Unveiling the power of Vue.js
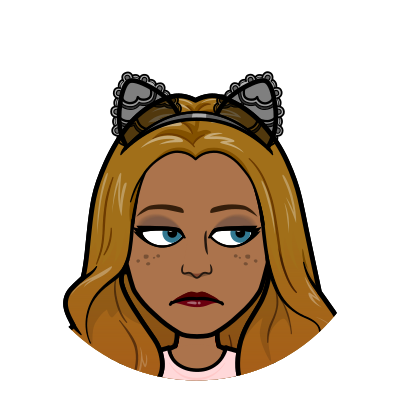
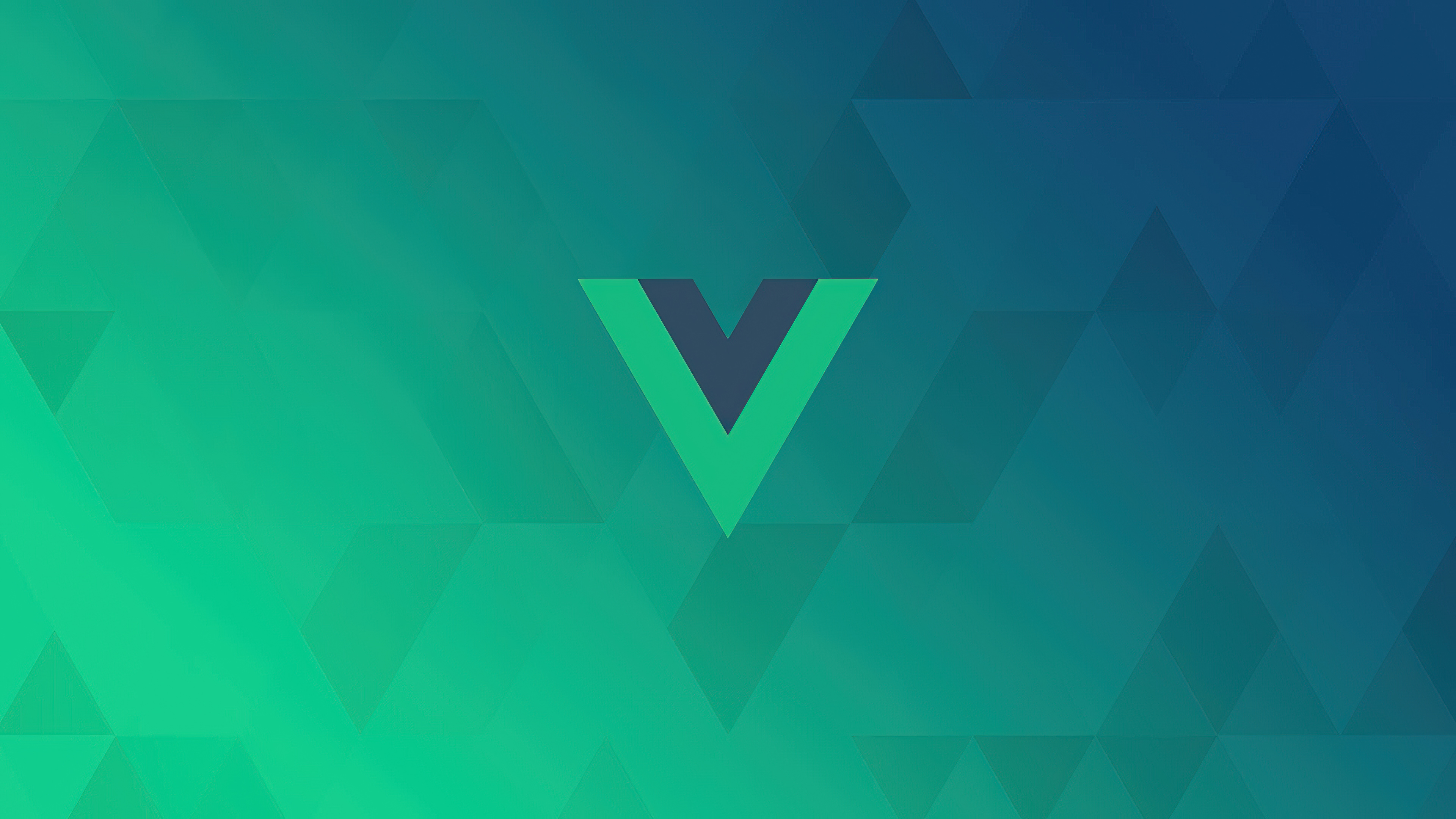
Vue.js, the brainchild of Evan You, has emerged as a compelling front-end framework that seamlessly blends the best of both worlds—resembling Angular's architectural elegance while prioritizing the intuitive templating functions. While at Google, Evan embarked on a journey to develop a framework that offered a fresh perspective on building user interfaces, ultimately giving rise to Vue.js.
