client and server components. Changes to the interface or implementation of a remote procedure can break existing clients, requiring updates to both sides.
Security Concerns: Ensuring the security of RPC communication can be challenging. Without proper authentication and encryption, sensitive data sent over RPC may be vulnerable to interception or tampering.
Compatibility Issues: Versioning and compatibility between client and server can be challenging in RPC systems. Changes to the interface or behavior of remote procedures must be carefully managed to prevent compatibility issues between different versions of the system.
I hope you learned something from this blog and if yes then please share this blog on your socials and don't forget to join my newsletter for more such content.
Subscribe to my newsletter
Read articles from Vishal Sharma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
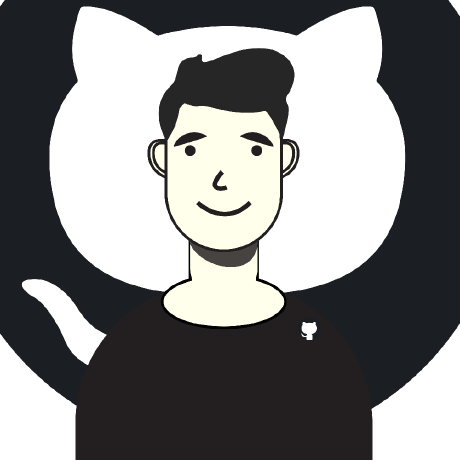
Vishal Sharma
Vishal Sharma
I am a developer from Surat who loves to write about DSA,Web Development, and Computer Science stuff.