In this article, the first installment of our multi-part series, we will delve into the heart of Vue.js—the Options API. We'll take a focused look at key aspects of the Options API that enable you to build dynamic and interactive user interfaces. By the end of this article, you'll have a strong foundation in utilizing the Options API to manage state, control rendering, and harness the component lifecycle within your Vue.js applications.
Introduction to Vue.js and the Options API
At the heart of Vue.js lies the concept of components. These encapsulated and reusable building blocks enable developers to construct complex applications easily. This Options API provides a structured approach to defining component behavior. Its intuitive syntax enables developers to clearly specify how components should respond to user interactions and data changes. The Options API and the Composition API are both approaches provided by Vue.js to structure and manage the logic within components, but they offer different paradigms for achieving this goal. The Options API follows an object-oriented approach. Each Vue component is defined as an object, and the component's options (such as data, methods, computed, etc.) are properties of this object. The Composition API introduces a function-based approach to defining component logic called "composition functions." Developers can organize related logic into reusable functions instead of scattering the logic across multiple option properties.
Options API: Managing State
At the core of any web application lies its state, which encompasses data, user interactions, and the current application state. Managing this state effectively is paramount to delivering a reliable and interactive user experience. Vue's Options API is a fundamental part of the framework that allows developers to define and manipulate the state of their components. It provides a structured way to declare data properties, computed properties, methods, and watchers, all of which play pivotal roles in managing state. By combining these options, Vue provides developers with an easy way to define and manipulate data while ensuring reactivity.
Using data properties
When you need to declare reactive variables in Vue, the first option to consider is usually the data object. The variables you want to use in your component are declared as properties of the data object. Vue will recursively convert all these properties you create in the data object into getters and setters. This is the mechanism that Vue uses to ensure the reactivity of these properties. This simply means that a change in these properties would cause an automatic DOM update.
However, it's essential to be aware of some nuances, particularly when dealing with properties that are objects themselves. For example, suppose you define an object named user
with a property called name
in the data
object. In this case, any new properties added to the user
object after its initial declaration would not trigger reactivity by default. Vue's reactivity system won't automatically track these additions. To make these newly added properties reactive, you must use Vue's $set
method.
Executing methods for interactivity
You might be wondering how to execute functions in Vue. In comes methods in Vue. Methods allow you to declare functions that you can run anywhere else in your Vue code. Methods can be bound to events like click and change in the Vue template or they can be called in other parts of your Vue script using the this
keyword. Based on this, it is important to note that Vue methods should be regular functions and not arrow functions. When you declare a method in a Vue component using a regular function (not an arrow function), Vue automatically binds that method to the Vue instance. If for example, we have a method called addAge
, we can call this.addAge()
in another method to run the addAge function in that method.
In JavaScript, when we use an arrow function, it behaves differently from regular functions when it comes to the this
keyword. With arrow functions, this
is not determined by how or where the function is called; instead, it's determined by the surrounding lexical context, which means the context in which the arrow function is defined/created. In Vue components, methods often need access to the Vue instance itself to interact with data properties, computed properties, and other methods. Vue binds these methods to the Vue instance, ensuring that when you use this
inside a method, it correctly refers to the Vue instance.
Utilizing computed properties
Computed properties are halfway between properties of the data object and methods. You can access them as if they were properties of the data object but they are specified as functions. The difference between computed values and methods is that computed values are cached and will run once except one of its reactive dependencies change but methods will run every single time the method is called. In other words, computed values even if called in multiple places, will run once and use the computed/cached value each time it is called instead of running again. Note that if an unreactive dependency of a computed property is changed, the computed property will not be updated. Another important caveat to note is that you cannot change the value of a computed property the way you would a property of the data object, except you specify a getter and setter in the computed property.
Reacting to data changes with watchers
In Vue, you can watch a property of the data object or a computed property for changes by using watchers. The watch object is an object in the Vue instance that has the value to be watched as a key and the value of that key is the callback function to be executed when there is a change in the key. Note that the callback function to be executed when the watched value changes can also not be an arrow function. Again, for the reasons mentioned above.
Note that watchers can be computationally expensive and the use of them should be minimized. In cases where their usage can be avoided, you could use a computed property with a setter instead.
Options API: Controlling Rendering
Vue options API also provides you a way of controlling what is rendered to the user by using a combination of templates, directives, slots, etc. We will now dive into the toolkit that Vue provides for us to dynamically control rendering in our applications.
Rendering with templates
Templates in Vue are a way of combining HTML with special attributes called directives as well as dynamic data by means of interpolation which together control what Vue will render to the browser.
Interpolation in Vue.js is a mechanism that allows you to inject dynamic data into your templates. It's achieved by wrapping an expression within double curly braces {{ }}
.
Vue directives are special tokens in the markup that tell the library to do something to a DOM element or a group of elements. They are prefixed with v-
to indicate that they are special attributes provided by Vue. Examples are:
Conditional Rendering with
v-if
,v-else
,v-else-if
.Looping with
v-for
.Binding HTML Attributes to dynamic values with
v-bind
.Handling Events with
v-on
.Two-way Data Binding with
v-model
.And many more.
v-on and v-bind have shortcuts that you can use, which are :
for v-bind and @
for v-on. As a rule of thumb, if you are going to use the shortcut, ensure to use it through out the codebase. And if using the long form, use it throughout the code base so as to ensure consistency.
Conditional rendering with v-if and v-show
By using the v-if, v-else-if and v-else directives, you can control what HTML is rendered bases on the result of an operation. If the operation returns a truthy value, then that block of HTML would be rendered to the DOM.
Another way of controlling what is rendered on the DOM is with v-show. While v-if and v-show are quite similar, the difference is that with v-show, the element is actually part of the DOM, it is just hidden but with v-if, the element is actually not rendered to the DOM at all. This means that if a computation for v-if becomes falsy, Vue would destroy the event-listeners, child components and literally everything related to that element and recreate them when the v-if becomes truthy again. Wheras with v-show, it simply hides it from the DOM with CSS and can toggle the CSS when the computation becomes truthy.
Based on this, we can see that v-if has a greater toggling cost. The computational cost of destroying and recreating the element or component whenever the v-if values toggles from truthy to falsy can be reduced by using v-show. Whereas v-show has a higher initial render cost because Vue still has to compile the component as if it were part of the DOM even though it is technically not visible. So, it might be preferable to use v-show if you plan to toggle the visibility of a component often.
List rendering with v-for
Say you have a list or an array of items from your server and you want to render them to you DOM. Vue provides you with the v-for directive that allows you to do this very easily.
When looping through an object, value is the key and name is the value for each object property. When looping through an array, value is the value and name is the index of the item. To access the index in an object, you add a third parameter.
References
Vue Official Documentation - https://vuejs.org/
Vue.js Up and Running - Callum Macrae
Subscribe to my newsletter
Read articles from Laura Eno directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
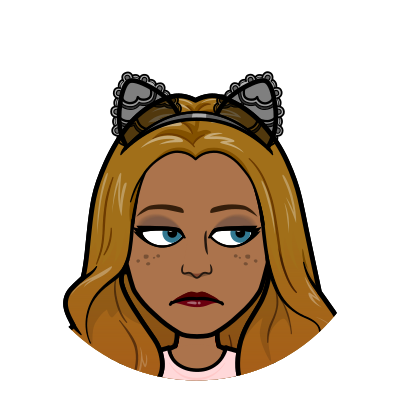